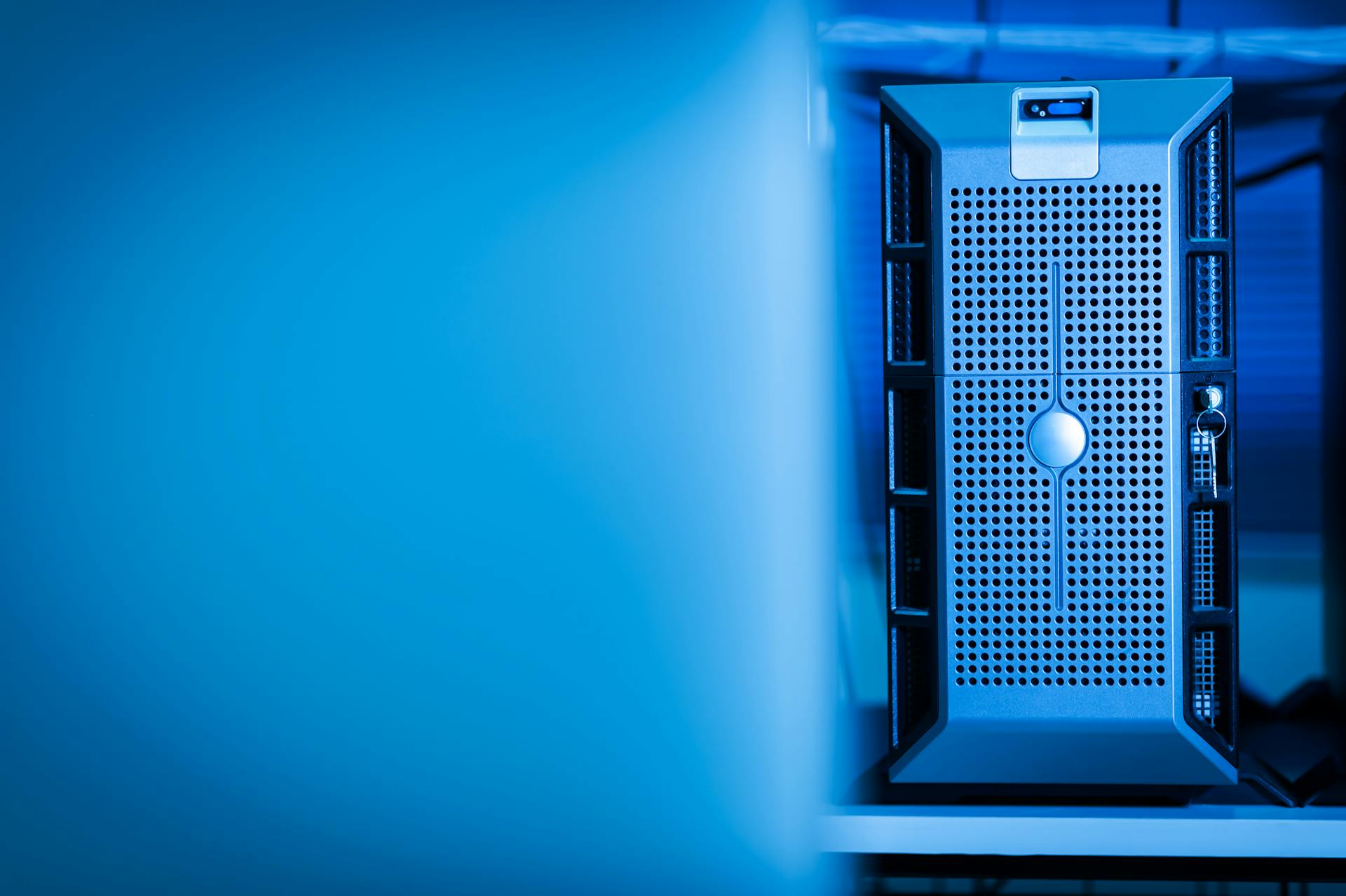
Azure Webjobs Storage and Azure Functions Integration is a powerful combination that can streamline your cloud-based workflow. Azure Functions can be triggered by events in Azure Storage, such as blob uploads or new table rows.
With Azure Functions and Azure Storage integration, you can automate tasks and workflows without needing to write custom code. This integration is seamless and scalable.
Azure Functions can be triggered by Azure Storage events, including blob, queue, and table operations. This allows for real-time processing of data stored in Azure Storage.
Consider reading: Azure Events
Configuring Storage
Configuring storage is a crucial step in setting up your Azure WebJobs storage. You can configure AzureWebJobsStorage to use an identity-based connection without a secret. This is recommended for function apps running in a Consumption plan (Windows only) or an Elastic Premium plan (Windows or Linux).
