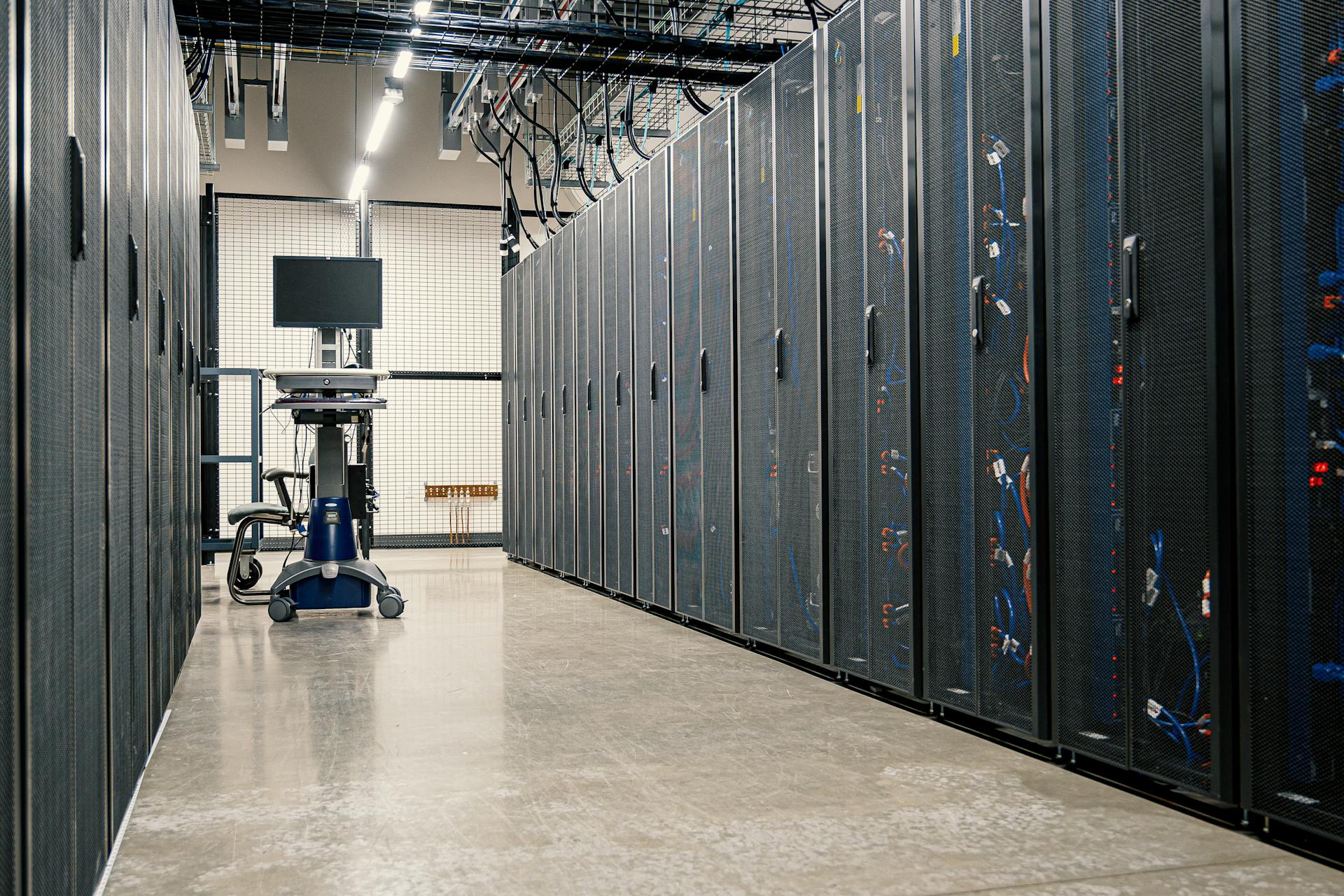
To deploy to Azure Container Apps with Docker and .NET Core, you'll need to create a Dockerfile. The Dockerfile is used to define the build process for your containerized application.
