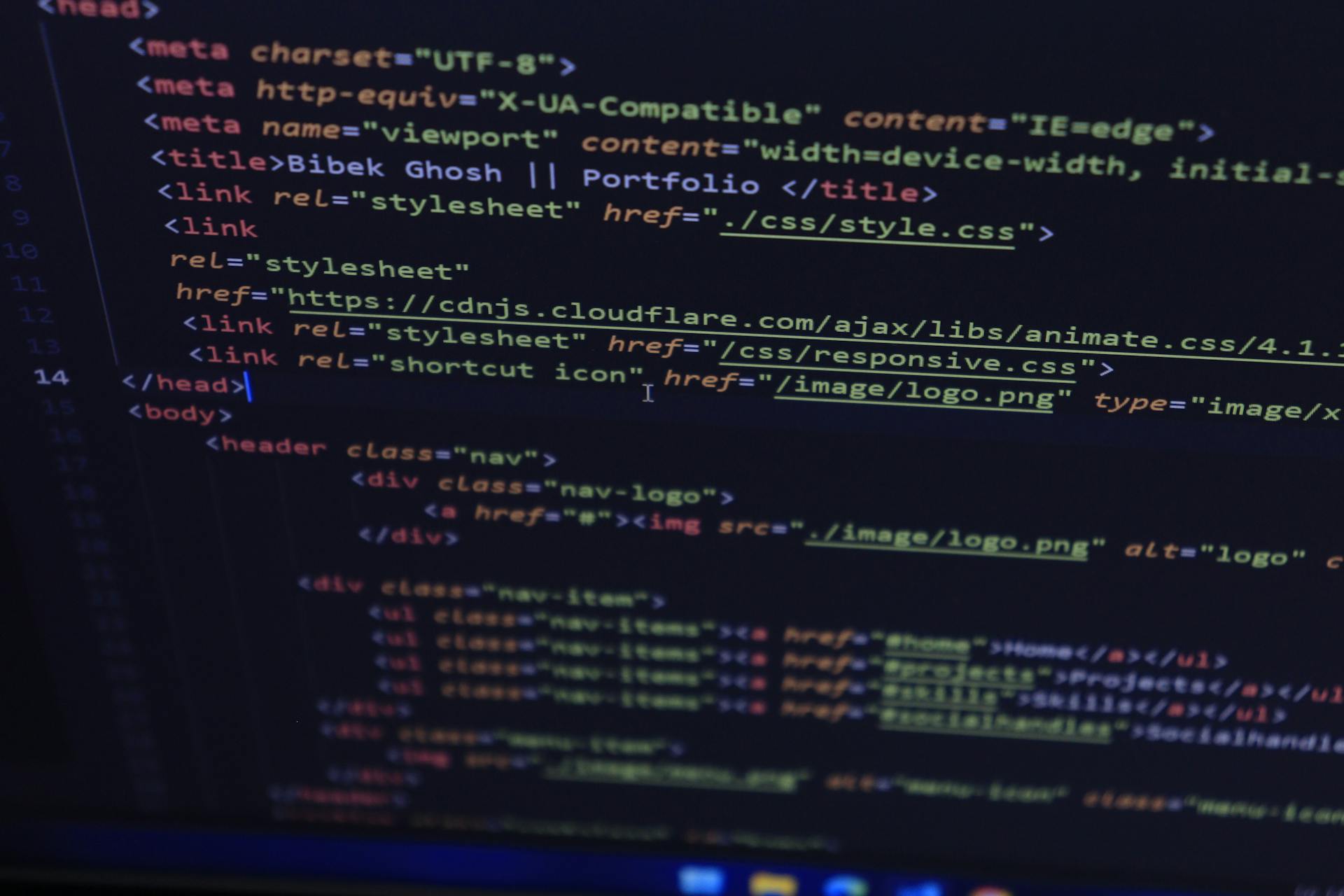
Implementing drag and drop functionality with jQuery UI is a breeze, especially with the right tools and knowledge. The jQuery UI library provides a robust set of widgets and plugins that make it easy to create interactive web applications.
