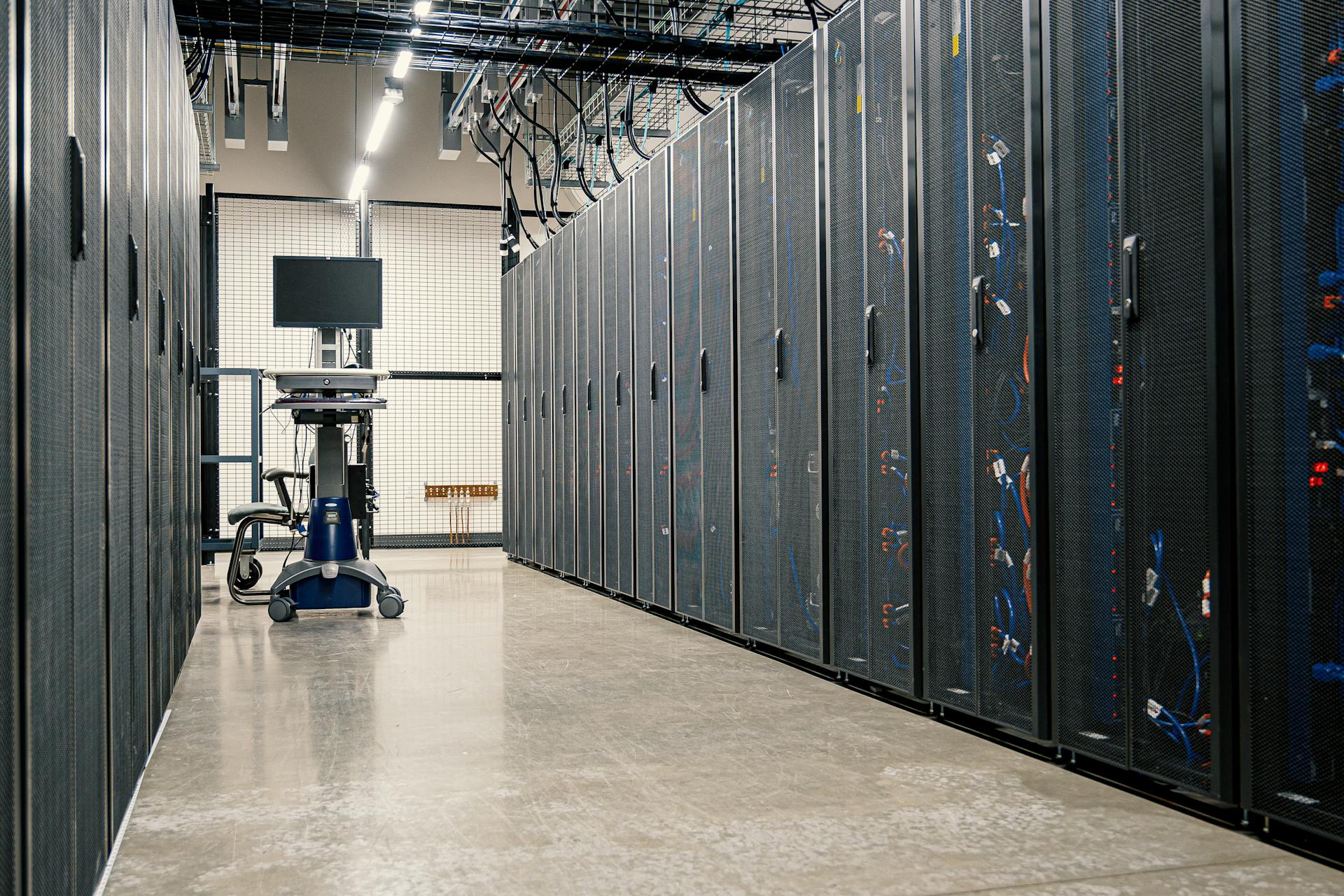
Azure Functions is a serverless compute service that allows you to run small pieces of code, called functions, in response to events or triggers.
To get started with Azure Functions in C#, you'll need to install the Azure Functions Core Tools and create a new function project using the Azure Functions CLI.
The Azure Functions CLI is a command-line interface that allows you to create, deploy, and manage Azure Functions projects.