A simple Dockerfile for a .NET Core application might look like this: `FROM mcr.microsoft.com/dotnet/core/sdk:3.1`. This line tells Docker to use the official .NET Core SDK image as the base for your application.
Once you've created your Dockerfile, you can build a Docker image using the `docker build` command. You can then push this image to Azure Container Registry (ACR) using the `docker push` command.
To deploy to Azure Container Apps, you'll need to create a container app in the Azure portal. From there, you can configure the container app to use your Docker image from ACR.
Prerequisites
To deploy to Azure Container Apps, you'll need a few things set up first. You'll need a basic understanding of building applications with the ASP.NET Core framework.
You'll also need to have the .Net Core runtime installed on your computer. This is a requirement to get started with Azure Container Apps.
Docker Desktop is another essential tool you'll need. You can install it on your local machine by following Docker's tutorial for Windows or macOS.
An Azure account is also required. If you don't already have one, you can create an account for free. You'll need the User Access Administrator or Owner permission on the Azure subscription to proceed.
You'll also need a GitHub account. Sign up for free to get started.
Here's a summary of the prerequisites:
Having a local source code directory is also necessary if you're using local source code. If you're using an existing image, you'll need your registry server, image name, and tag.
Creating Azure Container Apps
Creating Azure Container Apps is a straightforward process that can be done in Visual Studio or using the command line. You can choose to create a new Azure Container App or use an existing one.
To create a new Azure Container App, right-click the project node and select Publish. Then, choose Azure from the list of publishing options and select Next. On the Specific target screen, choose Azure Container Apps (Linux) and select Next again.
You'll then create an Azure Container App to host the project by selecting the green plus icon on the right. In the Create new dialog, enter the necessary values, including the name, resource group, and location. Select Create to finalize the creation of your container app.
Visual Studio and Azure will create the needed resources on your behalf, which may take a couple of minutes. Once the resources are created, you can select Next to proceed with the deployment process.
A container app needs to be accessible to ingress traffic, so ensure that you expose port 8080 to listen for incoming requests.
Here are the steps to create a new Azure Container App:
- Select Create to finalize the creation of your container app.
- Visual Studio and Azure create the needed resources on your behalf.
- Once the resources are created, select Next to proceed with the deployment process.
- Exposure port 8080 to listen for incoming requests.
Docker Image
To build a Docker image for your project, you'll need to use the registry URL from the previous step. This will involve running a command that maps the container image with the Azure Container Registry. Replace dotnetcoreapi.azurecr.io and dotnet-api with the names you chose for the registry URL and login server details.
The command to build the Docker image is straightforward: it's a simple run command that uses the registry name and login server you created earlier. You can find this command in the Azure documentation.
To build the Docker image, you'll need to have the Dockerfile in the same directory as the command. The Dockerfile is a text file that contains instructions for building the Docker image. It's created automatically when you enable Docker in your project.
Create Azure Registry
To create an Azure Container Registry, head to the Azure portal homepage and click Create a resource. Then select Containers > Container Registry.
You can use any name you want for the registry, but remember to use it instead of the example name "dockerdotnetcoreapi" as you follow the tutorial.
On the registry creation page, enter the details required for the registry. Click Review + Create to review the registry information, and then click Create to set up a new registry instance.
Enabling Docker access in the Azure Container Registry is crucial to the deployment process, as it lets you remotely log into the Azure container registry through the CLI and push images to it.
Here are the steps to create an Azure Container Registry:
- Create a new resource group in Azure.
- Go to the Azure portal homepage and click Create a resource.
- Then select Containers > Container Registry.
- Fill in the required details for the registry.
- Click Review + Create to review the registry information.
- Click Create to set up a new registry instance.
Alternatively, you can also create an Azure Container Registry through Visual Studio by following these steps:
1. Right-click the MyContainerApp project node and select Publish.
2. In the dialog, choose Azure from the list of publishing options, and then select Next.
3. On the Specific target screen, choose Azure Container Apps (Linux), and then select Next again.
4. On the Registry screen, you can either select an existing Registry if you have one, or create a new one by clicking the green + icon on the right.
