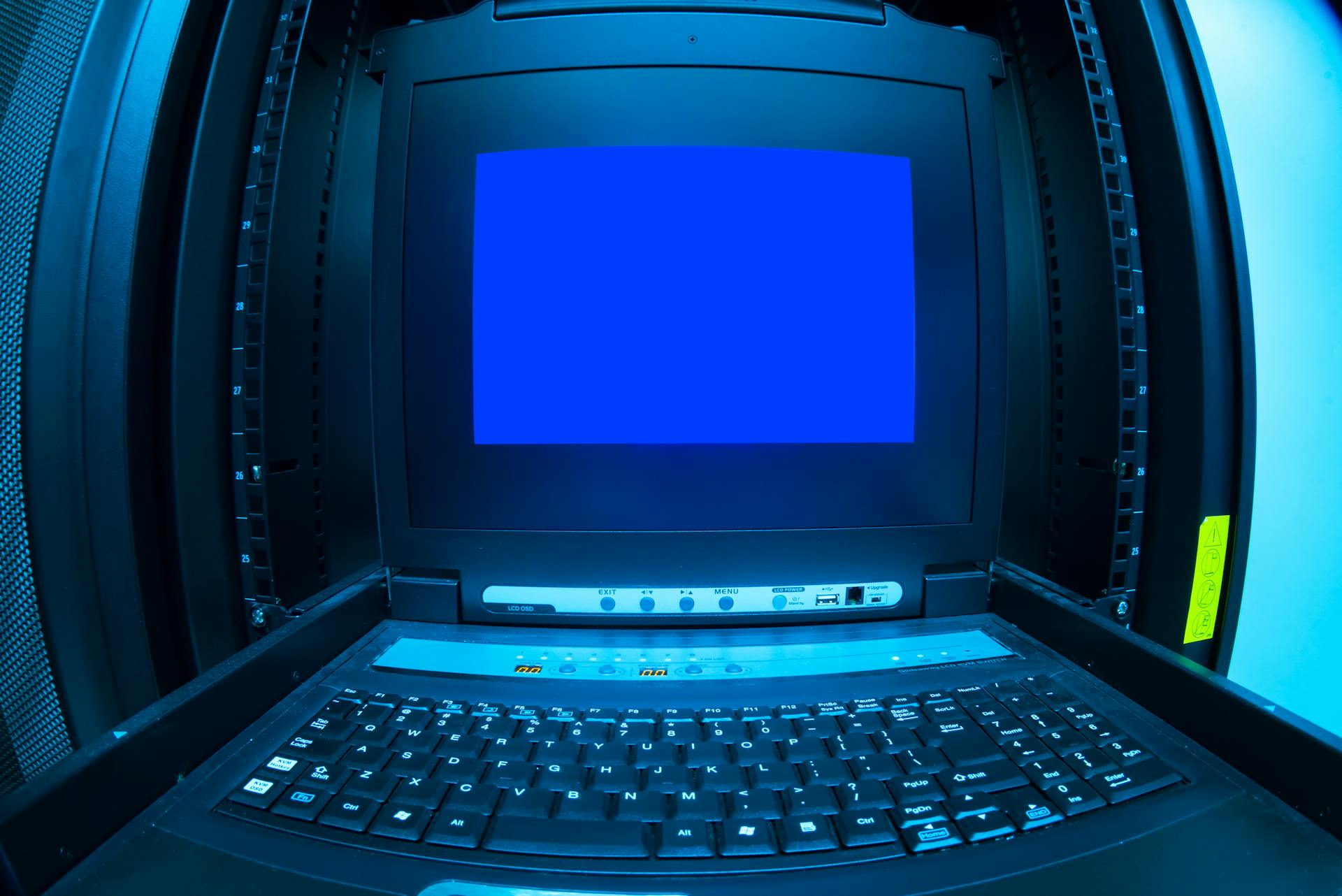
Azure Default Credential is a feature that allows Azure services to access resources without the need for explicit credentials. This is particularly useful for development and testing environments.
The default credential is based on the Azure Identity library, which provides a simple and secure way to authenticate with Azure services. This library is available for multiple programming languages, including Python, Java, and C#.
