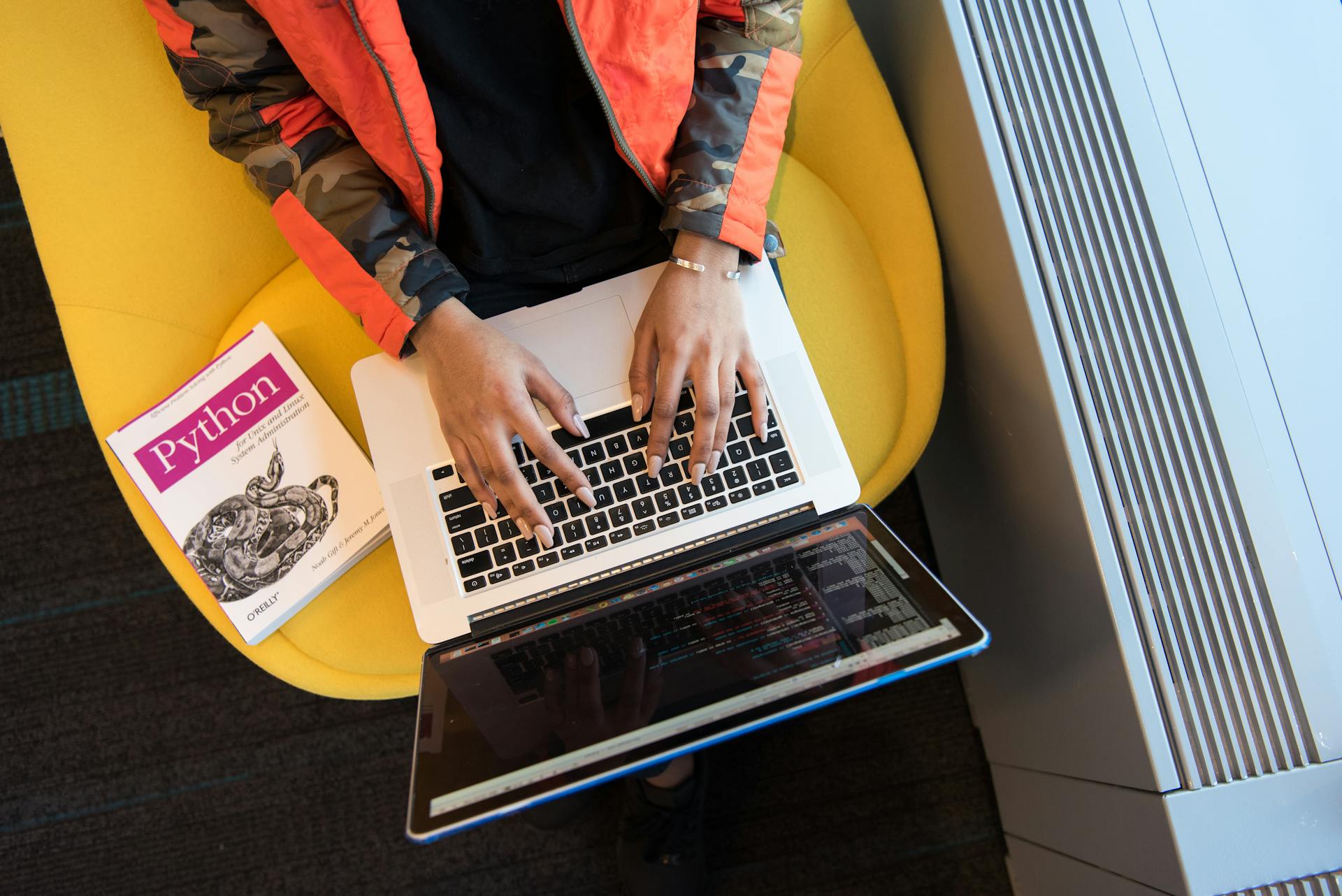
The Django 5 by Example book is a great resource for beginners. It's designed to be easy to follow, even if you have no prior experience with Django.