Azure Functions supports a variety of triggers, including HTTP, timer, and queue triggers, which can be used to invoke your C# functions.
Related reading: Azure Cli vs Azure Powershell
Azure Functions Basics
Azure Functions is a serverless compute service that lets you run small code snippets, or functions, in response to various events.
You can use C# to create Azure Functions, and it's a popular choice among developers.
Azure Functions supports multiple triggers, including HTTP requests, timer triggers, and storage queue triggers.
Each Azure Function has a trigger, which tells the function when to run.
Discover more: How to Run Azure Function Locally in Visual Studio 2022
A C# Azure Function can have one or more bindings, which allow it to access external resources like databases or file systems.
Bindings are configured in the function's code, and they can be used to read or write data.
Azure Functions uses a concept called "bindings" to connect to external resources, which makes it easy to integrate with other services.
You can also use Azure Functions to create event-driven applications, where the function responds to events like button clicks or message queues.
Event-driven programming is a powerful approach to building scalable and maintainable applications.
Azure Functions has a built-in timer trigger that allows you to schedule functions to run at specific times or intervals.
This is useful for tasks like sending reminders or generating reports.
Azure Functions supports a wide range of triggers and bindings, making it a versatile tool for a variety of use cases.
You can use Azure Functions to build everything from simple APIs to complex event-driven systems.
Azure Functions is a great choice for developers who want to build scalable and maintainable applications without worrying about the underlying infrastructure.
Curious to learn more? Check out: Build Azure
Function Triggers
Function triggers are a crucial aspect of Azure Functions C#. To develop functions locally, you need to consider trigger and binding behaviors. For HTTP triggers, you can simply call the HTTP endpoint on the local computer, using http://localhost/.
There are several options to run non-HTTP triggered functions locally, but be aware that targeting live Azure services can impact live service data. You can use connection strings that target live Azure services by adding the appropriate settings in the local.settings.json file.
You can also use a local storage emulator for storage-based triggers, or manually run non-HTTP trigger functions using special administrator endpoints. For more information, see Manually run a non HTTP-triggered function.
During local testing, you must be running the host provided by Core Tools (func.exe) locally.
HTTP triggers allow a function to be invoked by an HTTP request, and there are two different approaches to use: an ASP.NET Core integration model, and a built-in model that doesn't require extra dependencies.
Here are the different approaches to use for HTTP triggers:
- An ASP.NET Core integration model that uses concepts familiar to ASP.NET Core developers
- A built-in model, which doesn't require extra dependencies and uses custom types for HTTP requests and responses
Storage and Emulator
During local development, you can use the local Azurite emulator to test Azure Storage bindings without connecting to remote storage services. This emulator integrates with Visual Studio Code and Visual Studio, and can also be run from the command prompt using npm.
To use the Azurite emulator, you need to set the AzureWebJobsStorage connection in the local.settings.json file to "UseDevelopmentStorage=true". This setting tells the local Functions host to use Azurite for the default AzureWebJobsStorage connection.
Here are some things to keep in mind when using storage emulation during local execution:
- You must have Azurite installed and running.
- You should test with an actual storage connection to Azure services before publishing to Azure.
- When you publish your project, don't publish the AzureWebJobsStorage setting as UseDevelopmentStorage=true.
Files
When working on a local project, you'll notice several important files that help your code run smoothly. The host.json file is a crucial one, and you can learn more about it by checking out the host.json reference.
The local.settings.json file contains settings used by Core Tools when running locally, including app settings. You can find more information about this file in the local settings file.
Suggestion: Azure Function Host.json
To keep your local settings file from being accidentally published to a Git repository, you'll want to take a look at the .gitignore file. This file prevents the local.settings.json file from being published.
If you're working with Visual Studio Code, you'll also see the .vscode\extensions.json file, which is a settings file used when opening the project folder in Visual Studio Code.
Here are some key files to know about:
Storage Emulator
You can use the local Azurite emulator during local development to test functions with Azure Storage bindings without connecting to remote storage services. Azurite integrates with Visual Studio Code and Visual Studio, and you can also run it from the command prompt using npm.
To use Azurite, you need to have it installed and running. You should test with an actual storage connection to Azure services before publishing to Azure.
