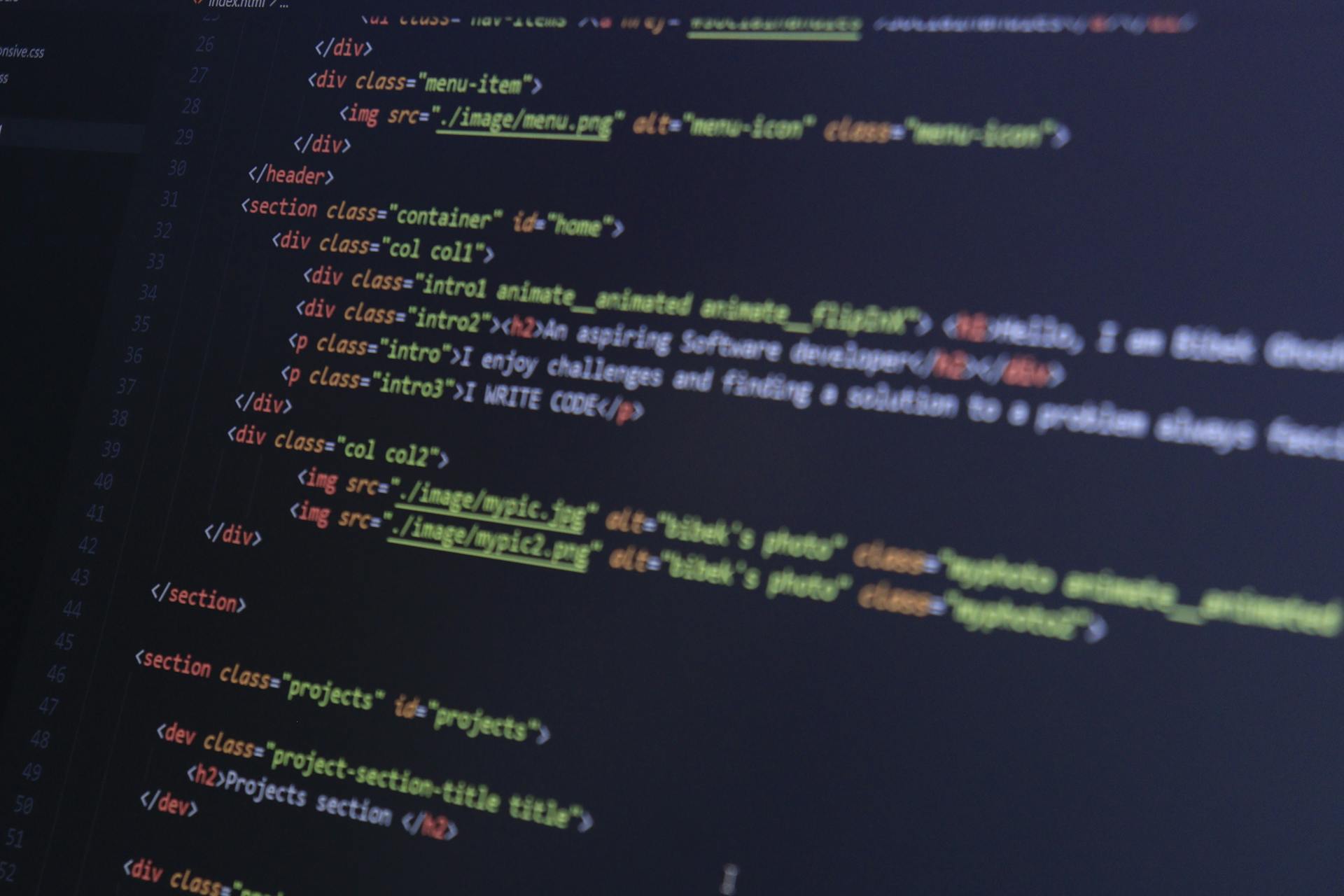
Building scalable and efficient apps in C# requires a solid understanding of the language's capabilities and limitations. With the right approach, you can create high-performance web applications that meet the demands of a growing user base.
