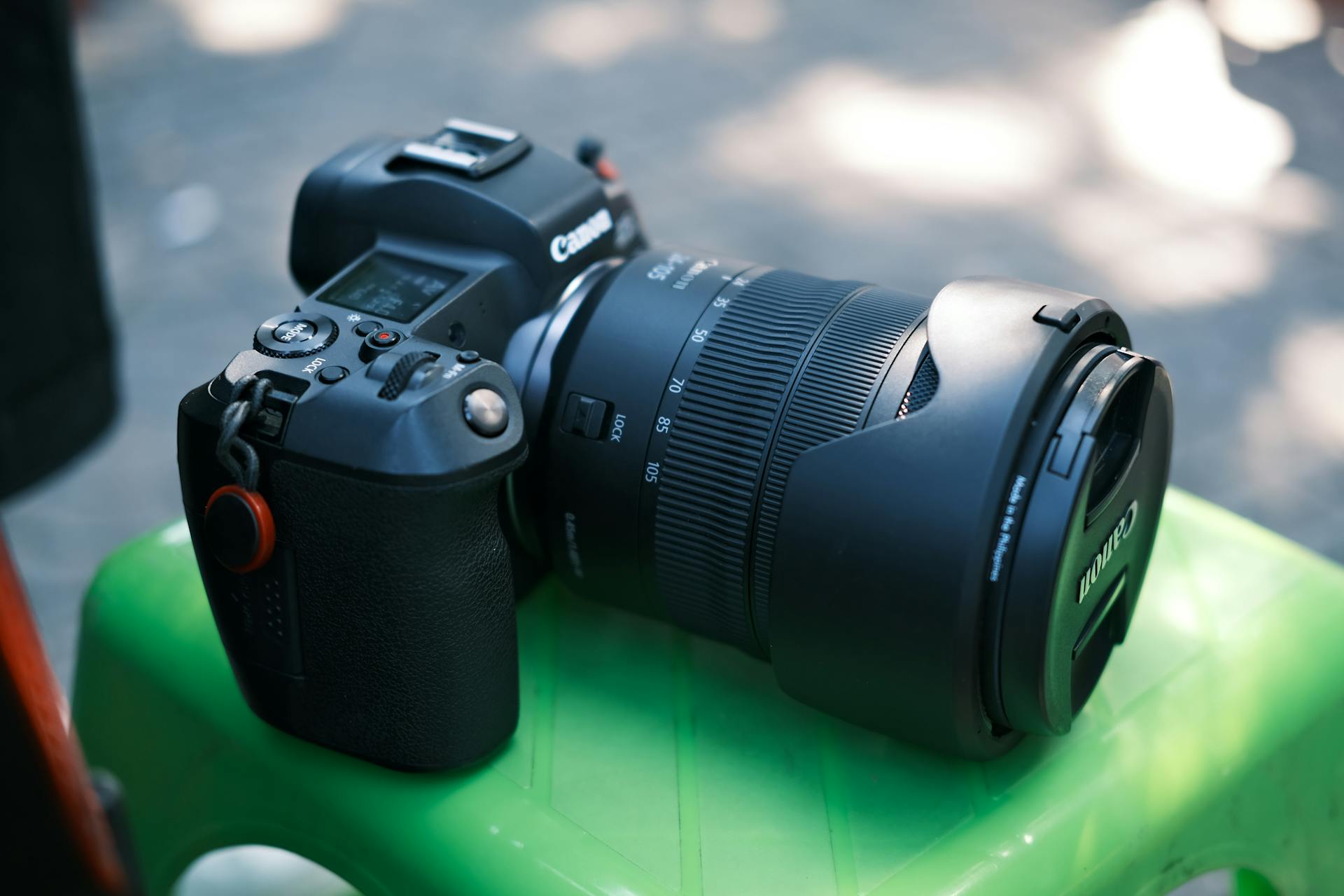
When using JavaScript to dynamically load images, it's essential to follow best practices to ensure efficient and secure image loading.
Using the `src` attribute to set the image source is the most straightforward method, as it allows you to load the image directly without any intermediate steps.
However, this approach can be problematic if the image source is not available, leading to a failed image load.
To mitigate this issue, you can use the `srcset` attribute, which provides a list of alternative image sources that can be used if the primary source is not available.
Broaden your view: Img Src Not Working
Changing Image Source
Changing Image Source is a fundamental technique for creating interactive and dynamic web pages.
You can change the src attribute of an image using the getElementById() or querySelector() methods to access the image element and then update its src attribute.
To change the src attribute, you can use the src property on the element, which is a common method of changing the value of the src attribute of an img element.
Consider reading: Change Css Class in Javascript
You can target each image element separately using ID or class attributes, or use querySelectorAll() to get a reference to all image elements.
Here are some common methods of changing the src attribute of an img element:
By using these methods, you can effectively manipulate images based on user interactions or other conditions, enhancing the user experience.
JavaScript can interact with user events to change image sources dynamically, making it a powerful tool for creating interactive web pages.
You can change the src attribute of an image using the following code: `document.getElementById('image').src = '../template/save.png';`
This code uses the getElementById() method to access the image element and then updates its src attribute using the src property.