This book is perfect for those who want to learn Django quickly and efficiently. It's filled with practical examples that will have you building your own projects in no time.
The book starts with the basics, covering topics like setting up a new Django project and understanding its structure. You'll learn how to create a new project using the `django-admin` command and understand the different components of a Django project.
As you progress through the book, you'll learn how to build a simple blog application using Django. You'll see how to create models, views, and templates, and how to connect them to the database.
Readers also liked: How to Create a Website in 5 Minutes for Free
Django 5 Basics
Django is a high-level Python framework that makes it easy to build robust and scalable web applications.
With Django, you can implement responsive user interfaces using Bootstrap, which will give your application a polished and modern look.
You'll learn to manage data storage in databases effectively, which is crucial for any web application. This includes understanding how to store and retrieve data efficiently.
Here are some key features you'll learn about in Django 5:
- URLs: mapping URLs to views
- Templates: rendering dynamic content
- Models: defining database tables
- Forms: handling user input
By mastering these basics, you'll be well on your way to building complex web applications with Django.
Management Commands
Django's management commands are a powerful tool for managing your project. They allow you to perform various tasks, such as creating and managing databases, without having to write custom code.
To create the file structure for a new Django project, you can use the command `django-admin startproject mysite`. This will create the basic directory structure for your project.
Creating a new Django application is also a breeze with the `python manage.py startapp blog` command. This will create the basic directory structure for your new application.
Applying all database migrations is a crucial step in setting up your project. You can do this with the `python manage.py migrate` command.
Readers also liked: I Will Always Write Back Online Book
Viewing the SQL statements that will be executed with the first migration of the blog application can be done with the `python manage.py sqlmigrate blog 0001` command. This will show you the exact SQL code that will be run to create the database tables.
Running the Django development server is as simple as typing `python manage.py runserver` in your terminal. This will start the development server, allowing you to access your project in your web browser.
You can also specify the host, port, and settings file when running the development server using the `python manage.py runserver 0.0.0.0:8000 myproject.settings` command. This gives you more control over the development server and allows you to customize its behavior to suit your needs.
Discover more: Next Js Server Side Api Call
What You'll Learn
In this Django 5 Basics course, you'll learn how to use Django's key features, including URLs, templates, models, and forms. This will give you a solid foundation in building robust and scalable web applications.
You'll also learn how to implement responsive user interfaces using Bootstrap, which is a popular front-end framework. This means your web application will look great on any device, whether it's a desktop computer, tablet, or smartphone.
To manage data storage effectively, you'll learn how to use databases with Django. This is a crucial skill for any web developer, as it allows you to store and retrieve data efficiently.
One of the most powerful features of Django is its built-in admin interface. You'll learn how to explore and use this interface to manage your web application's data.
Django also has a powerful built-in authentication system, which you'll learn how to harness to secure your web application. This includes creating user accounts, managing passwords, and more.
Finally, you'll learn how to deploy your Django project on the internet, making it accessible to users worldwide. This involves setting up a web server and configuring your project to work with it.
Here's a summary of what you'll learn in this course:
- Understand and use Django key features, including URLs, templates, models, and forms
- Implement responsive user interfaces using Bootstrap
- Manage data storage in databases effectively
- Explore the powerful built-in admin interface with Django
- Harness Django's powerful built-in authentication system
- Deploy your Django project on the internet for users
Django 5 by Example
Django 5 by Example is a fantastic resource for learning Django by doing. You can build four real-world Django apps and learn Django by creating fully functional web applications.
One of the benefits of this book is that it helps you get familiar with Django management commands, which are essential for managing your Django project.
To create a new Django project, you can use the command to create the file structure for a new Django project named mysite. This is a crucial step in setting up your project.
To create a new Django application, you use a similar command to create the file structure for a new Django application named blog. This will help you organize your project and create a new app.
To apply all database migrations, you can use the command. This is an important step in setting up your database.