Azurite connects to the local Functions host using a setting in the Values collection of the local.settings.json file. This setting tells the local Functions host to use Azurite for the default AzureWebJobsStorage connection.
For another approach, see: Azure Api Connection
Here are some considerations to keep in mind when using storage emulation during local execution:
- You must have Azurite installed and running.
- You should test with an actual storage connection to Azure services before publishing to Azure.
- When you publish your project, don't publish the AzureWebJobsStorage setting as UseDevelopmentStorage=true.
The AzureWebJobsStorage setting must always be the connection string of the storage account used by your function app.
Development and Deployment
Development and deployment are crucial steps in building Azure Functions in C#. You can use Visual Studio Code, Azure Functions Core Tools, or other integrated development environments (IDEs) like Eclipse, Gradle, IntelliJ IDEA, Quarkus, or Spring Cloud to create and publish your functions.
For local development, you'll need to configure your project to use an Azure Storage account. This can be done by setting the AzureWebJobsStorage key to a valid connection string or using the Azurite emulator. To set the storage account connection string, navigate to your storage account in the Azure portal, copy the connection string, and update the local.settings.json file in your project.
You can deploy your project files to Azure using the func azure functionapp publish command. This command publishes your project files as a .zip deployment package and can be done from the command prompt or terminal window. Make sure you've already created a function app in your Azure subscription, as the command will deploy your project code to this function app resource.
On a similar theme: Azure Blob Storage Move Files between Containers C#
Configure for Development
To configure your Azure Functions project for development, you need to set up your local environment. Start by installing the Azure Functions Core Tools, which provide an integrated development and publishing experience.
The recommended way to install Core Tools depends on your operating system. For Windows, you can download and run the Core Tools installer. For macOS and Linux, you can install Homebrew and then run the `brew install` command to install the Core Tools.
Once you have the Core Tools installed, you need to configure your project for local development. This involves setting up an Azure Storage account or using the Azurite emulator. To use the emulator, set the `AzureWebJobsStorage` key to `UseDevelopmentStorage=true` in your `local.settings.json` file.
If you're using a .NET project, you'll also need to install the necessary NuGet packages for your binding extensions. You can do this by adding references to the specific NuGet packages in your project.
Take a look at this: Azure Cli Macos
Here's a list of the tools and settings you need to configure for development:
- Visual Studio Code or another code editor
- Azure Functions Core Tools
- Azure Storage account or Azurite emulator
- NuGet packages for binding extensions (for .NET projects)
By following these steps, you'll be able to set up your Azure Functions project for local development and start building and testing your functions.
Deployment
Deployment is a crucial step in the development process, and Azure Functions makes it relatively straightforward. You can deploy your project to Azure using the func azure functionapp publish command, which publishes project files from the current directory to the function app as a .zip deployment package.
To deploy your project, you must have already created a function app in your Azure subscription. Core Tools deploys your project code to this function app resource. If the project requires compilation, it's done remotely during deployment.
The deployment process overwrites existing files in the remote function app deployment. A project folder may contain language-specific files and directories that shouldn't be published, which are listed in a .funcignore file in the root project folder.
Curious to learn more? Check out: Azure Function Disappeared after Deployment
You can also create function app resources using Visual Studio Code, Azure CLI, Azure PowerShell, ARM templates, or Bicep templates. Additionally, you can create these resources in the Azure portal.
Here are some key things to keep in mind when deploying your project:
The deployment payload should match the output of a dotnet publish command, without the enclosing parent folder. The zip archive should contain the following files:
- .azurefunctions/
- extensions.json
- functions.metadata
- host.json
- worker.config.json
- Your project executable (a console app)
- Other supporting files and directories peer to that executable
These files are generated by the build process and shouldn't be edited directly. When preparing a zip archive for deployment, compress only the contents of the output directory, not the enclosing directory itself.
Core Packages
To get started with developing Azure Functions, you'll need to install the required core packages.
The two essential packages are Microsoft.Azure.Functions.Worker and Microsoft.Azure.Functions.Worker.Sdk. These packages are required to run your .NET functions in an isolated worker process.
You can think of these packages as the foundation for your Azure Functions project, providing the necessary functionality for your code to run smoothly.
