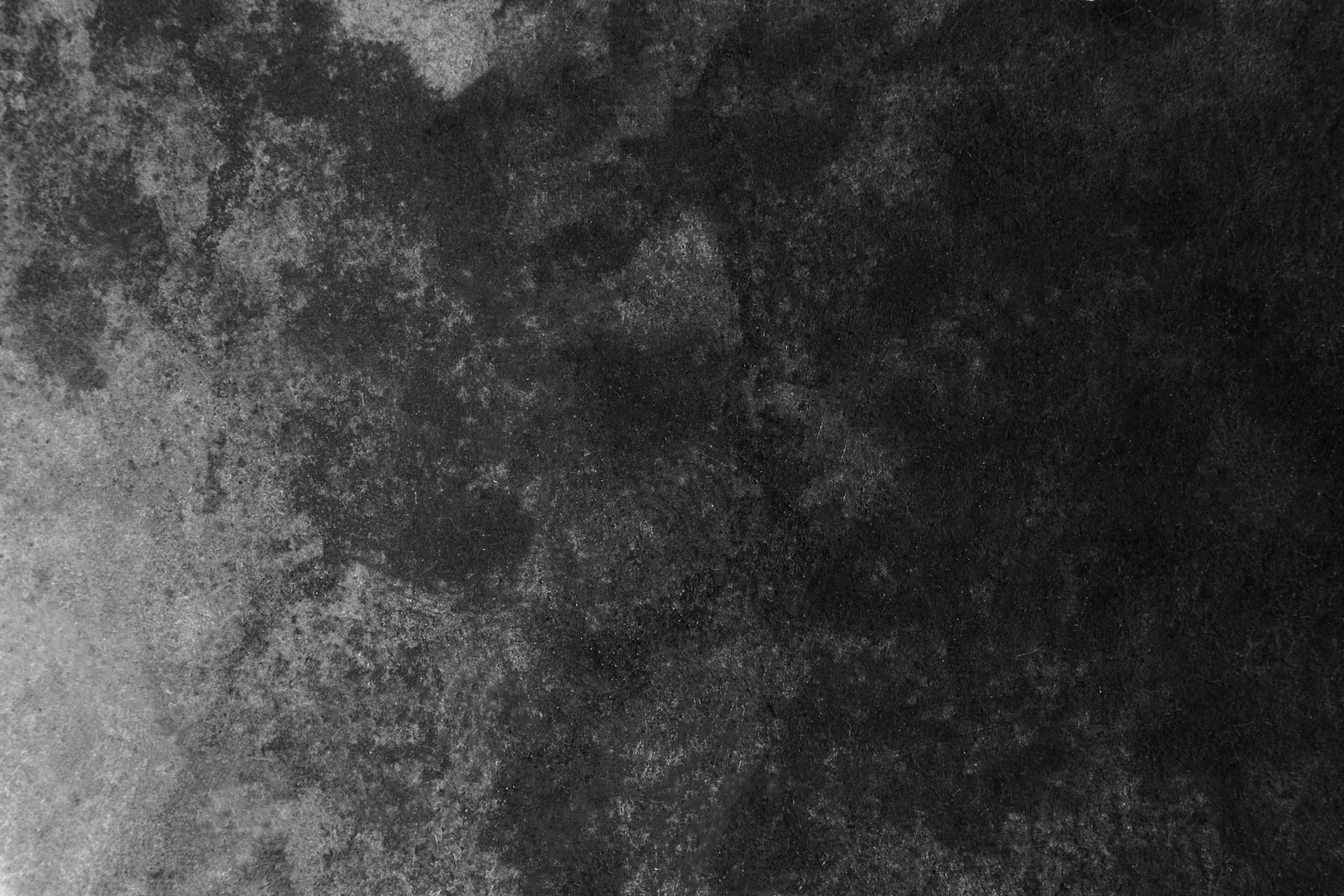
CSS class selectors are a fundamental part of CSS, allowing you to target specific HTML elements based on a class attribute. A class selector is defined by a dot followed by the class name, for example, `.my-class`.
Using class selectors can greatly simplify your CSS code by reducing the need for repetitive styles. By applying a class to multiple elements, you can apply the same styles to each one.
A CSS class selector can be applied to any HTML element, including divs, spans, and even form elements. For example, you can use `.error-message` to target a specific error message on a form.
Class selectors can also be combined with other selectors to create more complex rules. This allows you to target specific elements based on a combination of criteria.
