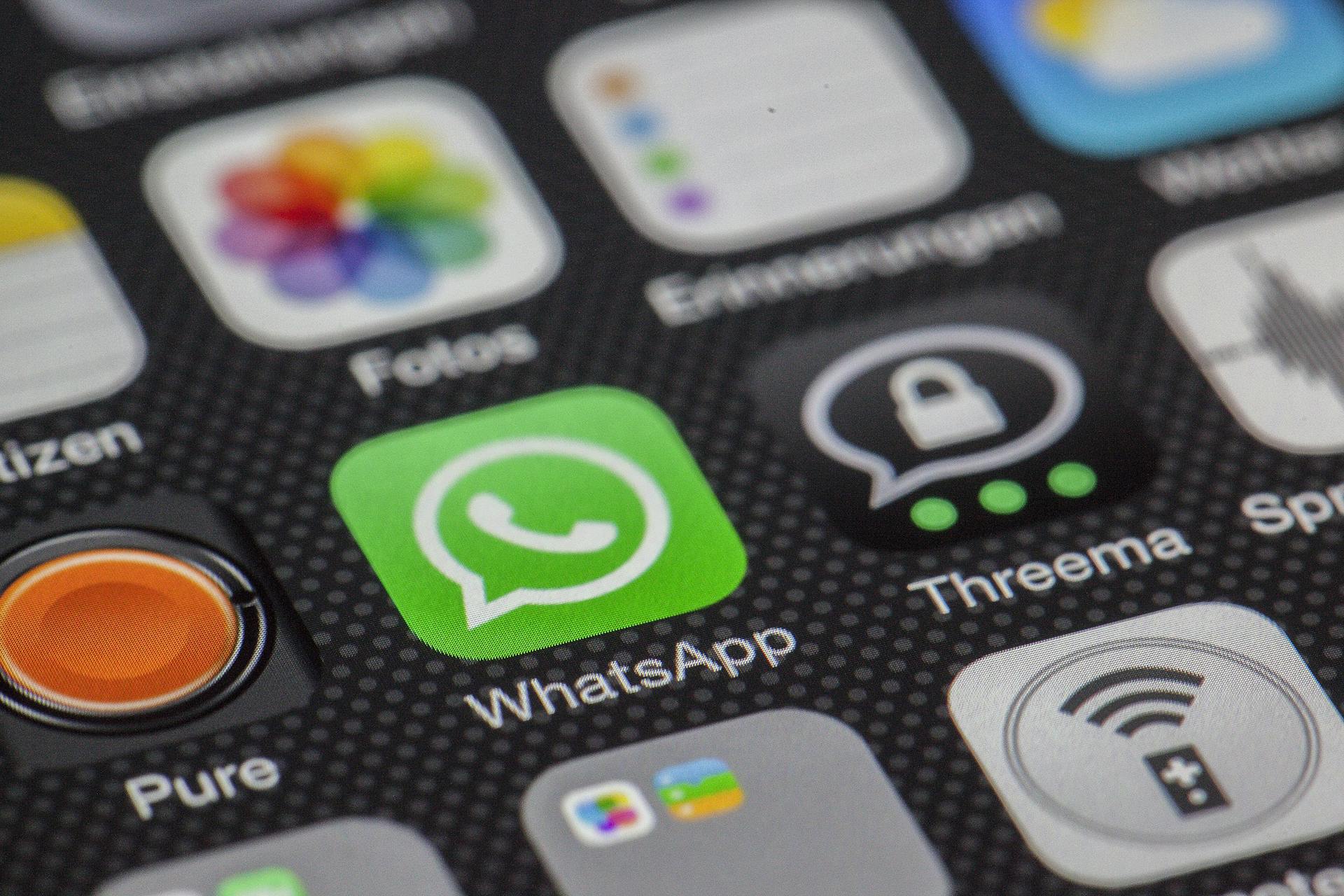
As a web developer, you're likely no stranger to CSS selectors, but have you ever stopped to think about how they work? CSS data selectors are a powerful tool for targeting specific elements on the page, and in this guide, we'll walk you through the basics.
CSS data selectors use the attribute selector syntax to target elements based on their attributes, such as class or id. For example, a selector like `[class~="example"]` targets any element with a class attribute that contains the word "example".
