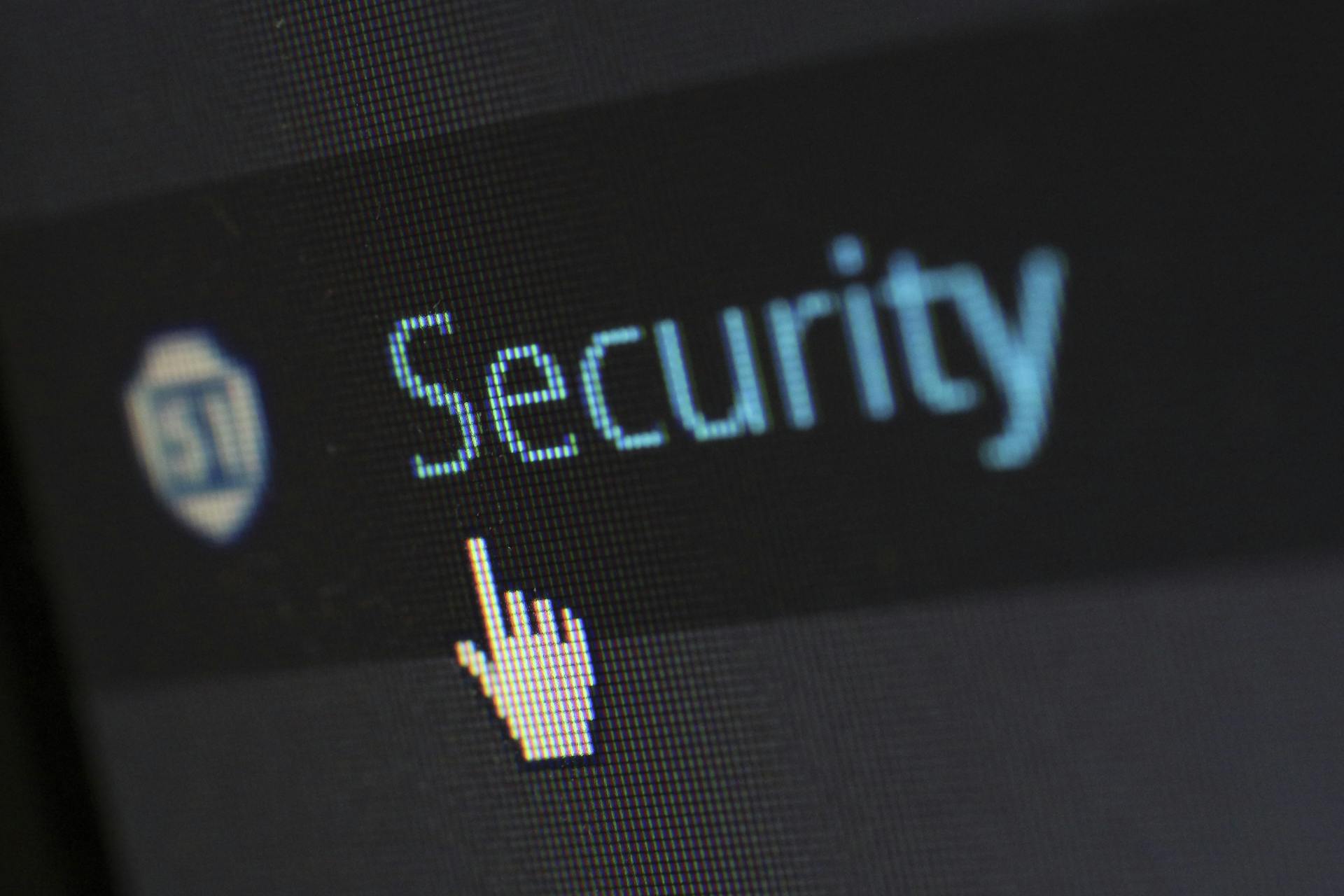
Azure Msal is a popular authentication library that helps developers secure their applications by providing a simple and efficient way to authenticate users. It's widely used in many applications.
