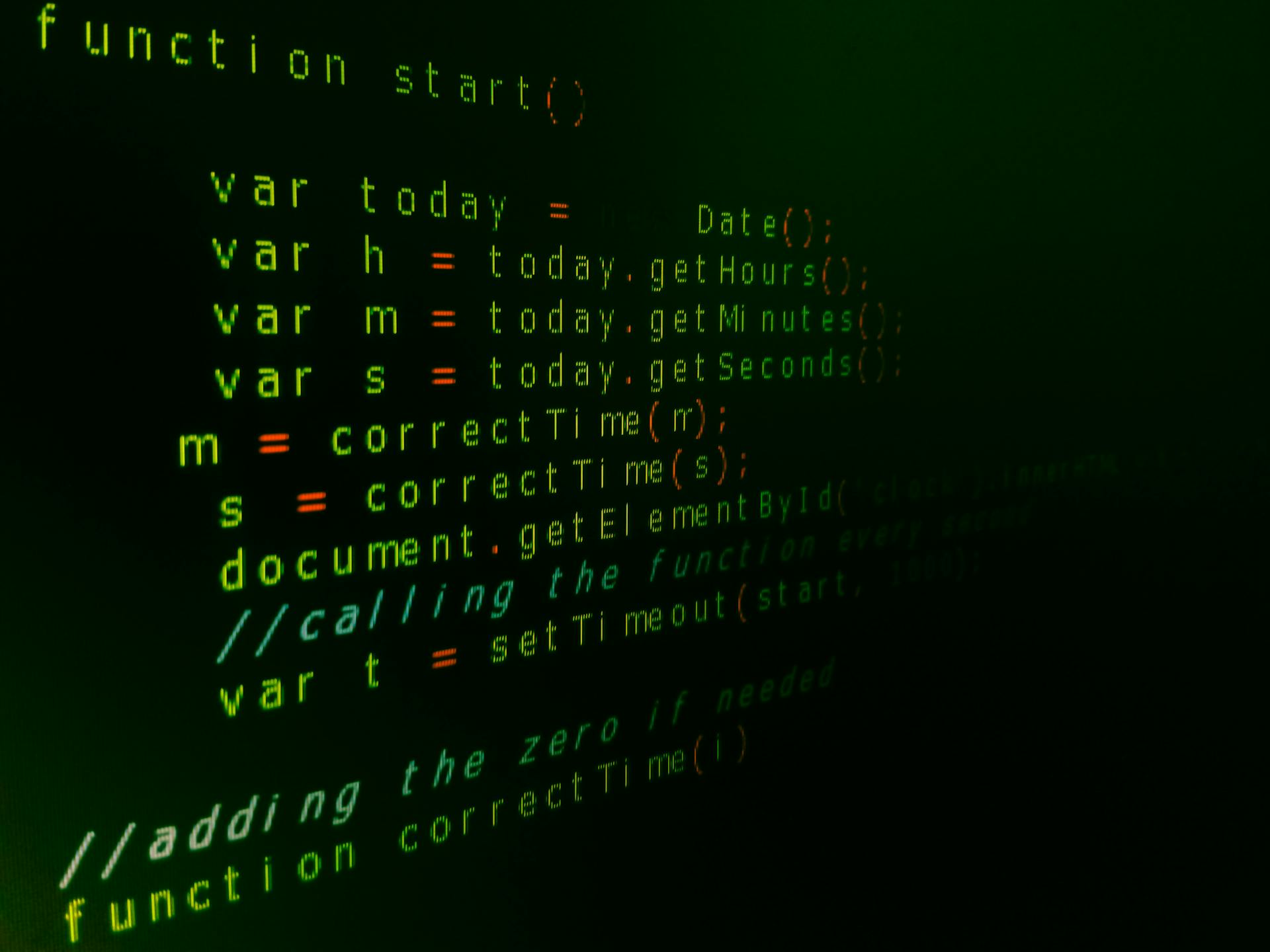
Learning R programming web scraping from scratch can be a bit overwhelming, but don't worry, we'll break it down into manageable chunks.