To use CSS data selectors effectively, it's essential to understand the different types of selectors available. There are attribute selectors, which target elements based on their attributes, and pseudo-class selectors, which target elements based on their state or position in the document.
A simple example of an attribute selector is `[hreflang="en"]`, which targets any link element with a hreflang attribute set to "en". This type of selector can be incredibly useful for targeting specific languages or regions on your website.
Here's an interesting read: Css Selector That Targets a Specific Style Declaration
Basic Selectors
Basic Selectors are the foundation of CSS data selectors. They allow you to target and select specific parts of your document for styling purposes.
You can use the universal selector, *, to select all elements on a page. This is a simple but powerful way to apply styles to an entire document.
Class selectors are another type of simple selector. They target elements based on the value of their class attribute. For example, the selector `.btn` targets all elements with a class of "btn".
Here are some examples of simple selectors:
- Universal selector: `*`
- Element selector: `h1`, `li`, etc.
- Class selector: `.btn`, `.header`, etc.
- ID selector: `#logo`, `#header`, etc.
These simple selectors are the building blocks of more complex selectors, and understanding how they work will help you write more effective CSS code.
Simple
Simple selectors are the building blocks of CSS, and understanding how they work is essential for writing effective CSS code. They allow you to directly select one or more elements on a web page.
By using the universal selector, *, you can select all elements on a web page. You can also select elements based on their name or type, such as selecting all paragraph elements with p.
Consider reading: Css Selector Select Child of Parent
To select elements based on their class value, you start the selector with a period, followed by the class attribute value. For example, .highlight would select all elements with a class of "highlight".
Here's a summary of the different types of simple selectors:
- Universal selector: * selects all elements
- Element selector: selects elements based on their name or type, such as p selects all paragraph elements
- Class selector: selects elements based on their class value, such as .highlight selects all elements with a class of "highlight"
- ID selector: selects elements based on their ID value, such as #id selects the element with the ID "id"
Simple selectors will be your go-to choice for most CSS styling tasks, and mastering them will make your CSS code more efficient and effective.
Specificity
Specificity is a key concept in CSS that helps resolve conflicts between selectors.
The more specific a selector is, the higher its weight in the cascade.
A selector that uses an ID has a higher specificity than one that uses a class, which in turn has a higher specificity than one that uses an element type.
For example, consider a selector that targets a specific element on a page, like #myId, which has a higher specificity than a selector that targets a class, like .myClass.
Intriguing read: How to Find Css Selector
Descendant and Child Selectors
Descendant selectors match an element that is a descendant of another element. This uses two separate selectors, separated by a space.
To style only the paragraphs that are inside the div, you would use the descendant selector. For example, if you wanted to style only the paragraphs inside the div, you would use the CSS rule `div p`.
The descendant combinator selects only the descendants of the specified element. You first mention the parent element, leave a space, and then mention the descendant of the first element.
There are four types of combinators, including the descendant combinator, the direct child combinator, the general sibling combinator, and the adjacent sibling combinator.
The direct child combinator, also known as the direct descendant, selects only the direct children of the parent. To use the direct child combinator, specify the parent element, then add the > character followed by the direct children of the parent element you want to select.
Curious to learn more? Check out: Css Selector That Styles Child If Parent Has an Attribute
Here are some examples of descendant and child selectors:
The descendant selector is useful for styling elements that are nested inside other elements. For example, if you wanted to style all emphasized text in your paragraphs to be green text, you would use the CSS rule `em { color: green; }`.
Intriguing read: Css Selector the Last 2 Child Elements
Direct Combinator
The Direct Child Combinator is a powerful tool in CSS that allows you to target specific elements within a parent element. It's a type of selector that specifies the parent element, followed by the > character, and then the direct children of the parent.
To use the direct child combinator, you specify the parent element, then add the > character followed by the direct children of the parent element you want to select. This is demonstrated in Example 3, where the code `div > a` matches the a elements directly nested inside the div element.
The direct child combinator is more specific than the descendant combinator, as it only selects direct children, not all descendants. This is important to keep in mind when writing your CSS selectors.
For another approach, see: Div Styling Css
Here are the four types of combinators, including the direct child combinator:
- Descendant combinator
- Direct child combinator
- General sibling combinator
- Adjacent sibling combinator
The direct child combinator is useful when you need to target specific elements within a parent element, such as in Example 3, where the a elements inside the div element are targeted.