Azure Msal uses the OAuth 2.0 authorization framework to authenticate users. This framework is an industry standard for authentication.
To use Azure Msal, you need to register your application in Azure Active Directory. This is where you'll get your client ID and client secret, which are essential for authentication.
With Azure Msal, you can authenticate users using various account types, including personal accounts, work and school accounts, and Azure Active Directory accounts.
Curious to learn more? Check out: Azure Clientid
Prerequisites
To get started with Azure MSAL, you first need to register your application with the Microsoft identity platform. This will give you the necessary information to integrate your app with the platform.
You'll need to obtain the following details from the app registration page in the Microsoft Entra admin center:
- Application (client) ID: a string representing a GUID
- Directory (tenant) ID: provides identity and access management (IAM) capabilities to applications and resources used by your organization
These two pieces of information are crucial for setting up your Azure MSAL application.
You'll also need to determine the identity provider URL (instance) and the sign-in audience for your application. These two parameters are collectively known as the authority.
Additionally, you may need to set the Redirect URI where the identity provider will contact back your application with the security tokens. This is particularly important for web apps and public client apps that use a broker.
Recommended read: Identity Provider Azure
Single Sign-On (SSO)
Single Sign-On (SSO) is a feature that allows users to access multiple applications with a single set of credentials. To enable SSO between browser tabs for the same app, set the cacheLocation in MSAL.js configuration object to localStorage.
This allows application instances in different browser tabs to share the same MSAL cache, thus sharing the authentication state between them. You can also use MSAL events for updating application instances when a user logs in from another browser tab or window.
MSAL.js relies on a session cookie set on the Microsoft Entra domain to provide SSO for the user between different applications. The ssoSilent method is used to sign-in the user and obtain tokens without an interaction.
Here's an interesting read: Azure Msal Browser
To improve performance and ensure the authorization server will look for the correct account session, you can pass one of the following options in the request object of the ssoSilent method to obtain the token silently:
- login_hint, which can be retrieved from the account object username property or the upn claim in the ID token
- Session ID, sid, which can be retrieved from idTokenClaims of an account object
- account, which can be retrieved from using one the account methods
It's recommended to use the login_hint option, as it's the most reliable account hint for silent and interactive requests.
Single Sign-On Across Apps
MSAL.js relies on a session cookie set on the Microsoft Entra domain in the browser to provide Single Sign-On (SSO) for users between different applications.
The ssoSilent method in MSAL.js allows users to sign-in and obtain tokens without interacting with the application, making it a convenient option for SSO.
However, if a user has multiple user accounts in a session with Microsoft Entra ID, they'll be prompted to pick an account to sign in with.
There are two ways to achieve SSO using the ssoSilent method, which can be used depending on the specific use case.
Related reading: Azure Msal Angular
With User Hint
To improve performance and ensure that the authorization server will look for the correct account session, you can pass one of the following options in the request object of the ssoSilent method to obtain the token silently.
You can use the login_hint option, which can be retrieved from the account object username property or the upn claim in the ID token. If your app is authenticating users with B2C, you should see: Configure B2C user-flows to emit username in ID tokens.
The login_hint option is the most reliable account hint for silent and interactive requests. It provides a hint to Microsoft Entra ID about the user account attempting to sign in.
You can also use the sid option, which can be retrieved from idTokenClaims of an account object. However, this option is not as reliable as login_hint.
Alternatively, you can use the account option, which can be retrieved from using one of the account methods. This option is also not as reliable as login_hint.
Take a look at this: Azure App Id
Here are the options you can use to pass in the request object:
- login_hint: retrieved from the account object username property or the upn claim in the ID token
- sid: retrieved from idTokenClaims of an account object
- account: retrieved from using one of the account methods
It's recommended to use the login_hint option as it is the most reliable account hint for silent and interactive requests.