To get started, you'll need to include the jQuery UI CSS and JavaScript files in your HTML document. This can be done by linking to the jQuery UI CDN or by hosting the files locally. The jQuery UI widget factory is also essential for creating custom widgets.
Drag and drop functionality is made possible by the `draggable` and `droppable` widgets in jQuery UI. These widgets can be initialized on elements using the `draggable()` and `droppable()` methods, respectively. The `containment` option is also crucial in defining the boundaries of the drag and drop area.
By following these simple steps, you can create a basic drag and drop interface using jQuery UI. The possibilities are endless, and with a little creativity, you can create complex and interactive web applications that engage your users.
Drag and Drop Basics
Drag and drop is a fundamental interaction in jQuery UI, allowing users to easily move elements around on a page.
To initiate a drag, the user must click and hold on the draggable element, which is typically achieved by setting the `draggable` option to `true`.
The element will then enter a dragging state, and the user can move it around the page by moving the mouse.
In jQuery UI, the `helper` option is used to specify a helper element that is displayed while the user is dragging.
$ Selector Method
The $ selector method is a crucial part of drag and drop functionality, allowing you to select elements to make draggable.
You can use the $ selector method in two forms: $(selector, context).draggable (options) Method and $(selector, context).draggable ("action", [params]) Method.
The first form is used to create a draggable element, while the second form is used to perform an action on the movable elements.
To get started with drag and drop, you'll want to select the elements you want to make draggable. You can do this using the $ selector method, which is used to select elements based on their HTML attributes.
Here are the actions you can perform on the movable elements using the $ selector method:
By using the $ selector method and the actions listed above, you can easily create draggable elements and customize their behavior to suit your needs.
Example
Let's explore some examples that demonstrate the basics of drag and drop functionality.
You can save the code from Example 1 in an HTML file, such as dragexample.htm, and open it in a standard browser that supports JavaScript. The output will show the first element disabled and the second element's dragging enabled.
The code from Example 2 uses jQuery UI Draggable to create a simple draggable element. The HTML code includes a link to the jQuery UI CSS file and a script that initializes the draggable functionality.
The code from Example 3 is similar to Example 2, but it uses an older version of jQuery and jQuery UI. The HTML code includes links to the jQuery 1.10.2 and jQuery UI 1.10.4 files.
To create a draggable element, you'll need to include the jQuery and jQuery UI libraries in your HTML code. The jQuery UI library provides the draggable functionality, which can be initialized using the $( "#draggable" ).draggable() method.
Here are the key elements you'll need to include in your HTML code to create a draggable element:
- A link to the jQuery UI CSS file
- A script that initializes the draggable functionality using the $( "#draggable" ).draggable() method
- A div element with an ID of "draggable" that will be draggable
Scope
The scope of a drag and drop interaction is a crucial aspect to consider, and it's surprisingly simple to set up. The scope option is used to specify the scope of the interaction.
You can initialize the droppable or draggable with the scope option specified, like this: $( ".selector" ).droppable({scope: "tasks"}); or $( ".selector" ).draggable({scope: "tasks"});.
To get or set the scope option, you can use the "option" method, like this: var scope = $( ".selector" ).droppable( "option", "scope" ); or $( ".selector" ).droppable( "option", "scope", "tasks" );.
The scope option is a string value that specifies the scope of the interaction. You can set it to any string value, such as "tasks" or "projects".
Here are some examples of initializing the droppable and draggable with the scope option specified:
Remember, the scope option is a powerful tool that can help you organize and structure your drag and drop interactions.
Heading
Droppable widgets are a great way to create targets for draggable elements. They're easy to use and can be very useful in interactive web applications.
To determine which draggable objects or CSS classes will be accepted by a Droppable object, you can use the accept property. This property is commonly used in Droppable features.