A different take: Free Css Templates Responsive
Understanding CSS Class Selectors
CSS class selectors are a great way to apply unique styles to HTML elements without repeating code. They're defined with a period followed by the class name, like .intro {color: blue;}.
Classes allow CSS to select HTML elements with the class selector and apply styles to these elements.
By using a class selector, you can apply the same style to multiple HTML elements, ensuring consistency in design and saving time.
The class makes it possible to style a single HTML element differently from other elements with the same class name by adding another class name after a previously defined class.
Class selectors are a good option for practicing the Don’t Repeat Yourself (DRY) principle because they can be applied to multiple tags, making them reusable.
For another approach, see: Css Selector the Last 2 Child Elements
CSS Class Selector Basics
To use a CSS class selector in HTML, you need to assign the class name to the HTML element using the ‘class’ attribute. For instance, if you have a class selector named ‘intro’ in your CSS file, you can apply it to a paragraph in HTML like this.
A class selector in CSS can be used directly with a class name as in the following example: .child selector has selected both the div as well as the p elements and has applied the same styles to them.
To create a CSS class selector, you need to define the class in CSS and then apply styles with the class name. For example, you can create a class named ‘first-header’ and apply styles to it like this: .first-header { text-align: center; } .first-header h3 { font-size: 32px; color: #333; } .first-header p { font-size: 18px; color: #1d1e1e; }
Here are the basic steps to use a CSS class selector:
- Create an HTML document and add the element to be styled.
- Define the class in CSS and apply styles with the class name.
- Use the !important rule to override a CSS class style, but note that it can be overwritten only by another !important rule with the same or higher specificity.
How to?
To use a CSS class selector in HTML, you need to assign the class name to the HTML element using the 'class' attribute. For instance, if you have a class selector named 'intro' in your CSS file, you can apply it to a paragraph in HTML like this.
To target a specific class attribute in HTML, use a CSS class selector together with a class name. You can do this by assigning the class name to the HTML element using the 'class' attribute.
You might like: Css Attr Selector
In CSS, counting the number of inline styles, ID selectors, class selectors, and element selectors an element contains determines its specificity. This means that in conflicting class styles, CSS class declarations with higher specificity take precedence over the class selectors with lower specificity.
To determine the specificity of a CSS class declaration, count the number of inline styles, ID selectors, class selectors, and element selectors an element contains. The more selectors an element has, the higher its specificity will be.
A class selector in CSS can be used directly with a class name to target elements with that class. For example, if you have a class selector named 'child' in your CSS file, you can apply it to an element in HTML like this.
To use a CSS class selector in CSS, follow these steps:
- Create an HTML document and add the element to be styled.
- Define the class in CSS.
- Apply styles with the class name.
By following these steps, you can effectively use a CSS class selector to target and style elements in your HTML document.
Important Declaration
The !important Declaration is a powerful tool in CSS that can override class styles. It's a game-changer for situations where you need to prioritize one style over others.
The syntax for using the !important rule is straightforward: it can overwrite all other styling rules of an element.
Be careful when using !important, as it can be overwritten only by another !important rule on a declaration with the same or higher specificity. This means you need to be strategic about when to use it to avoid unintended consequences.
A fresh viewpoint: Css Selector That Targets a Specific Property Declaration
Cascading
Cascading is a fundamental concept in CSS that allows users to have more control over presentation. It's like a layered cake, where each layer can be adjusted without affecting the others.
The style sheet with the highest priority controls the content display. This means that if a user applies a different style sheet, it will override any previous styles. For example, if a user finds red italic headings difficult to read, they can apply a new style sheet to change the color or font style.
The process of cascading allows users to choose from various style sheets provided by designers, or remove all added styles and view the site using the browser's default styling. Browser extensions like Stylish and Stylus have been created to facilitate the management of user style sheets.
Here's a breakdown of the CSS priority scheme:
By understanding the cascading process, developers can use it to determine which style has a higher priority when integrating third-party styles, and to further resolve conflicts.
Distinguishing and ID
In CSS, it's essential to understand the difference between class selectors and ID selectors. IDs are unique, meaning only one ID per element can be on a page.
One key difference between ID and class selectors is how they're used in JavaScript. IDs are typically used to target and assign functionality to a specific element on a page, while classes are used to select and assign functionality to a group of elements.
Check this out: Id Selector in Css
In CSS, ID selectors are preceded by a hash symbol (#), while class selectors are declared with a preceding period symbol (.) - a simple but crucial distinction to make.
To quickly locate an element on a page, IDs can be used with the hash value as a browser jump link. This is a convenient feature that's not available with class selectors.