Configure
To configure Azure AD B2C with MSAL, you'll need to register your application in Azure AD B2C and create a client ID.
You can do this by going to the Azure portal, navigating to Azure AD B2C, and clicking on "App registrations".
The client ID will be used in your application code to authenticate users.
Make sure to save the client ID securely, as you'll need it later.
To configure the authentication settings, you'll need to specify the tenant ID, client ID, and the redirect URI.
The tenant ID can be found in the Azure portal under Azure AD B2C, and the client ID is the one you registered earlier.
The redirect URI is the URL that users will be redirected to after they've authenticated.
You can also configure the authentication settings to use a custom policy, which allows for more complex authentication scenarios.
Custom policies can be created and managed through the Azure portal under Azure AD B2C.
See what others are reading: How to Connect to Aks from Azure Portal
Sharing Authentication State Between ADAL.js and MSAL.js
To share authentication state between ADAL.js and MSAL.js, you'll need to ensure both libraries are using localStorage for caching tokens. Set the cacheLocation to localStorage in both the MSAL.js and ADAL.js configuration at initialization.
MSAL.js reads the ID token representing the user's session in ADAL.js cache, making the migration from ADAL.js to MSAL.js easier.
To take advantage of this feature, you'll need to set the cacheLocation to localStorage in both the MSAL.js and ADAL.js configuration at initialization. This allows MSAL.js to share authentication state with ADAL.js.
By using localStorage for caching tokens, you can share authentication state between ADAL.js and MSAL.js, making the migration process smoother.
You might like: Azure Auth Json Website Azure Ad Authentication
Next Steps
Now that you've set up Azure MSAL, it's time to think about the next steps.
You'll want to explore the MSAL.js prompt behavior, which will help you understand how users interact with your application.
To take your application to the next level, consider adding support for user sign-in and authorized API access. This will allow you to acquire an access token to access an API on behalf of the user.
Here are some key areas to focus on:
- MSAL.js prompt behavior
- Optional token claims
- Configurable token lifetimes
- Sign-in and sign-out
- Acquire a token
Installation and Setup
To get started with Azure MSAL, you'll first need to install and set it up in your project. This involves installing the MSAL Node package via npm.
You can install the package by running the following command in your terminal: install MSAL Node package via npm:
After installation, import MSAL Node in your code by adding the following line: After that, import MSAL Node in your code:
Update App Registration
Updating your app registration is a crucial step in the migration process from ADAL to MSAL.
To ensure a smooth transition, you'll need to switch to the Azure AD v2.0 endpoint, which is the recommended endpoint for MSAL.
First, uninstall the ADAL Node package from your project to remove any unnecessary dependencies.
Remove any references to ADAL in your code to prevent conflicts with MSAL.
By following these steps, you'll be able to update your app registration settings and take advantage of the latest features and improvements in MSAL.
If this caught your attention, see: Azure App Insights vs Azure Monitor
Install and Import
To get started with installation and setup, you'll first need to install and import MSAL.
This is a crucial step that lays the foundation for the rest of the process.
To install MSAL, you'll need to use npm, the Node package manager. You can do this by running the following command in your terminal: install MSAL Node package via npm:
Once the installation is complete, you'll need to import MSAL in your code. This can be done by adding the following line: After that, import MSAL Node in your code:
Initialize
To initialize MSAL, you have two alternatives depending on the type of application you're building. If you're creating a mobile app or desktop app, you instantiate a PublicClientApplication object, which expects a configuration object with the clientId parameter at the very least.
In MSAL Node, if you don't specify the authority URI, it defaults to https://login.microsoftonline.com/common, allowing users to sign in with any Microsoft Entra organization or a personal Microsoft account. However, if you want to restrict login to any Microsoft Entra account, use https://login.microsoftonline.com/organizations instead.