One key aspect of C# web development is the use of asynchronous programming, which allows your apps to handle multiple requests simultaneously without blocking. This is particularly important for web applications that rely on database queries or API calls.
To take full advantage of asynchronous programming in C#, you can use the Task Parallel Library (TPL) to simplify concurrent code and improve performance. By leveraging the TPL, you can write more efficient and scalable code that can handle the demands of a high-traffic web application.
A well-designed caching strategy is also crucial for building scalable and efficient apps in C#. By caching frequently accessed data, you can reduce the load on your database and improve response times.
Additional reading: Web Designers Code Crossword Clue
Getting Started
To get started with C# web development, you'll need to set up your development environment.
The first step is to install the necessary tools and frameworks. This involves downloading and installing the .NET SDK, which includes everything you need to build and run C# applications.
To install the .NET SDK, download the .NET Core SDK and follow the installation instructions.
After installation, you'll need to open a new command prompt or terminal and run the command to ensure it's installed correctly. This command is not explicitly stated in the provided examples, but it's implied to be something that displays information about how to use dotnet.
If the command runs successfully, you can proceed to the next step. If you encounter an error stating that 'dotnet' is not recognized as an internal or external command, make sure you opened a new command prompt or terminal after installation.
If this caught your attention, see: Text Content Does Not Match Server-rendered Html
Why Use C#
C# is a great choice for web development because it's an object-oriented language that gives a clear structure to programs.
This structure allows code to be reused, which is fantastic for lowering development costs and saving time too.
The end product will be smooth and efficient to use and will create great successful projects.
Advantages of C#
C# is an object-oriented language that gives a clear structure to programs, allowing code to be reused and lowering development costs and saving time. This makes it easy to create amazing websites and web development projects.
Developers can build web applications that exceed user expectations by harnessing the power of advanced concepts in C#. By implementing real-time communication, creating interactive user interfaces, and optimizing application performance, developers can deliver superior user experiences.
The large community support for C# is a major advantage, especially for web developers. With massive community support from Microsoft and others, developers can seek insight, help, and advice from others, making it easier to tackle complex projects.
Recommended read: Hire Mern Stack Developers
Easy to Use
C# makes it easy to create amazing websites and web development projects with huge support from this language. Object-oriented language gives a clear structure to programs and allows code to be reused.
This means you can lower development costs and save time too. With C#, you can focus on building great projects without getting bogged down in complicated code.
Developers can build web applications that exceed user expectations, delivering superior user experiences. By harnessing the power of advanced concepts in C#, you can create fast, responsive, and feature-rich applications.
For another approach, see: Webflow Multi Language
Big Community Support
Having a large community behind you can make all the difference in your web development journey. Microsoft, the creator of C#, provides massive community support.
Community support is essential for web developers, where they can seek insight, help, and advice from others.
Advantages of Factory
Using a WebApplicationFactory in C# can greatly improve your testing experience. It creates an in-memory TestServer instance of your web application, allowing you to test HTTP requests and responses without needing a full deployment.
This feature is particularly useful for in-memory testing, which enables you to test individual components without the need for external dependencies like databases or authentication systems. This makes your tests faster and more reliable.
With WebApplicationFactory, you can also perform integration testing, which creates an environment configured like production. This allows you to test HTTP requests and responses in a realistic setting.
Here are some key advantages of using WebApplicationFactory:
- Integration Testing: WebApplicationFactory creates an in-memory TestServer instance of the web application being tested.
- Isolated Testing: It enables testing of individual functions without the need to interact with other system components.
These features make it easier to write unit tests and ensure that your applications are working as expected. By leveraging the power of WebApplicationFactory, you can create high-quality web applications that meet the needs of your users.
Recommended read: Building Web App
Building Applications
Building Applications with C# requires a solid foundation in testing to ensure your application meets its intended purpose. Effective testing in ASP.NET Core can help identify and prevent potential issues before they arise in production environments.
You can leverage the vast ecosystem of libraries and frameworks that C# has to offer, such as ASP.NET MVC and ASP.NET Core, which provide architectural patterns and tools for building scalable and maintainable web applications.