If you drop a draggable object on a Droppable object on the right, the CSS classes and drop function will work as expected. This is a good thing to keep in mind when designing your interactive web application.
You can initialize a draggable element with the scroll option specified, like this: $( ".selector" ).draggable({scroll: false});. This will prevent the draggable element from scrolling when it's dragged.
The scroll option can also be set or gotten after initialization, like this: var scroll = $( ".selector" ).draggable( "option", "scroll" ); or $( ".selector" ).draggable( "option", "scroll", false );.
Here are some common methods for working with the scroll option:
Droppable Options
You can set or get the value of a droppable option using the "option" method. For example, you can check if an option is disabled with `var isDisabled = $( ".selector" ).droppable( "option", "disabled" );`.
The "option" method can also be used to set the value of an option. You can do this by specifying the option name and value, like this: `$( ".selector" ).droppable( "option", "disabled", true );`.
You can also use the "option" method to set multiple options at once by passing an object with the options and values as properties. For example: `var options = $( ".selector" ).droppable( "option" );`.
Accept
The accept option is a crucial part of making your Droppable widget work smoothly. It determines which draggable elements are accepted by the Droppable object.
A selector or a function can be used to specify which draggable elements are accepted. This is where things get interesting, as you can choose to accept specific elements or classes.
To initialize the Droppable widget with the accept option, you can use a selector like ".special". This means that only elements with the class "special" will be accepted by the Droppable object.
Here's a breakdown of the accept option:
- Selector: A selector indicating which draggable elements are accepted.
- Function: A function that will be called for each draggable on the page (passed as the first argument to the function). The function must return true if the draggable should be accepted.
You can also get or set the accept option after initialization using the "option" method. For example, you can get the current accept option like this: var accept = $( ".selector" ).droppable( "option", "accept" );.
Append To
The appendTo option is a crucial setting for the Droppable widget, allowing you to specify where the draggable helper should be appended to while dragging.
You can initialize the draggable with the appendTo option specified, like this: $( ".selector" ).draggable({appendTo: "body"});. This will append the helper to the specified element, which in this case is the body of the HTML document.
The appendTo option can also be set to a jQuery object, an element, a selector, or even the string "parent", which will cause the helper to be a sibling of the draggable.
Here are the possible values for the appendTo option:
- jQuery: A jQuery object containing the element to append the helper to.
- Element: The element to append the helper to.
- Selector: A selector specifying which element to append the helper to.
- String: The string "parent" will cause the helper to be a sibling of the draggable.
To get or set the appendTo option after initialization, you can use the "option" method, like this: $( ".selector" ).draggable( "option", "appendTo" ); or $( ".selector" ).draggable( "option", "appendTo", "body" );.
Disable Returns
Disable Returns is a crucial aspect of Droppable Options. The disable() method returns jQuery (plugin only).
Invoking the disable method is straightforward. You can do it by calling $( ".selector" ).draggable( "disable" );.
If you're working with a plugin, you'll notice that the disable method returns the jQuery object itself. This is useful for chaining method calls.
Droppable Events
Droppable events are a crucial part of creating interactive drag and drop experiences. They allow you to specify actions that should be taken when a draggable element is dropped onto a droppable element.
There are several types of droppable events, including dropactivate, dropdeactivate, drop, dropout, and dropover. Each of these events can be bound to a specific callback function using the jQuery UI API.
Here are the different types of droppable events and how to bind them:
Drop Event
The drop event is a crucial part of the droppable functionality in jQuery UI. It's triggered when an item is dropped onto a droppable element.
Let's take a look at the different types of drop events. You can initialize the droppable with the drop callback specified, like this: $( ".selector" ).droppable({drop: function( event, ui ) {}});. This allows you to perform actions when an item is dropped.
Alternatively, you can bind an event listener to the drop event using the on() method: $( ".selector" ).on( "drop", function( event, ui ) {} );.
