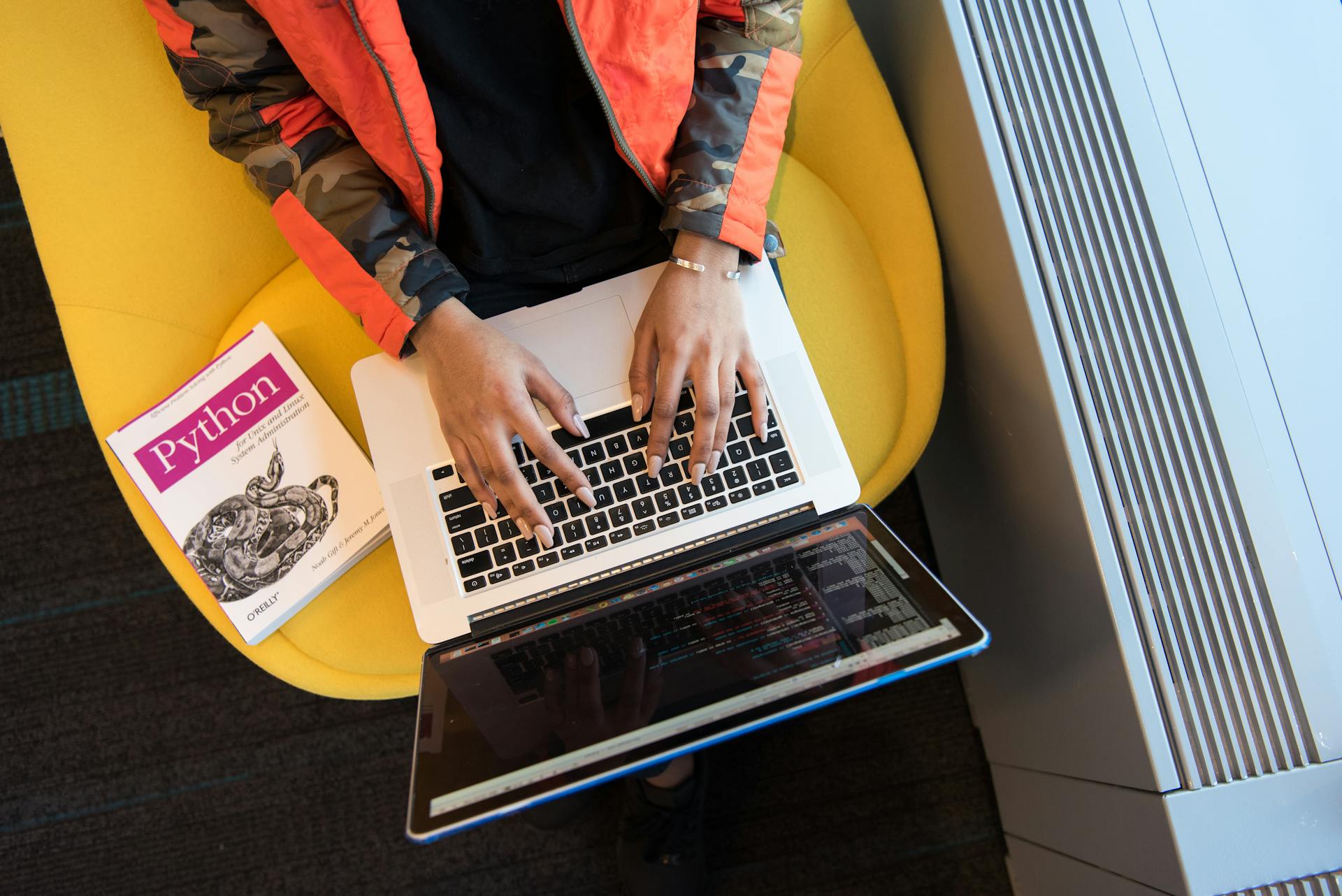
Building a web scraper in Python is a great way to extract data from websites, and it's easier than you think.
First, you'll need to choose a library to use for scraping, and one popular option is Scrapy, which is a powerful and flexible tool that can handle complex tasks.
The first step in building a web scraper is to identify the website you want to scrape and understand its structure. You can use the Inspect Element feature in your browser to see the HTML code behind the website, which will help you find the data you're looking for.
To start scraping, you'll need to install the necessary libraries, including Scrapy, which you can do with pip, the Python package manager.
Explore further: Search Engine Scraping
Setting Up Environment
To set up your environment for building a web scraper in Python, you'll need to choose a coding environment. A good coding environment can make a huge difference in your productivity and overall experience.