To use Azure Files, set the connection string for the storage account in the WEBSITE_CONTENTAZUREFILECONNECTIONSTRING setting and the name of the file share in the WEBSITE_CONTENTSHARE setting. This is usually the same account used for AzureWebJobsStorage.
You can also configure AzureWebJobsStorage to use the Azure Storage Emulator or Azure Storage directly. To use the emulator, run the following command: func settings add AzureWebJobsStorage UseDevelopmentStorage=true or add "AzureWebJobsStorage": "UseDevelopmentStorage=true" to the AppSettings.json file.
Suggestion: Azure Blob Storage Add Metadata
Connection Setting
The AzureWebJobsStorage property is used for the Azure Functions runtime's normal operation, including key management, timer trigger management, and Event Hubs checkpoints. It should contain a general-purpose storage account connection string that supports blobs, queues, and tables.
To run the runtime locally, you should set the AzureWebJobsStorage property in your local.settings.json file to "UseDevelopmentStorage=true", which will use your Azure Storage Explorer or Azurite locally.
A storage account connection string must be updated when you regenerate storage keys, and you can read more about storage key management here.
You can also configure AzureWebJobsStorage to use an identity-based connection without a secret, which is a good option for security-conscious developers.
Function apps running in a Consumption plan (Windows only) or an Elastic Premium plan (Windows or Linux) can use Azure Files to store images required to enable dynamic scaling, and you can set the connection string for the storage account in the WEBSITE_CONTENTAZUREFILECONNECTIONSTRING setting and the name of the file share in the WEBSITE_CONTENTSHARE setting.
Broaden your view: Upload File to Azure Blob Storage
Configure Local Environment
To configure your local environment, you need to install a few essential tools. You'll want to install the Azure Storage extension for Visual Studio Code, as well as Azure Storage Explorer, which is supported on macOS, Windows, and Linux-based operating systems.
You'll also need to install .NET Core CLI tools and complete the steps in part 1 of the Visual Studio Code quickstart. This will get you set up with the necessary tools to work with Azure Storage.
Here are the specific steps you need to take:
- Install the Azure Storage extension for Visual Studio Code.
- Install Azure Storage Explorer.
- Install .NET Core CLI tools.
- Complete the steps in part 1 of the Visual Studio Code quickstart.
Once you've completed these steps, you'll be able to add the storage output binding to your project and start working with Azure Storage.
Mount File Shares
Mounting a file share can increase the cold start time of your function app by at least 200-300 ms, or even more if the storage account is in a different region.
You can mount up to five shares to a given function app, but be aware that this can impact performance.
To mount an existing Azure Files share, you'll need to use the `az webapp config storage-account add` command in the Azure CLI.
The command requires you to specify the share name, a custom ID, and the mount path, which must be in the format `/dir-name` and cannot start with `/home`.
Alternatively, you can use Azure PowerShell with the `Set-AzWebApp` cmdlet.
Here's a brief summary of the required parameters:
- Share name: the name of the existing Azure Files share
- Custom ID: a string that uniquely defines the share when mounted to the function app
- Mount path: the path from which the share is accessed in your function app (must be in the format `/dir-name` and cannot start with `/home`)
Once you've created the path, you can access the mounted share using file system APIs in your function code.
Using
Using the Azure Storage Emulator is a great way to test your Azure Functions locally without incurring costs. You can install it by downloading the Azure Storage Emulator from the official Microsoft documentation.
To use the emulator, you'll need to run it on your machine and configure your local environment to use it. This can be done by running the command `func settings add AzureWebJobsStorage UseDevelopmentStorage=true` or by adding `"AzureWebJobsStorage": "UseDevelopmentStorage=true"` to your `AppSettings.json` file.
The Azure Storage Emulator is a convenient way to test your Azure Functions without needing to install the Azure Storage SDK. Once you've configured your environment, you can use the emulator to test your functions.
To stop the Azure Storage Emulator, you can run the command `AzureStorageEmulator.exe stop`.
Table Storage
Table Storage is a scalable and cost-effective solution for storing structured data in Azure WebJobs. It's designed to handle large amounts of data and support high-throughput applications.
Azure Table Storage is a NoSQL key-value store that allows you to store large amounts of structured data in the cloud. You can store up to 100 TB of data in a single table.
The data is stored in a table format, with each row representing a single entity and each column representing a property of that entity. This makes it easy to query and retrieve data from the table.
For more insights, see: What Is the Data Storage in Azure Called
Table Entity
Table Entity is a crucial concept in Table Storage.
Entities bound to tables should inherit from ITableEntity, which includes essential properties like PartitionKey, RowKey, and ETag.
