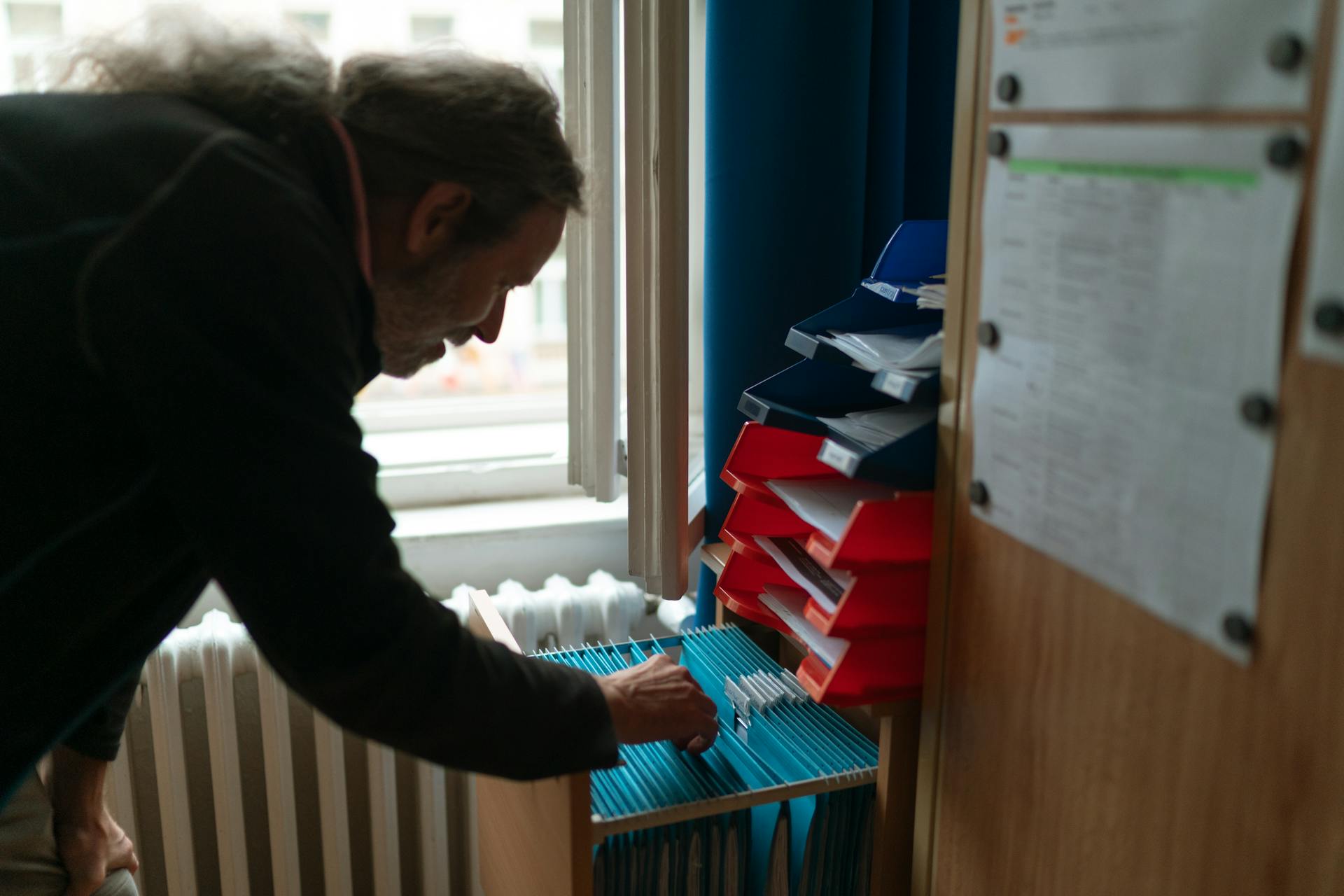
To list all files not folders in Google Drive API, you'll need to use the `files.list` method with specific parameters.
You can use the `fields` parameter to specify the fields you want to retrieve, such as `files(id, name, mimeType)`, to only get the files' IDs, names, and MIME types.
The `q` parameter can be used to filter the results, using the `mimeType` field to exclude folders. For example, `mimeType = 'application/vnd.google-apps.folder'` will exclude folders from the results.
This approach allows you to efficiently list all files in your Google Drive account, without getting unnecessary folder information.
A fresh viewpoint: How to Use Google Drive Cloud Sync
Google Drive API Basics
The Google Drive API is a powerful tool that allows developers to interact with Google Drive programmatically.
To use the Google Drive API, you need to enable the Drive API in the Google Cloud Console. This requires creating a project, enabling the API, and creating credentials.
The API provides a simple way to list all files, not folders, in a Google Drive account.
The List Method
The List Method is a crucial part of working with the Google Drive API. This method allows you to list all the files in a user's Google Drive account, but it can be cumbersome, especially if the user has a large number of files.
The Google Drive API V3 has a limitation of only 1000 rows per page, which can lead to a lot of pagination in your application. To avoid this, you can use the Q parameter in the files.list method to search for files with specific criteria, such as file type, file name, or directory.
