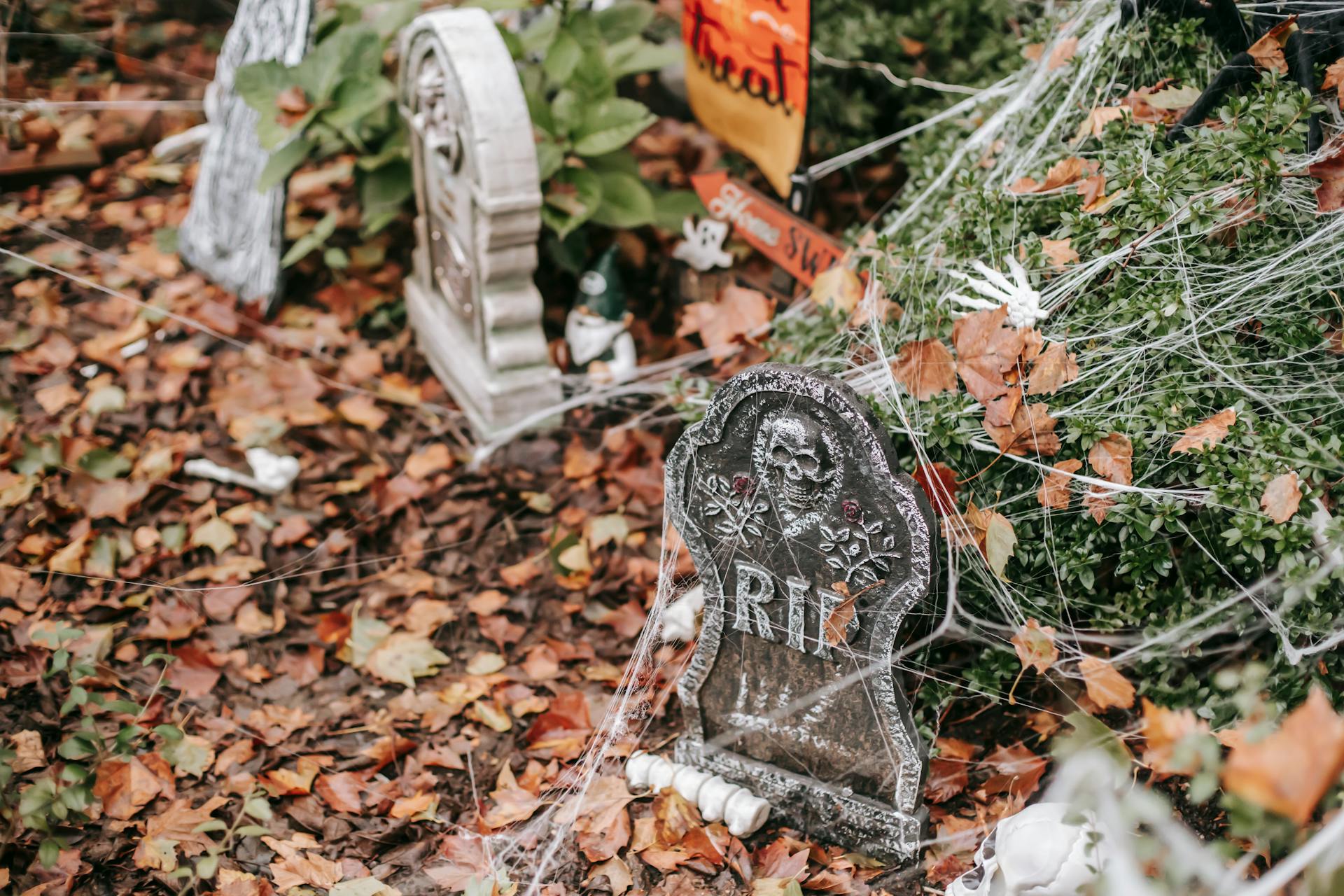
A web server is a crucial part of the internet, handling requests and serving up web pages to users. It's typically implemented using a programming language like C.
To get started with building a C programming web server, you'll need to understand the basics of socket programming. Sockets are endpoints for communication between two devices in a network.
A socket is created using the socket() function, which returns a file descriptor that represents the socket. This file descriptor is used to send and receive data over the network.
Building a web server from scratch requires a solid understanding of networking concepts, including TCP/IP and HTTP.
Building a Web Server
Building a web server in C is a great way to solidify your understanding of HTTP and networking concepts.
C is a powerful and efficient language that allows you to get close to the machine level of operation, providing a deep understanding of how things work.
Building a web server in C can be a hands-on way to learn about multi-threading and socket programming.
Our server will be able to handle HTTP GET requests and send back the requested file if it exists.
If the file doesn't exist, the server will send back a 404 Not Found error.
The server enters an infinite loop where it accepts client connections, reads data from the clients, parses the HTTP requests, sends back the requested files or 404 errors, and closes the client connections.
Inside handle_client(), a function called build_http_response() constructs an HTTP response with a header and the actual file.
