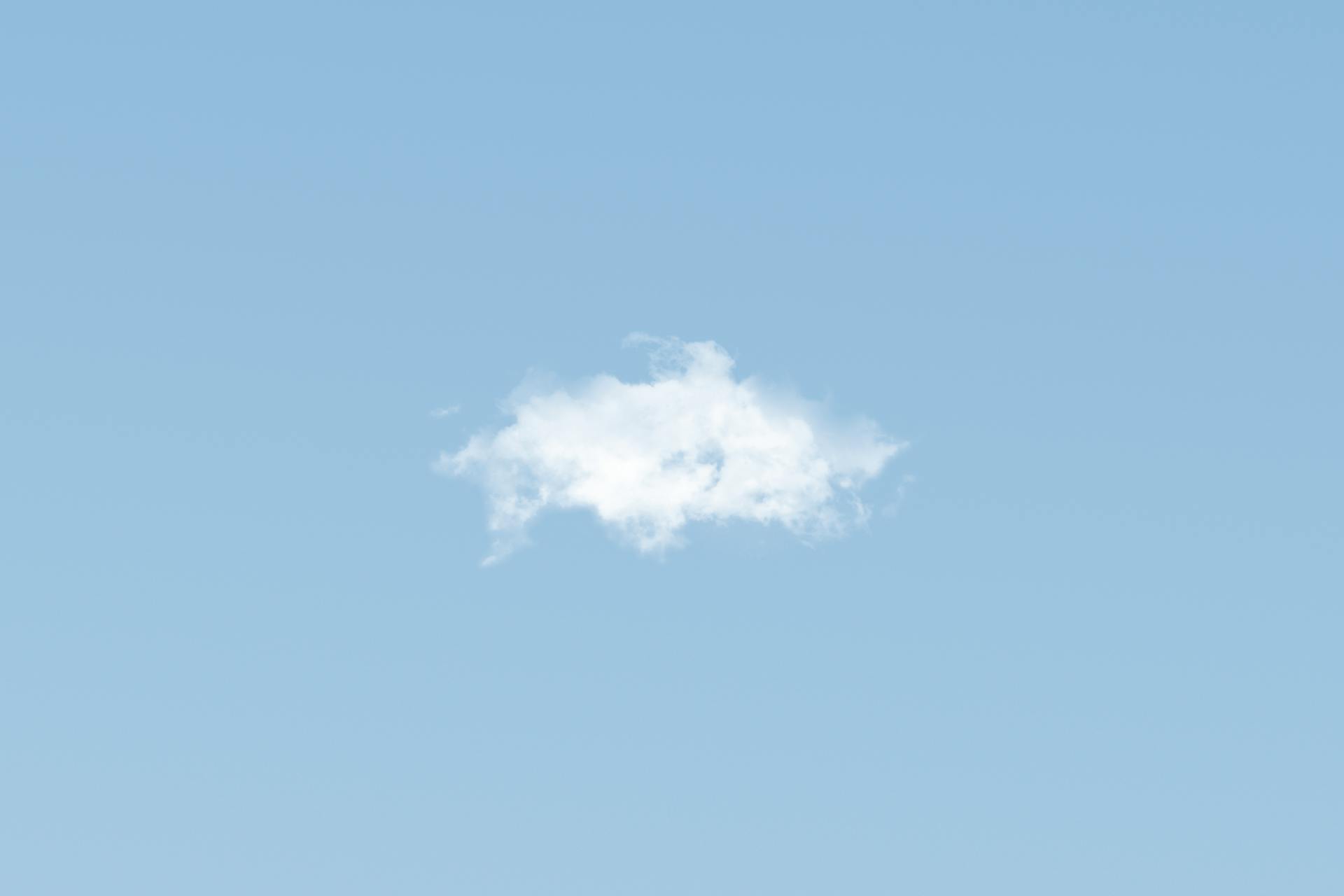
Using Microsoft.Identity.Web for Azure AD Authentication in ASP.NET Core is a game-changer for developers. This library simplifies the process of integrating Azure Active Directory (AAD) authentication into your ASP.NET Core application.
Microsoft.Identity.Web is a NuGet package that provides a set of APIs and tools for authenticating users with Azure AD. It's designed to work seamlessly with ASP.NET Core, making it easy to add authentication to your web app.
To get started, you'll need to install the Microsoft.Identity.Web package in your project using the NuGet Package Manager. This will give you access to the library's APIs and tools.
By using Microsoft.Identity.Web, you can easily configure Azure AD authentication in your ASP.NET Core application, including setting up authentication flows and handling authentication responses.
Readers also liked: Azure Functions Core Tools
Prerequisites
To get started with ASP.NET Core Azure AD authentication, you'll need to meet some prerequisites. Visual Studio 2022 17.0 or later, with the ASP.NET and web development workload, is a must-have.