For your interest: Microsoft Azure Dev
Service Connections
Service connections are a crucial part of Azure Functions, and they can be secured and managed with ease. Azure Functions takes advantage of the application settings functionality of Azure App Service to store connection data securely, and you can access it at runtime as environment variable name-value pairs.
You can store connection data in application settings and reference it in your triggers and bindings using environment variable names. This approach makes your apps more secure and easier to manage across environments. For example, you can set the application setting name instead of the actual connection string in your trigger definition.
Azure Functions also supports identity-based connections, which allow you to use a managed identity instead of a secret. This approach is supported on Functions 4.x and later, and it's only available for certain components, such as Azure Blobs, Azure Queues, and Azure SQL Database. Identity-based connections use a managed identity, and the system-assigned identity is used by default, although a user-assigned identity can be specified with the credential and clientID properties.
You might enjoy: Azure Environment Setup and Adf Setup
Here are some supported components for identity-based connections:
Synchronize
Synchronizing settings is crucial for a seamless experience with Azure Functions. You need to ensure that local settings required by your app match the app settings of the function app to which your code is deployed.
Visual Studio Code, Visual Studio, and Azure Functions Core Tools can help you synchronize app settings with local settings in your project. These tools make it easier to manage settings and avoid discrepancies.
You can use Visual Studio Code to synchronize settings, making it a convenient option for developers who prefer this code editor. This tool streamlines the process of matching local and app settings.
Visual Studio and Azure Functions Core Tools are also viable options for synchronizing settings. They provide similar functionality to Visual Studio Code, ensuring that your local and app settings are in sync.
Here are some tools you can use to synchronize settings:
- Visual Studio Code
- Visual Studio
- Azure Functions Core Tools
Connect to Services
Connecting to services is a crucial aspect of Azure Functions, and it's made easier with the extensive set of bindings provided by Functions.
You can connect to services without having to work with client SDKs, thanks to the binding extensions provided by Functions. This makes it easier to read data from and write data to other cloud services.
Functions securely stores connection data, and you shouldn't include it in your code. Instead, you should store it in application settings, which are encrypted and can be accessed at runtime as environment variable name-value pairs.
Azure Functions takes advantage of the application settings functionality of Azure App Service to help you more securely store strings, keys, and other tokens required to connect to other services.
To configure a binding directly with a connection string or key, you can't do it. You should set the application setting name instead of the actual connection string.
For more insights, see: Azure Data Studio Connect to Azure Sql
Here's a list of common application settings used for connections:
You can also use identity-based connections, which use a managed identity and are supported on Functions 4.x and later.
For example, you can use the WEBSITE_AZUREFILESCONNECTIONSTRING and WEBSITE_CONTENTSHARE settings when connecting to Azure Files on the storage account used by your function app.
Identity-based connections are only supported on Functions 4.x and later, so if you're using version 1.x, you must first migrate to version 4.x.
Some connections in Azure Functions can be configured to use an identity instead of a secret, and support depends on the runtime version and the extension using the connection.
For more information, see the "Configure an identity-based connection" section.
Worth a look: How to Access Azure Key Vault in C#
Function Configuration
Function configuration is a crucial aspect of Azure Functions development in C#. You have direct access to the configuration pipeline for your app when using the isolated worker model.
In this pipeline, you can add configurations, inject dependencies, and run your own middleware. The ConfigureFunctionsWorkerDefaults method is used to add the settings required for the function app to run, including default converters, JsonSerializerOptions, and Azure Functions logging.
For more insights, see: Azure App Config
You can also use the FunctionsApplication.CreateBuilder() method to add these settings, which includes default converters, JsonSerializerOptions, and Azure Functions logging, as well as applying other defaults from Host.CreateDefaultBuilder().
To configure app-specific settings, you can call the ConfigureAppConfiguration method on HostBuilder one or more times to add any configuration sources required by your code. This allows you to set any app-specific configurations during initialization.
Here are some of the configurations you can include in your function method signature:
- Default set of converters.
- Default JsonSerializerOptions to ignore casing on property names.
- Azure Functions logging.
- Output binding middleware and features.
- Function execution middleware.
- Default gRPC support.
Configure Identity-Based Connection
Configuring an identity-based connection in Azure Functions is a powerful feature that allows you to securely connect to various services without exposing sensitive information.
