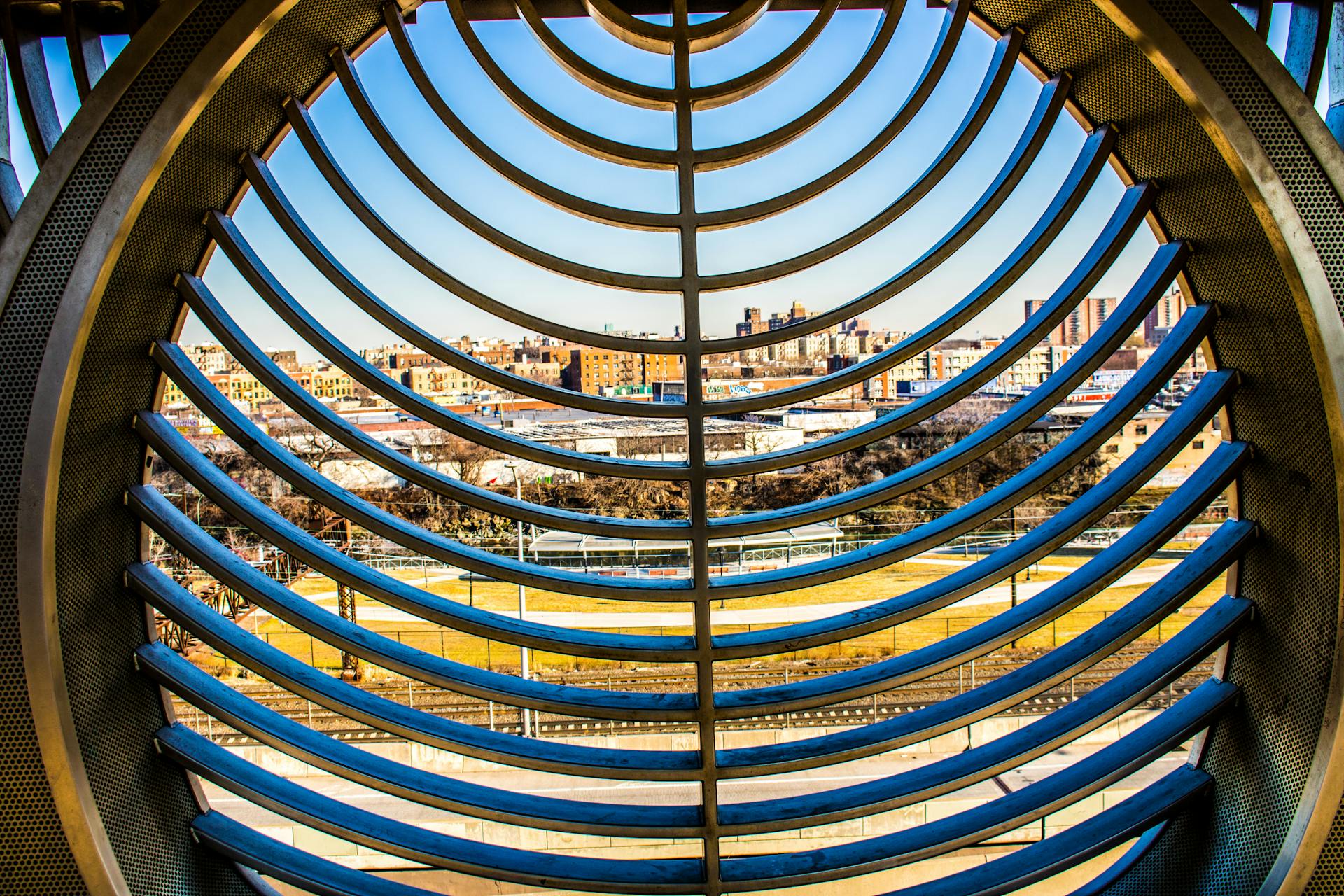
The world of CSS Grid is a game-changer for web designers and developers. By using the `grid-auto-flow` property, you can control how grid items are automatically placed in the grid.
You can set `grid-auto-flow` to `row` or `column` to determine the direction of auto-placed items. For example, in the "Two-way auto-flow" example, setting `grid-auto-flow` to `row dense` and `column dense` allowed for a two-way auto-flow layout.
In the "Single column auto-flow" example, setting `grid-auto-flow` to `column` resulted in a single column layout. This is because the `grid-auto-flow` property dictates how items are placed in the grid, allowing for more flexibility in layout design.
Grid Tracks and Setup
Grid tracks are the building blocks of a CSS grid layout, and they can be set up in two ways: explicitly or implicitly.
To set up column tracks explicitly, you can use the grid-template-columns property, like this: grid-template-columns: 200px 1fr 2fr. This creates a three-column grid layout with a fixed width of 200px for the first column.
The fractional unit, or "fr", is a new flexible unit that takes up a portion of the available space. In the example above, the remaining available width is 900px after subtracting the fixed 200px width of column 1.
The fr unit is calculated by dividing the remaining available space by the total number of fractional units. In this case, 1fr equals 300px, and 2fr equals 600px.
