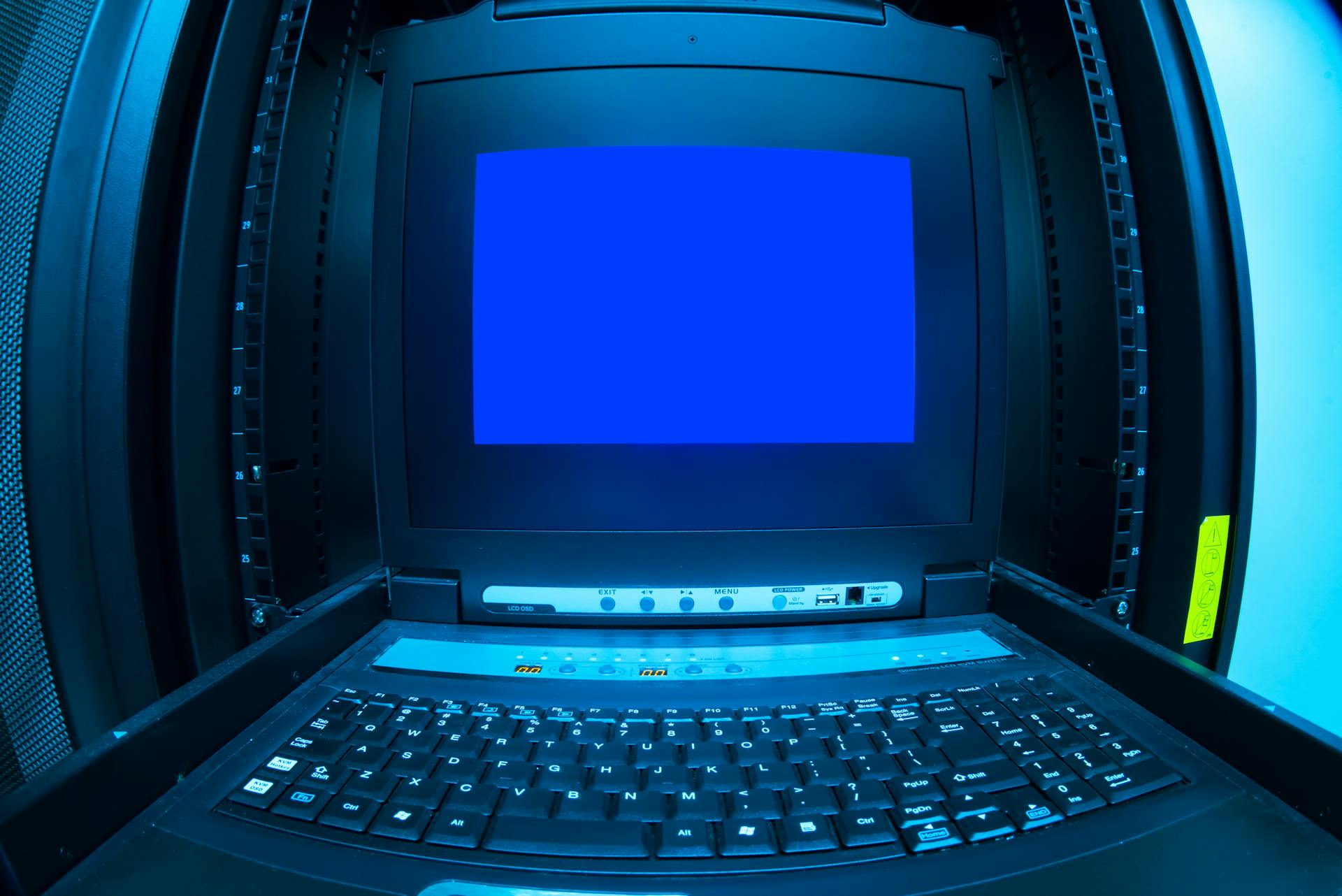
To get started with Azure MSAL Angular, you need to install the necessary packages, including @azure/msal-angular and @azure/msal-browser.
You can then configure the MSAL instance with your Azure AD application's client ID and tenant ID. The client ID is obtained from the Azure AD portal, and the tenant ID is automatically populated in the MSAL configuration.
The next step is to add the MSAL instance to your Angular application's module, typically in the app.module.ts file. This will enable the MSAL library to handle authentication and authorization for your application.
With the MSAL instance configured, you can now use the library's API to authenticate users and obtain access tokens for protected resources. The access token is obtained by calling the acquireTokenSilent function, which returns a promise that resolves to the access token.
