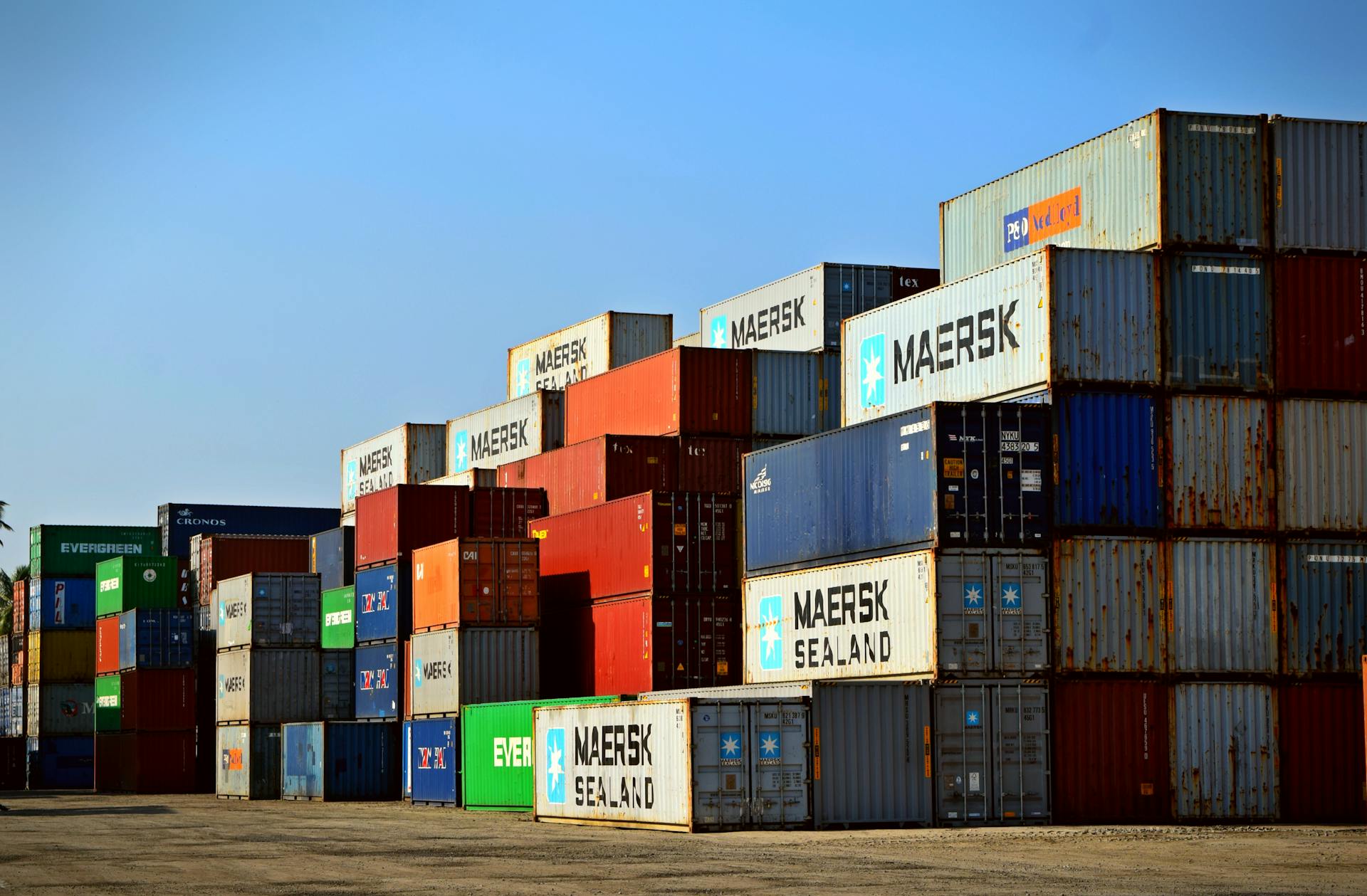
Azure Storage offers a highly scalable and durable object store, which is perfect for storing large amounts of unstructured data.