The function builds an HTTP header with the appropriate MIME type based on the file extension, such as image/jpeg for a .jpg file.
If the file doesn't exist, the function creates a 404 Not Found response.
The function retrieves the file's size and appends the 200 OK header to the response buffer.
The response buffer is then sent back to the client.
The server can handle any HTTP response with a size up to 1MB, as the BUFFER_SIZE is set to 1MB.
Networking Concepts
To build a C programming web server, you need a solid grasp of networking concepts. A basic understanding of IP addresses, ports, TCP/IP, and HTTP is essential.
IP addresses are a crucial part of networking, and they're used to identify devices on a network. They're usually written in a dotted decimal notation, like 192.0.2.1.
TCP/IP is a fundamental protocol that governs how data is transmitted over the internet. It's responsible for breaking down data into packets and reassembling them at the receiving end.
Networking Concepts
Understanding of Networking Concepts is crucial for effective communication. You should be familiar with concepts like IP addresses, ports, TCP/IP, and HTTP.
IP addresses are used to identify devices on a network. They're like a unique address that helps devices communicate with each other.
TCP/IP is the foundation of the internet, and it's used for communication between devices. It's like a set of rules that devices follow to talk to each other.
Ports are used to differentiate between different services running on a device. They're like separate doors on a building, each leading to a different room.
HTTP is a protocol used for transferring data over the internet. It's like a set of instructions that devices follow to send and receive data.
Using IPv6
Using IPv6 can be a bit tricky, but it's definitely doable. You'll need to have an Embedded Web Server library that's compiled with support for IPv6's new functions.
To create an instance of HttpServCon that uses IPv6, you'll need to specify the IP version with argument #3. This is crucial if you want to use IPv6.
You can even create two instances of HttpServCon using the same port number, as long as one instance is using IPv4 and the other is using IPv6. This is a handy feature that can be useful in certain situations.
Just remember that the IP version is specified with argument #3 when creating an instance of HttpServCon.
Creating the Server
To start building a web server, we need to create a socket, which is the foundation of our server. This is done using the socket() function in C.
In C, a socket is created using the socket() function, which takes three parameters: the domain of the socket (AF_INET for IPv4), the type of the socket (SOCK_STREAM for TCP), and the protocol (0 to let the system decide).
Our simple HTTP server will be able to handle HTTP GET requests and send back the requested file. If the file doesn’t exist, the server will send back a 404 Not Found error.
Once we have a socket, we need to bind it to a specific IP address and port number. After binding, we make the server listen for incoming client connections.
In this code, we first enter an infinite loop where we accept client connections, read data from the clients, parse the HTTP requests, send back the requested files or 404 errors, and close the client connections.
This server can handle one client connection at a time. If you want to handle multiple client connections simultaneously, you’ll need to modify the server to use multi-threading or fork a new process for each client connection.
Handling Requests and Responses
A web server is essentially a program that listens for incoming requests from clients, processes them, and sends back responses. This involves handling HTTP requests and responses, which is a fundamental aspect of web server programming.
To handle client requests, you need to set up communication between sockets and create threads for communication with multiple clients. This allows your server to handle multiple requests concurrently.
The request line, headers, and optional body make up an HTTP request. Conversely, an HTTP response consists of a status line, headers, and an optional body. Understanding this structure is crucial for handling requests and responses effectively.
When a client connects to your server, you need to handle the incoming request using a function like handle_client(). This function receives the request data, extracts the requested file name, and decodes the URL. It then identifies the file extension, builds an HTTP response containing the requested file, and sends it back to the client.
The build_http_response() function constructs an HTTP response, containing a header and the actual file. It starts by building an HTTP header with the appropriate MIME type based on the file extension. If the file doesn't exist, it creates a 404 Not Found response. Otherwise, it retrieves the file's size and appends the 200 OK header to the response buffer.
You can read data from the client using the read() function and send data to the client using the write() function. Both functions take three parameters: the socket, a buffer to read from or write to, and the number of bytes to read or write.
Implementing Features
Implementing features in a C programming web server is crucial for its robustness and efficiency. Our simple HTTP server can only handle HTTP GET requests and send back the requested file, but we can implement HTTP/1.1 features to make it more robust.
To start, we can add persistent connections and chunked transfer encoding to our server, making it more efficient and capable of handling complex requests. This will also allow us to handle multiple client connections simultaneously, which is essential for a production-level web server.
Adding multi-threading support is another important feature that can be implemented, allowing our server to handle multiple client connections at the same time. This will significantly improve its performance and scalability.
Implementing Features
Implementing features in a server is crucial for its efficiency and robustness. To add HTTP/1.1 features to our simple HTTP server, we can implement persistent connections and chunked transfer encoding.
Persistent connections allow multiple requests to be sent over a single connection, reducing overhead and improving performance. This can be achieved by implementing HTTP/1.1 features such as keep-alive and pipelining.
