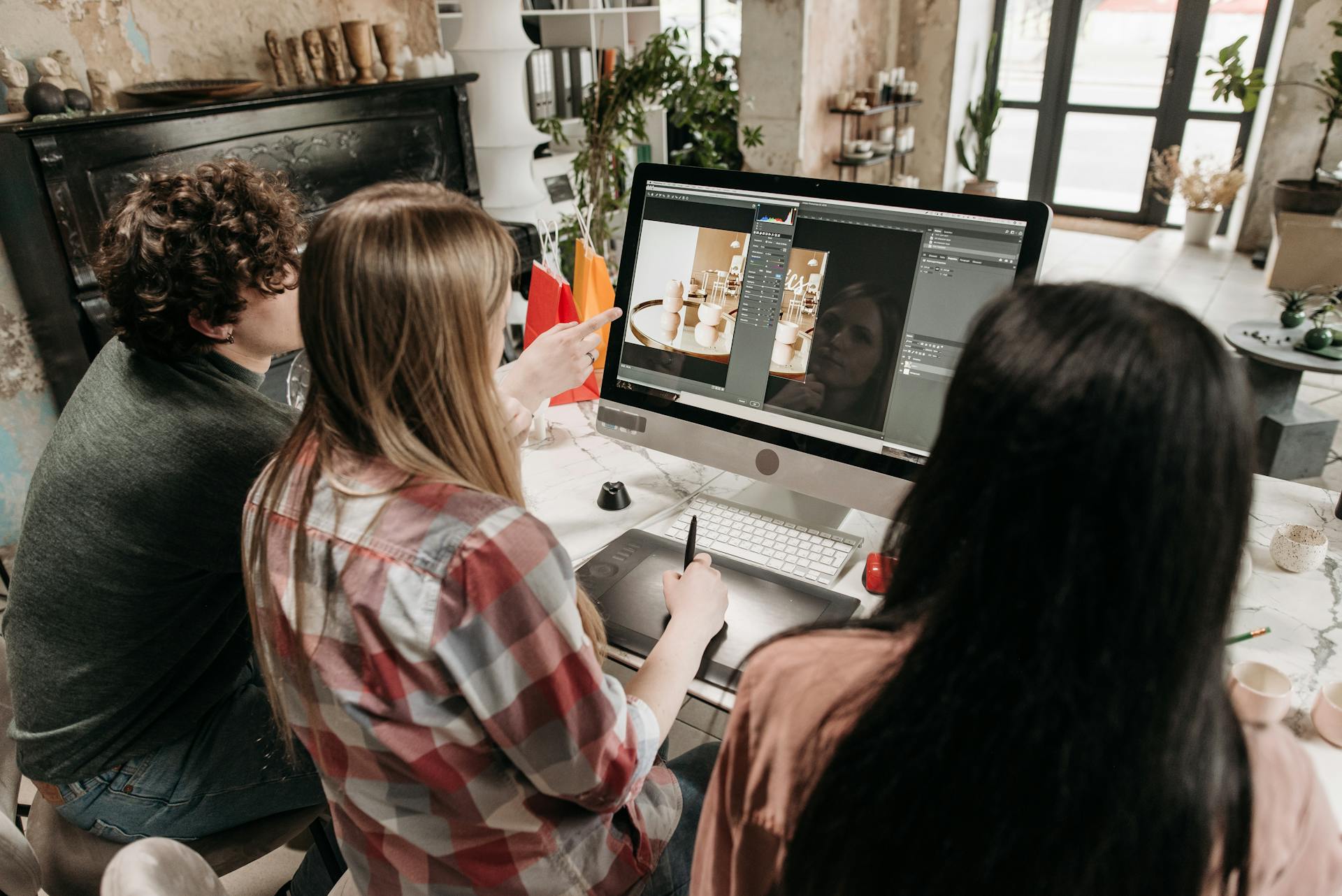
Implementing HTML drag and drop file upload can be a game-changer for user experience, especially for file-intensive applications.
To get started, you'll need to add the drag and drop functionality to your HTML file. This can be achieved using the HTML5 drag and drop API, which allows users to drag files from their desktop or cloud storage directly into your application.
The HTML5 drag and drop API is supported by most modern browsers, making it a reliable choice for implementing drag and drop functionality. This means you can focus on writing the JavaScript code to handle the file uploads without worrying about compatibility issues.
With the drag and drop functionality in place, users can now easily upload files by dragging and dropping them into your application. The next step is to write the JavaScript code to handle the file uploads, which we'll cover in the next section.
Intriguing read: Html Code for Download Pdf File
Implementing Website Functionality
Implementing website functionality for drag-and-drop file uploads involves several key steps. You start by learning the HTML5 Drag and Drop API, which allows users to drag items like files and drop them into a specific area on your web page.