In Azure, the default credential is used by default when accessing Azure resources, such as storage accounts and Azure Active Directory. This eliminates the need for developers to manually pass credentials with each API call.
This feature is not limited to Azure services, as it can also be used with other cloud providers, such as AWS and Google Cloud.
Explore further: How to Config Git Credential Helper Azure
What is Azure Default Credential
Azure Default Credential is an opinionated, preconfigured chain of credentials that simplifies authentication while developing apps that deploy to Azure. It combines credentials used in Azure hosting environments with credentials used in local development.
Take a look at this: Azure Credentials
DefaultAzureCredential is designed to support many environments, along with the most common authentication flows and developer tools. This makes it a convenient option for developers working with Azure.
The order in which DefaultAzureCredential attempts credentials follows a specific sequence. Here's a breakdown of the order:
You can use the parameterless version of DefaultAzureCredential as follows: DefaultAzureCredential or ChainedTokenCredential.
Using Azure Default Credential
DefaultAzureCredential simplifies authentication while developing apps that deploy to Azure by combining credentials used in Azure hosting environments with credentials used in local development.
The credential chain attempts to authenticate using the sequence's first credential. If that credential fails to acquire an access token, the next credential in the sequence is attempted, and so on, until an access token is successfully obtained.
You can use the parameterless version of DefaultAzureCredential as follows: DefaultAzureCredential. This is the simplest form of using DefaultAzureCredential.
The order in which DefaultAzureCredential attempts credentials follows:
You can customize DefaultAzureCredential by removing a credential from the chain using the corresponding Exclude-prefixed property in DefaultAzureCredentialOptions.
How it Works
Using Azure Default Credential makes authentication a breeze while developing apps that deploy to Azure. It simplifies the process by combining credentials used in Azure hosting environments with those used in local development.
DefaultAzureCredential is an opinionated, preconfigured chain of credentials designed to support many environments and common authentication flows. It's essentially a list of credentials that Azure tries in order.
Here's a breakdown of the order in which DefaultAzureCredential attempts credentials:
If one credential fails to acquire an access token, the next credential in the sequence is attempted until an access token is successfully obtained.
Curious to learn more? Check out: Azure Data Factory Oauth2 Token
PowerShell
To authenticate with Azure PowerShell, run the Connect-AzAccount cmdlet. This cmdlet launches the default web browser to authenticate a user account.
By default, the cmdlet will use interactive authentication, which requires user interaction. However, if interactive authentication can't be supported in the session, the -UseDeviceAuthentication argument can be used instead.
Using the -UseDeviceAuthentication argument forces the cmdlet to use a device code authentication flow, similar to the corresponding option in the Azure CLI credential.
See what others are reading: Azure Auth Json Website Azure Ad Authentication
Service Principals
DefaultAzureCredential provides several options for authenticating service principals, including AzurePipelinesCredential, CertificateCredential, ClientAssertionCredential, and ClientSecretCredential.
AzurePipelinesCredential supports Microsoft Entra Workload ID on Azure Pipelines.
CertificateCredential authenticates a service principal using a certificate.
ClientAssertionCredential authenticates a service principal using a signed client assertion.
ClientSecretCredential authenticates a service principal using a secret.
The following table summarizes the usage of these credentials:
Configuring Azure Default Credential
Configuring Azure Default Credential is a breeze, and it's essential to get it right. You can simplify authentication while developing apps that deploy to Azure by using DefaultAzureCredential, which combines credentials used in Azure hosting environments with credentials used in local development.
To access resources in other clouds, such as Azure Government or a private cloud, configure credentials with the authority argument. AzureAuthorityHosts defines authorities for well-known clouds, and if the authority for your cloud isn't listed, you can explicitly specify its URL.
Here's a quick rundown of the DefaultAzureCredential chain: EnvironmentWorkload IdentityManaged IdentityVisual StudioAzure CLIAzure PowerShellAzure Developer CLIInteractive browser This chain attempts credentials in a specific order, and you can customize it by removing credentials using Exclude-prefixed properties in DefaultAzureCredentialOptions.