To use an identity-based connection, you need to ensure that your runtime version is 4.x or later, as this feature is only supported in Functions 4.x. If you're using an older version, you'll need to migrate to 4.x first.
Azure Functions supports identity-based connections for various services, including Azure Blobs, Queues, Tables, SQL Database, Event Hubs, Service Bus, Event Grid, Cosmos DB, SignalR, and Durable Functions storage provider.
See what others are reading: Azure Function Change Runtime Version
To configure identity-based connections, you can use the Azure portal or Azure CLI to set up a system-assigned identity or a user-assigned identity. Note that configuring a user-assigned identity with a resource ID is not supported.
Here's a list of supported components and their corresponding extension versions:
When using identity-based connections, note that Azure Files doesn't support using managed identity when accessing the file share. Additionally, when creating a function app with identity-based connections, you should ensure that all uses of AzureWebJobsStorage are able to use the identity-based connection format before changing this connection from a connection string.
A unique perspective: Access Azure Key Vault Using Service Principal C#
Configure Binding Extensions
To configure binding extensions, you need to install the specific NuGet packages for the binding extensions required by your functions. For C# class library projects, add references to these packages, while C# script projects must use extension bundles.
Functions provides extension bundles to make it easy to work with binding extensions in your project. These bundles are versioned and defined in the host.json file, which should already have extension bundles enabled.
A unique perspective: Azure Projects
If you need to add or update the extension bundle in the host.json file, see Extension bundles. Otherwise, if you must use a binding extension or an extension version not in a supported bundle, you can manually install extensions using the func extensions install command.
Here are the key things to keep in mind when configuring binding extensions:
- For C# class library projects, add references to the specific NuGet packages for the binding extensions required by your functions.
- C# script projects must use extension bundles.
- If you need to add or update the extension bundle in the host.json file, see Extension bundles.
- If you must use a binding extension or an extension version not in a supported bundle, you can manually install extensions using the func extensions install command.
By following these steps, you can ensure that your binding extensions are properly configured and ready to use in your Azure Functions project.
Configure Startup
To configure the startup of your Azure Function app, you need to call AddApplicationInsightsTelemetryWorkerService() and ConfigureFunctionsApplicationInsights() during service configuration in your Program.cs file. This is because Application Insights requires this setup to support distributed tracing.
You can add the necessary configuration in the ConfigureServices() delegate, where you can also configure any services or app configuration your project requires. For example, if you're planning to use Application Insights, you need to call AddApplicationInsightsTelemetryWorkerService() and ConfigureFunctionsApplicationInsights() in the ConfigureServices() delegate.
For another approach, see: Azure Insights
If your project targets .NET Framework 4.8, you also need to add FunctionsDebugger.Enable(); before creating the HostBuilder. This should be the first line of your Main() method.
Here are the necessary steps to configure Application Insights:
- Call AddApplicationInsightsTelemetryWorkerService()
- Call ConfigureFunctionsApplicationInsights()
These steps will enable the dependency telemetry required for distributed tracing, and allow you to use Application Insights in your Azure Function app.
Disable Remote
Remote debugging is automatically disabled after 48 hours, so you don't have to worry about forgetting to turn it off.
To manually disable remote debugging, you'll need to access the Azure portal. You can do this by selecting the ellipses in the Hosting section of your project's Publish tab.
In the Azure portal, navigate to the Configuration tab under settings, then choose General Settings. From there, set Remote Debugging to Off and select Save and Continue. This will disable remote debugging for your function app.
After you've disabled remote debugging, your function app will restart, and you'll no longer be able to remotely connect to your processes.
A fresh viewpoint: Azure Function Local Settings Json
Managing Log Levels
Managing Log Levels is crucial for Functions, and it's not as straightforward as you'd think. The Functions host and the isolated process worker have separate configuration for log levels.
You need to apply changes in both places if your scenario requires customization at both layers. This can be a challenge, especially if you're working with a team.
The default behavior for log levels is to capture only warnings and more severe logs. If you want to disable this behavior, you need to remove the filter rule as part of service configuration.
This means you'll need to configure your Application Insights SDK to capture all log levels, not just warnings and more severe logs. This can be done by removing the logging filter that's added by default.
To do this, you'll need to make changes in both your host.json file and your worker code. This can be a bit tedious, but it's necessary to ensure your logs are configured correctly.