To set up row tracks explicitly, you can use the grid-template-rows property, like this: grid-template-rows: 100px 300px. This explicitly defines the first two rows, which are 100px and 300px high, respectively.
Any row after the second row will have a height determined by its content by default, unless you set up an implicit grid to change this behavior.
Grid Properties
Grid Properties are essential in creating flexible and dynamic layouts with CSS Grid. Using the repeat() function with the min-content keyword is a great way to achieve this.
The min-content keyword sizes tracks based on intrinsic content, taking the minimum space required by the content within. This is useful for avoiding unnecessarily wide empty spaces within the grid.
To use the min-content keyword, simply set the grid-template-columns property to repeat(4, min-content), for example. This will create four columns where each column's width is determined by its content.
Here's a quick rundown of the benefits of using the min-content keyword:
- Avoids unnecessarily wide empty spaces
- Columns have different widths depending on their content
- Content dictates the width of the tracks
Important Terminology
Grid terminology can be confusing, but it's essential to understand the basics. The Grid specification defines several key terms.
To create responsive grid layouts, we use keywords like auto-fill and auto-fit. Auto-fill and auto-fit are used to create responsive grid layouts without media queries.
The Grid specification defines several key terms, but fortunately, there aren't many of them. Understanding these terms will help you navigate Grid concepts with ease.
Grid items can be expanded to fill available space using the auto-fit keyword. This is in contrast to auto-fill, which keeps the available space.
Named Lines
Named lines in CSS Grid are a game-changer for creating structured and readable layouts. They provide human-readable labels to the lines in the grid, making it easier to reference and position items within the grid.
You can name both column and row lines, and even use custom names like [start-tech] and [end-content] to simplify content alignment. This removes the need to rely on numerical indices, making the layout more intuitive.
For example, in the code `grid-template-columns: [start-tech]1fr[end-tech start-content]2fr[end-content start-lifestyle]1fr[end-lifestyle];`, we used named grid lines to create a repeating pattern of 3 columns, each controlled by the named lines.
Named lines can be combined with the `repeat()` function to streamline the creation of repetitive column patterns. For instance, `repeat(5, [ham]1fr[burger])` establishes a pattern of 3 columns, each controlled by the named lines.
You can also use -start and -end suffixes on your named areas, as shown in the example `grid-row: row1-start / row2-end;`. Notice how the same variable like `bd-end` will be different for `grid-row` and `grid-column`.
Here's a quick summary of the syntax for naming lines:
Named lines offer a structured and adaptable approach to creating dynamic grid layouts in CSS, making it easier to create complex and responsive designs.
Template-Columns Template-Rows
Grid template columns and rows are the building blocks of any grid layout. They define the size and arrangement of the columns and rows of the grid.
The grid-template-columns property creates a space-separated list of values that represent the track size, with the space between them representing the grid line. You can use a length, a percentage, or the fr unit, which takes up a fraction of the free space in the grid.
The fr unit is a flexible unit that allows you to set the size of a track as a fraction of the free space of the grid container. For example, 1fr equals 300px, and 2fr equals 600px.
Here's an example of how to use grid-template-columns: grid-template-columns: 200px 1fr 2fr. This creates a three-column grid layout with a fixed width of 200px for the first column, and the second and third columns taking up 1fr and 2fr of the remaining space, respectively.
Grid template rows work similarly, with the grid-template-rows property creating a space-separated list of values that represent the track size. You can use a length or the fr unit, just like with grid-template-columns.
If you only explicitly define the height for the first two rows, the height of any row after that is determined by the height of its content by default.
Justify-Content
Justify-content is a CSS grid property that helps you align the grid items along the inline (row) axis. It's a crucial property when working with grid layouts, and it's used to control the spacing and alignment of grid items.
There are several values you can use for justify-content, including start, end, center, stretch, space-around, space-between, and space-evenly. The start value aligns the grid to be flush with the start edge of the grid container, while the end value aligns it to be flush with the end edge.
The center value aligns the grid in the center of the grid container, and the stretch value resizes the grid items to allow the grid to fill the full width of the grid container. The space-around value places an even amount of space between each grid item, with half-sized spaces on the far ends.
Here are some key values for justify-content:
Using justify-content, you can create flexible and responsive grid layouts that adapt to different screen sizes and devices.
Align-Items
Align-items is a property that aligns grid items along the block (column) axis. It's the opposite of justify-items, which aligns along the inline (row) axis.
The align-items property has several values, including stretch, start, end, center, and baseline. The default value is stretch, which fills the whole height of the cell.
You can also use modifiers like first baseline and last baseline with the baseline value. These modifiers will use the baseline from the first or last line in the case of multi-line text.
Here are the values of the align-items property in a list:
- stretch – fills the whole height of the cell
- start – aligns items to be flush with the start edge of their cell
- end – aligns items to be flush with the end edge of their cell
- center – aligns items in the center of their cell
- baseline – aligns items along text baseline
- first baseline – uses the baseline from the first line in the case of multi-line text
- last baseline – uses the baseline from the last line in the case of multi-line text
You can set the align-items property on individual grid items using the align-self property. This allows you to customize the alignment of specific items within the grid.