Here are some key management commands to keep in mind:
Building Features
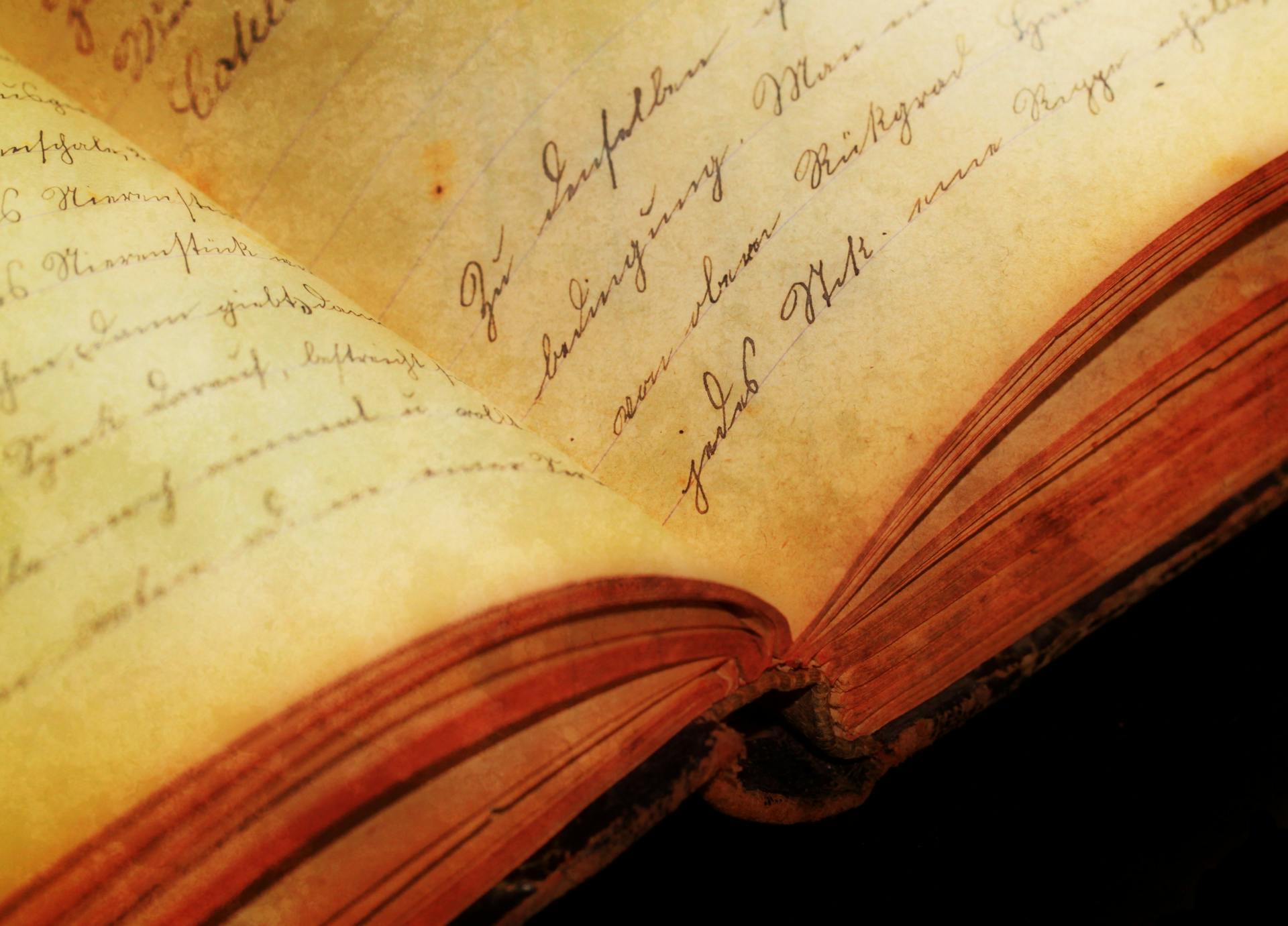
Building a comment system is a crucial feature in any blog application, and Django makes it relatively straightforward. To build a comment system, you'll need to create a comment model to store user comments on posts.
