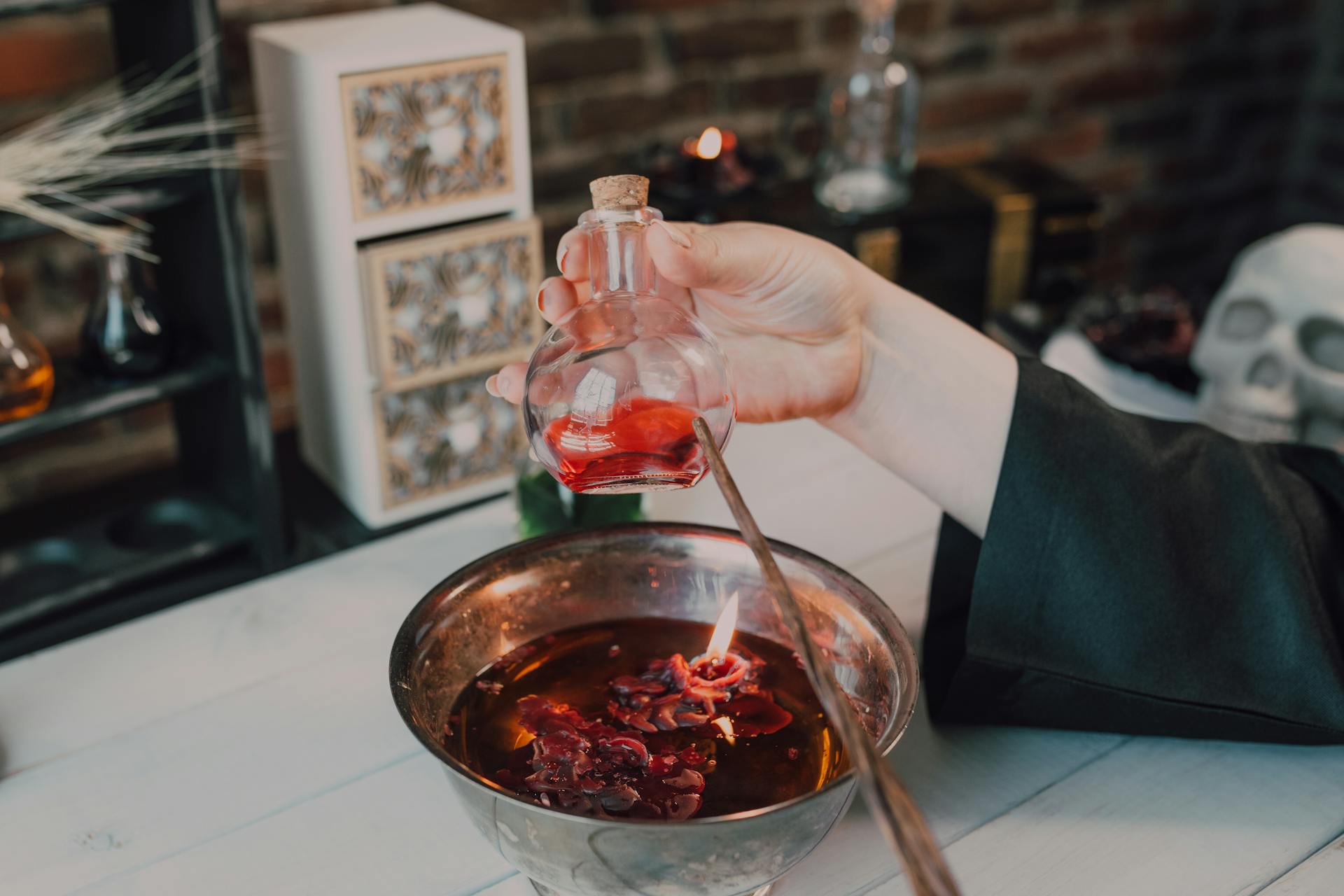
Elixir is a great language for building robust web apps because it's designed to handle high concurrency and fault tolerance.
Elixir's concurrency model is based on the Actor model, which allows for efficient handling of multiple requests simultaneously.