Explore further: Azure Msal Browser
Authorization Flow
Authorization Flow is a crucial aspect of Azure MSAL Angular, and it's essential to understand the recommended pattern for web applications using the OpenID Connect authorization code flow. This pattern involves instantiating a confidential client application with a token cache and customized serialization, and then acquiring the token using the authorization code flow.
In public client applications, which aren't trusted to safely keep application secrets, the authentication libraries need to be started. This involves importing the MSAL Angular and MSAL Browser libraries, and configuring the HTTP_INTERCEPTORS and MsalGuardAngular providers. By doing so, you'll ensure that the MSAL initialization includes passing the multiple account public client application object.
Here are the key steps to follow for the authorization flow:
- Instantiate a confidential client application with a token cache and customized serialization.
- Acquire the token using the authorization code flow.
- Start the authentication libraries by importing the necessary libraries and configuring the providers.
By following these steps, you'll be able to implement the authorization flow correctly and ensure secure authentication for your Azure MSAL Angular application.
Authorization Code Flow in Web Apps
Authorization Code Flow in Web Apps is a recommended pattern in controllers for web applications that use the OpenID Connect authorization code flow. This pattern involves instantiating a confidential client application with a token cache and customized serialization.
To implement this pattern, you'll need to acquire a token using the authorization code flow. This involves sending the client's authorization code to the authorization server to obtain an access token.
The recommended steps for instantiating a confidential client application are:
- Instantiate a confidential client application with a token cache.
- Customize the token cache serialization.
Here's a summary of the steps:
App Redirects
App Redirects are a crucial part of the Authorization Flow, and they require some special handling.
You'll need to add the app-redirect directive to your index.html file, specifically in the src folder.
Expand your knowledge: Azure App Insights vs Azure Monitor
Confidential Clients
Confidential clients are a special type of application that can acquire tokens for themselves, not for a specific user.
To acquire tokens for a confidential client, you can use the client credentials flow, which is suitable for syncing tools or tools that process users in general.
For web APIs that need to call an API on behalf of a user, you can use the on-behalf-of (OBO) flow, where the application is identified with client credentials to acquire a token based on a user assertion, such as SAML or a JWT token.
Here are the three main techniques for confidential clients:
- Client Credentials Flow: suitable for syncing tools or tools that process users in general.
- On-Behalf-Of (OBO) Flow: for web APIs that need to call an API on behalf of a user.
- Authorization Code Flow: for web apps after the user signs in through the authorization request URL.
Tokens acquired using the authorization code flow can be cached on a user or on a session basis, but if caching on a user basis, it's recommended to limit the session lifetime.
Discover more: Azure User
Authentication
Authentication is a crucial part of any web application, and Azure MSAL Angular provides a robust solution for authentication and authorization. The recommended call pattern in web apps using the authorization code flow is to instantiate a confidential client application with a token cache and acquire the token using the authorization code flow.
To add authentication components, you'll need to create a folder for each component, update the app.module.ts and app-routing.module.ts files with references to the new components, and create the necessary TypeScript, HTML, CSS, and test files. This will enable you to run your app and test the sign-in experience.
The authentication result exposes various metadata about the access token, including the expiry time, scopes, and user information. The authentication result also includes the access token, ID token, token expiration, tenant ID, and scopes for which the token was issued.
Worth a look: Azure Id
Authentication Results
Authentication results are a crucial part of the authentication process, providing valuable metadata about the access token.
For more insights, see: Azure Auth Json Website Azure Ad Authentication
The authentication result includes the access token for the web API to access resources, which is usually a Base64-encoded JWT, but the client should never look inside the access token.
The result also exposes the ID token for the user, a JWT that contains user information.
The token expiration is another important piece of information, telling the date/time when the token expires.
Additionally, the authentication result contains the tenant ID, which includes the tenant in which the user was found.
The scopes for which the token was issued are also included in the authentication result.
A list of the authentication result components is as follows:
- The access token for the web API to access resources.
- The ID token for the user.
- The token expiration.
- The tenant ID.
- The scopes for which the token was issued.
- The unique ID for the user.
This information allows your app to do intelligent caching of access tokens without having to parse the access token itself.
Start Authentication
To start authentication, you'll need to import the necessary libraries. Import the MSAL Angular and MSAL Browser libraries, as well as the Azure AD B2C configuration module. You'll also need to import HttpClientModule, which is used to call web APIs.