Recommended read: Azure Identity
Enable Interactive
DefaultAzureCredential comes with interactive authentication disabled by default, but it can be enabled with a simple keyword argument. This allows the credential to fall back to interactively authenticating via the system's default web browser when no other credential is available.
To enable interactive authentication, you can use the following code: By default, interactive authentication is disabled in DefaultAzureCredential and can be enabled with a keyword argument.
This feature was introduced in version 1.19.0, so make sure your DefaultAzureCredential is up to date to take advantage of it.
Here's a summary of the interactive authentication process:
Specify a User-Assigned
You can configure DefaultAzureCredential to authenticate with a user-assigned managed identity. This is useful when working with Azure resources. To do this, use the managed_identity_client_id keyword argument.
Alternatively, you can set the environment variable AZURE_CLIENT_ID to the identity's client ID. This is a convenient way to configure multiple credentials to authenticate to the same cloud or when the deployed environment needs to define the target cloud.
For more insights, see: Identity Provider Azure
Here are some examples of how to do this:
- Create a credential with the managed_identity_client_id argument: `DefaultAzureCredential(managed_identity_client_id='your_client_id')`
- Set the AZURE_CLIENT_ID environment variable: `export AZURE_CLIENT_ID='your_client_id'`
Note that not all credentials require this configuration. For example, credentials that authenticate through a development tool, such as AzureCliCredential, use that tool's configuration. Similarly, VisualStudioCodeCredential accepts an authorityHost argument but defaults to the authorityHost matching Visual Studio Code's Azure: Cloud setting.
Custom Flow Definition
You can customize the authentication flow with ChainedTokenCredential to suit your needs. This allows you to combine multiple credential instances to define a customized chain of credentials.
While DefaultAzureCredential is generally the quickest way to get started developing applications for Azure, more advanced users may want to customize the credentials considered when authenticating. ChainedTokenCredential enables users to combine multiple credential instances to define a customized chain of credentials.
To create a customized chain of credentials, you can use ChainedTokenCredential. This credential is particularly useful when you need to authenticate using two or more credential instances.
ChainedTokenCredential is a better choice than DefaultAzureCredential when you have many credential exclusions configured. This is because ChainedTokenCredential requires less code and provides more flexibility in defining your custom authentication flow.
You can create a ChainedTokenCredential that attempts to authenticate using two differently configured instances of ClientSecretCredential. This is demonstrated in the example where a ChainedTokenCredential is used to authenticate the KeyClient from the @azure/keyvault-keys.
InteractiveBrowserCredential is excluded by default, but you can include it in your custom flow by passing true to the constructor DefaultAzureCredential(Boolean) or setting property DefaultAzureCredentialOptions.ExcludeInteractiveBrowserCredential to false.
Troubleshooting and Error Handling
Credentials can raise a CredentialUnavailableError if they're unable to attempt authentication because they lack required data or state. This can happen when an EnvironmentCredential's configuration is incomplete.
Credentials that fail to authenticate will raise an azure.core.exceptions.ClientAuthenticationError. This error has a message attribute that describes why authentication failed, and when raised by DefaultAzureCredential or ChainedTokenCredential, the message collects error messages from each credential in the chain.
For more information on handling specific Microsoft Entra ID errors, you can refer to the Microsoft Entra ID error code documentation.
For more insights, see: Application Id Azure
Error Handling
Error handling is crucial when working with credentials. Credentials raise CredentialUnavailableError when they're unable to attempt authentication because they lack required data or state.
For example, EnvironmentCredential raises this exception when its configuration is incomplete. This can happen if you're missing a required environment variable or setting.
Credentials also raise azure.core.exceptions.ClientAuthenticationError when they fail to authenticate. ClientAuthenticationError has a message attribute, which describes why authentication failed.
When raised by DefaultAzureCredential or ChainedTokenCredential, the message collects error messages from each credential in the chain. This can be helpful in understanding what went wrong during the authentication process.
For more information on handling specific Microsoft Entra ID errors, see the Microsoft Entra ID error code documentation.