You'll also need the .NET 6.0 SDK installed on your machine.
Alternatively, you can use Visual Studio Code, which requires C# for Visual Studio Code (latest version) and the .NET 6.0 SDK.
Here are the specific requirements for both Visual Studio and Visual Studio Code:
Azure AD Configuration
To create an App Registration in Azure AD for the Web API, search for "App Registrations" in the Azure Portal and create a new App Registration. Give it a name like "My Web API" and leave "Supported account type" set to the default of "Accounts in this organizational directory only".
You'll need to edit the manifest by selecting "Manifest" in the App Registration left-hand menu and find the place in the JSON that defines the appRoles. Paste in a new DaemonAppRole into this section.
The Application ID URI is generated when you click "Set" next to "Application ID URI" in the portal. This will also show you the "Application (client) ID" and "Directory (tenant) ID", which you'll need later.
A fresh viewpoint: Azure Ad Directory Roles
You can use the same GUID for the DaemonAppRole, it's not really important.
To configure the Web API, you need to reference the Microsoft.Identity.Web NuGet package and update appsettings.json by adding the following JSON. Replace the values with your own, such as the Tenant ID and Client ID found on the Overview tab for the App Registration.
The Domain can be found by navigating to the main "Azure Active Directory" page in the Azure portal and looking at the Primary domain.
Here are the required configuration settings for the Web API:
In the appsettings.json file, you'll also need to add the following settings:
```json
{
"AzureAd": {
"Instance": "https://login.microsoftonline.com/",
"Domain": "your_domain.com",
"TenantId": "your_tenant_id",
"ClientId": "your_client_id"
}
}
```
Here's an interesting read: Gitlab Auth Json Website Azure Ad Authentication
API Setup
To set up your ASP.NET Core API for Azure AD authentication, start by creating a new project using the "minimal APIs" template from .NET 7 with dotnet new web.
You'll need to reference the Microsoft.Identity.Web NuGet package with dotnet add package Microsoft.Identity.Web. Then, update your appsettings.json file by adding the necessary JSON, replacing the dummy values with your own Tenant ID, Client ID, and Domain.
You might like: Azure Auth Json Website Azure Ad Authentication
Be sure to remove any additional "Authentication" settings that may be interfering with the configuration. The Domain can be found in the Azure portal under the "Azure Active Directory" page.
You can require authorization on a per-endpoint basis like this: [Authorize], or for an entire group of endpoints like this: [Authorize(Roles = "Admin")]. Don't forget to add the URL for the web API, which in this example is localhost.
To configure JWT Bearer authentication as the default, add authentication services and middleware in the dependency injection and middleware configuration. This involves configuring JWT Bearer authentication and applying authentication with app.UseAuthentication();.
Note that you should require the caller to have the user_impersonation scope, which is the default delegated permission that exists in every Web app/API in Azure AD. This is important to prevent apps from calling your API unless this basic permission has been granted.
Here are some common Azure AD endpoints:
- https://login.microsoftonline.com/your-tenant-id (general Azure AD endpoints)
- https://login.microsoftonline.de/your-tenant-id (Germany)
- https://login.microsoftonline.cn/your-tenant-id (China)
- https://login.microsoftonline.us/your-tenant-id (U.S. Government)
Authentication Flow
To set up authentication in your ASP.NET Core app, you need to configure your user flow. This defines and controls the user experience when users sign in to your app.
See what others are reading: Get Azure Ad User
Azure AD B2C offers three types of user flows: a combined Sign in and sign up user flow, a Profile editing user flow, and a Password reset user flow. These user flows are named susi, edit_profile, and reset_password, respectively.
Azure AD B2C prepends B2C_1_ to the user flow name, so susi becomes B2C_1_susi.
Here are some common user flow names:
Fetching Access Tokens
To fetch access tokens, you'll need to grant permission to the Azure CLI to access your Web API app registration.
First, go to the App Registration for the Web API and add a new scope in the "Expose an API" tab. The name of the scope doesn't matter, just give it a name.
You'll need to add the Azure CLI client ID, which is 04b07795-8ddb-461a-bbee-02f9e1bf7b46, as an authorized client application. Select the scope you created as an authorized scope.
After setting up the permission, you can use the az account get-access-token command from the Azure CLI to fetch the token.
Additional reading: Azure Ad Connect Client
Sign Out
Let's take a closer look at the sign-out flow. The sign-out process involves several steps that ensure a secure and seamless experience for users.
First, users initiate the sign-out process from within the app. This is the first step in terminating the current session.
The app then clears its session objects, which effectively ends the user's interaction with the app. This is a crucial step to prevent any potential security risks.
Next, the authentication library clears its token cache, which is a critical component of the authentication process. This ensures that no sensitive information is left behind.
