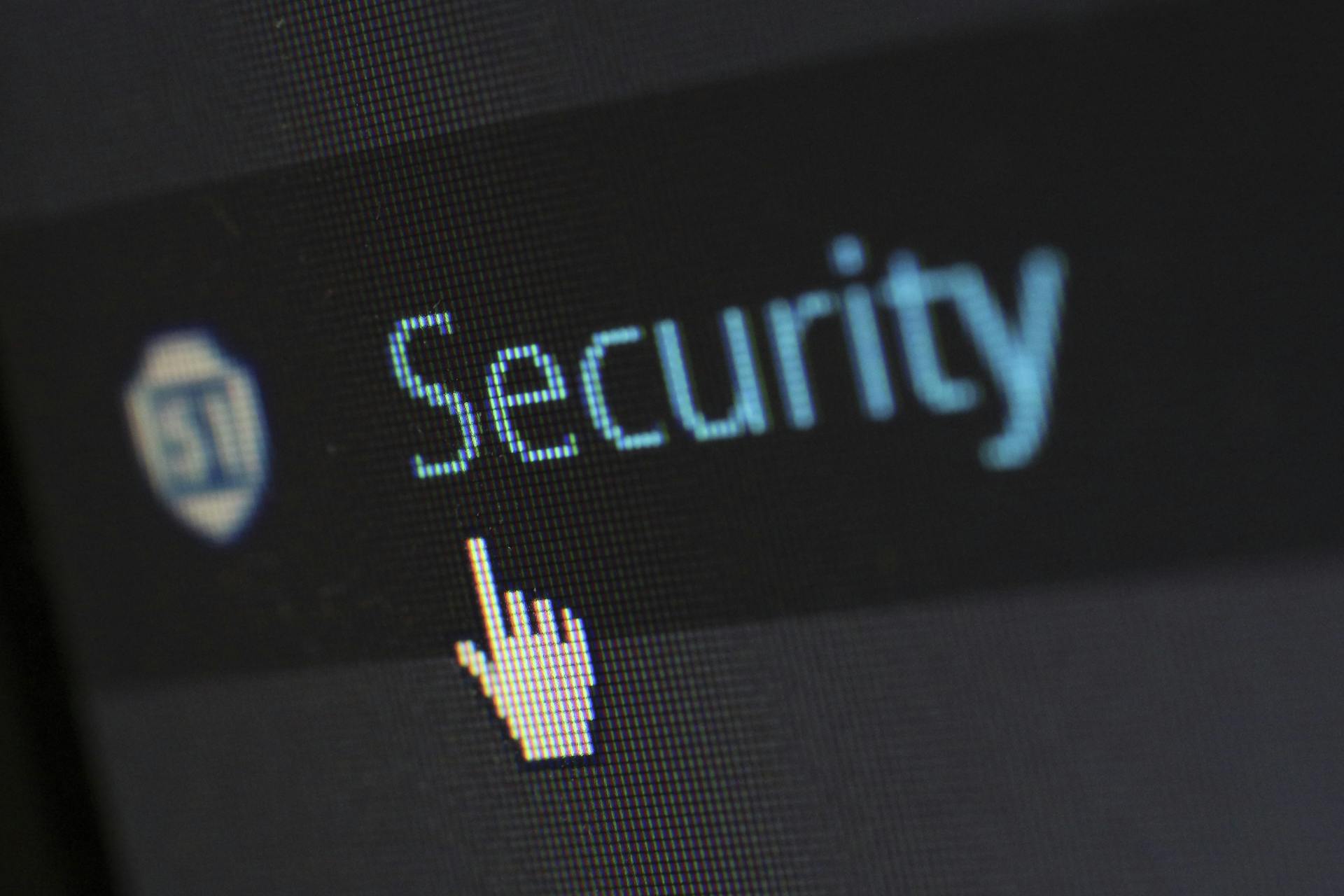
Using Azure Identity Library for Authentication is a straightforward process with the Azure TokenCredential C# example. You can leverage the Azure Identity library to acquire tokens for your Azure services.