Best Practices
Proper application of CSS class selectors is crucial for a functional, scalable, and well-organized codebase. This means using semantic class naming to improve clarity, reusability, and flexibility within a codebase.
Use semantic class naming to convey the element's purpose. This will make your code easier to understand and maintain.
Use descriptive names that reflect the element's role within the document. For example, submit-btn or hero-content provide more context and clarity than btn or content.
Adopting a consistent naming convention is essential. Popular conventions include Block Element Modifier (BEM), Scalable and Modular Architecture for CSS (SMACSS), and Object-Oriented CSS (OOCSS).
Here are some key best practices to consider:
- Use semantic class naming.
- Use descriptive names.
- Use short and simple names.
- Consistent naming convention.
- BEM convention.
Remember, class names are case-sensitive and should not start with a number. You can use hyphens (-) or underscores (_) to separate words in a class name.
Overriding Styles
Overriding styles in CSS class selectors is a common practice, and it's useful for changing predefined styles of an element.
You can override styles by defining a new style later in the CSS file, which will override the earlier one. This is because the browser follows the CSS specificity and inheritance rules to resolve conflicts.
More specific selectors will have higher priority, so using them can also help you override styles.
The 'important' rule can also be used to give a style higher priority, but use it sparingly as it can make your CSS hard to manage.
Conflicting styles in CSS class selectors can be resolved by the browser following the CSS specificity and inheritance rules, giving priority to the style defined later in the CSS file.
For another approach, see: Css in Html File
CSS Class Selector Advanced Topics
Using multiple class selectors for a single HTML element is a great way to add specificity to your CSS styles. You can separate each class name with a space within the 'class' attribute in HTML.
For example, if you have an HTML element with multiple classes, you can use each class name to target specific styles. This helps keep your CSS organized and makes it easier to maintain.
To take it a step further, you can combine class and type selectors to target specific HTML elements. This allows you to be more precise in your styling and avoid unnecessary class name pollution.
Pseudo-Elements
Pseudo-Elements are a powerful tool in CSS, allowing you to style specific parts of an element.
You can use pseudo-elements to style the first line and first letter of an element, which is super useful for adding a touch of elegance to your typography.
To style the first line, you can use the ::first-line pseudo-element, while the ::first-letter pseudo-element is used for styling the first letter.
Pseudo-elements can also be used to add content before or after an element, which can be useful for adding icons or other visual elements to your design.
Here are some examples of pseudo-elements:
Descendant
Descendant selectors are a powerful tool in CSS that allow you to target all descendants of a specified element. They're denoted by a space between two selectors.
The descendant selector is used to match all elements that are descendants of a specified element, such as a parent element. This means you can apply styles to all the HTML elements within a container or parent element.
For example, if you have a HTML structure like the one shown in Example 2, you can use the descendant selector to apply styles to all elements with class "child" that are descendants of an element with class "parent". This is achieved by using the space between the two selectors, like this: .parent .child.
By using the descendant selector, you can avoid creating excessive and unnecessary classes and HTML elements, as mentioned in Example 3. This approach helps maintain clean and semantic markup and styles.
Consider reading: Css Selector Select Child of Parent
You can use the descendant selector to add styles to all the immediate descendants of a specified element, such as adding a blue border to all descendants associated with the element featuring the “container” class, as shown in Example 1. This is done by using the descendant selector with a CSS class selector.
The descendant selector is a type of combinator that is used to match all elements that are descendants of a specified element, as explained in Example 2.
Pseudo-Class
Pseudo-class selectors are context-dependent and select an HTML element based on its state and context. They are prefixed with a colon, “:”, and should appear after a CSS selector.
These selectors come in four types: dynamic pseudo-classes, structural pseudo-classes, language pseudo-class, and functional pseudo-classes. You can use them to define specific states of HTML elements, such as visited links or active links.
To create a pseudo-class selector, you can use the :hover pseudo-class to change the background color of an element when the mouse is over it. This is useful for creating interactive and engaging user interfaces.
Consider reading: Online Yoga Classes for Back Pain
Pseudo-class selectors can be combined with class selectors to target specific elements on a page. For example, you can use the :first-child pseudo-class to target the first paragraph on a page.
Here are some common pseudo-class selectors:
- :dynamic pseudo-classes
- :structural pseudo-classes
- :language pseudo-class
- :functional pseudo-classes
By using pseudo-class selectors, you can add extra functionality to your HTML elements and create a more engaging user experience.