After clearing the token cache, the app redirects users to the Azure AD B2C sign-out endpoint. This is where the Azure AD B2C session is terminated, providing an additional layer of security.
Finally, users are redirected back to the app, marking the end of the sign-out process.
For your interest: Azure Ad B2c Userinfo_endpoint
Add Claims View
Adding the claims view is a crucial step in the authentication flow. This involves linking the Claims.cshtml view to the Home controller.
For more insights, see: Attributes and Claims Azure Ad
You'll need to add the Claims action to the /Controllers/HomeController.cs controller. This action uses the Authorize attribute, which limits access to authenticated users.
The Authorize attribute ensures that only authenticated users can access the claims view. This is a key aspect of the authentication flow, as it helps to protect sensitive information.
In the Claims action, you'll need to add the following code to link the Claims.cshtml view to the Home controller. This involves using the Authorize attribute to limit access to authenticated users.
Sample and Deployment
To implement authentication in an ASP.NET Core Azure AD application, you'll need to create an instance of the Azure AD authentication builder and configure it to use the Azure AD B2C instance.
The Azure AD authentication builder is created using the AddAzureADAuthentication method, which takes the instance of the Azure AD B2C application as a parameter.
To deploy your application, you can use Azure App Service, which supports automatic deployment from Visual Studio or Azure DevOps. This allows you to easily manage and deploy your application to Azure.
Configure the Sample
To configure the sample, start by opening the WebApp-OpenIDConnect-DotNet.csproj project in Visual Studio or Visual Studio Code.
You'll find the project in the 1-WebApp-OIDC/1-5-B2C/ folder within the sample folder.
Next, navigate to the appsettings.json file located under the project root folder.
This file contains information about your Azure AD B2C identity provider, so update the app settings properties accordingly.
Your final configuration file should resemble the following JSON:
To authenticate against Azure AD, you'll need an Azure AD application and an Azure subscription.
If you don't have an Azure subscription, you can get some free credits here.
Now, change the Redirect URIs from before to complete the configuration process.
If this caught your attention, see: Azure Ad Configuration
Deploy Your
Deploying your application is a crucial step in getting your app up and running. You'll want to add a publicly accessible endpoint where your app is running, such as https://contoso.com/signin-oidc.
To do this, you can add and modify redirect URIs in your registered applications at any time. There are some restrictions to keep in mind: the reply URL must begin with the scheme https, and its case must match the case of the URL path of your running application.
Here are the specific restrictions to keep in mind:
- The reply URL must begin with the scheme https.
- The reply URL is case-sensitive. Its case must match the case of the URL path of your running application.
Libraries and Setup
To get started with Azure AD authentication in ASP.NET Core, you'll need to add the Microsoft Identity Web library. This library simplifies the process of adding Azure AD authentication and authorization support to your web app.
You can install the packages by running the following commands: Add the Microsoft Identity Web library, which is a set of ASP.NET Core libraries that simplify adding Azure AD B2C authentication and authorization support to your web app.
The Microsoft Identity Web library sets up the authentication pipeline with cookie-based authentication, taking care of sending and receiving HTTP authentication messages, token validation, and claims extraction.
In the Startup.cs file, you'll need to initiate the authentication libraries. This involves adding the necessary code to initiate the middleware controller with its view.
You'll also need to add the cookie policy and use the authentication model. This involves replacing the ConfigureServices(IServiceCollection services) function with the following code snippet: services.AddMicrosoftWebAppAuthentication(Configuration, "AzureAd");
For more insights, see: Application Registration Azure
The "AzureAd" value specifies which section holds all the configuration information in the appsettings.json file. This is a crucial step in setting up the Azure AD authentication.
The ConfigureServices function also includes the line services.AddAuthentication, which is used to specify the authentication method. However, with the Microsoft.Identity.Web middleware, this line is no longer needed.
The AddRazorPages() series of lines includes a call to AddMicrosoftIdentityUI(), which pops in some controllers from Microsoft.Identity.Web.UI that handle calling out to Azure AD and also handle the callbacks.
To authenticate against Azure AD, you'll need to configure an Azure AD application. This involves changing the Redirect URIs in the Azure AD application settings.
For another approach, see: Azure Ad Application
Sources
- https://www.markheath.net/post/secure-aspnet-core-web-api-azure-ad
- https://learn.microsoft.com/en-us/azure/active-directory-b2c/configure-authentication-sample-web-app
- https://codemilltech.com/authenticate-a-asp-net-core-web-app-with-microsoft-identity-web/
- https://joonasw.net/view/azure-ad-authentication-aspnet-core-api-part-1
- https://learn.microsoft.com/en-us/azure/active-directory-b2c/enable-authentication-web-application
Featured Images: pexels.com