A different take: Azure Msal
The HTTP client is a crucial part of authentication, as it allows your application to securely call web APIs. To use the HTTP client, you'll need to import the Angular HTTP interceptor, which injects the bearer token into the HTTP authorization header.
To configure the HTTP_INTERCEPTORS and MsalGuardAngular providers, you'll need to add the following code to your app.module.ts file. This will enable the MSAL Angular library to handle authentication and authorization.
Here's a step-by-step guide to configuring the HTTP_INTERCEPTORS and MsalGuardAngular providers:
- Import the MSAL Angular and MSAL Browser libraries
- Import the Azure AD B2C configuration module
- Import HttpClientModule
- Import the Angular HTTP interceptor
- Add the essential Angular materials
- Instantiate MSAL using the multiple account public client application object
By following these steps, you'll be able to start authentication in your Angular application.
Here's a summary of the necessary imports:
- MSAL Angular
- MSAL Browser
- Azure AD B2C configuration module
- HttpClientModule
- Angular HTTP interceptor
- Essential Angular materials
App Setup
To set up your Azure MSAL Angular app, start by importing the necessary libraries in your app.module.ts file. This includes MSAL Angular and MSAL Browser libraries, as well as the Azure AD B2C configuration module. You'll also need to import HttpClientModule for calling web APIs.
You'll need to register an application in Azure AD to get your clientId, which is a prerequisite for using @azure/msal-angular.
Here are the essential steps to set up your app:
- Import MSAL Angular and MSAL Browser libraries.
- Import Azure AD B2C configuration module.
- Register an application in Azure AD to get your clientId.
App Project
To create an Angular app project, you can use an existing one or start from scratch. Run the following commands to create a new project.
To create a new Angular workspace with a routing module, you'll need to install the Angular CLI using npm. Then, create a new workspace with a specific app name, such as msal-angular-tutorial.
You'll need to change to the app directory folder to continue with the project setup. This is done using the command line.
Here are the essential steps to create an Angular app project:
- Install the Angular CLI using npm.
- Create an Angular workspace with a routing module, specifying the app name.
- Change to the app directory folder.
Installation
To set up your app, you'll need to install the necessary dependencies. The @azure/msal-angular package is available on NPM.
You can install the MSAL Browser and MSAL Angular libraries by running a command in your command shell. This command will get you started with the authentication process.
To install the MSAL Angular library, you'll need to use the following command: npm install @azure/msal-angular. This will add the necessary library to your project.
