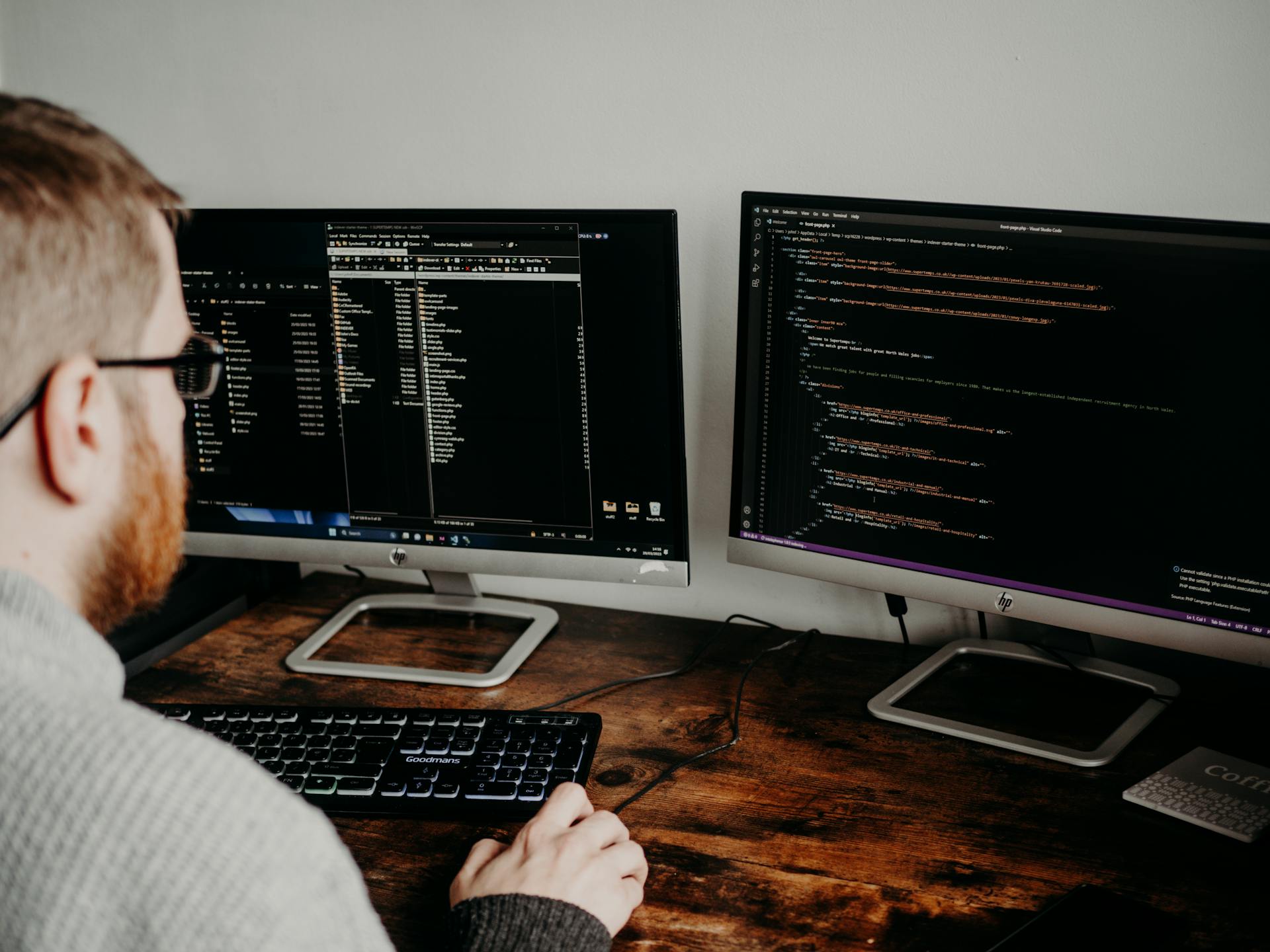
C++ is a powerful language that can be used for web programming, and one of the ways to make it happen is by using the Common Gateway Interface (CGI). CGI is a standard that allows web servers to run external programs on the server-side, enabling dynamic content generation.
CGI is a mature technology that has been around since the early days of the web. It's still widely used today, especially for simple web applications.
To get started with CGI in C++, you'll need to set up your development environment, which includes a C++ compiler, a web server, and a CGI library. The CGI library will handle the communication between your C++ program and the web server.
CGI programs typically use standard input and output streams to communicate with the web server. You'll also need to handle errors and exceptions properly to ensure your program runs smoothly.
