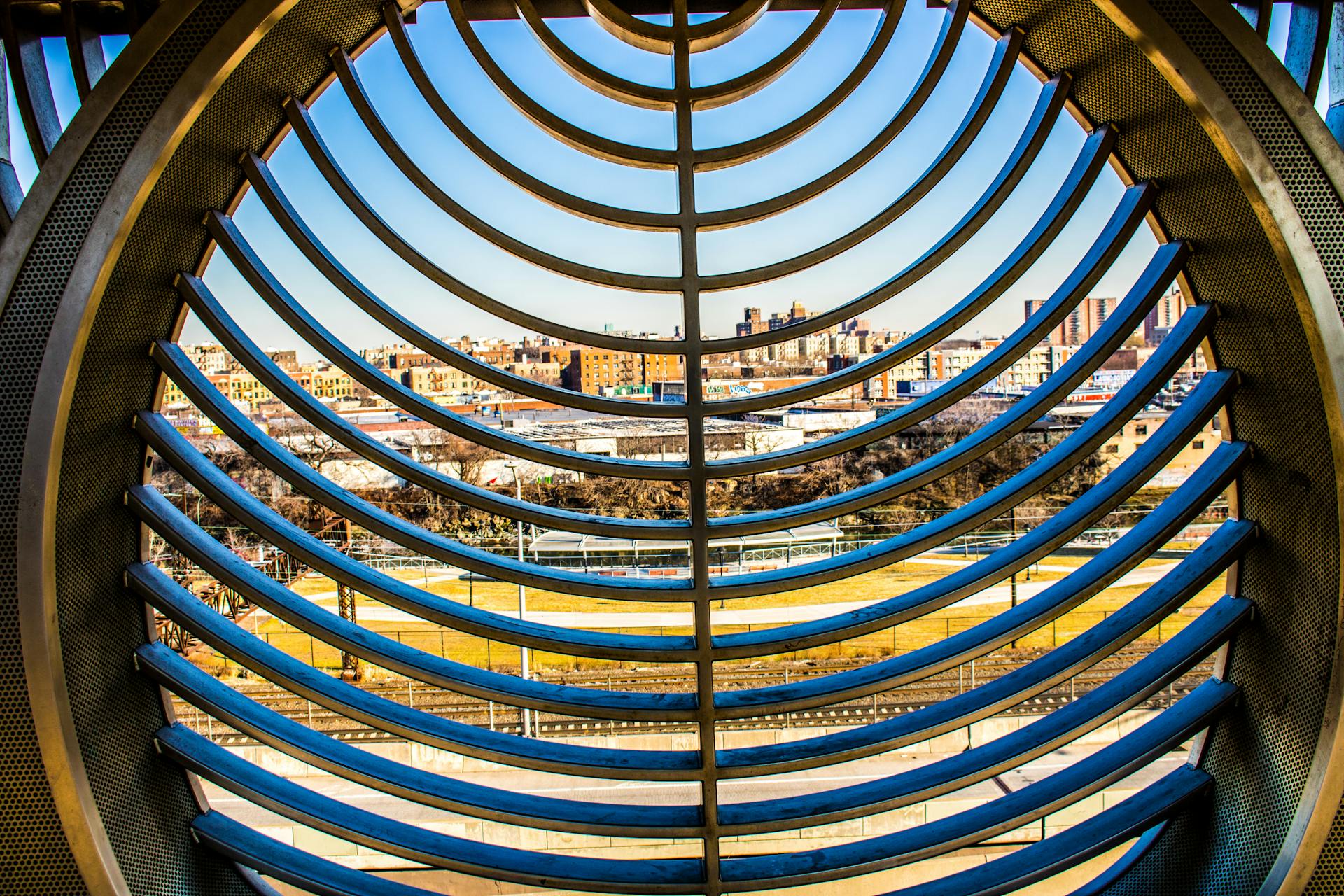
Setting up a CSS Grid with three columns is straightforward. You can achieve this by defining a grid container with the grid-template-columns property and specifying three equal columns using the repeat function, like this: grid-template-columns: repeat(3, 1fr).
To create a responsive grid, you can use the minmax function to set a minimum and maximum width for each column. For example, grid-template-columns: repeat(3, minmax(100px, 1fr)) creates columns that are at least 100px wide and can grow up to 1fr (one fractional unit).
The grid-template-columns property can also be used to create unequal columns. For instance, grid-template-columns: 1fr 2fr 1fr creates three columns where the middle column is twice as wide as the other two.
Setting Up 3 Column Layout
Static 3 column layouts use CSS grid to make columns stay side-by-side, equal-width, and equal-height even on small mobile screens.
You can create a three-column layout using CSS grid by defining grid column & row tracks explicitly via grid-template-columns and grid-template-rows settings of the grid container.
Grid-template-columns property creates a column track with each value. For example, grid-template-columns: 200px 1fr 2fr creates a three-column grid layout with column 1 having a fixed width of 200px, column 2 being 1fr wide, and column 3 being 2fr wide.
The fractional unit, fr, is a new flexible unit that takes up x parts of the available space. To calculate the width of a column with a fractional unit, subtract the fixed widths and gaps, then divide the remaining space by the total number of fractional units.
Three equal-width columns across all viewports and devices can be created by using the .g-col-4 classes.
To change the layout by viewport size, add responsive classes to the .g-col-4 classes.
