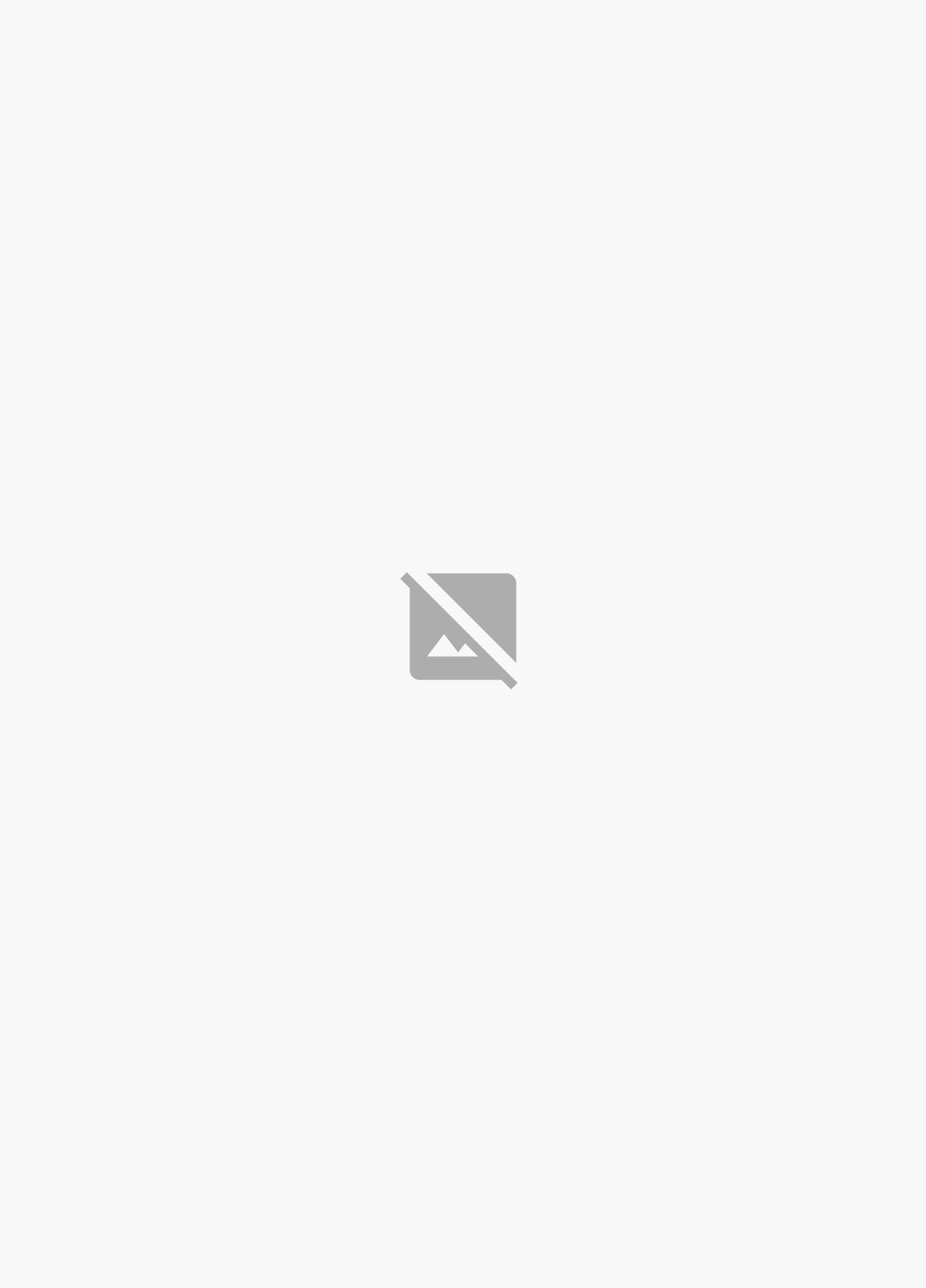
Setting the image source with jQuery is a straightforward process. You can use the attr() method to change the src attribute of an image.
To use the attr() method, you simply need to pass the name of the attribute you want to change, in this case 'src', and the new value. The syntax is $(selector).attr('src', 'new_src_value');.
The attr() method can also be used to get the current value of an attribute, which can be useful when you need to know the current image source. For example, $(selector).attr('src') will return the current src attribute value.
Recommended read: How to Change Browser Settings to Not Allow Tracking
Setting Image Source with Attr()
To set the image source with the attr() method in jQuery, you simply need to specify the name of the attribute and its new value as parameters. The attr() method takes two parameters, the attribute name and the new value, and assigns the new value to the specified attribute.
You can use the attr() method to change the src attribute of an img element, which is a common use case. To do this, you'll need to add the jQuery link to the head of your document, add an img element with a src attribute, and a button element with an onclick event.
You might enjoy: How to Add Img to Nextjs
Here's a step-by-step guide to setting the image source with the attr() method:
1. Add the jQuery link to the head of your document.
2. Add an img element with a src attribute.
3. Add a button element with an onclick event.
4. Use the attr() method to change the src attribute of the img element.
For example, if you want to change the src attribute of an img element to 'new-image.jpg', you would use the following code: `$('img').attr('src', 'new-image.jpg');`
This will change the src attribute of all img elements on the page to 'new-image.jpg'. If you want to change the src attribute of a specific img element, you can use a more specific selector, such as `$('#my-image').attr('src', 'new-image.jpg');`
Expand your knowledge: Change Img Src with Javascript
Setting Image Source with Prop()
The prop() method in jQuery allows you to change the value of any attribute, including the src attribute of an image.
You can use the prop() method to change the value of a particular attribute, such as the src attribute of an image, using the following syntax: prop(propertyName, newValue).
The prop() method can be used to change the value of multiple attributes at the same time, making it a convenient option when working with multiple elements.
Here's a simple example of how to use the prop() method to change the src attribute of an image:
The algorithm for using the prop() method is similar to the algorithm for using the attr() method, but with the prop() method, you just need to replace the attr() method with the prop() method to get the work done.
The prop() method can be used to change the value of the src attribute of an image in an HTML document, as shown in the following example:
The code example above demonstrates how to use the prop() method to change the src attribute of an image in an HTML document.
If this caught your attention, see: Image Html Coding
Sources
- https://www.tutorialspoint.com/how-to-change-the-src-attribute-of-an-img-element-in-javascript-jquery
- https://fancyapps.com/fancybox/getting-started/
- https://developer.mozilla.org/en-US/docs/Web/API/File_API/Using_files_from_web_applications
- https://lokeshdhakar.com/projects/lightbox2/
- https://dev.to/santiagocodes/replacing-empty-img-src-attributes-using-javascript-2pc8
Featured Images: pexels.com