There are many options to choose from, including a simple text editor or a fully-featured IDE like PyCharm. If you already have Visual Studio Code installed, you can use that, but PyCharm is highly recommended for newcomers due to its intuitive UI and low entry barrier.
To use PyCharm, right-click on the project area and select New > Python File. Give your file a nice name and you're ready to go!
If this caught your attention, see: How to Get Html File from Website
Libraries
There are many useful libraries available for web scraping in Python, making it easy to get started. You can choose from various types of libraries, including Requests, Beautiful Soup, lxml, Selenium, and Scrapy.
The Requests library simplifies the process of making HTTP requests by reducing the lines of code, making it easier to understand and debug. However, it doesn't parse the extracted HTML data, so you'll need to use another library for that.
Here's a comparison of the popular Python web scraping libraries:
Libraries Compared
Let's take a look at the popular web scraping libraries available for Python. You've got a few options to choose from, including Requests, Beautiful Soup, lxml, Selenium, and Scrapy.
Requests is a high-level library that simplifies making HTTP requests, making it a great option for beginners. It's also very fast and has excellent technical documentation.
Beautiful Soup is another popular choice, known for its ease of use and parsing capabilities. It's also very fast and has excellent technical documentation, making it a great option for beginners.
lxml is a parsing library that's particularly well-suited for large and small projects alike. It's also very fast, but has a slightly steeper learning curve compared to some of the other options.
Selenium is a complete scraping framework that's particularly well-suited for websites that use JavaScript to load content. However, it's also the slowest option on this list and has a more difficult learning curve.
Here's a comparison of these libraries in a table:
Ultimately, the choice of library will depend on your specific needs and the type of project you're working on.
Lxml
Lxml is a fast and powerful parsing library that works with both HTML and XML files. It's ideal for extracting data from large datasets, but can be impacted by poorly designed HTML.
The library can be installed from the terminal using the pip command. This allows you to work with HTML using its html module.
The lxml library needs an HTML string first, which can be retrieved using the requests library. Once the HTML is available, you can build a tree using the fromstring method.
You can query the tree using XPath. For example, if you're looking for the titles of blogs, you can use an XPath expression to get them. The contains() function selects elements with a specific class value, and you can give this XPath to the tree.xpath() function to return all matching elements.
The newest iteration of your code should look something like this, allowing you to integrate lxml into your web scraping efforts.
For another approach, see: Python save Html to File
Choosing a Coding Environment
Before we dive into the programming part of our web scraping tutorial, we need to choose a coding environment. There are many options to consider.
A simple text editor is a viable option, but if you're using a programming language like Python, you'll want something more advanced. PyCharm is highly recommended for newcomers due to its intuitive UI and low entry barrier.
If you already have Visual Studio Code installed, you can use that as well. Otherwise, PyCharm is a great choice. In PyCharm, you can create a new Python file by right-clicking on the project area and selecting New > Python File.
Picking a URL
Picking a URL is the first step in building a web scraper in Python. This is where you choose the website you want to scrape, and it's crucial to pick a simple target URL.
Avoid data hidden in Javascript elements, as these sometimes need to be triggered by performing specific actions to display the required data. This requires more sophisticated use of Python and its logic.
Here's an interesting read: How to Build Data Lake
Avoid image scraping, as images can be downloaded directly with Selenium. This is a great way to save time and effort.
Always ensure that you're scraping public data and are in no way breaching third-party rights. It's also a good idea to check the robots.txt file for guidance.
Here are some tips to keep in mind when selecting a URL:
- Choose a website with a simple structure.
- Make sure the website is not too dynamic, as this can make scraping more difficult.
- Consider using a website that provides a clear and easy-to-parse HTML structure.
Remember to attach "http://" or "https://" to the URL, as Selenium requires the connection protocol to be provided.
Exporting
Exporting is a crucial step in building a web scraper in Python, and it's surprisingly easy to get wrong.
Constant double-checking of the code is necessary when exporting data to ensure you're getting the data assigned to the right object.
Even if your code runs without errors, there might be semantic errors that need to be caught.
Use print statements to check if the data you acquired is being collected correctly, especially when dealing with arrays.
A simple loop can be used to print each entry in the array on a separate line.
Remove print loops when moving data to a CSV file, as you'll be doing something similar.
The pandas library is useful for creating a two-dimensional data table from your data.
You can create multiple columns with pandas, but you'll need more lists to utilize them.
The to_csv() function can be used to move your data to a CSV file, specifying a name, extension, and encoding.
UTF-8 encoding is a good choice for most cases, as it's widely supported.