A unique perspective: Web Design Referral Program
Choosing a Language
The first thing you need to decide is where the program will be run, as not all languages are capable of running on all kinds of platforms. For example, a program written in C++ requires compilers to run on Windows and Linux based systems.
Recommended read: Web Design Certificate Program
Language domain match is crucial, as you want to choose a language that is commonly used in the same domain or industry as your project. Searching for languages used by others in the same domain can be a good starting point.
Efficiency is key, as you want the compilers to be efficient to make the language perform fast. This can make a big difference in the overall performance of your program.
A language with good elasticity and performance is essential, as it should be flexible enough to let you add more programs or features in it. This will save you time and effort in the long run.
Availability of libraries is also important, as you want to make sure there's a library that can solve all your problems with the language you select. This will make development faster and easier.
Project size is another factor to consider, as you want to choose a language that can support your cause and suits the project size well. This will ensure that your program can handle the workload without any issues.
Here are some key factors to consider when choosing a language:
- Targeted platform
- Language domain match
- Efficiency
- Elasticity and Performance
- Availability of libraries
- Project size
- Expressiveness & Time to production
- Tool support
CGI Basics
The TEXTAREA element is used when multiline text has to be passed to a CGI program, and it's perfect for forms that require users to input a lot of text.
To handle input from a TEXTAREA box, you can use a CGI program like cpp_textarea.cgi, which can be generated using a C++ program.
CGI programs can be accessed using URLs, and the GET method is a simple way to pass values to a program like hello_get.py, which can be handled by a CGI program like cpp_get.cgi.
The GET method sends information as a text string after a ? in the URL, but the POST method is more reliable and sends information as a separate message, which comes into the CGI script as standard input.
CGI Environment Variables
CGI Environment Variables play a crucial role in writing any CGI program. All CGI programs have access to the following environment variables.
The CONTENT_TYPE variable determines the data type of the content, used when the client is sending attached content to the server. For example, file upload.
The CONTENT_LENGTH variable holds the length of the query information that is available only for POST requests. This is essential for handling POST requests.
HTTP_COOKIE returns the set cookies in the form of key & value pair. This is useful for storing user preferences or session data.
The HTTP_USER_AGENT variable contains information about the user agent originating the request, including the name of the web browser.
The PATH_INFO variable specifies the path for the CGI script. This is used to determine the location of the script on the server.
QUERY_STRING contains the URL-encoded information that is sent with GET method requests. This is useful for passing parameters to the CGI script.
REMOTE_ADDR holds the IP address of the remote host making the request. This can be used for logging or authentication purposes.
REMOTE_HOST returns the fully qualified name of the host making the request. If this information is not available, REMOTE_ADDR can be used to get the IP address.
REQUEST_METHOD specifies the method used to make the request, such as GET or POST. This is essential for determining how to handle the request.
Recommended read: Web Traffic Name
SCRIPT_FILENAME contains the full path to the CGI script. This is used to locate the script on the server.
SCRIPT_NAME holds the name of the CGI script. This is used to identify the script.
SERVER_NAME returns the server's hostname or IP Address. This is useful for identifying the server.
SERVER_SOFTWARE contains the name and version of the software the server is running. This can be used for debugging or troubleshooting purposes.
Here is a list of the CGI environment variables:
CGI Library
You can use a CGI library to simplify your CGI programming tasks.
One such library is the C++ CGI Library, which can be downloaded from ftp://ftp.gnu.org/gnu/cgicc/.
This library can save you a lot of time and effort by providing pre-written code for common CGI operations.
To get started with the C++ CGI Library, you'll need to follow the installation steps provided with the download.
Suggestion: C Programming Web Server
Get Method Example
You can pass values to a CGI program using the GET method. This involves creating a URL that includes the values as query string parameters.
To get started, you can use a simple URL example, such as the one mentioned in the article. This example passes two values to a program called hello_get.py using the GET method.
You can also use a CGI library, such as the one available at ftp://ftp.gnu.org/gnu/cgicc/, to make it easier to access the passed information. This library is written for C++ programs and can be downloaded and installed following the provided steps.
The same CGI script, cpp_get.cgi, can be used to handle input given by a web browser using both the GET and POST methods. This means you can use the same script to handle different types of input.
You can try accessing the cpp_get.cgi script using a link, such as the one mentioned in the article, to see the result of passing values using the GET method.
Get Method
You can pass values to a C++ CGI program using the GET method, as shown in a simple URL example where two values are passed to the hello_get.py program.
To handle input from a web browser, you can use the C++ CGI library, which makes it easy to access passed information.