To make development easier, you can create your own base class, like BaseTableEntity, to use instead of the old SDK's TableEntity.
This will help you organize your code and avoid potential issues.
For more insights, see: Azure Storage Tables
Inheriting from ITableEntity is necessary to take advantage of Table Storage's features.
It may have been done previously using a helpful base class, but now it's essential to do it manually.
Using a custom base class like BaseTableEntity can make your code more readable and maintainable.
This approach can be especially helpful when working with complex entities.
Working with Blobs
Blob storage input and output bindings support blob-only accounts. This is a key advantage when working with blobs, especially for scenarios like image processing or sentiment analysis.
If you're looking to process file uploads, Azure Functions is a great choice. It allows you to work seamlessly with blobs and even provides a tutorial to get you started.
You can use Azure Functions to execute your code based on changes to blobs in a storage container. There are several strategies to choose from, each with its own strengths and weaknesses.
Here's a quick rundown of the strategies:
Each strategy has its own trade-offs, so be sure to choose the one that best fits your needs.
Output Bindings
Output Bindings are a powerful feature in Azure WebJobs Storage, allowing you to easily write data to a queue without needing to use the Azure Storage SDK.
To use an output binding, you must have the Storage bindings extension installed, which can be added using the dotnet add package command. The extension package is implemented as an extension bundle, which automatically installs a predefined set of extension packages.
The run method definition should include the name of the binding to access it as an attribute in the function signature. This allows you to use the output binding object to create a queue message, such as writing a string message to the queue using the set method.
Here are the key benefits of using output bindings:
- No need to use the Azure Storage SDK for authentication, getting a queue reference, or writing data.
- The Functions runtime and queue output binding do those tasks for you.
By using output bindings, you can simplify your code and focus on writing the logic of your function, rather than worrying about the underlying storage implementation.
Table Binding Extensions
Table binding extensions can be a bit tricky to navigate, especially since they've recently moved to a new location. The Table storage bindings are now in the Microsoft.Azure.WebJobs.Extensions.Tables NuGet package, not the Microsoft.Azure.WebJobs.Extensions.Storage package.
If you're using both blob storage and table storage bindings, you'll need to reference both packages. This is what I did in my application.
The good news is that Microsoft.Azure.WebJobs.Extensions.Tables is now available as v1.0.0, after a brief period of confusion when it was still in preview and the new Microsoft.Azure.WebJobs.Extensions.Storage package didn't support table binding.
You can learn more about the changes in the official documentation for the table storage binding.
Add Output Binding
Adding an output binding is a crucial step in creating a function that interacts with Azure Storage. This process involves defining a binding in your project's host.json file.
To begin, you need to have the Storage bindings extension installed, which can be done by running the dotnet add package command in your Terminal window. This command adds the Storage extension package to your project.
The run method definition should be updated to include the output binding. This involves adding a parameter to the function definition and using the set method to write a string message to the queue.
You can use the Push-OutputBinding cmdlet to write text to the queue using the output binding. This involves adding code that uses the output binding object on context.extraOutputs to create a queue message.
Here's a step-by-step guide to adding an output binding:
1. Define the output binding in your project's host.json file.
2. Update the run method definition to include the output binding.
3. Add code that uses the output binding object to create a queue message.
4. Use the set method to write a string message to the queue.
By following these steps, you can successfully add an output binding to your function and interact with Azure Storage.
You might enjoy: How to Create Blob Storage in Azure
Frequently Asked Questions
Is AzureWebJobsStorage required?
Yes, AzureWebJobsStorage is required for your function app to operate. It's a crucial setting that enables coordination between multiple instances, making it a must-have for a functioning function app.
What is AzureWebJobsSecretStorageType?
AzureWebJobsSecretStorageType is a setting that determines where your Azure function app stores sensitive keys, such as API keys and connection strings, securely and encrypted. It's a crucial configuration for protecting your app's secrets.
Where is AzureWebJobsStorage?
AzureWebJobsStorage is automatically created in the Function App configuration when created from the Azure Portal. Its value contains the secret connection string of the associated storage account.
Sources
- https://www.koskila.net/how-to-fix-missing-value-for-azurewebjobsstorage-in-local-settings-json-when-youre-debugging-azure-functions-locally/
- https://markheath.net/post/azure-functions-table-storage
- https://learn.microsoft.com/en-us/azure/azure-functions/functions-add-output-binding-storage-queue-vs-code
- https://www.eliostruyf.com/set-up-azure-storage-for-local-develop-of-timer-or-queue-triggered-azure-functions/
- https://learn.microsoft.com/en-us/azure/azure-functions/storage-considerations
Featured Images: pexels.com