Chunked transfer encoding is another important feature that enables efficient transfer of large files by breaking them into smaller chunks. Our server can be designed to handle chunked transfer encoding using the Barracuda Server's components for advanced data transfer.
To manage multiple client connections simultaneously, we can add multi-threading support to our server. This involves creating a new thread for each client connection, as described in Example 4, "Adding Multi-threading Support".
To ensure proper synchronization for client requests processing, we need to handle client threads and concurrency control, as explained in Example 9, "Client Thread Handling and Synchronization". This involves using semaphores for wait and check operations, as described in Example 10, "Semaphore Usage".
Implementing a simple HTTP server that can handle multiple client connections requires careful management of resources and synchronization. By using a mutex to protect the server's API calls, as described in Example 7, "Using Multiple Threads", we can ensure thread safety and prevent data corruption.
SharkSSL Stack
The SharkSSL stack is a compact SSL/TLS stack designed specifically for the Barracuda Embedded Web Server. It's easy to use with the Barracuda Server without needing to read the SharkSSL API documentation.
Enabling secure sockets in the Barracuda Server using SharkSSL involves creating an instance of the HttpSharkSslServCon class. This class requires a pointer to a SharkSSL encoded X.509 certificate as the third argument in its constructor.
The SharkSSL stack includes a command line tool called SharkParseCert that converts a regular X.509 certificate to a SharkSSL encoded X.509 certificate. This tool produces a binary representation of a standard ASCII encoded X.509 certificate.
You can use the SharkParseCert tool to produce the SharkSSL certificate as C code or as a binary file. To produce a binary file, you need to use the -be or -le flag, depending on your target platform's endianness.
The SharkParseCert tool requires that the private key file and the certificate request file are separated. It's recommended to use the free command-line tool openssl for certificate creation, as we don't provide tools for handling certificate creation.
Troubleshooting and Testing
Building a web server in C can be challenging, and you might encounter some issues along the way. Troubleshooting these issues is crucial to ensure your web server runs smoothly.
Developing and testing a web server can be done on a host machine before deploying the code into an embedded device. This allows for a complete test of the Embedded Web Server on a host machine.
You can separate content and logic with the help of CSP, making it easier to design a clean interface between the Embedded Web Server code and the firmware.
How to Develop and Test
Developing and testing your Embedded Web Server code can be a complex process, but there are ways to make it more manageable. Building a web server in C can be challenging, and you might encounter some issues along the way.
To develop and test your code, you can use Barracuda C/C++ Server Pages (CSP), which are specifically designed for rapid development on any Unix or Windows machine. You develop, run, and test the code on a host machine before you deploy the code into an embedded device.
Designing a clean interface between the Embedded Web Server code and the firmware is crucial for a successful test. The firmware interface should be simulated such that you can do a complete test of the Embedded Web Server on a host machine.
CSP makes it easier to separate content and logic, allowing a web developer to do a complete GUI design, development, and unit testing offsite. This can save you a lot of time and effort in the long run.
Debug vs Release Build
Debug builds are connected to a DiskIo instance, which has its root set to the html directory. This allows for easier debugging of file access issues.
In release mode, the examples are compiled with a ZipIo instance connected to a HttpResRdr. This is likely done to reduce the size of the executable.
When compiling in debug mode, the html directory is not zipped into a ZIP file. Instead, it remains accessible directly from the file system.
The ZIP file used in release mode can be referenced from the file system, stored directly in flash memory, or inserted into the executable. This flexibility is useful for deployment scenarios.
Advanced Topics
The Barracuda Server includes a number of components for advanced data transfer. These components are used by plugins such as WebDAV for uploading and downloading in asynchronous mode.
The server also supports a number of methods for moving the active socket connection out of the server to a separate thread. This is a key feature for handling high-traffic websites.
The components used for advanced data transfer are documented in the C/C++ reference manual. This manual is a must-read for anyone looking to dive deep into the Barracuda Server's internals.
An introduction to these components can be found in the introduction to the internals. This section provides a solid foundation for understanding the server's advanced features.
Final Steps
As you near the end of building your C programming web server, it's essential to focus on the final steps. Returning a pointer is a crucial aspect of this process.
To handle requests efficiently, you'll want to set up headers properly. This includes copying strings, which is a common operation in web server development.
Setting request headers is a delicate task, and it's essential to check if they're working as expected. This can be done by printing errors or messages when setting headers doesn't work properly.
Connection closure is another critical step, ensuring that your server properly closes connections after each request.
Sources
- https://www.skillseminary.com/c-programming/building-a-web-server-in-c/
- http://realtimelogic.com/ba/doc/en/C/introduction.html
- https://www.chaindesk.ai/tools/youtube-summarizer/build-your-own-web-server-multithreaded-proxy-web-server-in-c-eTvSgOoc_BE
- https://dl.acm.org/doi/fullHtml/10.5555/1767726.1767728
- https://dev.to/jeffreythecoder/how-i-built-a-simple-http-server-from-scratch-using-c-739
Featured Images: pexels.com