Here are some examples of how to use the Q parameter:
- File type: You can search for files of a specific type, such as image/jpeg, using the mimeType query term.
- File name: You can search for files with a specific name, such as "image.jpg", using the name query term.
- Directory: You can search for files in a specific directory by using the parent parameter and passing the file ID of the folder.
The response body of the List files method contains data with the following structure:
The files[] field contains a list of File objects, which have the following structure:
- nextPageToken: The page token for the next page of files.
- kind: Identifies what kind of resource this is.
- incompleteSearch: Whether the search process was incomplete.
- files: The list of files.
You can use the nextPageToken field to fetch the next page of results. If nextPageToken is populated, it means that the search results are incomplete, and you should fetch additional pages to get the complete results.
Query String Examples
When searching for files in Google Drive using the Drive API, you need to construct a query string to filter the results. The query string is a string that contains the search criteria. You can use various operators and parameters to construct the query string.
The simplest query string is a single word or phrase enclosed in quotes, like "hello". This will search for files with the exact name "hello".
Here are some examples of query strings:
You can also use the "contains" operator to search for files that contain a specific word or phrase. For example, if you want to search for files that contain the text "hello", you would use the query string "fullText contains 'hello'".
Note that you should escape special characters in your file names to make sure the query works correctly. For example, if a filename contains both an apostrophe and a backslash, you would use a backslash to escape them, like this: name contains 'quinn\'s paper\\essay'.
See what others are reading: How to Use Google Drive on Android
Searching Files
You can use the Google Drive API to search for files using a query string.
To filter search results, you can use a client library, as shown in Example 2.
You can use the mimeType query term to narrow results to files of a specific type, such as JPEG files.
For example, you can use the following code to search for JPEG files: mimeType = 'image/jpeg'.
You can also use the properties or appProperties search query term to search for files with a custom file property.
For example, to search for a custom file property that's private to the requesting app called additionalID with a value of 8e8aceg2af2ge72e78, you can use the following query: appProperties has { key='additionalID' and value='8e8aceg2af2ge72e78' }.
To search for files within a specific folder, you can use the parent parameter, as shown in Example 4.
Here's a list of some basic query strings you can use to search for files:
You can also use the query parameter to specify the corpus, driveId, includeItemsFromAllDrives, orderBy, pageSize, pageToken, q, spaces, supportsAllDrives, includePermissionsForView, and includeLabels, as shown in Example 6.
For example, you can use the following query to search for files in a shared drive: q = 'sharedDriveId' in parents.
Additional reading: How to Use Google Drive
Filtering and Sorting
Filtering and sorting your Google Drive API search results can be a game-changer for large file lists.
You can use a client library to filter search results by file name and ID. The mimeType query term can be used to narrow results to specific file types, such as image/jpeg.
To further narrow your search, you can set spaces to drive, which will limit the search to the Drive space. This can help reduce the number of results you need to sift through.
If you're using the nextPageToken, be aware that it will return null when there are no more results.
Worth a look: Search in Folder Google Drive
Search by Custom Property
You can search for files with a custom file property using the properties or appProperties search query term with a key and value.
For example, to search for a custom file property called additionalID with a value of 8e8aceg2af2ge72e78, use the properties or appProperties search query term.
To search for files without a specific custom property, you can use the properties or appProperties search query term without a value.
For more information on custom file properties, see Add custom file properties.
A fresh viewpoint: Open Shared Google Drive without Gmail
Filter Search Results with a Client Library
Filtering search results with a client library is a powerful tool for narrowing down your search. You can use a client library to filter search results to file names and IDs of specific file types, such as JPEG files.
The mimeType query term can be used to narrow results to files of a specific type, in this case image/jpeg. This helps to focus your search on the exact type of file you're looking for.
Setting spaces to drive can further narrow the search to the Drive space, which is useful if you're only interested in files stored in Google Drive. This helps to eliminate irrelevant results and make your search more efficient.
When you've finished filtering your search results, you can use the nextPageToken to determine if there are more results available. If nextPageToken returns null, you know there are no more results to display.
On a similar theme: Unsupported File Type Google Drive
Add Page Size to List
Adding page size to your files.list method can greatly improve performance, especially when dealing with large numbers of files. The default page size is 100, but you can increase it to 1000 rows by passing the PageSize parameter.
You can test this by passing the PageSize parameter to the files.list method. This will tell the API to return 1000 rows instead of the default.
The Google Drive API v3 has a free tier that returns file storage information, making it a great option for developers.
A fresh viewpoint: Google Drive Folder Size
Frequently Asked Questions
How do I list all files in Google Drive?
To list all files in Google Drive, use the files.list method, which returns a list of files and folders. This method can also retrieve the fileId needed for other resource methods.
Sources
- https://tanaikech.github.io/tags/drive-api/
- https://developers.google.com/drive/api/guides/search-files
- https://developers.google.com/drive/api/reference/rest/v3/files/list
- https://www.daimto.com/how-to-search-for-files-with-the-google-drive-api-and-net-core/
- https://www.acrosswalls.org/ortext-datalinks/list-google-drive-folder-file-names-urls/
Featured Images: pexels.com