You might enjoy: Drag and Drop Web Page Design
To create a drop zone, use a simple HTML container and handle file drop events with JavaScript by adding event listeners. This will enable basic drag-and-drop file functionality on your site. For example, you can use the following HTML code snippet:
- Create an intuitive drop interface to make file uploads easy.
- Use clear instructions and well-marked drop areas.
- Provide visual feedback during file drag operations so users know when they can start dropping files.
- When the user drops a file, the interface should respond immediately.
- It’s also important to add a fallback option for users who can’t use drag-and-drop. A simple file upload button works well.
To add visual feedback, you can use CSS to change the drop area's look when a dragged file is over it. For example, the following CSS code snippet changes the drop area's look when a dragged file is over it:
This helps improve the user experience.
Optimizing for Performance
Optimizing file uploads for performance is crucial, especially for large files. One effective way is to use chunked uploads, which break large files into smaller parts and upload each part separately.
Using Web Workers is another useful technique. Web Workers run JavaScript in the background, keeping the main thread free and ensuring the user interface remains responsive, even during heavy tasks.
Compressing images on the client side before uploading is also key. Smaller files upload faster and use less bandwidth.
To ensure consistency, make sure your site's body tag has the appropriate font family set.
Here's an interesting read: Upload Large Files to Google Drive
Adding the Functionality
Implementing drag-and-drop functionality in a website is a great way to make file uploads easy and intuitive for users. To start, you'll need to learn the HTML5 Drag and Drop API, which lets users drag items like files and drop them into a specific area on your web page.
Create a drop zone using a simple HTML container in your HTML file, and handle file drop events with JavaScript by adding event listeners. This will enable basic drag-and-drop file functionality on your site.
A well-designed drop interface is essential for making file uploads easy. To create one, use clear instructions and well-marked drop areas, and provide visual feedback during file drag operations.
Here are some key steps to follow when implementing drag-and-drop functionality:
- Create an intuitive drop interface to make file uploads easy.
- Use clear instructions and well-marked drop areas.
- Provide visual feedback during file drag operations.
- When the user drops a file, the interface should respond immediately.
- Add a fallback option for users who can’t use drag-and-drop.
To add visual feedback to your drop area, you can use CSS to change the look of the area when a file is dragged over it. This will help improve the user experience.
To implement the drag-and-drop functionality, you'll need to add a script to your page that references the drop area and attaches events to it. This will prevent default behaviors and stop the events from bubbling up any higher than necessary.
You'll also need to add an indicator to let the user know that they have indeed dragged the item over the correct area by using CSS to change the border color of the drop area. This will be done by adding and removing the #drop-area.highlight class when necessary.
When the user drops a file, you'll need to figure out what to do with it. To do this, you'll need to get the data for the files that were dropped and convert the FileList to an array so you can iterate over it more easily.
Here's a basic outline of the steps involved in handling dropped files:
- Get the data for the files that were dropped.
- Convert the FileList to an array.
- Use FormData to create form data to send to the server.
- Use the fetch API to send the image to the server.
Alternatively, if you want to support Internet Explorer, you may want to use XMLHttpRequest instead of the fetch API.
Ensuring Compatibility
Testing your site across various browsers is crucial to ensure everything works smoothly, especially when it comes to drag-and-drop file uploaders.
Different browsers handle code in different ways, so use feature detection and polyfills if needed to keep things consistent.
Optimizing for mobile devices is also important, as many users access web applications from their phones. To support mobile drag-and-drop, add touch events to your code, as touch interactions are different from mouse interactions.
Consider using the border-radius and margin-bottom CSS properties to enhance the look and spacing of your file uploader.
You might enjoy: How to Run an Html File in vs Code
Cross-Browser Support
Cross-Browser Support is crucial for a seamless user experience. Different browsers handle code in different ways, so it's essential to test your site across various browsers to ensure everything works smoothly.
Feature detection and polyfills can be used to keep things consistent. This is especially important for modern browsers that support advanced features.
To support mobile devices, you should add touch events to your site. This is necessary because touch interactions are different from mouse interactions.
Readers also liked: How to Transfer Webflow Site to Another Account
Here's a simple code snippet for mobile-friendly drag-and-drop:
```html
// Add touch events to support mobile devices
```
Optimizing for mobile devices is also crucial. Many users access web applications from their phones, and you want to make sure your site looks good and works well on smaller screens.
You should also consider the border-radius and margin-bottom CSS properties to enhance the look and spacing of your site. Using the form tag properly in your HTML source code can also ensure smooth integration.
To distinguish which Ajax method will work on different browsers, you can use your existing isAdvancedUpload test. This can help you determine whether to use XMLHttpRequest or a different Ajax technique.
Recommended read: No Code Html Editor
Legacy Browser Support
Legacy browsers can be a challenge to support, but there are workarounds. Ajax for legacy browsers is a solution for older browsers like IE 9-. We don't need to collect dragged & dropped files because these browsers don't support drag & drop file upload.
In some cases, targeting the form on a dynamically inserted iframe can help. This is exactly what works for IE 9-. The browser's limitations can be overcome with a little creativity.