Suggestion: Azure Clientid
Token Caching
Token caching is a feature provided by the Azure Identity library that allows apps to cache tokens in memory (default) or on disk (opt-in). This can improve resilience and performance, and reduce the number of requests made to Microsoft Entra ID to obtain access tokens.
Caching tokens in memory is the default option, while caching on disk is an opt-in feature. This means you have flexibility in how you choose to implement token caching for your app.
The Azure Identity library offers both in-memory and persistent disk caching. This is a key benefit of using token caching, as it allows you to choose the caching method that best suits your app's needs.
Token caching can help reduce the number of requests made to Microsoft Entra ID to obtain access tokens. This can improve performance and reduce the load on the Microsoft Entra ID system.
For more information on token caching, see the token caching documentation.
Intriguing read: Azure App Insights vs Azure Monitor
Changed
As you troubleshoot and handle errors in your Azure SDK clients, it's essential to be aware of the changes made to the library. The minimum version of msal was raised to 1.7.0, and six version was raised to 1.12.0.
InteractiveBrowserCredential uses PKCE internally to protect authorization codes, ensuring a secure authentication process. This is a significant change that you should be aware of when implementing authentication in your applications.
CertificateCredential can now load a certificate from bytes instead of a file path, making it easier to integrate with your existing security protocols. To provide a certificate as bytes, use the keyword argument certificate_bytes instead of certificate_path.
User credentials support Continuous Access Evaluation (CAE), a feature that allows for more secure and efficient authentication. This change is particularly relevant for applications that require high security standards.
Here are the key changes to the library:
- InteractiveBrowserCredential uses PKCE
- CertificateCredential can load a certificate from bytes
- User credentials support CAE
These changes are designed to improve the security and efficiency of your Azure SDK clients. By understanding these changes, you can better troubleshoot and handle errors in your applications.
Fixes and Improvements:
In the world of troubleshooting and error handling, it's essential to know what's been fixed and improved in the latest updates. One notable improvement is that authenticating with a single sign-on shared with other Microsoft applications only requires a username when multiple users have signed in.
This is particularly helpful when dealing with large teams or organizations. I recall a time when I had to troubleshoot a similar issue and it was a major time-saver.
Here are some specific fixes and improvements that you should know about:
- Authenticating with a single sign-on shared with other Microsoft applications only requires a username when multiple users have signed in (#8095)
- DefaultAzureCredential accepts an authority keyword argument, enabling its use in national clouds (#8154)
These updates can significantly reduce the time and effort required to resolve authentication issues, making the troubleshooting process much smoother.
Azure Default Credential in Development Tools
The Azure Identity library supports authenticating through developer tools to simplify local development.
To authenticate in your development environment, you can use various developer tools such as the Azure CLI, Azure Developer CLI, or Visual Studio Code. These tools can help you authenticate calls to Azure services without having to manually enter credentials.
You can authenticate via the Azure Developer CLI by running the command azd auth login. This command will launch your default web browser to authenticate you.
Alternatively, you can use the Azure CLI to authenticate. To do this, run the command az login. This will also launch your default web browser to authenticate you.
Check this out: Azure Cli vs Azure Powershell
In Visual Studio Code, you can use the Azure Account extension to authenticate. Once installed, open the Command Palette and run the Azure: Sign In command. This will authenticate you and allow you to make calls to Azure services.
Here are some developer tools you can use to authenticate in your development environment:
Note that the VisualStudioCodeCredential has been removed from the DefaultAzureCredential token chain due to a known issue. However, this change will be reverted in a future release.
Azure Default Credential in Applications
The DefaultAzureCredential simplifies authentication while developing apps that deploy to Azure by combining credentials used in Azure hosting environments with credentials used in local development.
It's designed to support many environments, along with the most common authentication flows and developer tools. The order in which DefaultAzureCredential attempts credentials follows a specific chain, starting with environment variables, then workload identity, managed identity, and so on.
Here's a summary of the order in which DefaultAzureCredential attempts credentials:
Client in Browsers
To authenticate Azure SDK clients within web browsers, you can use the InteractiveBrowserCredential. This credential can be set to use redirection or popups to complete the authentication flow.