Min-Content Keyword
The min-content keyword is a game-changer for creating flexible grid layouts. It allows you to size columns or rows based on the intrinsic content of their items, taking the minimum space required by the content within the track.
This keyword is particularly useful when you want to avoid unnecessarily wide empty spaces within the grid, allowing the content to dictate the width of the tracks. In the example from Example 2, the grid-template-columns property is set to repeat(4, min-content), which means that the grid will have four columns, and each column's width will be determined by its content.
The min-content keyword is not limited to grid-template-columns; it can also be used with grid-template-rows. This means you can create flexible grid layouts where rows or columns adjust their size based on the content they contain.
Here are some key facts about the min-content keyword:
- The min-content keyword takes the minimum space required by the content within the track.
- It's useful for avoiding unnecessarily wide empty spaces within the grid.
- It can be used with both grid-template-columns and grid-template-rows.
By using the min-content keyword, you can create responsive grid layouts that adapt well to various content lengths while maintaining a balance between track sizes. For example, in Example 7, the max-content keyword is used to create a grid where columns adjust their width to accommodate the widest content within them. However, if you want to use min-content instead, you can simply replace max-content with min-content in the grid-template-columns property.
Grid Layouts
Creating a grid layout is straightforward: simply set the Display value to grid for any layout element, and it becomes your Grid Container. This element is the foundation of your grid layout.
Every direct child element of your Grid container is a Grid Item, with settings for Grid column & Grid row to place it within the grid.
Grid Layouts
Grid layouts are a powerful tool for arranging elements on a web page.
You can turn any layout element into a CSS grid layout by setting the Display value to grid.
This element is your Grid Container, and every direct child element of your Grid container is a Grid Item.
Grid items are laid out in rows by default, covering the full width of the grid container.
To visualize the grid cells, a grid overlay becomes visible when editing a grid container in the builder.
Align Content
Aligning content in grid layouts can be a bit tricky, but don't worry, I've got you covered. If your grid items are sized with non-flexible units like px, you can use the align-content property to align the grid within the grid container.
The align-content property aligns the grid along the block (column) axis, which is the opposite of justify-content that aligns the grid along the inline (row) axis. You can set the alignment to start, end, center, stretch, space-around, space-between, or space-evenly.
To give you a better idea, here are the options you have for align-content:
- start – aligns the grid to be flush with the start edge of the grid container
- end – aligns the grid to be flush with the end edge of the grid container
- center – aligns the grid in the center of the grid container
- stretch – resizes the grid items to allow the grid to fill the full height of the grid container
- space-around – places an even amount of space between each grid item, with half-sized spaces on the far ends
- space-between – places an even amount of space between each grid item, with no space at the far ends
- space-evenly – places an even amount of space between each grid item, including the far ends
Keep in mind that not all browsers support the place-content shorthand property, except for Edge.
Flow
Grid layouts offer a flexible way to arrange items on a web page, and one key aspect of this is how items are placed when not explicitly defined. The grid-auto-flow property controls this behavior.
This property determines how the auto-placement algorithm works, which kicks in to place items when not explicitly placed on the grid.
There are three options for grid-auto-flow: row, column, and dense. The default is row.
The row option tells the auto-placement algorithm to fill in each row in turn, adding new rows as necessary.
The column option tells the auto-placement algorithm to fill in each column in turn, adding new columns as necessary.
The dense option attempts to fill in holes earlier in the grid if smaller items come up later, but it only changes the visual order of items and can cause them to appear out of order, which is bad for accessibility.
Here are the three options for grid-auto-flow:
- row – fills in each row in turn, adding new rows as necessary (default)
- column – fills in each column in turn, adding new columns as necessary
- dense – attempts to fill in holes earlier in the grid if smaller items come up later
It's worth noting that the dense option can affect accessibility, so use it with caution.
Explicit vs Implicit Layout
Grid layouts in CSS can be classified into two types: explicit and implicit. Explicit grid layouts are defined using CSS properties like grid-template-columns and grid-template-rows, where you specify the number and size of rows and columns.
The explicit grid layout is created using a defined grid structure, where you determine the dimensions and layout of the grid. For example, in the code `.grid-container{display:grid;grid-template-columns:1fr2fr1fr;grid-template-rows:100px200px100px100px;}`, three columns with relative widths and four rows with fixed heights are explicitly defined.
Implicit grid layouts, on the other hand, are automatically created to accommodate additional content beyond what is explicitly defined. This is particularly useful when there are more items to place in the grid than the number of tracks that have been explicitly defined.
The implicit grid adapts to the added content, automatically creating additional rows or columns as needed. For instance, in the code `.grid-container{display:grid;grid-template-columns:1fr1fr1fr;grid-template-rows:100px100px;grid-auto-rows:200px;}`, additional rows are created automatically when more items are added to the grid.
You can define the column & row sizes of the implicit grid via the Grid auto columns (grid-auto-columns) and Grid auto rows (grid-auto-rows) settings of your grid container. This is useful when you want to customize the size of the implicitly created tracks.
Here's a summary of the key differences between explicit and implicit grid layouts:
Featured Images: pexels.com