The Azure Identity library provides a simple and secure way to authenticate with Azure services. This is achieved through the use of the Azure TokenCredential class, which allows you to obtain tokens for your Azure resources.
To get started, you'll need to install the Azure Identity library in your project. This can be done using NuGet Package Manager or the .NET CLI.
The Azure Identity library supports multiple authentication methods, including client secret, certificate, and managed identity.
A different take: Asp.net Core Azure Ad Authentication Example
Authentication Approaches
There are several authentication approaches you can use when working with Azure TokenCredential in C#. The recommended approach is token-based authentication.
You can authenticate to Azure using either an application service principal for local development or the developer's Azure credentials. However, this approach doesn't accurately replicate the permissions the app will run with in production.
Readers also liked: Azure Auth Json Website Azure Ad Authentication
For apps hosted on Azure, you should authenticate to Azure resources using a managed identity. This approach is discussed in more detail at authentication in server environments.
In server environments, each app should be assigned a unique application identity per environment. The type of service principal to use depends on where your app is running.
Here are the main authentication methods for apps hosted in different environments:
You can also use the DefaultAzureCredential class, which allows apps to use different authentication methods depending on the environment in which they're run. This approach is discussed in more detail at introducing DefaultAzureCredential.
The DefaultAzureCredential class tries different authentication methods in a cascading way, starting with EnvironmentCredential and ending with InteractiveBrowserCredential. However, this approach can take too much time and it's not always clear what authentication method got executed.
If you want to be in charge of what authentication methods get evaluated, you can use the DefaultAzureCredentialOptions class. This class allows you to exclude certain authentication methods, such as ExcludeEnvironmentCredential or ExcludeManagedIdentityCredential.
Another approach is to use the ChainedTokenCredential class, which allows you to define your preferred authentication methods and their respective order of execution. This is a simple way to handle authentication in different environments.
Related reading: How to Access Azure Key Vault in C#
Token-Based Authentication
Token-based authentication is the recommended approach for authenticating to Azure resources. This method offers several advantages over authenticating with connection strings.
The Azure Identity library provides classes that support token-based authentication, allowing apps to seamlessly authenticate to Azure resources whether they're in local development, deployed to Azure, or deployed to an on-premises server.
Token-based authentication methods scope access to the resource to only the app(s) intended to access the resource, following the principle of least privilege. This means that only the necessary permissions are granted to the app.
There are several types of token-based authentication, including application service principal for local development, managed identity for Azure-hosted apps, and application service principal for on-premises apps.
Here's a summary of the recommended authentication methods for different scenarios:
Each app should be assigned a unique application identity per environment in which the app is run. In Azure, an application identity is represented by a service principal, a special type of security principal intended to identify and authenticate apps to Azure.
The Azure.Identity package acquires and manages Microsoft Entra tokens for you, making it easy to use token-based authentication as easy as a connection string.
Suggestion: Azure App Insights vs Azure Monitor
Default Credential
The DefaultAzureCredential class is an abstract base class that contains two methods to request an access token. Microsoft provides many types that implement this abstract class, including the DefaultAzureCredential type.
It's an opinionated, ordered sequence of mechanisms for authenticating to Microsoft Entra ID. Each authentication mechanism is a class derived from the TokenCredential class and is known as a credential. At runtime, DefaultAzureCredential attempts to authenticate using the first credential. If that credential fails to acquire an access token, the next credential in the sequence is attempted, and so on, until an access token is successfully obtained.
DefaultAzureCredential tries different authentication methods in a cascading way. The first authentication method that provides valid authentication information will be executed. The aim is that this single credential gets resolved in both your local development environment and Azure.