5. Fill in the required values for the new registry, and then select Create.
6. Once the container registry is created, make sure it's selected, and then choose Finish.
The Docker Image
The Docker image is the core of your containerized application. It's a package that contains everything your application needs to run, including code, dependencies, and configurations. You can create a Docker image using a Dockerfile, which is a text file that contains instructions for building the image.
To build a Docker image, you'll need to use the registry URL from the Azure Container Registry. This URL is used to map the container image with the registry. You can replace the default registry URL with your own by using the `az acr login` command.
The Docker image can be built using the `az acr build` command. This command initiates the image build and push process using Azure Container Registry. You can also build the image locally using Docker by running the `docker build` command.
Here are the steps to build a Docker image:
- Use the `az acr build` command to initiate the image build and push process.
- Replace the default registry URL with your own using the `az acr login` command.
- Use the `docker build` command to build the image locally.
Note: Make sure to run the `az acr login` command before building the image using `az acr build`.
Publishing the App
Publishing the app is a crucial step in deploying to Azure Container Apps. You can publish the app using Visual Studio, GitHub Actions, or the Azure CLI.
To publish the app using Visual Studio, you need to choose Publish in the upper right of the publishing profile screen. This process might take a moment, so wait for it to complete.
Visual Studio uses the Dockerfile to build the container image that the Azure Container Apps run. The Dockerfile is created because the project template had the Enable Docker setting selected.
You can also publish the app using GitHub Actions. GitHub Actions is a powerful tool for automating, customizing, and executing development workflows directly through the GitHub repository of your project.
To publish the app using GitHub Actions, Visual Studio creates a .github folder to the root directory of the project, along with a generated YAML file inside of it. The YAML file contains GitHub Actions configurations to build and deploy your app to Azure every time you push your code.
The workflow is complete when you see a green checkmark next to the build and deploy jobs. When you browse to your Container Apps site, you should see the latest changes applied.
Here are the steps to deploy from a GitHub repository:
- Creates a resource group.
- Creates an environment and Log Analytics workspace.
- Creates a registry in Azure Container Registry.
- Builds the container image using the Dockerfile.
- Pushes the image to the registry.
- Creates and deploys the container app.
- Creates a GitHub Actions workflow to build the container image and deploy the container app when future changes are pushed to the GitHub repository.
Note that the up command creates a GitHub Actions workflow in your repository's .github/workflows folder. The workflow is triggered to build and deploy your container app when you push changes to the repository.
A Dockerfile is required to build the image. When the Dockerfile includes the EXPOSE instruction, the command configures the container app's ingress and target port using the information in the Dockerfile.
Build the App
Building the app is a crucial step in deploying to Azure Container Apps. You can use Azure Container Registry (ACR) tasks to build and push the Docker image for the album API without installing Docker locally.
To initiate the image build and push process using ACR, run the following command, which should be executed within the src folder where the Dockerfile is located. The . at the end of the command represents the docker build context.
You can also use the az acr build command to build and push the image to the new container registry. This command shows the upload progress of the source code to Azure and the details of the docker build and docker push operations.
To build the container image locally using Docker and push the image to the new container registry, you'll need to run the following command within the src folder where the Dockerfile is located. The . at the end of the command represents the docker build context.
Here are the steps to build the container image using Docker:
- Build a container image for the album API and tag it with the fully qualified name of the ACR login server.
- Run the command within the src folder where the Dockerfile is located.
In the case of using ACR tasks, you can build and deploy your first container app from your forked GitHub repository with the containerapp up command. This command will create the resource group, Container Apps environment with a Log Analytics workspace, an Azure Container Registry, and a GitHub Action workflow to build and deploy the container app.
Sources
- https://circleci.com/blog/deploy-dockerized-dotnet-core-to-azure/
- https://learn.microsoft.com/en-us/azure/container-apps/tutorial-code-to-cloud
- https://learn.microsoft.com/en-us/azure/container-apps/deploy-visual-studio
- https://learn.microsoft.com/en-us/azure/container-apps/quickstart-repo-to-cloud
- https://learn.microsoft.com/en-us/azure/container-apps/containerapp-up
Featured Images: pexels.com