To get started with Azure Storage in Django, you'll need to install the Azure Storage SDK for Python and configure your Django project to use it.
The Azure Storage SDK for Python provides a simple and intuitive API for interacting with Azure Storage services, making it easy to integrate with your Django application.
You can use the Azure Storage Blob service to store and serve files, and the Azure Storage Queue service to handle tasks and messages.
Broaden your view: Activar Vpn Google One
Azure Storage Setup
To set up Azure Storage for your Django project, start by installing the necessary packages from the project directory using the pip install command. This includes the azure-identity package for passwordless connections to Azure services.
You'll also need to decide between using the Azure Blob Storage client libraries or the Azure Storage Emulator, Azurite. Azurite is a local emulator for Azure Storage accounts that allows for local testing and development without an Azure account.
On a similar theme: Google Project on Drive
To use Azurite, download and install it from the Azurite page, then copy the default connection string and set it in your settings. Note that Django Storages won't create containers if they don't exist, so you'll need to create any storage containers using the Azurite CLI or the Azure Storage Explorer.
To connect your app code to Azure using DefaultAzureCredential, make sure you're authenticated with the same Microsoft Entra account you assigned the role to on your storage account. You can authenticate via the Azure CLI, Visual Studio Code, or Azure PowerShell.
Here's a summary of the steps to authorize data access with the storage account access key:
- Sign in to the Azure portal.
- Locate your storage account.
- In the storage account menu pane, under Security + networking, select Access keys.
- Select Show keys and copy the Connection string value.
Remember to always be careful to never expose the keys in an unsecure location, and to update the storage account name in the URI of your BlobServiceClient object.
Install the Packages
To install the packages for Azure Storage, navigate to your project directory and use the pip install command.
You'll need to install the azure-identity package for passwordless connections to Azure services.
From the command line, run pip install azure-identity to install the package.
This will enable you to connect to Azure services without a password.
After installing the azure-identity package, you can install the Azure Blob Storage client library using pip install azure-storage-blob.
Broaden your view: Connections - Oracle Fusion Cloud Applications
Configure Connection String
To configure your Azure Storage connection string, you'll need to set up an environment variable in Windows. This is where you'll store the connection string securely.
The connection string is used to construct a service client object, which is essential for interacting with your Azure Storage account. You can retrieve the connection string from the environment variable using the code below.
To add the environment variable, follow the same steps as in Example 5. This will ensure that your connection string is secure and not accidentally exposed.
Broaden your view: How to Get Azure Blob Storage Connection String
Uploading and Storing Files
You can dynamically upload files to Azure Storage with each incoming request. This is a great way to handle file uploads in your Django application.
Storing both Static and Media files in the cloud is a good idea. It's more efficient to serve your static files from a remote storage instead of from your web server.
To upload files to Azure Storage, you can use the upload_blob method. This method creates a text file in the local data directory to upload to the container.
Storing your media files in the cloud opens up the possibilities for scaling your web application in the future. If you upload user files to the web server, it can cause issues when trying to scale horizontally with multiple web servers.
You can verify that the resources were created correctly before deleting them. This is a good practice to follow when working with Azure Storage.
By storing your files at a remote storage, you can enable horizontal scaling. This is a must if you want to scale your web application in the future.
You can upload files to Azure Storage and confirm that they were uploaded successfully. This can be done by searching for the file in the Azure portal.
Additional reading: Backblaze Server Backup
Configuration and Settings
In Django 4.2, configuring file storage objects has become easier, allowing for independent configuration and the ability to add multiple storage backends.
You can now pass configuration options under the key OPTIONS, whereas in Django < 4.2, you'd need to subclass the backend because the settings were global.
To configure multiple storage objects, you can define a dictionary with the storage backends, similar to this: {'default': {'BACKEND': 'django.core.files.storage.FileSystemStorage', 'OPTIONS': {'location': '/path/to/media'}}, 'azure': {'BACKEND': 'storages.backends.azure_storage.AzureStorage', 'OPTIONS': {'account_name': 'your_account_name', 'account_key': 'your_account_key'}}}.