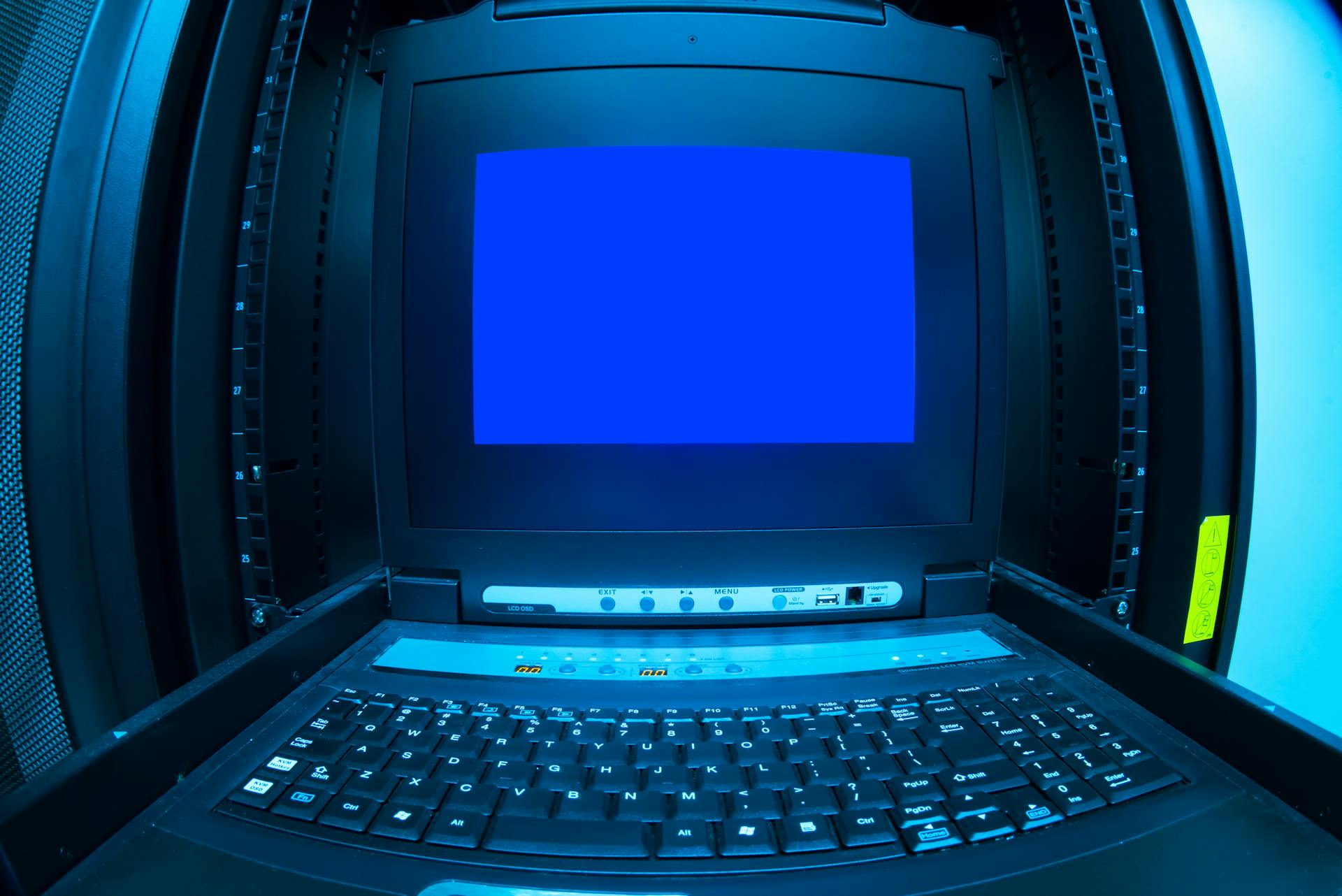
For web apps or daemon apps, you instantiate a ConfidentialClientApplication object, which requires a client credential like a client secret or a certificate. This is different from ADAL's AuthenticationContext, which isn't bound to a client ID.