No imports should be grayed out after using the pandas library.
Running your application should output the name of the CSV file in your project directory.
The saved CSV file will look like a table when opened in a program that supports CSV files.
Full Code Example
Let's dive into the full code example for building a web scraper in Python. You'll need to create a new .py file in your project directory and paste the following code, which demonstrates a simple two-step scraper.
The code uses the requests library and BeautifulSoup for basic crawling and extraction, handling the first step of gathering product URLs from 5 search result pages.
Here's a breakdown of the two steps involved:
- Crawl through 5 search result pages to gather product URLs.
- Visit each product URL, scrape and parse the relevant data, and save it to a CSV file.
This code leverages Selenium to load each product page fully, ensuring you capture all dynamic content.
After running this code, your console should print the scraper progress information, which includes a progress tracker to help monitor the scraper's status.
Best Practices
As you build your web scraper in Python, it's essential to follow some best practices to make your tool efficient and effective.
Experiment with different features to upgrade your scraper, like creating matched data extraction by creating a loop that makes lists of even length.
A basic scraper is a good starting point, but it's not enough for serious data acquisition. To take it to the next level, try scraping several URLs in one go by building a loop and an array of URLs to visit.
You can also create several arrays to store different sets of data and output it into one file with different rows. This is especially useful for e-commerce data acquisition.
Running a headless version of a browser like Chrome or Firefox can reduce load times and make your scraper more efficient.
To create a realistic scraping pattern, think about how a regular user would browse the internet and try to automate their actions. This might involve using libraries like import time and from random import randint to create wait times between web pages.
Here are some key features to consider when building a scraping pattern:
Data on certain websites might be time-sensitive, so consider creating a monitoring process that rechecks certain URLs and scrapes data at set intervals.
To optimize your HTTP methods, make use of the Python requests library, which allows you to optimize HTTP methods sent to servers.
Common Errors
Building a web scraper in Python can be a fun and rewarding project, but it's not without its pitfalls. Not handling HTTP exceptions and errors properly can cause your scraper to crash or miss critical data.
You should implement error handling using try and except blocks to ensure your scraper can manage unexpected responses. This will save you a lot of frustration and debugging time down the line.
Ignoring rate limiting and making too many requests in a short time frame can lead to your IP address being blocked. Websites often have mechanisms to detect and prevent scraping activities that burden their servers.
Implement delays between requests and respect the website's robots.txt file to avoid being flagged as malicious traffic. This is a simple yet crucial step in building a reliable web scraper.
Not setting an appropriate User-Agent header can also get your scraper blocked. Many websites block HTTP requests that come with the default Python requests User-Agent.
Here are some common errors to watch out for:
- Not handling HTTP exceptions and errors properly
- Not accounting for dynamic content loaded via JavaScript
- Ignoring rate limiting and making too many requests
- Not setting an appropriate User-Agent header
- Mishandling data extraction due to incorrect HTML parsing
- Overlooking legal and ethical considerations
Introduction to
Building a web scraper in Python is an exciting project that requires a solid foundation in the language. Python is the preferred programming language for developing custom web scraping solutions due to its robust features and supportive ecosystem.
One of the main reasons Python stands out is its ease of use and readability. Python's straightforward syntax ensures that scripts are easy to write and maintain, which is vital for businesses looking to adapt quickly to market changes.
Python offers specialized libraries such as BeautifulSoup, Scrapy, and Selenium that simplify tasks related to data retrieval, HTML parsing, and browser automation. These tools significantly reduce development time and make web scraping more efficient.
Python's flexibility supports the development of both simple scripts for small-scale data needs and complex systems for large-scale corporate data processing. This scalability is a major advantage when building a web scraper.
A strong community support is also a significant benefit of using Python. The extensive Python developer community is an invaluable resource for troubleshooting, updates, and continuous learning, ensuring that business solutions stay current and effective.
For another approach, see: Outline of Web Design and Web Development
Tools and Libraries
Building a web scraper in Python requires the right tools and libraries. You can choose from a large selection of libraries, with over 500,000 projects on PyPI alone.
One of the most popular libraries is Requests, which simplifies making HTTP requests and reduces the lines of code needed. It's easy to install using pip and provides methods for sending HTTP GET and POST requests.
For parsing HTML data, you can use BeautifulSoup, which is part of the Python web scraping libraries. It's easy to use and can be installed using pip.
Other popular libraries include lxml, Selenium, and Scrapy. Scrapy is a complete scraping framework that takes a lot of the work out of building and configuring spiders.