Visual Studio, the integrated development environment for C#, offers extensive features and tools that facilitate rapid web application development, including code editors with intelligent auto-completion and debugging tools.
Discover more: Webflow A/b Testing
Understanding Application Factory
Understanding Application Factory is crucial for building high-quality web applications. It simplifies the process of setting up integration and unit tests for your applications.
The WebApplicationFactory class in the Microsoft.AspNetCore.Mvc.Testing namespace makes this process easier. It creates an in-memory TestServer instance of your web application, allowing you to test HTTP requests and responses without needing a full deployment.
Testing is crucial for ensuring the functionality and performance of your applications. By following the steps outlined in the documentation, you can master C# web development and build high-quality web applications.
WebApplicationFactory provides several advantages, including in-memory testing and isolated testing. In-memory testing allows you to test HTTP requests and responses without needing a full deployment, while isolated testing enables you to test individual components without the need for external dependencies.
Here are the key benefits of using WebApplicationFactory:
- Integration Testing: It creates an in-memory TestServer instance of the web application being tested, allowing for testing of HTTP requests and responses in an environment configured like production.
- Isolated Testing: It enables testing of individual functions without the need to interact with other system components, such as databases or authentication systems, making targeted testing quicker and easier.
Dependency injection (DI) is a design pattern in C# that promotes loose coupling between components by dynamically injecting dependencies at runtime. This enhances modularity, testability, and extensibility in web applications.
Building Applications
ASP.NET Core makes testing a breeze, allowing you to verify the functionality and performance of your application before it hits production environments.
Effective testing in ASP.NET Core can help identify and prevent potential issues, ensuring your application meets its intended purpose.
For your interest: Azure Web App Asp.net V4.8
You can use WebApplicationFactory in ASP.NET Core to work with, making testing a seamless part of your development process.
The .NET framework provides many pre-built components and libraries that simplify web development, giving you a vast ecosystem of libraries and frameworks to draw from.
C# developers can leverage these libraries to enhance their web applications, making development faster and more efficient.
Visual Studio offers extensive features and tools that facilitate rapid web application development, including code editors with intelligent auto-completion and debugging tools.
With Visual Studio, you can write clean and efficient code, boosting your productivity and making development a more enjoyable experience.
ASP.NET WebAPI enables seamless communication between the client and server, allowing you to expose your application's functionality to a variety of clients.
With support for content negotiation and a lightweight architecture, WebAPI streamlines the development of scalable and flexible APIs, making it a crucial tool for building modern web applications.
Check this out: Azure Color Code
Entity Framework Core
Entity Framework Core is an object-relational mapping (ORM) framework in C# that simplifies database access and management in web applications.
By mapping database tables to C# objects, Entity Framework Core streamlines data access operations and reduces boilerplate code.
It provides a high-level abstraction layer that promotes code consistency and maintainability, making it easier to focus on building business logic rather than database interactions.
Entity Framework Core comes with features like migrations, change tracking, and LINQ support that accelerate development workflows.
These features enable developers to focus on building business logic rather than database interactions, making development more efficient.
Consider reading: C Programming Web Server
Frequently Asked Questions
Can I build a website using C#?
Yes, you can build a website using C# with .NET, which provides a robust and scalable platform for creating secure, fast, and high-performance web applications. With C#, you can leverage the power of .NET to build websites that meet the demands of millions of users.
Is C# backend or frontend?
C# is primarily used for creating system backends, where its features like Windows server automation and fast code execution shine. It's not typically used for frontend development, but rather for building robust backend systems.
Sources
- https://www.blacklightsoftware.com/blog/posts/2023/march/exploring-the-ins-and-outs-of-c-web-development/
- https://www.restack.io/p/csharp-web-development-answer-beginners
- https://dzone.com/articles/role-of-c-in-building-dynamic-and-secure-web-appli
- https://8seneca.com/blog/c-web-development-advanced-concepts
- https://www.linkedin.com/pulse/modern-web-development-aspnet-mvc-c-webapi-swapnil-kunkekar-zlfic
Featured Images: pexels.com