Pseudo Classes
Pseudo classes are a powerful tool in CSS that allow you to style elements based on their state or position. They start with a colon followed by a keyword that reflects the state of the specified element.
The :link selector applies styling when the element has not been visited before. The :visited selector applies when the element has been visited before in the current browser. The :hover selector applies when the mouse pointer hovers over an element. The :active selector applies when the element is selected after being clicked on and after holding down a mouse button.
Some pseudo classes target specific types of elements, such as form inputs. The :focus selector applies when a user has tabbed onto an element. The :required selector selects inputs that are required. The :checked selector selects checkboxes or radio buttons that have been checked. The :disabled selector selects inputs that are disabled.
Here are some common pseudo classes used with links: :link:visited:hover:active
Related reading: Css Disabled Selector
The Pseudo
The pseudo-class :first-letter is used to select the first letter of a paragraph, which is helpful when you want to style the first letter in a certain way.
You can use the ::first-letter pseudo-element to select the first letter of a paragraph, which is helpful when you want to style the first letter in a certain way.
The :nth-child() selector selects a child element inside a container based on its position in a group of siblings.
For example, you can use the :nth-child() selector to select even or odd elements.
The :first-of-type selector selects elements that are the first of that specific type in the parent container.
For example, you can use the :first-of-type selector to select the first p inside a div.
The :last-of-type selector selects elements that are the last of that specific type in the parent container.
For example, you can use the :last-of-type selector to select the last p inside a div.
Curious to learn more? Check out: Css Last of Class
The :link selector applies styling when the element has not been visited before.
The :visited selector applies when the element has been visited before in the current browser.
The :hover selector applies when the mouse pointer hovers over an element.
The :active selector applies when the element is selected after being clicked on and after holding down a mouse button.
Here are the 4 pseudo-classes useful to select elements such as links in various states:
- :link - Targets unvisited links.
- :visited - Targets links that have already been visited by the user.
- :hover - Targets elements when they are being hovered over by the user's pointer.
- :active - Targets elements during the moment they are being activated.
Pseudo for Inputs
Pseudo classes for inputs are really useful for styling specific states of form elements. You can use the :focus selector to apply styling when a user tabs onto an input.
The :checked selector is another useful pseudo class for inputs, selecting checkboxes or radio buttons that have been checked. This is helpful when you want to style the input and its associated label differently when the checkbox is checked.
The :disabled selector selects inputs that are disabled, which is useful for styling elements that are not interactive. Many browsers style disabled inputs with a faded-out gray color by default.
Take a look at this: Css Image Styling
Here are some pseudo classes for inputs and their descriptions:
The :required pseudo class targets input elements that have the required attribute, indicating that they must be filled out before the form can be submitted.
22nd of
The nth-child pseudo class allows you to target specific elements in a stack. You can use it to select a specific child by its position in the list.
The nth-child pseudo class accepts an integer as a parameter, but it's not zero-based. This means that if you want to target the second list item, you need to use li:nth-child(2).
You can even use nth-child to select a variable set of children. For example, you could use li:nth-child(4n) to select every fourth list item.
Here are some examples of how you can use nth-child:
Remember, nth-child is a powerful tool for selecting specific elements in a list. With practice, you'll get the hang of using it to target exactly what you need.
Before and After
Pseudo classes are used to select elements based on their state or position, but did you know that they can also be used to change the appearance of an element based on its state or position?
The :hover pseudo class is used to select an element when the user is hovering over it, and it can be used to change the background color of an element to a different color when hovered over.
You can use the :focus pseudo class to change the background color of a form field when it has focus, making it easier for users to see which field they are currently filling out.
The :visited pseudo class is used to select links that have been visited, and it can be used to change the color of visited links to a different color.
By using pseudo classes, you can create a more interactive and engaging user experience, and it's a great way to add some visual flair to your website or application.
You might enjoy: Css Pseudo Selector All Spans after Selector
Attribute Selectors
Attribute selectors are a powerful tool in CSS that allow you to target elements based on the value of one of their attributes. You can use attribute selectors to target elements based on the existence of an attribute, or on the value of an attribute that matches a specific pattern.
For example, you can use the [attr] attribute selector to target all img elements with an alt attribute containing the word "logo". This is useful for styling specific images on your website. Attribute selectors can also be used to target elements based on the existence of an attribute, such as targeting all anchor tags that have a title attribute.