To instantiate a public client application from code, you can use the following example code, which signs in users in the Microsoft Azure public cloud with their work, school, or personal Microsoft accounts. This is a straightforward way to get started with MSAL.
Alternatively, you can instantiate a public client application from a configuration object, which can be filled in programmatically or read from a configuration file. This approach is useful when you need to customize the application's behavior or read settings from a file.
For confidential client applications, you can use a client secret, but in production, certificates are recommended for their added security. You can create and upload certificates to the Microsoft Entra admin center, and then use them to instantiate the application.
You can also instantiate a confidential client application from a configuration object, adding other parameters using .WithXXX modifiers. This example uses .WithCertificate, demonstrating how to add custom settings to the application.
Explore further: Invalid Client Secret Provided Azure
Use Promises
Using promises instead of callbacks is a game-changer in MSAL Node. In this library, promises are used instead of callbacks.
You can use the async/await syntax that comes with ES8, making your code look cleaner and easier to read.
Enable Token Caching
To enable token caching, you need to configure your MSAL Node instance to use an in-memory token cache by default, which is exposed as part of the ConfidentialClientApplication and PublicClientApplication classes.
MSAL Node uses an in-memory token cache by default, so you don't need to explicitly import it.
However, if you were using ADAL Node previously, you won't be able to transfer your previous token cache to MSAL Node since cache schemas are incompatible.
You can still use valid refresh tokens your app obtained previously with ADAL Node in MSAL Node, but you'll need to see the section on refresh tokens for more.
To write your cache to disk, you can provide your own cache plugin that implements the interface ICachePlugin.
Worth a look: Azure Data Factory Oauth2 Token
An example cache plugin can be implemented as shown in the article section example, where you create a cache plugin class that implements the ICachePlugin interface.
For public client applications like desktop apps, you can use the Microsoft Authentication Extensions for Node to perform cross-platform token cache serialization and persistence, which is supported on Windows, Mac, and Linux.
However, this is not recommended for web applications, as it may lead to scale and performance issues, and instead, you should persist the cache in session.
Remove Refresh Token Logic
You can ditch the logic around refresh tokens, as MSAL Node handles refreshing tokens for you. This means you don't need to build your own logic to handle token refresh.
MSAL Node doesn't expose refresh tokens for security reasons, so you won't find them in your code. Instead, use the acquireTokenByRefreshToken method to get a new set of tokens.
You can still use your previously acquired refresh tokens from ADAL Node's cache to get a new set of tokens. This is done using the acquireTokenByRefreshToken method.
Destroy the older ADAL Node token cache once you've utilized the still valid refresh tokens to get a new set of tokens using MSAL Node.
Suggestion: Azure Token
Frequently Asked Questions
What is the difference between MSAL and identity library in Azure?
The main difference between MSAL and the Azure Identity library is that MSAL is designed for general resources, while Azure Identity is specifically designed for use with other Azure SDK resources. If you're working with Azure SDK resources, use Azure Identity for a streamlined experience.
What is the msal package?
The msal package, or Microsoft Authentication Library, is a tool for developers to implement secure authentication in applications. It simplifies the integration of security protocols, supporting methods like OpenID Connect and JWT.
What is the difference between MSAL and Graph API?
MSAL (Microsoft Authentication Library) and Graph API are two distinct tools that work together to enable developers to access Azure AD and Microsoft services, with MSAL handling authentication and Graph API providing a single endpoint for accessing various APIs. By using both, developers can unlock advanced features like passwordless and Conditional Access.
Sources
- https://learn.microsoft.com/en-us/entra/identity-platform/msal-national-cloud
- https://learn.microsoft.com/en-us/entra/identity-platform/msal-js-sso
- https://learn.microsoft.com/en-us/entra/identity-platform/reference-v2-libraries
- https://learn.microsoft.com/en-us/entra/identity-platform/msal-node-migration
- https://learn.microsoft.com/en-us/entra/msal/dotnet/getting-started/initializing-client-applications
Featured Images: pexels.com