A simple URL like this one will pass values to the CGI program: hello_get.py?first=value&last=value.
You can compile the CGI program, put it in your CGI directory, and access it using a link like this: http://localhost/cgi-bin/cpp_get.cgi?first=value&last=value.
The result of accessing this link will be the output of the CGI program, which can be seen in the example output.
A simple form example using the GET method can also be used to pass values to the CGI program, as shown in an example HTML code for a form with one drop down box.
The CGI script cpp_get.cgi can handle input from this form, and you can enter values in the form fields and click the submit button to see the result.
Passing Information via POST
Passing Information via POST is a reliable method of passing information to a CGI program, packaging the information in the same way as GET methods, but sending it as a separate message.
This separate message comes into the CGI script in the form of the standard input, making it a more reliable option. The same C++ program that handles GET methods, cpp_get.cgi, will also handle POST methods.
To pass information using the POST method, you can use an HTML FORM with a submit button, just like with GET methods. However, the data will be sent as a separate message instead of being appended to the URL.
The POST method is particularly useful when you need to pass large amounts of data, such as multiline text. In these cases, you can use the TEXTAREA element in your HTML form to allow the user to input the text.
Take a look at this: Web Programming Html
Web Development
C++ can significantly increase the speed of web applications and reduce the load on servers.
Using C++ in web development provides fine control over every aspect of these applications, making it a great choice for highly loaded software.
This means that if you're working on a project that requires a lot of processing power, C++ is a good option to consider.
Get Method
The Get Method is a way to pass values to a web application, and it's surprisingly easy to use. You can pass values using a simple URL, like this: `http://example.com/hello_get.py?first_name=John&last_name=Doe`.
To handle this input, you'll need to write a CGI script, such as `cpp_get.cgi`, which can be compiled and placed in your CGI directory. This script will make it easy to access the passed information.
You can also use an HTML form to pass values, by submitting a form with input fields. The `cpp_get.cgi` script can handle this input as well.
The `cpp_get.cgi` script can be used to handle both simple URL examples and HTML form submissions, making it a versatile tool for web development.
When to Use in Web Development?
C++ significantly increases the speed of web applications and reduces the load on servers.
Using C++ in web development can be beneficial for highly loaded software, as it provides fine control over every aspect of these applications.
Projects resulting from web development using C++ are highly scalable and capable of processing large amounts of data efficiently.
C++ is a great choice for web development when speed and scalability are top priorities.
Broaden your view: Web Based Mobile Application Development
When Web Development Is a Bad Idea
Web development can be a bad idea if you're looking to create small web pages and web applications, as C++-based programs take up more memory compared to their counterparts using languages like PHP or Python.
Finding a qualified C++ developer is not easy, especially if you don't have access to a large pool of IT experts.
Using C++ for web development can be a bad idea because it's not the best fit for small projects.
Keep in mind that memory usage is a significant concern when working with C++-based programs, making them less suitable for small web applications.
Intriguing read: Web App Programming Language
Real-World Applications
C++ web programming has numerous real-world applications that make it a valuable skill to have.
The web framework Poco has been used in various projects, including the popular online shopping platform, Zalando.
Developers can use C++ to build high-performance web servers that handle large amounts of traffic, such as the open-source web server, Lighttpd.
