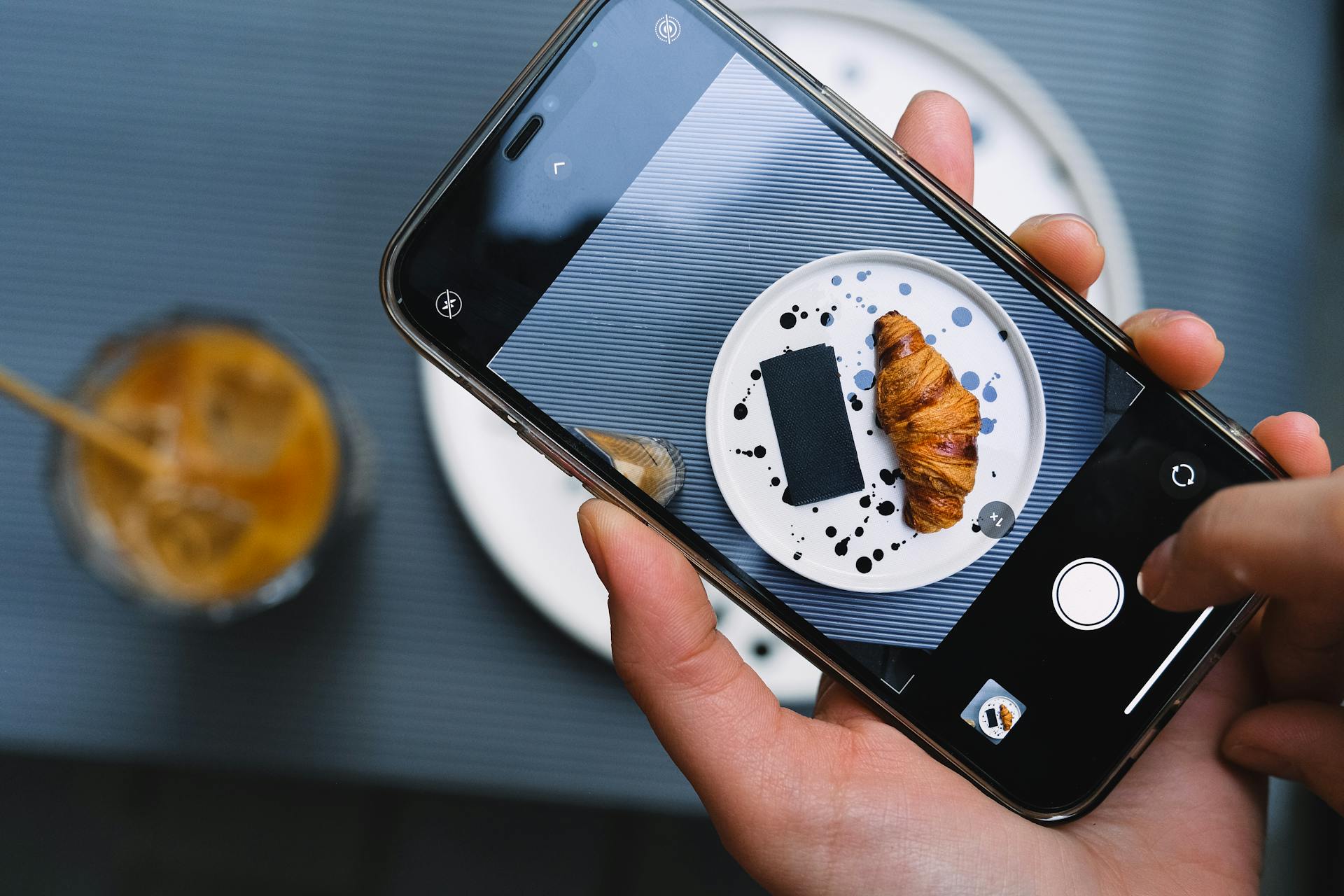
Using Base64-encoded images as a src attribute can have significant security implications. This is because the encoded data is not validated or sanitized, making it vulnerable to attacks.