You might like: How to save Multiple Photos from Dropbox to Camera Roll
Configuration and Settings
Django 4.2 changed the way file storage objects are configured, making it easier to independently configure storage backends and add additional ones.
In Django >= 4.2, you'd define multiple storage objects by passing them under the key OPTIONS in the settings file. For example, to save media files to Azure, you'd define a dictionary with the storage backend and its options.
On the other hand, in Django < 4.2, you'd subclass the backend to configure multiple storage objects. Subclassing is still an option, but it's no longer required.
The settings documented in this section include both the key for OPTIONS and the global value. Given the significant improvements provided by the new API, migration to Django 4.2 is strongly encouraged.
To configure storage backends and add additional ones, you can use the OPTIONS key in the settings file. This allows you to define multiple storage objects with different settings.
For example, to save static files on Azure via collectstatic, you'd include the staticfiles key in the STORAGES dictionary. This key should be at the same level as the default storage backend.
In Django >= 4.2, you can also use the OPTIONS key to configure the storage backend. This key should be a dictionary with the storage backend and its options.
The OPTIONS key is a powerful tool for configuring storage backends and adding additional ones. By using it, you can take advantage of the new API in Django 4.2.
Curious to learn more? Check out: New Google Drive Shortcuts
Filename Restrictions
When working with Azure files, it's essential to be mindful of their filename restrictions.
Azure file names can't end with a dot (.) or slash (/). This is to prevent potential issues with file identification and navigation.
You should also be aware that Azure file names can't contain more than 256 slashes (/). This helps maintain a clean and organized file structure.
Be sure to keep your file names concise, as they can't be longer than 1024 characters. This will help prevent errors and ensure smooth file operations.
Security and Authentication
Security and authentication are crucial aspects of Azure Storage, especially when integrating it with Django. You can protect your data by implementing best practices such as using a restrictive bucket policy to deny all actions on your storage objects unless the request comes from your domain.
To secure your data in transit, enable HTTPS by setting AWS_S3_SECURE_URLS to True in your Django settings. This ensures that all generated URLs are HTTPS, protecting the data as it moves between your server and the client.
You might enjoy: Https Drive Google Com Drive Quota
To authenticate with Azure, you can use the DefaultAzureCredential class from the Azure Identity library, which supports multiple authentication methods and determines which method to use at runtime. This approach enables your app to use different authentication methods in different environments without implementing environment-specific code.
Here are some authentication methods provided by Azure Identity:
- Passwordless (Recommended)
- Connection String
Note that using the account access key for authentication is not recommended, as it can expose your access key in an unsecure location.
Check this out: Azure Storage Account Key
Obtain Access Keys
To obtain access keys to your Azure Storage, you'll need to create a storage account and container, which can be done by following the steps mentioned in the Azure documentation.
First, create a storage account and container. You can do this by following the steps mentioned in the Azure documentation.
If you've already created a storage account and container, you can obtain the access keys by using the Azure portal or the Azure CLI.
Discover more: Azure Container Storage
The access keys are used to authenticate requests against the storage account, so it's essential to keep them secure.
Here's a list of the different authentication methods provided by Azure, in order of precedence:
- connection_string or AZURE_CONNECTION_STRING
- (account_key or AZURE_ACCOUNT_KEY) and (account_name or AZURE_ACCOUNT_NAME)
- token_credential or AZURE_TOKEN_CREDENTIAL with account_name or AZURE_ACCOUNT_NAME
- sas_token or AZURE_SAS_TOKEN
Remember, the account access key should be used with caution, as anyone who has it can authorize requests against the storage account.
Securing Your
Always ensure your bucket policies are tight, like Amazon S3, which allows you to define granular permissions that dictate who can access and modify your storage objects.
To prevent direct access to your files, you can deny all actions on all objects within your bucket unless the request comes from your domain.
Enabling encryption for data at rest is crucial, and S3 provides options for server-side encryption with AWS managed keys (SSE-S3), customer-provided keys (SSE-C), or KMS-managed keys (SSE-KMS).