Here's a summary of the drop event:
Remember, the drop event is a powerful tool for creating interactive and user-friendly interfaces.
Delay
Delay is a crucial option when it comes to making your draggable elements more user-friendly. It allows you to specify a delay period after a user starts dragging an element before it actually starts moving.
You can initialize the draggable with the delay option specified, like this: $( ".selector" ).draggable({delay: 300});. This sets the delay to 300 milliseconds.
The delay option can be retrieved or set after initialization using the draggable method, like this: var delay = $( ".selector" ).draggable( "option", "delay" ); or $( ".selector" ).draggable( "option", "delay", 300 );.
In practice, this means that if you set the delay to 300 milliseconds, the user will have to hold the mouse button for 300 milliseconds before the element starts moving. This can help prevent accidental dragging of elements.
Here's a quick recap of how to work with the delay option:
Droppable Features
The Droppable feature in jQuery UI is a powerful tool for creating interactive interfaces. It allows you to create targets for draggable elements, starting with version 1.0.
The accept property is a commonly used feature of Droppable, used to determine which draggable objects or CSS classes will be accepted by the Droppable object. This is crucial for making sure that the right elements can be dropped in the right places.
Theming
Theming is a crucial aspect of creating visually appealing droppable widgets. You can use the jQuery UI CSS framework to style its look and feel.
To customize the look and feel of your droppable widget, you can use the following CSS class names for overrides or as keys for the classes option: ui-droppable, ui-droppable-active, and ui-droppable-hover. These classes are added to the droppable element when a draggable is activated, dragging over, or not.
The ui-droppable class is the base class for the droppable element. When a draggable can be dropped on this droppable, the ui-droppable-active class is added, and when dragging a draggable over this droppable, the ui-droppable-hover class is added.
Here's a quick reference to the droppable CSS class names:
- ui-droppable: The droppable element.
- ui-droppable-active: Added when a draggable is activated.
- ui-droppable-hover: Added when dragging a draggable over the droppable.
Z-Index
The z-index option is a crucial feature of droppable elements. It determines the stacking order of elements when they overlap.