Function Execution
Function execution is a crucial aspect of Azure Functions C#. To run your functions locally, you can use the Azure Functions Core Tools. This allows you to test your code as you would a deployed function.
Press F5 to start your project, and you'll be prompted to download and install the Azure Functions Core Tools. You might also need to enable a firewall exception for the tools to handle HTTP requests.
The local Functions host starts to listen on a local port, usually 7071. Any callable function endpoints are written to the output, and you can use these endpoints for testing.
You can test your code just like you would a deployed function, and breakpoints will be hit as expected. This makes it easy to debug your functions locally before deploying them.
If you're using the in-process model, be aware that starting with version 4.0.6517 of the Core Tools, your project must reference version 4.5.0 or later of Microsoft.NET.Sdk.Functions.
See what others are reading: Azure Function Core Tools Vscode
Function Security
Function Security is a top priority when building Azure Functions in C#. One way to enhance security is by using identity-based connections. This feature allows you to configure connections using a managed identity instead of a secret.
Support for identity-based connections is dependent on the runtime version and the extension using the connection. In some cases, a connection string may still be required in Functions even though the service to which you're connecting supports identity-based connections.
Azure Functions 4.x and later versions support identity-based connections. If you're using version 1.x, you'll need to migrate to version 4.x first.
Several components support identity-based connections, including Azure Blobs, Azure Queues, Azure Tables, and Azure SQL Database. Here's a breakdown of the supported components:
When using identity-based connections, Azure Functions will use a managed identity. The system-assigned identity is used by default, but a user-assigned identity can be specified with the credential and clientID properties.
Run Tests
To run tests, you need to set a breakpoint on a test. This is done by navigating to the Test Explorer and selecting Run > Debug Last Run.
You can debug tests just like any other code. Simply set a breakpoint, run the test, and Visual Studio will break into the code at the breakpoint.
To debug tests, you need to run them in debug mode. This is done by navigating to the Test Explorer and selecting Run > Debug Last Run.
Here's a simple step-by-step guide to debugging tests:
- Set a breakpoint on a test.
- Navigate to the Test Explorer.
- Click on Run > Debug Last Run.
By following these steps, you should be able to debug your tests with ease.
Function Packaging and Deployment
Function packaging is a crucial step in deploying your Azure Functions C# project. A function app provides an execution context in Azure where your functions run, and it's the unit of deployment and management for your functions.
You can create a function app using Visual Studio Code, Azure CLI, Azure PowerShell, ARM templates, or Bicep templates. Alternatively, you can create it in the Azure portal.
A unique perspective: Azure Function .net 8
To publish your local code to a function app in Azure, use the `func azure functionapp publish` command. This command publishes project files from the current directory to the function app as a .zip deployment package.
A project folder may contain language-specific files and directories that shouldn't be published. Excluded items are listed in a `.funcignore` file in the root project folder.
Your project is deployed so that it runs from the deployment package by default. To disable this recommended deployment mode, use the `--nozip` option. A remote build is performed on compiled projects, which can be controlled by using the `--no-build` option.
Here are the deployment requirements for running .NET functions in the isolated worker model in Azure:
The deployment payload should match the output of a dotnet publish command, without the enclosing parent folder. The zip archive should be made from the following files: `.azurefunctions/`, `extensions.json`, `functions.metadata`, `host.json`, `worker.config.json`, your project executable (a console app), and other supporting files and directories peer to that executable.
Intriguing read: Azure Auth Json Website Azure Ad Authentication
Function Performance and Optimization
Function performance and optimization are crucial for Azure Functions C# developers. To improve performance around cold start, you should enable certain options.
Upgrading your core dependencies is a good starting point. You should upgrade Microsoft.Azure.Functions.Worker to version 1.19.0 or later, and Microsoft.Azure.Functions.Worker.Sdk to version 1.16.4 or later.
Here are the specific updates you should make: Upgrade Microsoft.Azure.Functions.Worker to version 1.19.0 or later.Upgrade Microsoft.Azure.Functions.Worker.Sdk to version 1.16.4 or later.Add a framework reference to Microsoft.AspNetCore.App, unless your app targets .NET Framework.
Additionally, an optimized executor is enabled by default starting with version 1.16.2 of the SDK, so no further configuration is required for this.