This approach enables Elixir to scale horizontally, making it an excellent choice for large-scale web applications.
Phoenix, a popular Elixir web framework, provides a simple and elegant way to build web applications.
Additional reading: Developing Web Applications
Why You Should Care
Elixir is a modern, high-performance language that runs on the Erlang VM, which means it can handle a massive number of concurrent connections with ease.
This makes it an ideal choice for building scalable and fault-tolerant systems that can handle high traffic and sudden spikes in usage.
Elixir's concurrency model is based on the Actor Model, which allows for easy parallelization of tasks and a significant reduction in latency.
As a result, Elixir applications can handle a large number of requests without significant performance degradation.
Elixir's strong focus on functional programming also makes it easier to write concurrent code that's predictable and maintainable.
This, combined with its concise and readable syntax, makes Elixir a joy to work with.
Elixir's ecosystem is also growing rapidly, with a wide range of libraries and tools available for tasks such as data processing, web development, and more.
This means you can focus on building your application without having to reinvent the wheel or spend time searching for the right tools.
Elixir's community is also very active and supportive, with many resources available for learning and troubleshooting.
This makes it easy to get help when you need it, and to stay up-to-date with the latest developments in the Elixir world.
Intriguing read: Web Dev Tools
Getting Started
To get started with Elixir web programming, you'll need to install Elixir on your machine.
You don't need to know anything about Phoenix, but it's assumed you've programmed a web app before in some language or framework.
To follow along with this guide, create a new project with mix new helloplug and add Cowboy, Plug, Ecto, and Sqlite.Ecto to your project's mix.exs file by adding the specified lines. Don't forget to run mix deps.get when you're done.
Following Along
To follow along, you'll need Elixir installed on your machine. You can install Elixir by following the official installation guide.
You'll also need to create a new project with mix new helloplug. This will set up a new project with a default directory structure and dependencies.
To add dependencies, you'll need to add the following lines to your project's mix.exs file: Cowboy, Plug, Ecto, and Sqlite.Ecto. Don't forget to run mix deps.get when you're done.
You can write any code in the lib/helloplug.ex file, but if you're comfortable with it, Elixir's standard directory structure is cleaner. A Plug doesn't need to be a router, and you can use this technique to include Plugs that do authentication, DOS protection, logging, and more.
If you're not familiar with Elixir's syntax, don't worry! I'll explain the trickier parts in more depth. However, if you get stuck, try taking a look at the Elixir Crash Course.
Start the Server
To start your Phoenix application, you'll need to run the Phoenix server. Start the Phoenix server using the following command:
The server will be running on port 4000.
Your new Phoenix application is now running, and you can access it by navigating to http://localhost:4000 in your browser.
Recommended read: C Programming Web Server
Ecto Database Wrapper
Ecto is a standalone project that we can use with Phoenix, and it's a powerful database wrapper and query generator.
It integrates seamlessly with Phoenix and provides a robust and flexible way to interact with databases.
Ecto's composable queries and changeset validations ensure data integrity and consistency.
To use Ecto, we'll need to create a new module for our database's "Repo", a module that holds functions that query our database.
We'll also need a User model, which is created using Ecto's schema.
The Ecto schema has way too many options to even start covering here, so we'll just need to read the docs if we start using Ecto more.
We can run migrations with mix ecto.migrate, which will update our database to match our Ecto schema.
The User struct is something we get for free when we create an Ecto model, and we can just pass the struct to Repo, and it'll automatically figure out which table to insert it into in the database.
Testing and Reliability
Testing is incredibly simple in Elixir, thanks to its Plug testing framework that allows you to pass a fake Conn to your router's call function and inspect the result.
Elixir's Plug test framework is a powerful tool that makes testing a breeze. With it, you can create fake connections to test your router's behavior.
Phoenix Framework's reliability and availability are thanks to Elixir and Erlang VM, which offer high availability and automatic process restoration in case of errors.
Erlang's "let it crash" philosophy is inherited by Elixir, which means your applications are designed to be resilient and can recover from unexpected failures.
Worth a look: Go Programming Web Framework
Phoenix comes with built-in support for ExUnit, Elixir's testing framework, making it easy to write and run tests for your web application.