Bem Convention
The BEM convention is a popular and preferred option for naming classes, providing a structured and modular way of naming classes that reflects the HTML structure. It consists of a Block, Elements, and Modifiers.
A Block is a standalone element, an Element is a bit that makes up a block, and a Modifier changes the appearance of the block or elements. BEM includes the following patterns: .block, .block__element, .block–modifier.
Using the BEM convention helps keep your code organized and easy to maintain, especially in large projects. It's essential to adopt and adhere to a consistent naming convention across all projects.
Using Specificity
CSS specificity is a crucial concept to grasp when working with CSS class selectors. It determines which styles take precedence when there are conflicts between multiple class declarations.
In CSS, the number of inline styles, ID selectors, class selectors, and element selectors an element contains determines its specificity. This means that an element with multiple class selectors will have higher specificity than one with fewer class selectors.
There are four levels of specificity, ranked from highest to lowest: inline styles, IDs, classes, and elements.
Here's a quick rundown of the levels of specificity:
- Inline styles: the highest level of specificity, as they are used directly on an HTML element
- IDs: using an ID attribute as a selector gives it the second-highest level of specificity
- Classes, Pseudo-classes, attribute selectors: class selectors have a lower level of specificity than IDs, but can still override other class selectors
- Elements and pseudo-elements: element selectors have the lowest level of specificity, but can still be useful for selecting specific elements
If there are multiple class selectors with the same specificity, the one that appears last in the stylesheet will be applied. This means that it's essential to consider the order of your class selectors when writing CSS.
Combining Multiple
You can use multiple class selectors for a single HTML element by separating each class name with a space within the 'class' attribute in HTML.
Combining multiple classes is a powerful aspect of class selectors, allowing you to apply different styles to different elements. For example, you can use two rules on one particular element.
Using multiple class names helps add specificity to CSS styles, making it easier to target specific elements. This is especially useful when you have multiple elements with the same class name but different styles.
You can combine multiple classes to create a unique style for a specific element. This is done by listing each class name separated by a space in the 'class' attribute.
For instance, if you have an HTML element with the classes 'child' and 'parent', you can style it differently using the classes individually or together. This gives you more control over the layout and design of your web page.
Using multiple class selectors can also help you avoid cluttering your HTML code with unnecessary classes. By combining multiple classes, you can achieve the same result with fewer classes.
See what others are reading: Css Text Size
JavaScript capabilities
JavaScript capabilities are quite impressive, and one of the most useful features is the ability to use CSS class selectors to manipulate HTML elements.
You can use the 'document.getElementsByClassName()' method to select elements with a specific class name. This method is a powerful tool for targeting specific elements on a webpage.
CSS class selectors can be used to change the styles, content, or attributes of selected elements using various JavaScript methods. This flexibility makes JavaScript an essential skill for any web developer.
With 'document.getElementsByClassName()', you can easily target elements with a specific class name, making it a valuable tool for dynamic web development.
Positioning
The position property is a crucial aspect of CSS that determines how an element is placed on the page. There are five possible values for the position property.
The default value of the position property places the item in the normal flow. This means subsequent flow items are laid out as if the item had not been moved.
Recommended read: Add Css Property to a Predefined Class Javascript
If an item is positioned in any way other than static, the further properties top, bottom, left, and right are used to specify offsets and positions. This is because static positioning doesn't allow for any offsets.
Absolute positioning places the item in relation to its nearest non-static ancestor. This means the item is positioned relative to its parent element, not the entire document.
Fixed positioning places the item in a fixed position on the screen, even as the rest of the document is scrolled. This is useful for creating navigation bars or other elements that need to stay visible at all times.
Frequently Asked Questions
What are the 5 CSS selectors?
There are 7 main types of CSS selectors, not 5, including Element Type, Descendant, Class, Id, Child, Adjacent sibling, and Pseudo selectors. To learn more about each type and how to use them effectively in your CSS coding, check out our in-depth guide.
How do you call a class selector in CSS?
To call a class selector in CSS, simply prefix the class name with a period (.) character. This is the basic syntax for selecting elements with a specific class attribute value.
How do I select multiple classes in CSS?
To select multiple classes in CSS, simply separate the class names with commas in your CSS selector. This allows you to apply styles to multiple classes at once.
What is a selector class in CSS?
A class selector in CSS is a way to target specific HTML elements with a unique class attribute, allowing for customized styling without affecting other elements. It's formatted with a period (.) followed by the class name, making it a powerful tool for CSS styling.
Featured Images: pexels.com