Here's a comparison of some of the popular libraries:
Remember to install the required libraries before starting your project. You'll need to install Requests and BeautifulSoup using pip.
Creating a Script
Creating a script is a crucial step in building a web scraper in Python. You can start scraping data in no time by breaking down the process into manageable steps.
To write a Python script, you'll need to use libraries such as requests and BeautifulSoup, just like in the example of creating your first Python scraping script. These libraries will help you send HTTP requests and parse the HTML content of a webpage.
By following a simple guide, you can create your first Python scraping script and be on your way to scraping data from webpages.
Explore further: Free Website Scraping
Create a Project
Creating a project is the first step to scraping a website. To do this, open your command prompt and navigate to the directory where you want to create your project.
Type `scrapy startproject scrapytutorial` and press enter. This will set up all the project files within a new directory automatically.
The new directory will contain two files: `scrapytutorial` and `Scrapy.cfg`.
Expand your knowledge: Link Building Directories
Create a Custom
Creating a custom script can seem like a daunting task, but it's actually quite straightforward. To create a custom spider with Scrapy, you'll need to open the project folder on VScode or your preferred code editor and create a new file within the spider folder called winespider.py.
The first step is to import Scrapy to your project at the top of the file. This is a crucial step that sets the foundation for your spider. You'll also need to define a new class (spider) and add the subclass Spider.
Take a look at this: Spider Web Team Building Activity
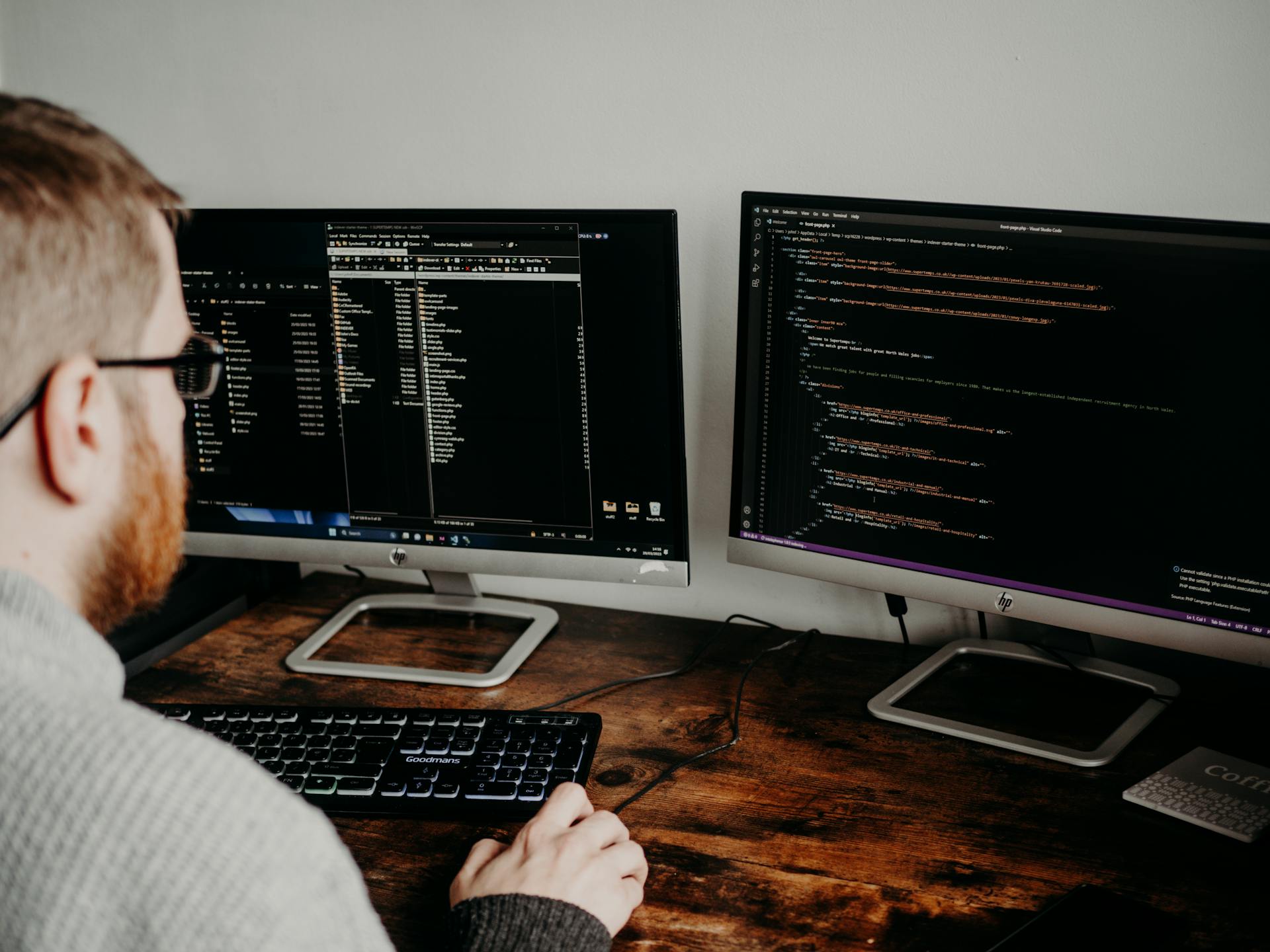
To give your spider a unique name, choose a name that cannot be the same as any other spider within the project. For example, you could name it "winy" as shown in the code snippet. This unique name will help you identify your spider later on.