Customizing Columns
To create a three-column grid layout, you can use the grid-template-columns property, which creates column tracks. For example, grid-template-columns: 200px 1fr 2fr creates a three-column grid with a fixed width of 200px for the first column, and 1fr and 2fr for the second and third columns, respectively.
The fractional unit (fr) takes up an equal part of the available space, so in this case, 1fr equals 300px. This means the second column is 300px wide, and the third column is 600px wide.
You can also add gutters between your columns by setting the column-gap property. For example, if you have gutters set to 2rem, each column width will be 100% minus 4rem (the total gutter-width) divided by 3.
Here's a summary of the grid-template-columns property:
Column-Gap
You can set the size of the grid lines using the column-gap property, which is equivalent to grid-column-gap. This property specifies the size of the gutters between the columns, and it's a length value, such as 2rem.
The gutters are only created between the columns, not on the outer edges.
If you want to add gutters between your columns using Flexbox, you can do so by setting the column-gap property. However, you'll need to explicitly set the correct width to each column to allow for the gutters.
To calculate the correct column width, subtract the total gutter width from 100% and divide by the number of columns. For example, if you have gutters set to 2rem and three columns, each column width would be 100% minus 4rem (2rem x 2 columns) divided by 3.
Here's a simple formula to calculate the correct column width:
- Column width = (100% - (n x gutter width)) / n
- Where n is the number of columns
Note that the units used in your calc should match the units used in your column-gap declaration.
Children's Properties
Grid items have some specific properties that control how they're laid out within a grid. These properties are known as the "children's properties".
Grid items can be placed anywhere within a grid using the grid-column-start and grid-column-end properties. These properties can be set to a specific number or a span value to define the size of the item.
You can also use grid-row-start and grid-row-end to place items at specific row locations. These properties work similarly to grid-column-start and grid-column-end.
Some properties have no effect on a grid item, including float, display: inline-block, display: table-cell, vertical-align, and column-*.
Here are some key children's properties in one place:
- grid-column-start
- grid-column-end
- grid-row-start
- grid-row-end
- grid-column
- grid-row
- grid-area
- justify-self
- align-self
- place-self
These properties can be used to fine-tune the layout of your grid items and create a custom look for your website or application.
Responsive Design
Responsive design is a game-changer for building websites that adapt to different screen sizes.
The key to responsive design is using a system that automatically adjusts the layout based on the screen size. In the case of a three-column layout, this means that the columns will stack on smaller screens and sit side-by-side on larger screens.
At the mobile breakpoint, typically referred to as "small", the layout will be one column. This is a common design decision to prioritize a single column on smaller screens for easier navigation.
The tablet breakpoint, or "medium", is where the layout transitions to three columns. This is because tablets often have a larger screen size than smartphones, making it more suitable for a multi-column layout.
We don't need to specify the desktop breakpoint, which is often referred to as "large", because the layout will automatically remain three columns. This is a convenient feature that saves us from having to define additional breakpoints.
Introduction to CSS Grid
CSS Grid is a powerful layout system that allows you to create complex and flexible layouts with ease. It's a game-changer for web development, and once you learn it, you'll wonder how you ever managed without it.
In a CSS Grid layout, you can define a grid container and specify the number of columns and rows it should have. For example, in a 3-column grid, you can define 3 grid tracks, each with a specific width and margin.
A grid container is the parent element that contains all the grid items, and it's where you define the grid layout.
Column & Row Tracks Setup
To set up column and row tracks in a grid layout, you can use the Grid template columns (grid-template-columns) and Grid template rows (grid-template-rows) settings of your grid container.
Explicitly defining grid column and row tracks is possible using these properties.
grid-template-columns: 200px 1fr 2fr creates a three-column grid layout with each value creating a column track.
Column 1 has a fixed width of 200px.
Column 2 is 1fr wide, meaning it takes up one part of the available space.
Column 3 is 2fr wide, meaning it takes up two parts of the available space.
The fractional unit (fr) is a new flexible unit that takes up x parts of the available space.
fr is calculated by subtracting all non-fr values and gaps, then allocating the remaining space towards the fractional units.
For example, if the remaining available width is 900px and there are 3 fractional units, 1fr equals 300px.
Column 2 is 300px wide, and column 3 is 600px wide.
grid-template-rows: 100px 300px explicitly defines the first two rows, with row 1 being 100px high and row 2 being 300px high.
The height of any row after row 2 is determined by the height of its content by default.
Introduction to CSS
CSS has always been used to layout our web pages, but it's never done a very good job of it.
Tables, floats, positioning, and inline-block were essentially hacks that left out important functionality, like vertical centering.
Grid is the very first CSS module created specifically to solve the layout problems we've all been hacking our way around for as long as we've been making websites.
Grid completely changes the way we design user interfaces compared to any web layout system of the past.
Flexbox is a great layout tool, but its one-directional flow has different use cases – and they actually work together quite well with Grid.
Sources
- https://matthewjamestaylor.com/3-column-layouts
- https://css-tricks.com/snippets/css/complete-guide-grid/
- https://getbootstrap.com/docs/5.1/layout/css-grid/
- https://academy.bricksbuilder.io/article/css-grid-layout/
- https://www.nobledesktop.com/learn/html-css/creating-columns-intro-to-css-grid-media-queries
Featured Images: pexels.com