A Django form is also required to allow users to submit comments and manage data validation. This form will handle the submission of new comments, ensuring that the necessary data is provided and validated.
To process the form and save a new comment to the database, a view is necessary. This view will handle the form submission, validate the data, and save the comment to the database.
A list of comments and the HTML form to add a new comment can be included in the post detail template. This will allow users to view existing comments and submit new ones.
Here's an interesting read: Data Lakehouse Examples
Adding Pagination to Posts
You can easily store tens or hundreds of posts in your database, making it necessary to split the list of posts across several pages and include navigation links to the different pages.
Django has a built-in pagination class that allows you to manage paginated data easily, enabling you to define the number of objects you want to be returned per page.
Google uses pagination to divide search results across multiple pages, a functionality you can find in almost every web application that displays long lists of items.
You can retrieve the posts that correspond to the page requested by the user using Django's built-in pagination class.
Pagination links, like those shown in Figure 2.3, typically include navigation links to the different pages, such as Previous and Next buttons.
Previous
12345...
Next
Key Features
Creating forms with Django is a breeze, thanks to its built-in forms framework that allows you to create forms quickly.
Django has a built-in forms framework that allows you to create forms with ease, making it a great choice for building web applications.
To build a comment system, you'll need a comment model to store user comments on posts, a Django form to manage data validation, and a view to process the form and save new comments to the database.
For another approach, see: Twitter Bootstrap Form Example
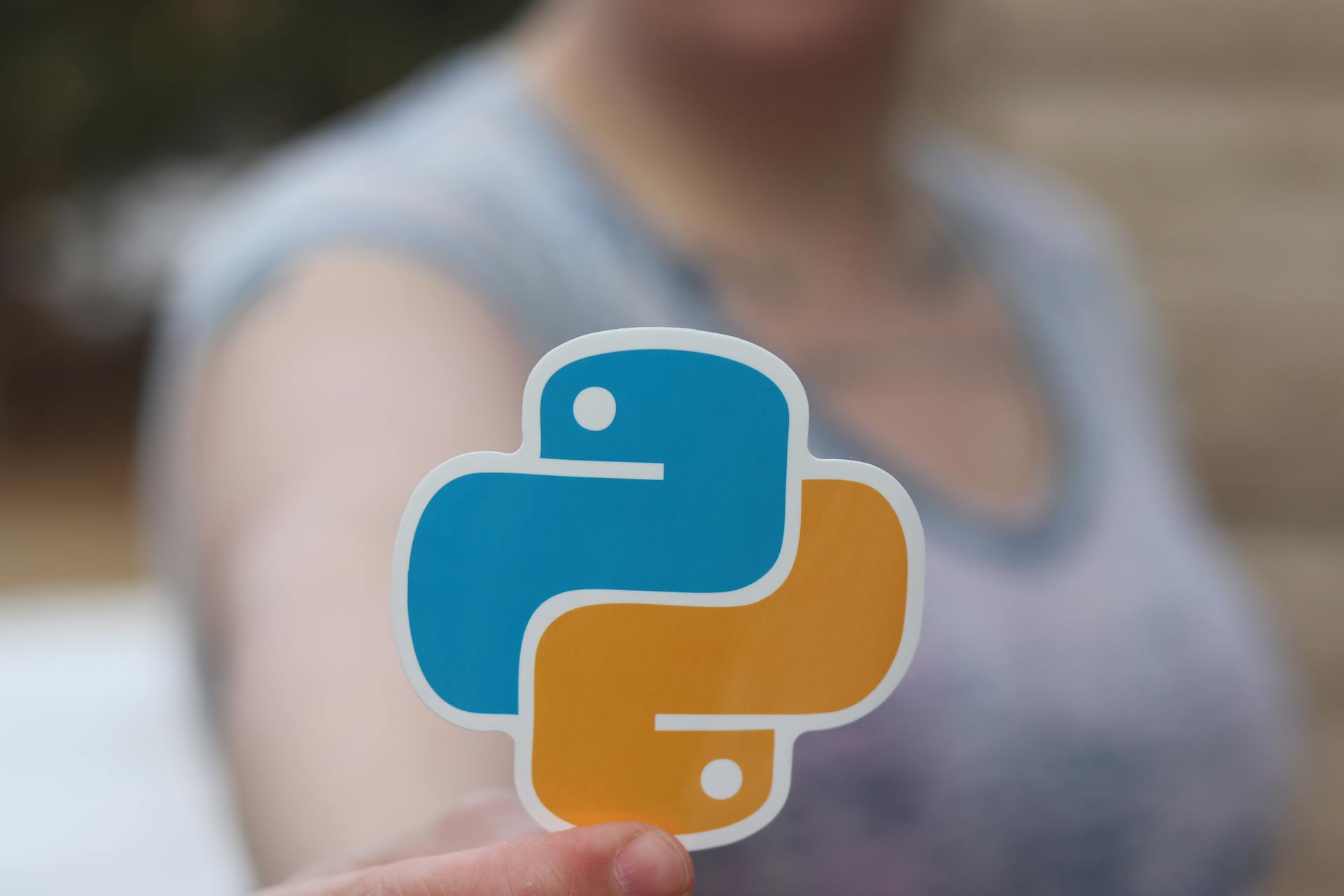
Here are the key features you can expect from a Django project:
- Develop web applications with Python and Django quickly
- Understand Django features with concise explanations and learn how to use them in a practical way
- Create a movie store app with a responsive user interface and deploy it to the cloud
- Purchase of the print or Kindle book includes a free PDF eBook
You'll also learn how to use different modules of the Django framework to solve specific problems, integrate third-party Django applications, and build complex web applications using Redis, Postgres, Celery/RabbitMQ, and Memcached.
Class-Based Views
Class-Based Views are an alternative way to implement views as Python objects instead of functions.
Django provides base view classes that you can use to implement your own views, and all of them inherit from the View class, which handles HTTP method dispatching and other common functionalities.
You can define your views as class methods, making them a great option for organizing code related to HTTP methods, such as GET, POST, or PUT, in separate methods.
This approach eliminates the need for conditional branching, making your code cleaner and more maintainable.
Using multiple inheritance, you can create reusable view classes, allowing you to build more complex and scalable views.
Here are some key benefits of using Class-Based Views:
- Organize code related to HTTP methods in separate methods
- Use multiple inheritance to create reusable view classes
Creating Functionality
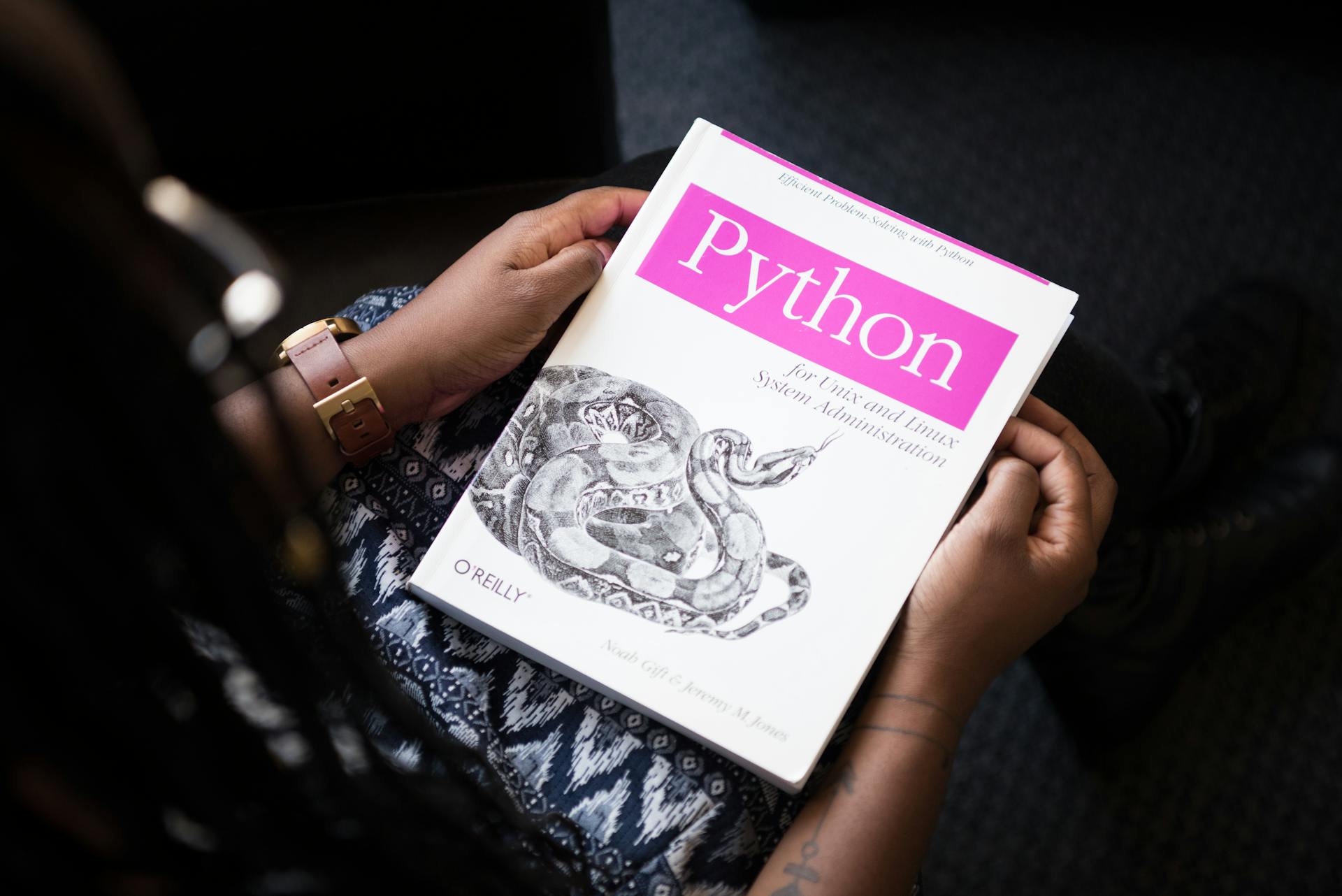
To build a comment system, you'll need to create a comment model to store user comments on posts.
A comment model is used to store user comments on posts, which will be displayed on the post detail page.
A Django form is also required to allow users to submit comments and manage data validation. This form will ensure that users can only submit comments with valid data.
The form will handle data validation, preventing users from submitting comments with invalid information.
A view is necessary to process the form and save a new comment to the database. This view will handle the form submission and save the comment.
To display all comments for a post, you'll need to create a list of comments and an HTML form to add a new comment.
Here's a list of the components needed to build a comment system:
- Comment model to store user comments
- Django form for submitting comments and data validation
- View to process the form and save a new comment
- List of comments and HTML form to add a new comment
Django Projects
The Django Projects section of the book is a highlight of the Django 5 by Example book. It covers four different projects that demonstrate real-world applications of Django.
The book starts with a Blog Application project, which is covered in chapters 1-3. This project helps you create a complete blog application from scratch.
The Social Website project, covered in chapters 4-7, takes it to the next level by allowing you to bookmark and share images. This project is a great way to learn about user authentication and image handling in Django.
Here's a breakdown of the projects:
The Ecommerce Application project, covered in chapters 8-11, helps you create a fully-featured online shop. This project is a great way to learn about payment gateways and order management in Django.