R is an excellent language for web scraping, and it's free and open-source, making it a great choice for beginners.
You'll need to install the necessary packages, such as rvest and RCurl, to get started with web scraping in R.
These packages will provide you with the tools you need to extract data from websites.
Additional reading: Search Engine Scraping
Setting Up the Environment
To get started with r programming web scraping, you'll need to set up your development environment.
First, install R within RStudio by following the instructions provided.
Next, open the console and install rvest, which is part of the tidyverse collection.
You can also install the tidyverse collection directly to extend the built-in functionalities of rvest with other packages like magrittr for code readability and xml2 for working with HTML and XML.
Curious to learn more? Check out: R Install Devtools
HTML Basics
Ever since Tim Berners-Lee proposed the idea of a platform of documents, HTML has been the foundation of the web and every website you are using.
HTML is the technical representation of a webpage and tells your browser which elements to display and how to display them.
HTML code is made up of tags, which are special markers that serve a special purpose and are interpreted differently by your browser.
Tags can be a pair of opening and closing markers, or self-closing tags on their own, and can have attributes that provide additional data and information.
An HTML document is a structured document with a tag hierarchy, which your crawler will use to extract the desired information.
The HTML format is designed to be machine parsable, making it easy to scrape and analyze.
Understanding the main concepts of HTML, its document tree, and tags will help you identify the parts of an HTML page that you are interested in.
You might enjoy: How to Get Html File from Website
Parsing HTML Content
Parsing HTML content is a crucial step in web scraping with R. HTML stands for HyperText Markup Language and is the technical representation of a webpage, telling your browser which elements to display and how to display them.
Additional reading: Python save Html to File
The HTML format is designed to be machine parsable, making it easy to extract the data we need. R's rvest package supports both CSS and XPATH selectors, allowing us to find exact parts of the page to extract.
HTML documents have a structured document tree, with tags serving a special purpose and being interpreted differently by your browser. Tags can be either a pair of an opening and a closing marker or self-closing tags on their own, with attributes providing additional data and information.
R's rvest package allows us to convert HTML tables to R's data frames, using the html_table() pipe function to automatically extract the whole table from a given selector. This function also picks up table headers from nodes and converts values to appropriate types.
Understanding HTML basics is essential for web scraping with R, as it allows us to identify the parts of the page we are interested in and extract the data we need. With rvest, we can parse HTML documents and extract the data we want, making web scraping a powerful tool for data analysis.
For another approach, see: Web Programming Html
Data Collection
R is a fantastic language for web scraping, and there are several packages available to help you get started. Rcrawler is one of them, designed for network graph related scraping tasks.
To begin, you'll need to store the information you want to scrape in a list, such as a list of scientists' names. This is what we did in the example, where we stored people's names in a list called list_of_scientists.
You can also use Rvest, a package that simplifies web scraping processes in R. With Rvest, you can scrape IMDb to extract titles, ratings, links, and cast members, and add them into a data frame for easy access.
Rcrawler's ContentScraper() function is a powerful tool for crawling pages and extracting data. To use it, you'll need to pass the URLs, XPath expressions, and pattern names for the data items you're interested in.
Here are the main steps to follow when using Rcrawler:
- Store the information you want to scrape in a list
- Create a list of URLs based on your data
- Use Rcrawler's ContentScraper() function to crawl the pages and extract the data
By following these steps, you'll be well on your way to collecting the data you need for your project. Just remember to plan your crawler strategy to avoid being rate limited by the site!
APIs and Integration
APIs are a set of rules and protocols that allow different software systems to communicate with each other, making it possible for developers to access website data in a structured and controlled way.
Using APIs is generally considered more ethical than web scraping, as it's done with the explicit permission of the website or service. However, APIs often have rate limits, which restrict the number of requests you can make within a certain time period.
Some websites don't provide APIs, leaving web scraping as the only option. To overcome these limitations, you can use services like ScraperAPI, which automatically handles roadblocks like IP bans, JavaScript execution, and CAPTCHAs.
Here are some common issues you might face when web scraping at scale:
- IP bans: The website owner may block your IP address, preventing you from accessing the site.