Base64-encoded images can also impact performance, as they can increase the size of the image file. For example, a 1KB image encoded in Base64 can increase to around 1.37KB, which can slow down page loading times.
However, using Base64-encoded images can also provide some benefits, such as reducing the number of HTTP requests required to load an image. This is because the encoded image data can be embedded directly into the HTML document, eliminating the need for a separate HTTP request.
Intriguing read: Data Text Html Base64
The Solution
To manually convert an image to Base64, use a site like http://base64online.org/encode. Upload your image to the site and copy the text it returns.
Your src attribute must begin with data:image/png;base64,. After that, you need to insert your image text, which can be surrounded on both sides by as much whitespace as you like.
See what others are reading: Data Text Html Charset Utf 8 Base64
Some browsers might fail to display your image if you use an unexpected text encoding. To be safe, always specify your MIME Content-Type and Content-Encoding.
You can also use a Base64 image as a background in CSS. A common error reported by programmers is mistakenly double-encoding their images. If you encounter an error you can’t debug, try to decode your Base64 text to check that you didn’t encode it twice accidentally.
Image as File/Object or Blob
If your image is a File object or Blob, you can use the FileReader API to convert it to a Base64 string. This API is also used when converting an image tag to a Base64 string.
The FileReader API is a powerful tool for working with files and Blobs in JavaScript. It allows you to read the contents of a file or Blob and convert it to a string, which can then be used as a Base64 string.
For another approach, see: The Html File
To use the FileReader API, you'll need to create a new FileReader object and call its readAsDataURL() method, passing in the File object or Blob as an argument. This will return a promise that resolves to a data URL containing the Base64-encoded image data.
Here's a brief example of how this might work:
- Create a new FileReader object: `const reader = new FileReader();`
- Call the readAsDataURL() method, passing in the File object or Blob: `reader.readAsDataURL(file);`
- Wait for the promise to resolve and retrieve the data URL: `reader.onload = () => { const dataURL = reader.result; }`
By using the FileReader API, you can easily convert your image to a Base64 string and use it in your HTML or CSS.
Enhanced Security
Base64 encoding is a simple yet effective way to add an extra layer of protection to your digital assets. By obscuring the binary data, it adds a layer of complexity that can deter casual tampering or unauthorized access.
This extra layer of security is particularly useful for images, as it makes it more difficult for others to directly access the file.
When to Use Images
You need to use images when you have no access to JavaScript. This is because JavaScript is often used to load images, and without it, images won't load properly.
For another approach, see: Change Src Img Jquery
Creating an offline, standalone, single-file HTML report requires images to be embedded directly into the HTML file, using Base64 encoding. This ensures the images load correctly even without an internet connection.
If you're sending HTML emails and the email software blocks external image links, using Base64 images is a good solution to ensure your email is displayed as intended.
See what others are reading: Css in Html File
Output Formats
Base64-encoded images can be formatted in various ways.
You can check examples to see how the result will look in each available format.
Some common output formats include GIF, which can be used for a one-pixel red dot image.
If you're unsure about the output format you need, it's a good idea to check the examples.
Base64-encoded images can be formatted in GIF, which can display a one-pixel red dot.
You can implement additional output formats if you know of any that are missing.
The author of the article would love to hear about any important output formats you think are missing.
Frequently Asked Questions
Can img src be Base64?
Yes, the src attribute of an tag can contain a Base64 encoded image, which is a Data URL composed of two Base64 encoded parts separated by a comma. This allows images to be embedded directly in HTML code.
How to display Base64 in IMG?
To display a Base64 image, use the HTML image tag () and set the "src" attribute to the data URL in the format "data:image/[format]; base64," where [format] is the image file type (e.g. PNG, JPEG, GIF). Simply embed the data URL into your HTML to display the image.
Featured Images: pexels.com