You can set a configuration in your Django settings to use S3's AES-256 encryption for your stored files.
A different take: Google Cloud Storage Bucket
Securing data in transit is also essential, and you can enforce HTTPS by setting the AWS_S3_SECURE_URLS to True in your Django settings, ensuring all generated URLs are HTTPS.
Regularly review your access logs and monitor for any unauthorized access attempts, as S3 provides detailed access logs that can be analyzed to spot suspicious activities.
Here are some best practices for securing your storage backends:
Private vs Public URLs
Private URLs include a SAS token, which allows you to override certain properties stored for the blob by specifying query parameters.
You can override properties like cache-control, content-type, content-encoding, content-language, and content-disposition with private URLs.
These properties can be overridden by specifying query parameters as part of the shared access signature.
Private URLs give you more control over the properties of your blobs, allowing you to customize them as needed.
You can use this feature to ensure that your blobs are served with the correct headers and metadata.
Consider reading: Azure Storage Account Private Endpoint
Best Practices and Monitoring
Scalability and security are crucial when integrating Azure Storage into your Django project, ensuring your application can handle growth smoothly and protect your data from unauthorized access.
Focusing on scalability from the outset is key, as it allows your application to adapt to growth without compromising performance.
To achieve this, consider implementing strategies for scalability, such as optimizing database queries and using load balancing techniques.
Ensuring the health and efficiency of your storage solutions is also vital, which involves optimizing performance, minimizing downtime, and securing your data.
Monitoring your storage backends regularly will help you identify potential issues before they become major problems.
Scalability and Security Best Practices
Scalability and security are crucial for a smooth-running application.
Focusing on scalability and security from the outset is essential when integrating Django Storages into your project. This ensures your application can handle growth smoothly.
Scalability best practices include maintaining and monitoring storage health to optimize performance and minimize downtime. Ensure your storage solutions are efficient and secure.
Suggestion: Azure Blob Storage Security
To maintain and monitor storage health, practical strategies and tools can be employed to keep your storage backends in top shape. This includes optimizing performance, minimizing downtime, and ensuring data remains secure and accessible.
Securing your storage backends is a necessity, especially when using popular choices like Amazon S3. Always ensure bucket policies are tight to prevent unauthorized access and leaks.
Setting a restrictive bucket policy can be as simple as defining granular permissions that dictate who can access and modify your storage objects. This can be done by denying all actions on all objects within your bucket unless the request comes from your domain.
Enabling encryption for data at rest is also crucial. S3 provides options for server-side encryption, such as using AWS managed keys or customer-provided keys. This can be set in your Django settings to use S3's AES-256 encryption for your stored files.
Securing data in transit is equally important by enforcing HTTPS. This can be done by setting the AWS_S3_SECURE_URLS to True in your Django settings, ensuring all generated URLs are HTTPS.
Regularly reviewing access logs and monitoring for unauthorized access attempts is also essential. S3 provides detailed access logs that can be analyzed to spot suspicious activities.
Intriguing read: Amazon Glacier Deep Archive
Backup and Archiving
Backing up your data is crucial, and Django Storages can automate this process by integrating with cloud storage solutions. You can set up periodic tasks to back up database dumps or critical files to a remote storage backend.
Automating backups ensures that your backups are always up to date and secure. This can be achieved using Celery, which allows you to run tasks asynchronously in the background.
By integrating with cloud storage solutions, you can store your backups securely and access them remotely in case of an emergency.
Here's an interesting read: Designing and Implementing Microsoft Azure Networking Solutions Pdf
Sources
- https://vivekyadavofficial.medium.com/upload-file-to-azure-blob-storage-in-django-python-696c995d2a25
- https://coderbook.com/@marcus/how-to-store-django-static-and-media-files-on-s3-in-production/
- https://sqlpad.io/tutorial/django-storages/
- https://django-storages.readthedocs.io/en/latest/backends/azure.html
- https://learn.microsoft.com/en-us/azure/storage/blobs/storage-quickstart-blobs-python
Featured Images: pexels.com