The eLearning Platform project, covered in chapters 12-17, is the most comprehensive project in the book. It helps you create an eLearning platform with a content management system (CMS).
Django Projects
Django Projects offer a comprehensive approach to web app development. The book covers four distinct projects that showcase various aspects of Django development.
The first project, the Blog Application, spans chapters 1-3 and aims to create a complete blog application. This project provides a solid foundation for understanding Django's basics.
The Social Website project, covered in chapters 4-7, focuses on creating a website for bookmarking and sharing images. This project delves into more advanced concepts, such as user authentication and image handling.
The Ecommerce Application project, found in chapters 8-11, creates a fully-featured online shop. This project is a great example of how to implement complex features, like payment gateways and product management.
The eLearning Platform project, covered in chapters 12-17, creates an eLearning platform with a Content Management System (CMS). This project showcases the power of Django for building robust and scalable applications.
Here's a brief overview of the four projects:
- Blog Application: Chapters 1-3
- Social Website: Chapters 4-7
- Ecommerce Application: Chapters 8-11
- eLearning Platform: Chapters 12-17
Requirements
To start a Django project, you'll need to have Python 3.12+ installed on your computer.
Python 3.12+ is the minimum version required to run Django projects, so make sure you have it updated before moving forward.
Django 5 is also a requirement for this project, so ensure you have it installed as well.
Key Concepts
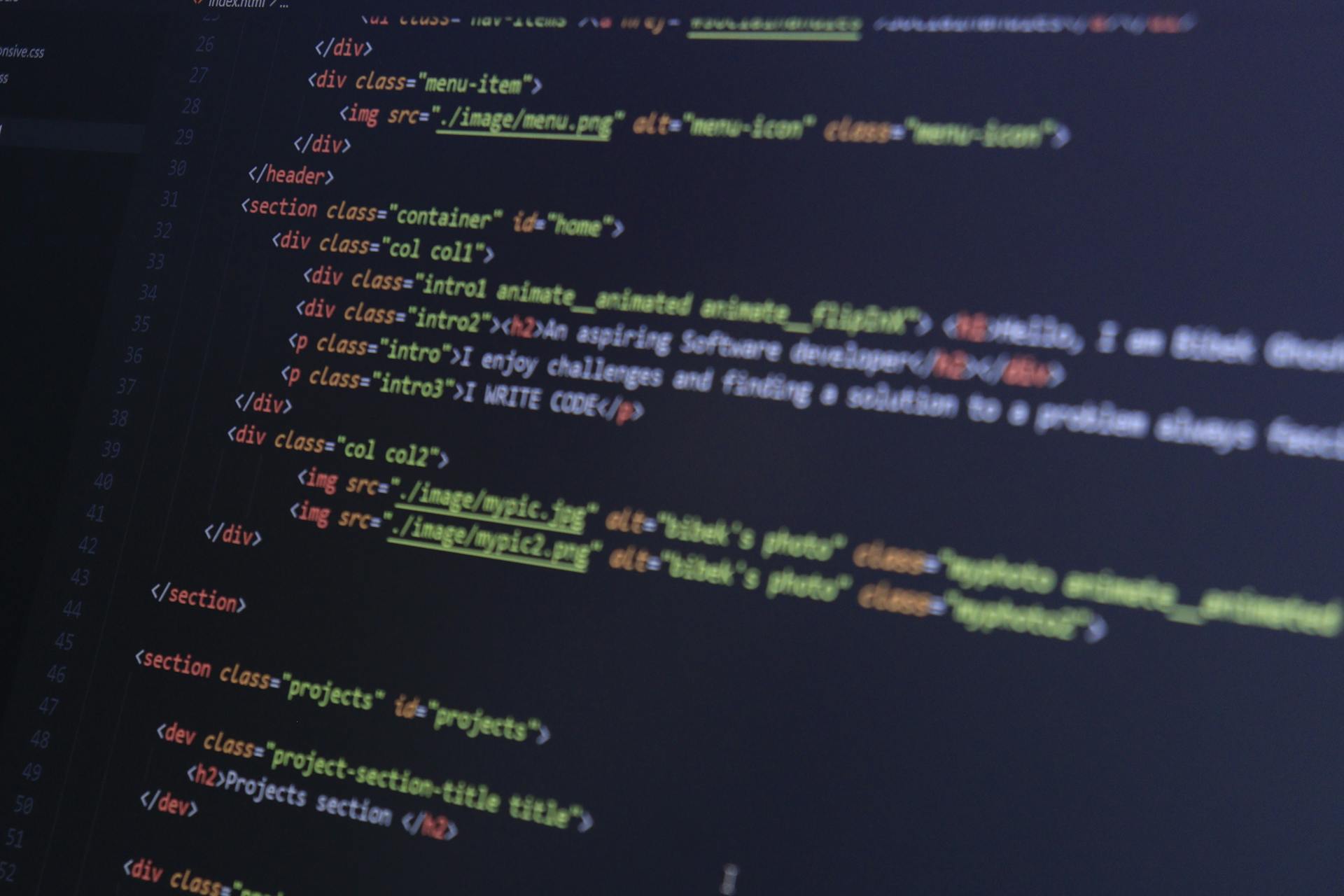
Django 5 by example book covers a wide range of topics, but some key concepts stand out.
You'll learn how to use different modules of the Django framework to solve specific problems, integrate third-party Django applications into your project, and build complex web applications using Redis, Postgres, Celery/RabbitMQ, and Memcached.
Some of the key features of this book include developing web applications with Python and Django quickly, understanding Django features with concise explanations, and creating a movie store app with a responsive user interface and deploying it to the cloud.
Here are some of the key benefits and features you can expect from this book:
- Updated with Django 5 features, detailed app planning, improved tooling, and GPT prompts for extending projects.
