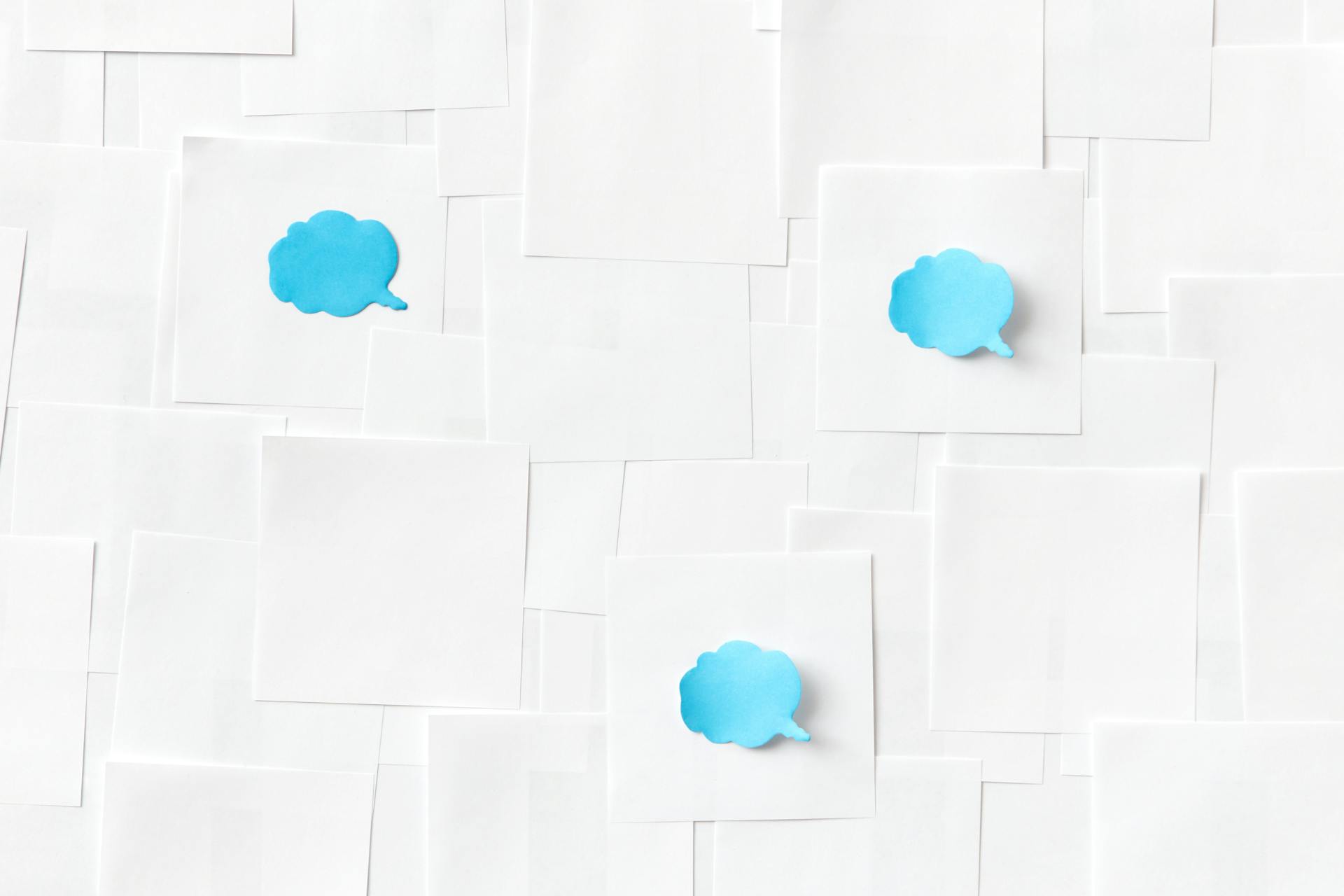
Configuring a jQuery UI dialog is a straightforward process, with several options available to customize its appearance and behavior.
You can set the dialog's title, width, and height using the dialog's options, such as `title`, `width`, and `height`.
The `modal` option determines whether the dialog will prevent the user from interacting with the rest of the page while it's open.
Dialog Options
Dialog options are a crucial part of customizing the jQuery UI dialog. You can check if a dialog is disabled by using the code `var isDisabled = $( ".selector" ).dialog( "option", "disabled" );`.
To set the value of a dialog option, you can use the `option()` method with the option name and value. For example, `option( optionName, value )` sets the value of the dialog option associated with the specified `optionName`.
You can also get the value of a dialog option using the `option()` method with just the option name. For example, `$( ".selector" ).dialog( "option", "optionName" );` will return the value of the option.
Here's a table summarizing the `option()` method:
Example
Dialog options are a crucial part of creating engaging and user-friendly interfaces. The isOpen() method in jQuery UI Dialog allows you to check if a dialog is currently open or not.
You can save the code in an HTML file, for example, dialogexample.htm, and open it in a standard browser that supports JavaScript. This will give you a clear view of the dialog's open state.
To open a dialog, you can use the open() method. This method can be triggered by a button click or any other event. For instance, in Example 2, the open() method is triggered when the button with the id "opener" is clicked.
The close() method is used to close a dialog. This method can be triggered manually or automatically, depending on your application's requirements. In Example 2, the close() method is not explicitly mentioned, but you can assume it would be used to close the dialog when the user is finished interacting with it.