Elixir applications are designed to be fault-tolerant, with robust supervision trees and process management that ensure high availability and reliability.
With robust supervision trees and process management, your web applications can recover gracefully from unexpected failures, ensuring high availability and reliability.
Our Performance
Our performance is one of the standout features of Elixir web programming. Elixir's framework is built on top of Erlang's virtual machine, which provides robust features like fault tolerance, runtime monitoring, and dynamic code loading.
The Erlang VM's pattern matching code has been heavily optimized, allowing for O(log n) time instead of O(n) for route lookups. This means that our route lookups run in logarithmic time, making them much faster.
We get all the performance benefits of Plug and Cowboy with very little overhead, thanks to the thin layer we use over these projects. This means that we can take advantage of their speed and efficiency without having to do extra work.
For more insights, see: Web Designers Code Nyt
Our framework is thread-safe by default, thanks to Elixir's immutable values. This makes it easy to handle multiple requests simultaneously without worrying about thread safety issues.
Here are some performance benefits of Elixir:
- Elixir runs on top of Erlang’s virtual machine (BEAM), which provides robust features like fault tolerance runtime monitoring and dynamic code loading.
- This allows Elixir developers to optimally monitor both memory usage and CPU load, ensuring that their application performs well under any workload.
- Elixir’s lightweight nature enables scalability, as each process typically uses less than 1 KB in RAM, making it easy to horizontally grow an Elixir-powered application with minimal resources required.
- Elixir also offers fast boot times, increasing time-to-market significantly compared to other platforms/languages.
Developer Productivity
Elixir's syntax is clean and expressive, promoting code readability and maintainability. Combined with the powerful features of the Phoenix framework, developers can build complex web applications quickly and efficiently.
Phoenix offers a "peace of mind, from prototype to production" experience, thanks to its well-written and well-structured documentation, generators, and good tooling. This enables developers to start building projects with ease.
Elixir's simplicity stands out with its readable and straightforward syntax, making it easy for developers to learn and use. The Elixir & Phoenix community is also known for its friendly environment, where developers can quickly solve their problems.
According to a company that introduced Elixir and Phoenix to developers who had never heard of the technology, about 80% of them were able to learn Elixir and Phoenix and build their first CRUD application with tests in just two or three weeks.
Worth a look: Web Programming Tutorial
Here are some key benefits of using Elixir and Phoenix for web development:
• Scalability and Real-Time Streaming: Phoenix provides amazing scalability options for web applications, allowing them to handle a large number of concurrent users.
• Integration with Frontend Libraries: Phoenix integrates easily with popular frontend libraries like React and Vue.js.
• Good tooling, including Elixir programming language simplicity and incredible community support.
The Elixir & Phoenix combo is easy to learn for developers who are familiar with the Rails framework, making it a great choice for web development.
See what others are reading: Web Mobile Application Development
Frequently Asked Questions
Is Elixir worth learning in 2024?
According to the 2024 Stack Overflow survey, Elixir is a highly sought-after programming language to learn, making it a valuable skill to acquire in 2024. If you're looking to boost your career prospects, learning Elixir is definitely worth considering.
Is Elixir frontend or backend?
Elixir is primarily a backend technology, but it can also be used to create interactive user interfaces with the help of Phoenix LiveView. Its versatility makes it a great choice for full-stack development.
What is Elixir programming used for?
Elixir is a high-performance language used for building scalable and concurrent applications, particularly for large-scale sites and apps in industries like telecommunications, e-commerce, and finance. Its unique design enables efficient handling of massive data volumes and low-latency operations.
Sources
- https://codewords.recurse.com/issues/five/building-a-web-framework-from-scratch-in-elixir
- https://curiosum.com/blog/phoenix-framework-guide
- https://blog.elixirmasters.com/the-ultimate-guide-to-web-development-with-elixir-and-phoenix-framework
- https://medium.com/@creolestudios/why-choose-elixir-for-web-app-development-bec076b7ca5a
- https://hackernoon.com/everything-you-need-to-know-about-using-elixir-for-web-development-9t2x35dh
Featured Images: pexels.com