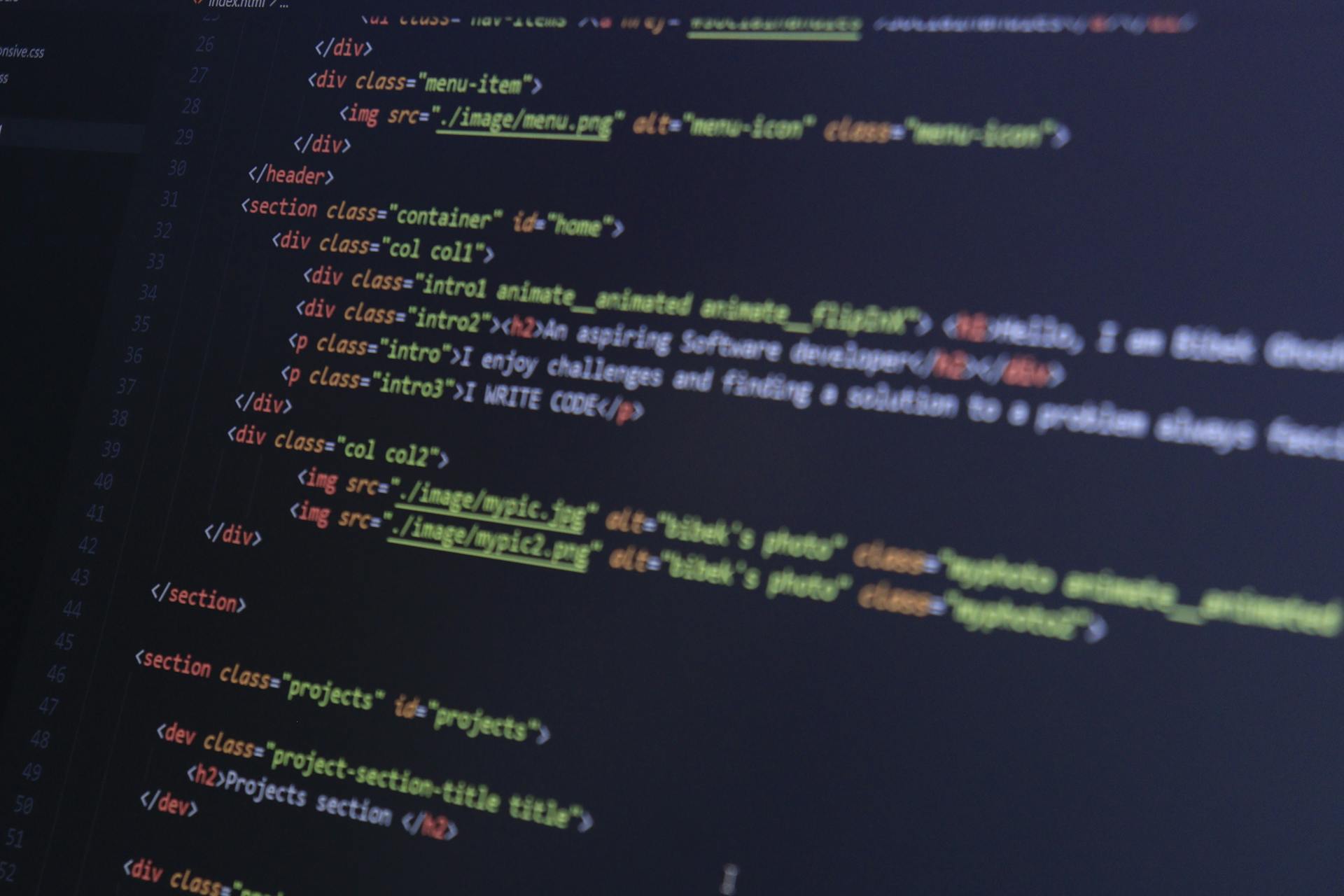
The Qt framework provides a comprehensive set of libraries and tools for building cross-platform GUI applications, making it a popular choice for desktop applications.
C++ can be used to build scalable and efficient web applications that handle complex data processing, such as the popular web application, Dropbox.
The use of C++ in web programming allows developers to take advantage of its high-performance capabilities, making it an ideal choice for applications that require fast data processing and low latency.
A fresh viewpoint: Web Developing Languages
Project Considerations
When working on a C++ web programming project, it's essential to consider the limitations of the language in handling web-specific tasks, such as file uploads and database interactions.
C++ is not designed for web development, so you'll need to use external libraries like Boost or Poco to handle web-related tasks.
Choose a framework like Wt or QScintilla that provides a higher-level interface for web development, making it easier to build web applications with C++.
Keep in mind that C++ web programming requires a good understanding of web development concepts, such as HTTP requests and responses, to ensure seamless integration with the web framework.
If this caught your attention, see: Software Development Web
Product Owner
Project owners often have to deal with time delays in the development process due to difficulties in reading C++ code from CGI programs.
Many software developers struggle to read their own and others' code, making it a common challenge in web development.
This struggle can lead to delays and hinder the project's progress.
Project managers and product owners need to be aware of this potential issue and plan accordingly.
Curious to learn more? Check out: Best Web Development Programming Language
Narrow-Focused Projects
Narrow-focused projects can be incredibly effective. The Opera browser is a great example of a successful narrow-focused project. It's fast and compatible with major web technologies.
One notable feature of the Opera browser is its multi-web page interface. This interface allows users to have multiple web pages open at the same time.
On a similar theme: Web Application Programming Interface
Sources
- https://www.codecademy.com/catalog/subject/web-development
- https://cleverism.com/programming-languages-web-development/
- https://www.tutorialspoint.com/cplusplus/cpp_web_programming.htm
- https://www.linuxlinks.com/free-open-source-c-web-frameworks/
- https://startups.epam.com/blog/c-plus-plus-for-web-development
Featured Images: pexels.com