To give your spider a target page, use the start_urls variable to specify the URL you want to scrape. In this case, you could use the URL 'https://www.wine-selection.com/shop'. You can also add a list of URLs separated by commas, but for now, let's just provide the first page.
Finally, you'll need to tell Scrapy what information you want it to find within the HTML. You can use the parse() function to handle the download page, just like we did in Scrapy Shell. This will allow you to extract the data you need from the webpage.
Here's a summary of the steps to create a custom spider:
- Import Scrapy to your project
- Define a new class (spider) and add the subclass Spider
- Give your spider a unique name
- Specify the target page using start_urls
- Tell Scrapy what information to find within the HTML using the parse() function
By following these steps, you'll be able to create your own custom spider with Scrapy.
Run and Save
You're almost ready to see your web scraper in action! To run your scraper, exit Scrapy Shell and move to the project folder on your command prompt.
Type scrapy crawl and your spider's name to initiate the scraping process. If everything is working, the data scraped will be logged into your command line.
Now that you know it's working, you can run it again with a specific option to store the scraped data. Use the -o option followed by the filename you want to save the data to, like winy.json.
If you want to add more information to an existing file, use a lower-case "o" instead, like -o winy.csv. This will append new data to the existing file.
Readers also liked: How to Run Nextjs to Build
Automating Updates
Automating updates is a crucial step in building a reliable web scraper. Use cron jobs on Linux or Task Scheduler on Windows to run your scraping scripts periodically.
You can set up these automated scripts to update your data at regular intervals, ensuring your web scraper stays up-to-date with the latest information.
To catch and resolve errors promptly, monitor the health and performance of your scraping operations. This will help you identify and fix issues before they cause problems.
Here are some ways to automate your web scraper updates:
- Use cron jobs (on Linux) or Task Scheduler (on Windows) to run your scraping scripts periodically.
- Monitor the health and performance of your scraping operations to catch and resolve errors promptly.
By automating updates, you can save time and effort, and ensure your web scraper continues to collect accurate and relevant data.
Request Handling
Request handling is a crucial part of building a web scraper in Python, and it's essential to get it right to avoid frustrating errors.
The requests library is a great tool for simplifying the process of making HTTP requests, reducing the lines of code and making it easier to understand and debug.
To handle retries, you can update the send_request method of your ScrapingClient to convert the requested URL into a API call to the ScrapeOps Proxy API Aggregator endpoint if the ScrapeOps proxy has been enabled.
You can also use the ThreadPoolExecutor found in the concurrent package to scrape numerous pages concurrently, controlling how many requests can be made concurrently using the max_workers attribute.
Python comes with two built-in modules, urllib and urllib2, designed to handle the HTTP requests, but most developers prefer to use the Requests library because it's easier to use and requires less code.
To send a request through ScraperAPI servers, you'll need to define a method to use the previous method and add the additional strings to your URL when sending the request.
The requests library doesn't parse the extracted HTML data, so you'll need to use other libraries like BeautifulSoup to accomplish the other aspects of the web scraping process.
Make sure you have Python installed on your system and install the requests library using pip before you start building your web scraper.
The requests library can be used to send HTTP GET and POST requests, and it provides easy methods for sending HTTP requests, making it a great tool for web scraping.
Broaden your view: R Programming Web Scraping
Sources
- https://oxylabs.io/blog/python-web-scraping
- https://www.promptcloud.com/blog/web-scraping-with-python/
- https://scrapeops.io/python-web-scraping-playbook/build-python-web-scraping-framework/
- https://www.zyte.com/learn/what-python-web-scraping-tools-are-available/
- https://www.scraperapi.com/blog/scrapy-web-scraping/
Featured Images: pexels.com