See what others are reading: Windows Azure Sdk for Java
Function Deployment and Publishing
Function deployment and publishing are crucial steps in getting your C# Azure Functions up and running. You can deploy your project files directly to your function app using zip deployment.
To deploy your project files, you can use the `func azure functionapp publish` command. This command deploys function project files directly to your function app using zip deployment.
Explore further: Azure Pipeline Deploy Function App
A function app is the unit of deployment and management for your functions, and it's composed of one or more individual functions that are managed, deployed, and scaled together.
You can create a function app in one of several ways, including using Visual Studio Code, programmatically using Azure CLI, Azure PowerShell, ARM templates, or Bicep templates, or in the Azure portal.
If you're using Visual Studio, you can publish your project to Azure by right-clicking the project and selecting Publish, then selecting Azure and following the prompts.
The Azure Functions Core Tools support three types of deployment: project files, Azure Container Apps, and Kubernetes cluster. You can use the `func azure functionapp publish` command to deploy your project files.
To deploy your project files, you'll need to have already created a function app in your Azure subscription. You can create a function app using the Azure portal, Azure CLI, or Azure PowerShell.
The deployment payload should match the output of a `dotnet publish` command, though without the enclosing parent folder. The zip archive should be made from the following files: `.azurefunctions/`, `extensions.json`, `functions.metadata`, `host.json`, `worker.config.json`, your project executable, and other supporting files and directories.
Take a look at this: Create Sample Azure Function for .net Frame Work
Here's a summary of the deployment requirements for .NET functions in the isolated worker model in Azure:
Function Integration and Best Practices
Function integration with Azure Functions requires careful consideration of several key points. The support for Azure Functions with .NET Aspire is currently in preview, and during this period, Functions projects are deployed as Azure Container Apps resources without event-driven scaling.
To use .NET Aspire with Azure Functions, you should use the IHostApplicationBuilder version of host instance startup in your Program.cs file. This allows you to call builder.AddServiceDefaults() to add .NET Aspire service defaults to your Functions project.
Here are some key considerations for .NET Aspire integration with Azure Functions:
- Trigger and binding configuration through Aspire is currently limited to specific integrations.
- Do not configure the Storage emulator for any connections in local.settings.json.
- Most of the application configuration should come from the Aspire app host project.
- Remove any direct Application Insights integrations from your Functions project when using Aspire.
ASP.NET Core Integration
ASP.NET Core Integration is a powerful feature that allows you to work with the underlying HTTP request and response objects using types from ASP.NET Core. This model is available only to apps targeting .NET Core, and not to apps targeting .NET Framework.
Suggestion: Azure Core
To enable ASP.NET Core integration for HTTP, you need to add a reference to the Microsoft.Azure.Functions.Worker.Extensions.Http.AspNetCore package, version 1.0.0 or later. This package is required to use the ASP.NET Core types.
Here are the steps to update your project for ASP.NET Core integration:
- Add a reference in your project to the Microsoft.Azure.Functions.Worker.Extensions.Http.AspNetCore package, version 1.0.0 or later.
- Update your project to use the specific package versions.
- In your Program.cs file, update the host builder configuration to call ConfigureFunctionsWebApplication().
- Update any existing HTTP-triggered functions to use the ASP.NET Core types.
For example, you can use the standard HttpRequest and an IActionResult in your functions, as shown in the following example: [Function("HttpFunction")] public IActionResult Run( [HttpTrigger(AuthorizationLevel.Anonymous, "get")] HttpRequest req) { return new OkObjectResult($"Welcome to Azure Functions, {req.Query["name"]}!"); }
For another approach, see: Azure Tokencredential C# Example
.NET Aspire Integration Best Practices
When evaluating .NET Aspire with Azure Functions, consider that support is currently in preview, and Functions projects are deployed as Azure Container Apps resources without event-driven scaling. This means Azure Functions support is not available for apps deployed in this mode.
You should use the IHostApplicationBuilder version of host instance startup in your Program.cs, which allows you to call builder.AddServiceDefaults() to add .NET Aspire service defaults to your Functions project.