- JavaScript execution: Modern sites often rely on JavaScript to add content to the page, which can be challenging for Rvest to handle.
- Geolocation: Some websites present different data depending on your location, requiring you to change your geolocation or use a service like ScraperAPI.
- CAPTCHAs: Many websites use CAPTCHAs to prevent scraping, which can be difficult to bypass.
APIs
APIs are typically provided by the website or service to allow access to their data, and using them is generally considered more ethical than web scraping. This is because APIs are done with the explicit permission of the website or service.
A unique perspective: Hacking Apis Breaking Web Application Programming Interfaces
Many APIs have rate limits, which means they'll only allow a certain number of requests to be made within a certain time period. This can limit how much data you can access.
Not all websites or online services provide APIs, which means the only way to access their data is via web scraping. This can be a problem if you need to access a lot of data.
Here are some common limitations of APIs:
- Rate limits
- Not all websites or online services provide APIs
APIs can be a powerful tool for accessing data, but they're not without their challenges.
File Download
FTP is still a fast way to exchange files, especially when working with directories like the CRAN FTP server.
The CRAN FTP server URL is ftp://cran.r-project.org/pub/R/web/packages/BayesMixSurv/.
To create a function for downloading files, we can use getURL() to download the file and save it to a local folder.
We can use the plyr package to download multiple files at once, passing in a list of files, a download function, and a local directory.
A cURL handle is required for network communication, which we can obtain for the actual download process.
By using functions like FTPDownloader, we can simplify the process of downloading files from FTP servers.
A fresh viewpoint: C Programming Web Server
Signup for a Fee Account
Signing up for a free account is a straightforward process. You can create a ScraperAPI account using your Gmail or GitHub, or just by creating an account from scratch.
You'll have access to all of ScraperAPI's functionalities for a month, and 1000 free API credits every month. This will be more than enough to test every feature that their API offers.
Inside your dashboard, you'll find your API key, which is essential for getting started with their API.
If this caught your attention, see: Free Website Scraping
Web Scraping Techniques
You can programmatically extract information from a web page by creating a script and running it via the Console using the source() command. This approach allows for traceability and reproducibility.
To load the necessary libraries, you'll need to use the installed libraries, such as rvest. You can then create a variable to store the URL to search, which will be used to download the HTML content of the web page.
Using RegEx to clean the text extracted via web scraping is common and recommended to ensure data quality. You can use the html_nodes() and html_text() functionalities of rvest to save the HTML content in separate objects, making it easier to extract specific information.
To extract the review titles, you can use the CSS Descriptors provided by Chrome's DevTools, modified to remove the specific customer review identifier. This will give you the title of each product review, such as "Very good controller if a little overpriced.".
Extracting the review body and rating can be done using similar methods, with the review body beginning with "In all honesty, I'm not sure why the price..." and the review rating being "4.0 out of 5 stars".
Web Scraping Techniques
You can scrape multiple pages in R by tricking the URL into showing you the results you're looking for by setting start=1. This allows you to write a for loop that increases the number by 50 and accesses all the pages you want to scrape.
Scraping multiple pages requires creating a variable called page_result and adding a sequence to it, so it increases from 1 to 101 by 50 every time. For this exercise, you can just scrape the first three pages.
To avoid resetting your data frame, you can create an empty data frame outside your loop and change your current movies = data.frame() into a rbind() function. This will use your new data frame as its first argument and add new rows to it on every run.
With this technique, you can extract data from multiple pages and accumulate it in your data frame. This is especially useful when scraping large datasets.
Ensuing
To avoid being blocked by web servers, it's essential to replicate the behavior of modern web browsers.
You can do this by setting specific metadata details, such as User-Agent and Accept headers, to mimic a common web browser like Chrome on a Windows platform.
Setting these headers can prevent a lot of web scraping blocking and is recommended for every web scraper.
Project Setup and Execution
To set up a web scraper in R, start by defining your constants, such as the HEADERS constant, which mimics the headers a Chrome browser would use on a Windows computer.
This ensures your scraper won't get blocked by the website.
The company parse function is where the magic happens, using CSS selectors to extract job details from the HTML page. You can use Chrome developer tools to help you find the right selectors.