Here are the authentication methods that DefaultAzureCredential tries, in this particular order:
- EnvironmentCredential: looks for environment variables, such as AZURE_CLIENT_ID and AZURE_CLIENT_SECRET to authenticate
- ManagedIdentityCredential: attempts to authenticate using a managed identity that is assigned to the deployment environment (if any)
- SharedTokenCacheCredential: tries to authenticate using tokens in the local cache shared between Microsoft applications
- VisualStudioCredential: tries authentication to Azure AD via the logged-in user in Visual Studio
- VisualStudioCodeCredential: tries authentication to Azure AD via the logged-in user in Visual Studio Code
- AzureCliCredential: attempts to authenticate by using the current user context of Azure CLI
- InteractiveBrowserCredential: launches the browser to interactively authenticate a user
You can exclude certain authentication methods by using the DefaultAzureCredentialOptions class. This can be useful if you want to be in charge of what authentication methods get evaluated.
It's best to create a single instance of DefaultAzureCredential and reuse it throughout your application. This approach is more efficient because it leverages the credential's internal token cache, reducing the need for repeated authentication calls.
TokenCredential Class
The TokenCredential class is an abstract base class that contains two methods to request an access token. It's the foundation for various credential types that implement this abstract class.
The TokenCredential class is implemented by multiple types provided by Microsoft, including EnvironmentCredential, ManagedIdentityCredential, and VisualStudioCredential. These types employ different approaches to acquiring the access token needed to access services in Azure.
Here are the different TokenCredential types:
- EnvironmentCredential: looks for environment variables such as AZURE_CLIENT_ID and AZURE_CLIENT_SECRET to authenticate
- ManagedIdentityCredential: attempts to authenticate using a managed identity that is assigned to the deployment environment (if any)
- VisualStudioCredential: tries authentication to Azure AD via the logged-in user in Visual Studio
- VisualStudioCodeCredential: tries authentication to Azure AD via the logged-in user in Visual Studio Code
- AzureCliCredential: attempts to authenticate by using the current user context of Azure CLI
- InteractiveBrowserCredential: launches the browser to interactively authenticate a user
Each TokenCredential type has its own approach to acquiring the access token, but the goal is always the same: to obtain the token safely and effectively, ensuring smooth and reliable access to Azure.
Consider reading: Azure Data Factory Oauth2 Token
What Does TokenCredential Class Represent?
The TokenCredential class represents a base class for acquiring access tokens in Azure. It contains two methods for requesting an access token.
The TokenCredential class is an abstract base class, meaning it cannot be instantiated directly. Instead, it serves as a blueprint for other credential types that implement its methods.
Explore further: Access Azure Key Vault Using Service Principal C#
Microsoft provides many types that implement this abstract class, including the DefaultAzureCredential class. This class is designed to automatically detect and use the appropriate authentication method for the environment in which the app is running.
Here are some of the credential types that implement the TokenCredential class:
- DefaultAzureCredential
- EnvironmentCredential
- ManagedIdentityCredential
- SharedTokenCacheCredential
- VisualStudioCredential
- VisualStudioCodeCredential
- AzureCliCredential
- InteractiveBrowserCredential
Each of these credential types has its own approach to acquiring the access token needed to access services in Azure.
Chained Token Credential
The Chained Token Credential is a credential type that allows you to define your preferred authentication methods and their respective order of execution.
It functions similarly to DefaultAzureCredential, but with the key difference being that it starts with an empty list of TokenCredentials. This makes it particularly advantageous for production environments, as it puts you in complete control and limits the credentials to only those you actively use.
You can add only the credential types relevant to your needs in the order that works best for you. For instance, you could set it up to use Managed Identity when deployed in Azure and Azure CLI for local development purposes.
Check this out: Default Azure Credential
Here are some examples of how to use ChainedTokenCredential:
This approach can be particularly advantageous for production environments, as it puts you in complete control and limits the credentials to only those you actively use.
Sources
- https://learn.microsoft.com/en-us/dotnet/azure/sdk/authentication/
- https://nestenius.se/azure/default-azure-credentials-under-the-hood/
- https://www.domstamand.com/migrating-to-the-new-csharp-azure-keyvault-sdk-libraries/
- https://yourazurecoach.com/2020/08/13/managed-identity-simplified-with-the-new-azure-net-sdks/
- https://learn.microsoft.com/en-us/azure/service-bus-messaging/service-bus-managed-service-identity
Featured Images: pexels.com