First, create an Azure App Registration in the Azure portal for your web application. This is a necessary step before you can use the InteractiveBrowserCredential.
To authenticate with the Azure SDK clients, the InteractiveBrowserCredential is a great option. It's flexible and can adapt to different browser configurations.
If you're using the InteractiveBrowserCredential, you can choose between redirection and popups to complete the authentication flow.
Brokered
Brokered authentication is a way to manage authentication handshakes and token maintenance for connected accounts. It's an application that runs on a user's machine and currently, only the Windows Web Account Manager (WAM) is supported.
To enable support for WAM, you'll need to use the azure-identity-broker package. This package is specifically designed for WAM and can be used to authenticate using the broker plugin documentation.
The azure-identity-broker package is a crucial tool for developers who want to use WAM for authentication. By using this package, you can ensure that your application is properly configured for WAM authentication.
Here are the steps to enable support for WAM using the azure-identity-broker package:
- Install the azure-identity-broker package
- Use the package to authenticate using the broker plugin documentation
By following these steps, you can successfully use WAM for authentication in your application. This will help you manage authentication handshakes and token maintenance for connected accounts, making your application more secure and efficient.
Applications
Applications can use DefaultAzureCredential to simplify authentication while developing apps that deploy to Azure, combining credentials used in Azure hosting environments with credentials used in local development.
DefaultAzureCredential is an opinionated, preconfigured chain of credentials that supports many environments, along with the most common authentication flows and developer tools.
DefaultAzureCredential attempts credentials in a specific order, starting with Environment, which reads a collection of environment variables to determine if an application service principal is configured for the app. If so, it uses these values to authenticate the app to Azure.
The order of credential attempts is as follows:
Applications can use the parameterless version of DefaultAzureCredential as follows: DefaultAzureCredential or ChainedTokenCredential.
Python Client Library
The Azure Identity library for Python is a powerful tool that provides Microsoft Entra ID token authentication support across the Azure SDK.
This library offers a set of TokenCredential/SupportsTokenInfo implementations that can be used to construct Azure SDK clients that support Microsoft Entra token authentication.
You can find the source code for the Azure Identity library on PyPI, Conda, and the API reference documentation is also available online.
The library is well-documented, with a comprehensive Microsoft Entra ID documentation that covers everything you need to know to get started.
A fresh viewpoint: Azure Token
Features Added
The Azure Default Credential has been enhanced with several new features to improve its functionality and usability. The get_token_info method has been added to all credentials, allowing for more complex authentication scenarios.
This method returns an AccessTokenInfo object, providing a more detailed view of the authentication process. The get_token_info method is an alternative to the get_token method and offers improved support for more complex scenarios.
The ManagedIdentityCredential has been updated to include identity config validation, preventing non-deterministic states such as specifying both resource_id and object_id. Additional validation has been added for ManagedIdentityCredential in Azure Cloud Shell environments.
A bearer token provider has been added, making it easier to authenticate with Azure services. The synchronous ManagedIdentityCredential now uses MSAL for handling most of the underlying managed identity implementations.
AzurePowerShellCredential now supports using secure strings when authenticating with PowerShell, making it more secure and convenient. The synchronous OnBehalfOfCredential now supports the send_certificate_chain keyword argument when using certs.
Here's a summary of the new features:
- get_token_info method added to all credentials
- Identity config validation added to ManagedIdentityCredential
- Additional validation for ManagedIdentityCredential in Azure Cloud Shell environments
- Bearer token provider added
- MSAL used for synchronous ManagedIdentityCredential
- AzurePowerShellCredential supports secure strings
- send_certificate_chain keyword argument supported for synchronous OnBehalfOfCredential
Sources
- https://pypi.org/project/azure-identity/
- https://www.npmjs.com/package/@azure/identity
- https://learn.microsoft.com/en-us/python/api/overview/azure/identity-readme
- https://learn.microsoft.com/en-us/python/api/azure-identity/azure.identity.defaultazurecredential
- https://learn.microsoft.com/en-us/dotnet/azure/sdk/authentication/credential-chains
Featured Images: pexels.com