For example, if we're using Ajax for legacy browsers, we can forget about collecting dragged & dropped files. The browser just won't support it, so we might as well move on.
Web Form and Upload Process
A web form with drag and drop file upload is made possible by including the DropzoneJS library files. This library enables the drag and drop file upload functionality.
To create a web form with drag and drop file upload, you need to include an HTML form with enctype="multipart/form-data". This is crucial for handling file uploads.
The following JavaScript code is used to attach the drag and drop file upload feature to the webform and process the form submission with multiple files. Initialize the Dropzone object and define configuration options, disable auto queue process in Dropzone, and start Dropzone queue process on button click.
Take a look at this: Drag and Drop Html Editor
To process form submission with multiple files, use the sendingmultiple method with the FormData object to combine form data with files. This method is essential for handling multiple file uploads.
Here are the essential APIs for handling drag and drop file uploads:
- Drag and Drop API
- FormData API
- XHR progress event
- FileReader API
These APIs work together to capture the drop event, read its data, post that binary data to the server, provide feedback on the progress of the upload, and optionally render a preview of what's being uploaded and its status.
Broaden your view: Data Text Html Base64
File Upload Implementation
To implement drag-and-drop file upload functionality on your website, you need to start by learning the HTML5 Drag and Drop API. This API lets users drag files and drop them into a specific area on your web page.
First, create a drop zone using a simple HTML container in your HTML file. Then, handle file drop events with JavaScript by adding event listeners. This lets the user drop files into the designated area, enabling basic drag-and-drop file functionality on your site.
A unique perspective: Drag and Drop Jquery Ui
To make file uploads easy, create an intuitive drop interface with clear instructions and well-marked drop areas. This is crucial for a seamless user experience.
Here are some best practices to keep in mind:
- Create an intuitive drop interface to make file uploads easy.
- Use clear instructions and well-marked drop areas.
- Provide visual feedback during file drag operations so users know when they can start dropping files.
- When the user drops a file, the interface should respond immediately.
- It’s also important to add a fallback option for users who can’t use drag-and-drop. A simple file upload button works well.
For file uploads, you'll need to use the FileReader API to read files on the client side. This allows you to show file previews or take other actions before sending them to the server, providing instant feedback and improving the user experience.
AJAX is also essential for uploading files, as it allows file uploads without reloading the page. This makes the process smoother, especially for large files. Adding a progress bar is also important, as it shows users the status of their upload and reduces frustration.
Here's a simple code snippet for AJAX file upload with a progress bar:
This code snippet shows how to handle the drop event and upload files using AJAX. It also displays the upload progress. When integrating this into your project, make sure the drop area is clearly defined in the form body tags, making drop interfaces more intuitive and user-friendly.
Ajax Upload
Ajax Upload is a crucial aspect of HTML drag and drop file upload. It allows file uploads without reloading the page, making the process smoother, especially for large files. This is essential for providing instant feedback and improving the user experience.
Some browsers, like IE and Firefox, don't allow setting the value of a file input, which can be submitted to the server in a usual way. Instead, we'll use Ajax when the form is submitted.
To prevent the form from being submitted repeatedly and indicate to the user that the submission is in progress, we can use a class like .is-uploading. This class can be used to prevent the form from submitting repeatedly and provide visual feedback to the user.
For another approach, see: Contact Form Webflow
In modern browsers like IE 10 and above, we can use XMLHttpRequest to upload files via Ajax. To distinguish which Ajax method will work, we can use a test like isAdvancedUpload. This test can help determine whether to use the XMLHttpRequest method or a different Ajax technique.
Here's a list of the key steps involved in using Ajax for file uploads:
- Collect data from all form inputs using FormData($form.get(0))
- Run through the dragged & dropped files using a loop and append them to the data stack with ajaxData.append()
- Submit the data via Ajax and handle the response in the data.success and data.error callbacks
These steps can be used to implement Ajax file uploads in HTML drag and drop file upload scenarios, providing a smoother and more user-friendly experience.
Frequently Asked Questions
How to enable drag and drop in HTML?
To enable drag and drop in HTML, simply set the "draggable" attribute to "true" on the desired element. This allows a wide range of elements, including images and links, to be drag-enabled with minimal CSS adjustments.
How to upload a file in HTML?
To upload a file in HTML, use the element, which creates a button that allows users to securely select files from their device's storage. This simple yet powerful element is the foundation of file uploading in HTML.
Sources
- https://blog.filestack.com/drag-and-drop-file-upload-site/
- https://www.codexworld.com/create-web-form-with-drag-and-drop-file-upload-using-javascript-php/
- http://html5doctor.com/drag-and-drop-to-server/
- https://css-tricks.com/drag-and-drop-file-uploading/
- https://www.smashingmagazine.com/2018/01/drag-drop-file-uploader-vanilla-js/
Featured Images: pexels.com