To set the z-index option when initializing a draggable element, you can use the zIndex property in the draggable method. For example, $( ".selector" ).draggable({zIndex: 100});.
You can also get or set the z-index option after initialization using the draggable method's option method. To get the current z-index, use $( ".selector" ).draggable( "option", "zIndex" );. To set a new z-index, use $( ".selector" ).draggable( "option", "zIndex", 100 );.
The z-index option is useful for ensuring that your draggable elements are displayed on top of other elements when they overlap.
Features
You can define cursor properties for the draggable object to determine the shape and position on the screen when you start dragging and holding the object on the object.
The cursor-at property specifies the position of the mouse object when we start dragging.
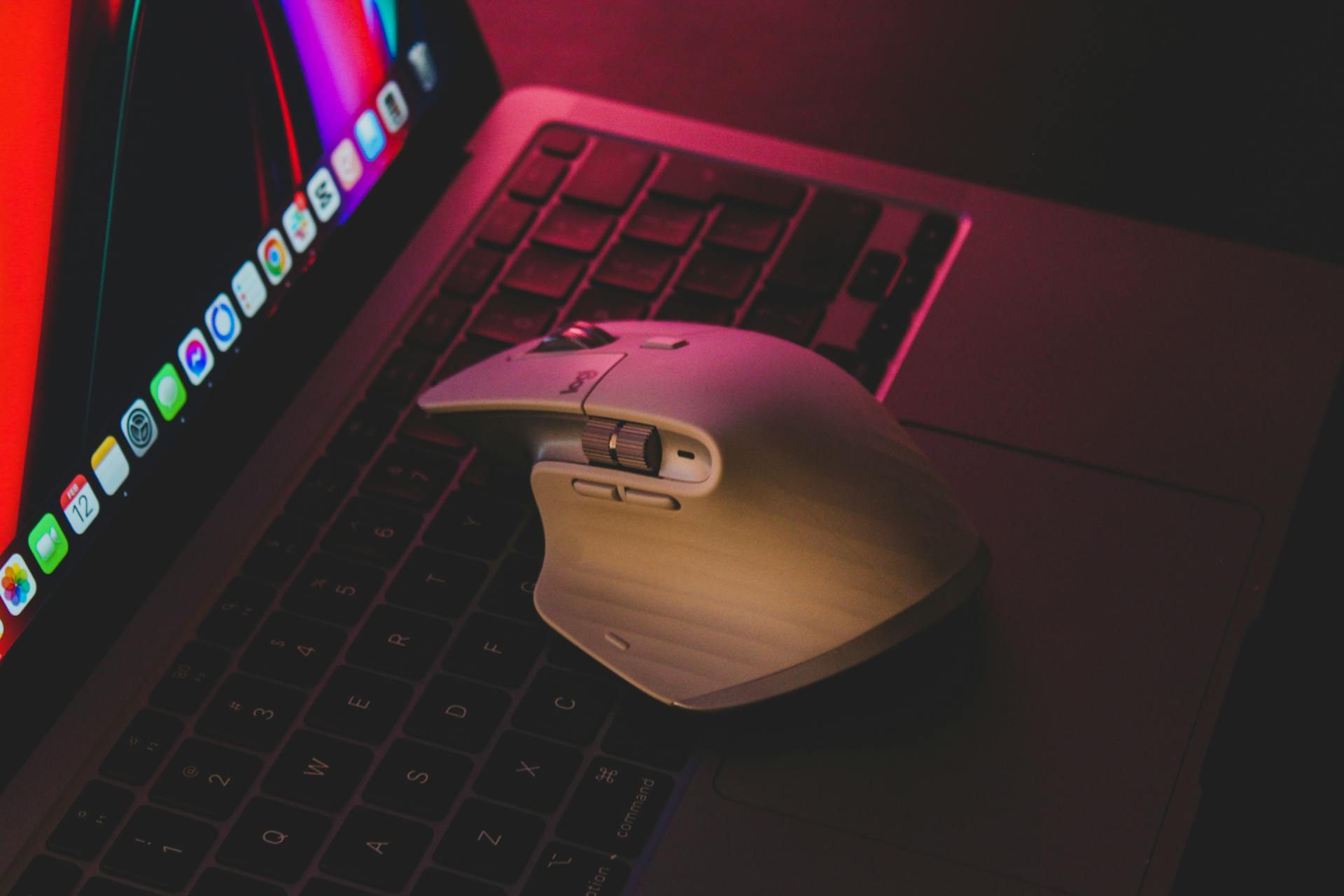
Auto Scroll feature allows you to give true to the scroll variable while dragging the HTML object to win scrollability in the page or another HTML object it is in.
Adjustments like scroll speed and precision can be made with the Auto Scroll feature.
The Axis property is used to move the draggable HTML object in only one direction, either x or y.
Containment allows the draggable HTML object to be draggable only within the container object.
The Stop function allows you to leave the draggable object and run it for a while, it will not work until you drag and drop it again.
Droppable Features
The Droppable feature is a powerful tool for creating interactive web applications. It allows you to create targets for draggable elements.
One of the most commonly used properties in Droppable is the accept property. It determines which draggable objects or CSS classes will be accepted by the Droppable object.
If you drop a draggable object on a Droppable object on the right, the CSS classes and drop function will work as expected.
The Droppable feature version added was 1.0, which introduced the ability to create targets for draggable elements.
Frequently Asked Questions
How to make drag and drop in jQuery?
To enable drag and drop functionality, use the jQuery UI draggable() method to make any DOM element movable. This allows users to click and drag the element within the viewport.
How does jQuery sortable work?
jQuery sortable allows you to drag and drop elements within a list, automatically rearranging the other items to fit. Learn how to customize and optimize this interactive feature for your web application.
Featured Images: pexels.com