- Integrate JavaScript, PostgreSQL, Redis, Celery, Docker, and Memcached into your applications.
- Build a RESTful API with Django Rest Framework (DRF) and implement advanced functionalities like full-text search engines and user activity streams.
Key Benefits
Learning Django can be a game-changer for web development, and understanding its key benefits can help you make the most of it.
Django 5 features, detailed app planning, improved tooling, and GPT prompts are all part of the updated framework, making it easier to extend and customize your projects.
Here are some key benefits of using Django:
- Learn Django essentials, including models, ORM, views, templates, URLs, forms, authentication, signals, and middleware
- Integrate JavaScript, PostgreSQL, Redis, Celery, Docker, and Memcached into your applications
By mastering these aspects, you'll be able to build robust and scalable web applications with ease.
Class-based views offer several advantages over function-based views, including the ability to organize code related to HTTP methods in separate methods, and using multiple inheritance to create reusable view classes.
Book Information
This book is designed to teach you how to use Django in just a few days.
With hundreds of tutorials and loads of documentation out there, it's easy to get overwhelmed and lose sight of what's most important.
The book focuses on a practical and pragmatic approach to learning full-stack development with Django 5.
You'll start building your first Django app within minutes, which is a great way to get hands-on experience.
You'll learn from concise explanations that will help you understand Django features such as URLs, views, templates, models, and more.
The book covers Django MVT (Model-View-Template) architectures and shows you how to implement them.
You'll use Django to develop a movie store application and deploy it to the internet.
By the end of this book, you'll be able to build and deploy your own Django web applications confidently.
Sources
- https://www.packtpub.com/en-us/product/django-5-by-example-9781805125457
- https://github.com/PacktPublishing/Django-5-By-Example
- https://www.paulox.net/2024/06/21/django-5-by-example-preface/
- https://www.abebooks.com/9781805125457/Django-Example-Fifth-Edition-Build-1805125451/plp
- https://www.packtpub.com/en-SG/product/django-5-by-example-9781805125457
- https://www.oreilly.com/library/view/django-5-for/9781835461556/
Featured Images: pexels.com