Here are some common attribute selectors and their uses:
By using attribute selectors, you can create more specific and effective CSS styles for your website.
14. X
Attribute selectors are a powerful tool in CSS, allowing you to target elements based on their attributes. They can be used to match a wide range of attributes, including class attributes.
See what others are reading: Css Selector Multiple Attributes
You can use attribute selectors to target elements with specific attribute values, such as a data-icon attribute with a value of "arrow". This can be particularly useful when you need to apply different styles to elements with different attribute values.
One way to use attribute selectors is to target elements with a custom attribute, such as a data-filetype attribute. This can be useful when you need to target elements with specific attribute values, such as all anchors that link to an image.
Here are some examples of attribute selectors:
By using attribute selectors, you can create more specific and targeted CSS rules, making it easier to style your web pages.
In some cases, attribute selectors can be used to target elements with specific attribute values, such as all anchors that link to an image. This can be particularly useful when you need to apply different styles to elements with different attribute values.
Checked Input
The :checked pseudo-class is a powerful tool in your CSS toolkit. It targets radio buttons, checkboxes, or options in a select element that are currently selected/checked.
You can use it to style the sibling label of a checkbox input, as shown in an example where appearance: none is used to remove the default styling of the checkbox input. This allows you to add styling on the label when the checkbox is focused.
Readers also liked: Css Hr Styling
Required Required Input
The :required pseudo-class targets input elements that have the required attribute, indicating that they must be filled out before the form can be submitted. This is crucial for ensuring users complete the necessary fields.
Inputs that are required have the required attribute, which is a key characteristic of the :required selector. This attribute is essential for form validation.
The :required selector selects inputs that are required, making it a valuable tool for developers. It's used to identify and style required fields in a form.
In many browsers, required fields are visually distinguished from non-required fields, making it easier for users to understand what's expected of them. This helps prevent errors and ensures a smoother user experience.
Return the revised heading
Attribute selectors are a powerful tool in CSS that allow you to target specific elements based on their attributes. They can be particularly useful when working with HTML elements that have specific attributes.
You can select element(s) that have a specified attribute using the [attr] attribute selector. This is a simple yet effective way to target elements with specific attributes.
The :first-child pseudo class is another useful tool that allows you to target only the first child of an element's parent. This can be particularly useful when working with lists or other elements where you want to apply a specific style to the first or last item.
9. X[title]
The X[title] attribute selector is a powerful tool for targeting elements based on their title attribute. This selector will only select the anchor tags that have a title attribute, leaving out those that don't.
You can use this selector to apply different styles to elements with and without a title attribute. For example, you can use it to highlight elements with a title attribute, making them stand out from the rest.
The X[title] selector is a great way to add interactivity to your web pages, and it's easy to use. Simply add the title attribute to the elements you want to target, and then use the X[title] selector in your CSS to apply the desired styles.
Here are some examples of how you can use the X[title] selector:
- Target all anchor tags with a title attribute: `a[title] { color: red; }`
- Target all anchor tags without a title attribute: `a:not([title]) { color: blue; }`
By using the X[title] selector, you can create complex and interactive designs that engage your users and enhance their experience.
Frequently Asked Questions
How to select data in CSS?
To select data in CSS, you can use basic tag name selectors or more specific attribute selectors, such as class and ID selectors, to target specific HTML elements. Learn more about advanced CSS selection methods to refine your styling and layout.
What are the 4 CSS selectors?
There are four main types of CSS selectors: simple, combinator, pseudo-class, and pseudo-element selectors, each used to target and style different aspects of HTML elements. Understanding these selectors is key to writing effective and efficient CSS code.
How to call data attribute in CSS?
To access a data attribute in CSS, use the attribute name without the "data-" prefix and convert any dashes to camel case. For example, "data-column-name" becomes "columnName
Sources
- https://fffuel.co/css-selectors/
- https://www.freecodecamp.org/news/css-selectors-cheat-sheet-for-beginners/
- https://javascript.plainenglish.io/class-vs-attribute-selectors-in-css-which-weighs-more-99067cb9c1e6
- https://webdesign.tutsplus.com/the-30-css-selectors-you-must-memorize--net-16048t
- http://web.simmons.edu/~grabiner/comm244/weekfour/selectors
Featured Images: pexels.com