Broaden your view: Azure Functions Are Built and Deployed
Aspire uses OpenTelemetry for monitoring, and you can configure it to export telemetry to Azure Monitor through the service defaults project. Be aware that direct integration with Application Insights is not recommended and can lead to runtime errors with version 2.22.0 of Microsoft.ApplicationInsights.WorkerService.
For Functions projects enlisted into an Aspire orchestration, most of the application configuration should come from the Aspire app host project. Avoid setting things in local.settings.json, except for the FUNCTIONS_WORKER_RUNTIME setting.
Do not configure the Storage emulator for any connections in local.settings.json, as this can prompt some IDEs to start a version of the emulator that can conflict with the version that Aspire uses.
Some Aspire integrations are enabled to provide connections through a call to WithReference() on the project resource, as shown in the following integrations table:
You can use WithEnvironment() to pass the connection information for the trigger or binding to resolve, as shown in the following example.
Function Considerations and Next Steps
As you explore Azure Functions with C#, it's essential to consider a few key points to ensure a smooth experience. Support for Azure Functions with .NET Aspire is currently in preview, so keep in mind that Functions projects are deployed as Azure Container Apps resources without event-driven scaling during this period.
Azure Functions support is not available for apps deployed in this mode, so be aware of the limitations. Trigger and binding configuration through Aspire is currently limited to specific integrations.
To get started, your Program.cs should use the IHostApplicationBuilder version of host instance startup, which allows you to call builder.AddServiceDefaults() to add .NET Aspire service defaults to your Functions project. This will enable you to take advantage of Aspire's features and integrations.
Here are some key considerations to keep in mind:
- Aspire uses OpenTelemetry for monitoring, which can be configured to export telemetry to Azure Monitor through the service defaults project.
- Direct integration with Application Insights is not recommended in Aspire and can lead to runtime errors with version 2.22.0 of Microsoft.ApplicationInsights.WorkerService.
- For Functions projects enlisted into an Aspire orchestration, most of the application configuration should come from the Aspire app host project.
- It's recommended to avoid setting environment variables in local.settings.json, except for the FUNCTIONS_WORKER_RUNTIME setting.
- Do not configure the Storage emulator for any connections in local.settings.json to avoid conflicts with Aspire.
Considerations for .NET Preview Versions
Using .NET preview versions with Azure Functions requires some extra consideration. You must use Visual Studio Preview to author your functions, as it's the only version that supports building Azure Functions projects with .NET preview SDKs.
To ensure you have the latest Functions tools and templates, you can update your tools by following the instructions provided. This is especially important during the preview period, as your development environment might have a more recent version of the .NET preview than the hosted service.
Your development environment might have a more recent version of the .NET preview than the hosted service, which can cause your function app to fail when deployed. To address this, you can specify the version of the SDK to use in global.json.
Function apps running on Windows can experience increased cold start times when compared against earlier GA versions due to the just-in-time loading of preview frameworks. This is a known issue that you should be aware of when using .NET preview versions.
Here are the key considerations for using .NET preview versions with Azure Functions:
- Use Visual Studio Preview to author your functions.
- Update your tools to the latest Functions version.
- Specify the version of the SDK to use in global.json.
- Be aware of increased cold start times on Windows.
Next Steps
Now that you've considered the key factors in choosing the right function for your project, it's time to take the next steps.
To get the most out of Azure Functions, be sure to learn more about best practices for using them effectively. This will help you optimize your functions for performance and scalability.
Migrating your .NET apps to the isolated worker model is a great way to improve their efficiency and reliability. This approach can help you take advantage of the latest features and improvements in Azure Functions.
By taking these next steps, you'll be able to build more robust and scalable functions that meet the needs of your project.
Frequently Asked Questions
What language does Azure Functions use?
Azure Functions support multiple languages, including C#, F#, JavaScript, and more. Discover the full range of supported languages and create scalable serverless applications with Azure Functions.
Sources
- https://learn.microsoft.com/en-us/azure/azure-functions/functions-develop-local
- https://learn.microsoft.com/en-us/azure/azure-functions/functions-reference
- https://learn.microsoft.com/en-us/azure/azure-functions/functions-develop-vs
- https://learn.microsoft.com/en-us/azure/azure-functions/functions-run-local
- https://learn.microsoft.com/en-us/azure/azure-functions/dotnet-isolated-process-guide
Featured Images: pexels.com