You might enjoy: Change Img Src with Javascript
Handling Errors and URLs
Handling errors with JavaScript can be frustrating, especially when it comes to image loading. An empty alt attribute can cause errors, as shown in the example where the alt attribute is left blank.
It's essential to check for missing or incorrect URLs, like the example where the src attribute is set to a non-existent image file. This can prevent errors and ensure smooth image loading.
A well-formed URL is crucial, and using a protocol-relative URL can help avoid issues, as demonstrated in the example where the URL starts with '//'.
Handling Errors in Image Src Changes
Handling errors in image src changes is crucial to ensure a smooth user experience. You can handle errors by setting an onerror event handler to provide a fallback image if the new src fails to load.
To create a fallback image, you can set the onerror event handler on the image element before changing its src attribute. This way, if the new src fails to load, the fallback image will be displayed instead.
If you're creating an image element using the createElement() method or the Image() constructor, you can set the onerror event handler immediately after creating or initializing the image element.
Consider reading: Jquery Set Img Src
Handling URLs Types
Handling URLs Types can be a bit tricky, but it's essential to understand the basics. You can use either relative or absolute URLs when changing the src attribute.
Relative URLs are convenient because they're easy to implement, but they can be problematic if the URL structure changes. For example, if you're using a relative URL, it might break if the URL structure changes.
Absolute URLs, on the other hand, are more reliable because they specify the full URL, including the protocol and domain. This makes them less prone to breaking if the URL structure changes.
Remember, using absolute URLs can provide more control and stability, especially in complex web applications.
Updating Image Sources
Updating Image Sources is a fundamental technique for creating interactive and dynamic web pages, and it's a great way to enhance the user experience.
You can change the src attribute of an image using the getElementById() or querySelector() methods to access the image element and then update its src attribute.
Here are some common methods for updating image sources dynamically:
- javascript
- html
- image
- src
By using methods like getElementById(), querySelector(), and event listeners, you can effectively manipulate images based on user interactions or other conditions.
Changing the image src in JavaScript is a fundamental technique for creating interactive and dynamic web pages.
You can change the src attribute of an image in JavaScript by inserting the updated src inside the edit() function, like this: "../template/save.png".
To avoid having to click the image twice, make sure to update the src attribute directly in the edit() function, without any extra steps.
Updating image sources dynamically can be achieved by interacting with user events, such as onclick, to change the image sources based on user interactions or other conditions.
A fresh viewpoint: Free Simple Html Website Templates
Setting Attributes
You can change the src attribute of an image in JavaScript using the getElementById() or querySelector() methods to access the image element and then update its src attribute.
To get a reference to the image element, you can use the querySelector() method, which works fine for a single image element.
You can also use querySelectorAll() to get a reference to all image elements, creating a Node List Array from them.
To update the src attribute, simply set the src property on the element, or use the square brackets syntax like this: element.src = 'new image URL'.
The src property in JavaScript allows you to get the already assigned value to it as well as to update or change the value of the same attribute, making it a very common method of changing the value of the src attribute of an img element.
Readers also liked: Add Css Property to a Predefined Class Javascript
Creating and Setting Image Element
Creating an image element in JavaScript is a straightforward process. You can use the createElement() method on the document object to create an image element.
On a similar theme: Inspect Element Javascript
To set an image URL, simply assign it to the src attribute of the image element. This will load the image from the specified URL.
Alternatively, you can use the Image() constructor, which creates a new HTMLImageElement instance. This is functionally equivalent to using document.createElement("img").
You can also specify the width and height of the image when creating it with the Image() constructor. This will set the initial dimensions of the image element.
Adding the image element to the DOM hierarchy is as simple as appending it to the body element. This will make the image visible on the page.
JavaScript Properties
You can change the src attribute of an image using the getElementById() or querySelector() methods to access the image element and then update its src attribute.
To get a reference to all image elements, you'll need to use querySelectorAll(), which creates a Node List Array from them.
The src property in JavaScript allows you to get the already assigned value to it, as well as to update or change the value of the same attribute.
Using the src property is a very common method of changing the value of the src attribute of an img element.
You can easily target each image element separately to set a different value to the src attribute using the ID or class attribute.
Frequently Asked Questions
How to select img src in JavaScript?
To select an image source in JavaScript, use the `getElementById()` method to target the image element by its ID, then update the `src` attribute with the new image URL. This simple method allows you to dynamically change the image displayed on your webpage.
What is src in JavaScript?
The src attribute in HTML specifies the location of an external resource, often used in conjunction with JavaScript to load external files. It's a fundamental concept in web development, and understanding its purpose is key to building dynamic web applications.
Sources
- https://stackoverflow.com/questions/11722400/programmatically-change-the-src-of-an-img-tag
- https://medium.com/@ryan_forrester_/how-to-change-image-src-in-javascript-86c49cf96f7d
- https://www.shecodes.io/athena/8867-how-to-change-image-source-using-javascript
- https://www.basedash.com/blog/how-to-change-an-image-src-with-javascript
- https://dev.to/rajatamil/create-image-elements-in-javascript-41ke
- https://www.tutorialspoint.com/how-to-change-the-src-attribute-of-an-img-element-in-javascript-jquery
Featured Images: pexels.com