To test your parser, explicitly scrape one company using the function, which will return the results of the first query page and the count of total listings.
Here's a breakdown of the steps to execute your web scraper:
- Scrape the first page of job listings using a library like rvest.
- Parse the results using your company parse function.
- Scrape the remaining pages in parallel using the parallel connection feature of crul.
- Loop through the pages to scrape all the job listings.
By following these steps, you can create a fast and easy-to-understand scraper that separates logic into single-purpose functions.
Here's a simple example of a scraping loop that utilizes the parallel connection feature of crul:
```r
# Scraping loop
pages <- 1:10
results <- lapply(pages, function(page) {
url <- paste0("https://uk.indeed.com/jobs?q=r&l=Scotland&page=", page)
response <- crul::http_get(url, headers = HEADERS)
parse_results(response)
})
```
For more insights, see: Web Scraping Service Provider
HTTP and Asynchronous Requests
HTTP involves a lot of waiting, with clients waiting for servers to respond, blocking code in the meantime.
This waiting can be avoided by making multiple concurrent connections, which can significantly speed up the scraping process.
For example, 1 synchronous request would take .1 second of actual processing and 2 seconds of waiting, compared to 10 asynchronous requests which will take 10x.1 second of processing and 1x2 seconds of waiting.
We can use R's crul package to make asynchronous requests, which makes them very accessible.
The crul package was chosen over httr for this feature because it makes asynchronous requests very accessible.
We can batch multiple URLs to execute them together, or mix varying type and parameter requests.
This approach allows us to make multiple requests at the same time, which can greatly speed up the scraping process.
The crul package offers vital optional functionality for web scraping, including asynchronous requests.
This is particularly useful for fast scraping, as it allows us to make multiple requests without waiting for each one to complete.
By using asynchronous requests, we can skip the blocked waiting and make our scraping process much faster.
Testing and Debugging
Testing and debugging is a crucial step in web scraping with R. It's essential to verify that your scraper is extracting the correct data.
Use the `browser()` function to inspect the data as it's being scraped. This can help identify any issues with your code.
A good debugging strategy is to test your scraper incrementally, starting with a small subset of the data and gradually increasing the scope. This can help isolate any problems that arise.
Consider reading: Building a Web Scraper in Python
Test Your Sct
Testing your R script is crucial to ensure it's working as expected. Make sure to run the entire script, starting with the library(rvest), to avoid getting an error message.
The library(rvest) is a powerful tool for web scraping, and it's perfect for initial exploration of data. To check if it's working correctly, type 'titles' in the terminal.
If your script is working correctly, it will return the titles of the movies like in the example, from 1 to 50. This is a great way to verify that your script is extracting the data correctly.
The > operator is used to pass the result of the previous operation as the first argument. However, by adding a comma and a dot after the string, you're telling the operator to pass the value as the second argument.
To test your R script, you need to run everything, including the library(rvest) and the code that extracts the data. This will help you identify any issues and make necessary adjustments.
Understanding and Sponses
Having a general overview of HTTP requests and responses is essential for web-scraping.
You don't need to know every detail about HTTP protocol, but understanding its basics is helpful.
A basic GET request is a great place to start.
To make sense of the HTML data retrieved, you can use HTML parsing with CSS and XPATH selectors.
The best way to explore web-scraping is with an example project, so let's do just that.
In web-scraping, knowing which parts of the HTTP protocol are useful is crucial.
For instance, understanding how to make sense of the HTML data is key.
You can use CSS and XPATH selectors to parse the HTML data in R's crul.
Broaden your view: Convert Xpath to Css Selector
Frequently Asked Questions
Is R good for web scraping?
Yes, R is a popular choice for web scraping due to its open-source nature and powerful libraries. It's also relatively easy to use, making it a great option for those new to data extraction.
What is the best R package for web scraping?
For web scraping, consider using rvest, a popular and efficient R package that simplifies the process of extracting data from websites. Alternatively, RSelenium and RCrawler are also viable options, offering more advanced features for complex web scraping tasks.
Featured Images: pexels.com