The @azure/msal-angular package is a popular choice for authentication, and it's widely used in the industry. It provides a lot of features and functionality out of the box.
Here are the steps to install the MSAL Browser library:
- Run the following command in your command shell: npm install @azure/msal-browser
App Setup
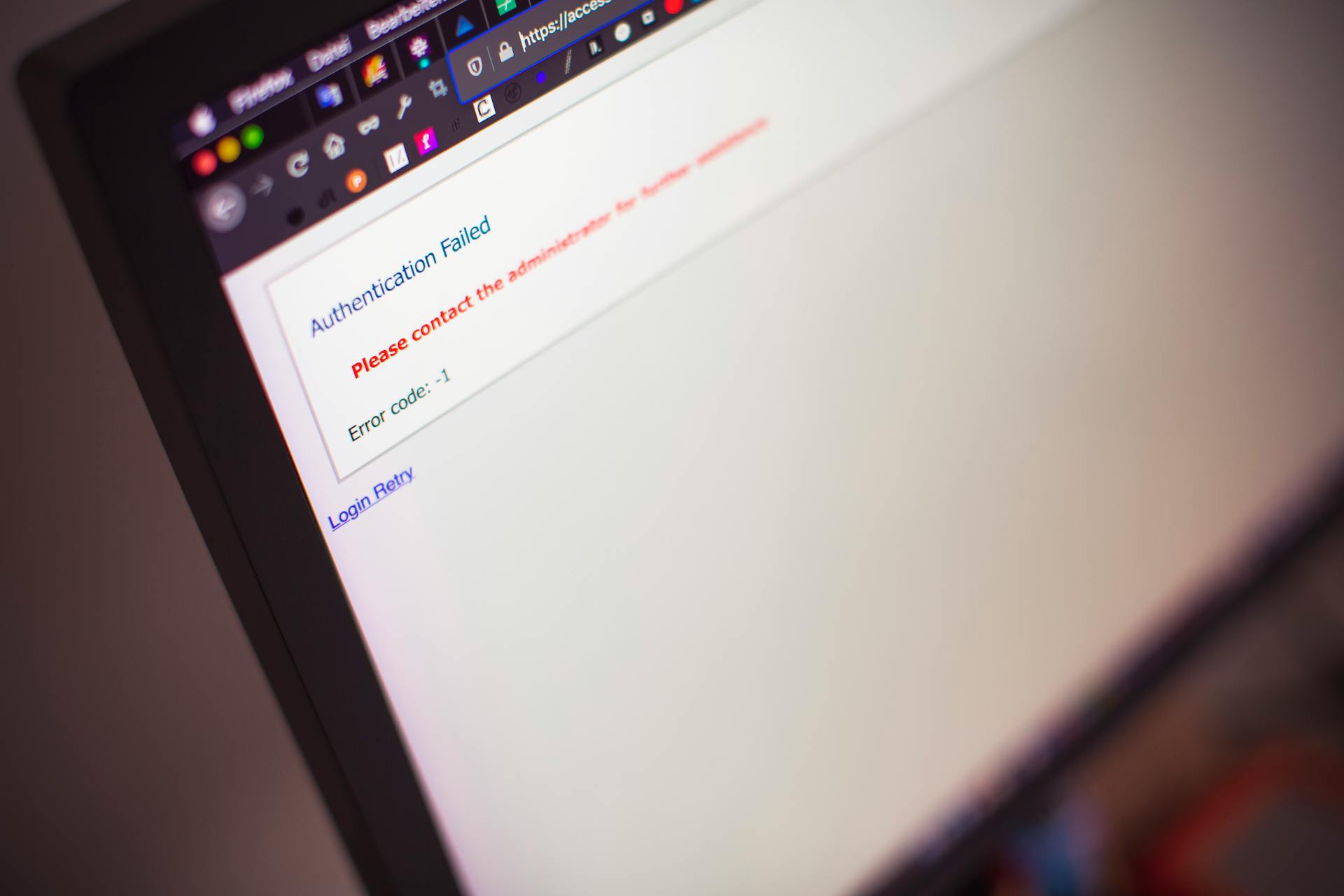
To set up your app, you'll need to create a folder for each component, such as auth-config.ts, app.module.ts, app-routing.module.ts, app.component.* and webapi.component.*. Each folder contains the necessary TypeScript, HTML, CSS, and test files.
You'll also need to update the app.module.ts and app-routing.module.ts files with references to the new components. This will enable navigation and protect components with MSAL Guard.
To run your app, you'll need to create a folder for each component and update the app.module.ts and app-routing.module.ts files. This will allow you to test the sign-in experience.
Here's a list of the components you'll need to create:
- auth-config.ts: This configuration file contains information about your Azure AD B2C identity provider and the web API service.
- app.module.ts: This is the root module that's used to bootstrap and open the application.
- app-routing.module.ts: This component enables navigation by interpreting a browser URL and loading the corresponding component.
- app.component.*: The ng new command created an Angular project with a root component.
- home.component.*: This component demonstrates how to check whether a user has signed in.
- profile.component.*: This component demonstrates how to read the ID token claims.
- webapi.component.*: This component demonstrates how to call a web API.
By following these steps, you'll be able to set up your app and start testing the sign-in experience.
Running Tests
When setting up your app, running tests is a crucial step to ensure everything is working as expected. The @azure/msal-angular library uses jest to run unit tests and coverage.
You can use jest to run unit tests and coverage, just like @azure/msal-angular does. This helps you catch bugs early and improve the overall quality of your app.
Running tests also helps you identify and fix issues quickly, which is essential for a smooth user experience.
Here's an interesting read: Azure Logic App Blob Storage Trigger
Frequently Asked Questions
What is the difference between MSAL Angular v2 and v3?
MSAL Angular v2 and v3 differ in their supported Angular versions, with v3 supporting newer versions (15-18) and v2 supporting older versions (9-14)
Sources
- https://learn.microsoft.com/en-us/entra/identity-platform/msal-acquire-cache-tokens
- https://learn.microsoft.com/en-us/entra/identity-platform/reference-v2-libraries
- https://azuread.github.io/microsoft-authentication-library-for-js/ref/modules/_azure_msal_angular.html
- https://learn.microsoft.com/en-us/azure/active-directory-b2c/enable-authentication-angular-spa-app
- https://snyk.io/advisor/npm-package/@azure/msal-angular
Featured Images: pexels.com