Here is a summary of the methods used to interact with a jQuery UI Dialog:
- isOpen(): checks if the dialog is currently open or not
- open(): opens the dialog
- close(): closes the dialog
Options
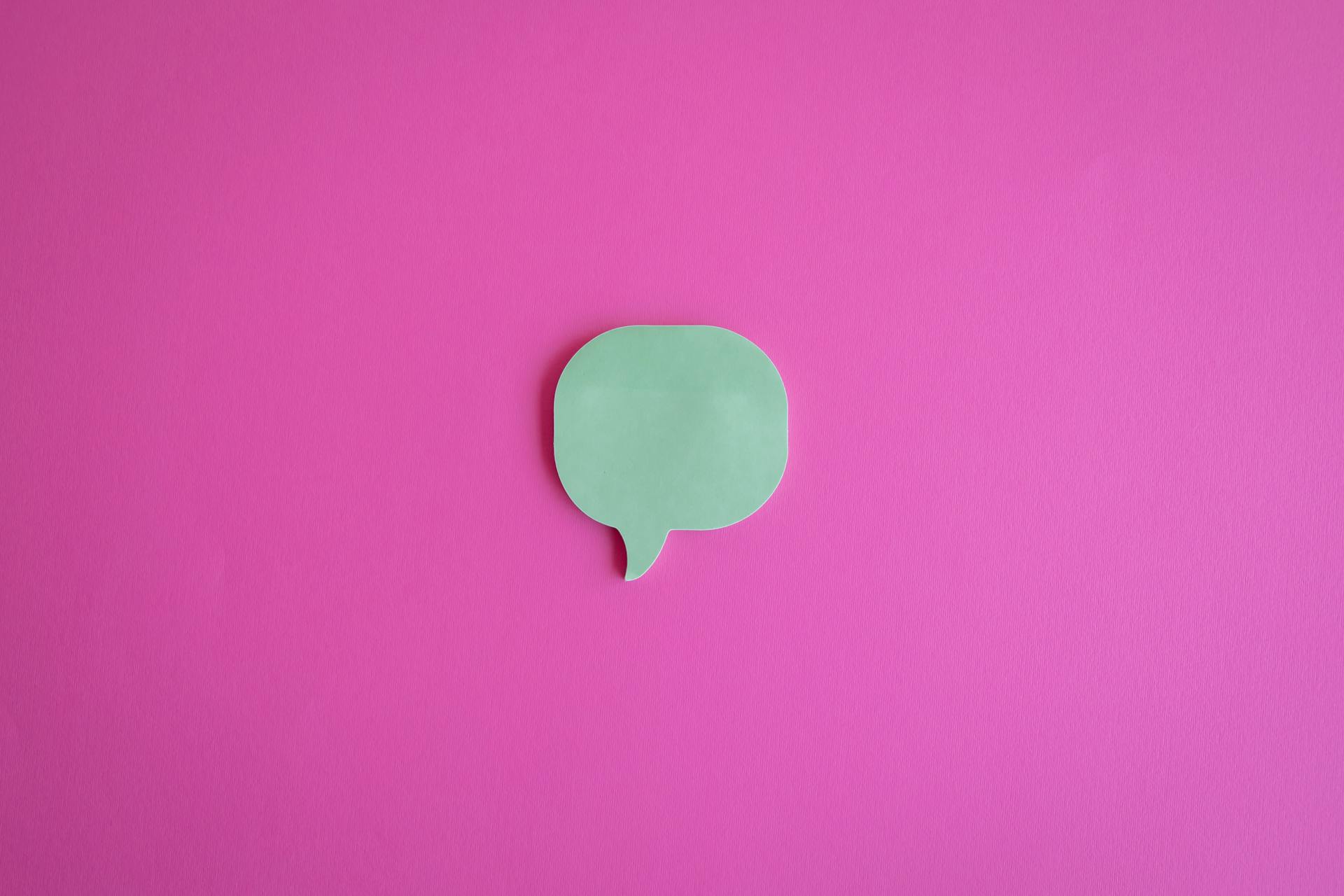
Options are a crucial part of working with dialogs. You can get or set options using the option method.
To get a specific option, you can use the option method without any arguments, like this: $( ".selector" ).dialog( "option" ); This will return an object with all the options set for the dialog.
To set an option, you can use the option method with two arguments: the option name and its value. For example: $( ".selector" ).dialog( "option", "disabled", true ); This will set the disabled option to true.
You can also use dot notation to set the value of an option that has an object as its value. For example: $( ".selector" ).dialog( "option", "foo.bar", "baz" ); This will set the bar property of the foo option to "baz".
Here are some key things to know about options:
The optionName can be a string, and the value can be an object. You can use dot notation to set the value of an option that has an object as its value.
Dialog Behavior
Dialog behavior is crucial for a seamless user experience.
The modal dialog is a blocking dialog, which means it prevents the user from interacting with the rest of the page until it's closed.
You can set the modal property to false to create a non-blocking dialog, allowing the user to interact with the page while the dialog is open.
A dialog can be closed in several ways, including clicking the close button, pressing the escape key, or clicking outside the dialog.
On a similar theme: Web Page Ui Design
On Escape
You can prevent a dialog from closing when the user presses the escape key by initializing it with the closeOnEscape option set to false. This is done by using the following code: $( ".selector" ).dialog({closeOnEscape: false});
To get or set the closeOnEscape option after initialization, you can use the following code: // Gettervar closeOnEscape = $( ".selector" ).dialog( "option", "closeOnEscape" );// Setter$( ".selector" ).dialog( "option", "closeOnEscape", false );
Here's a quick summary of how to work with the closeOnEscape option:
IsOpen Boolean
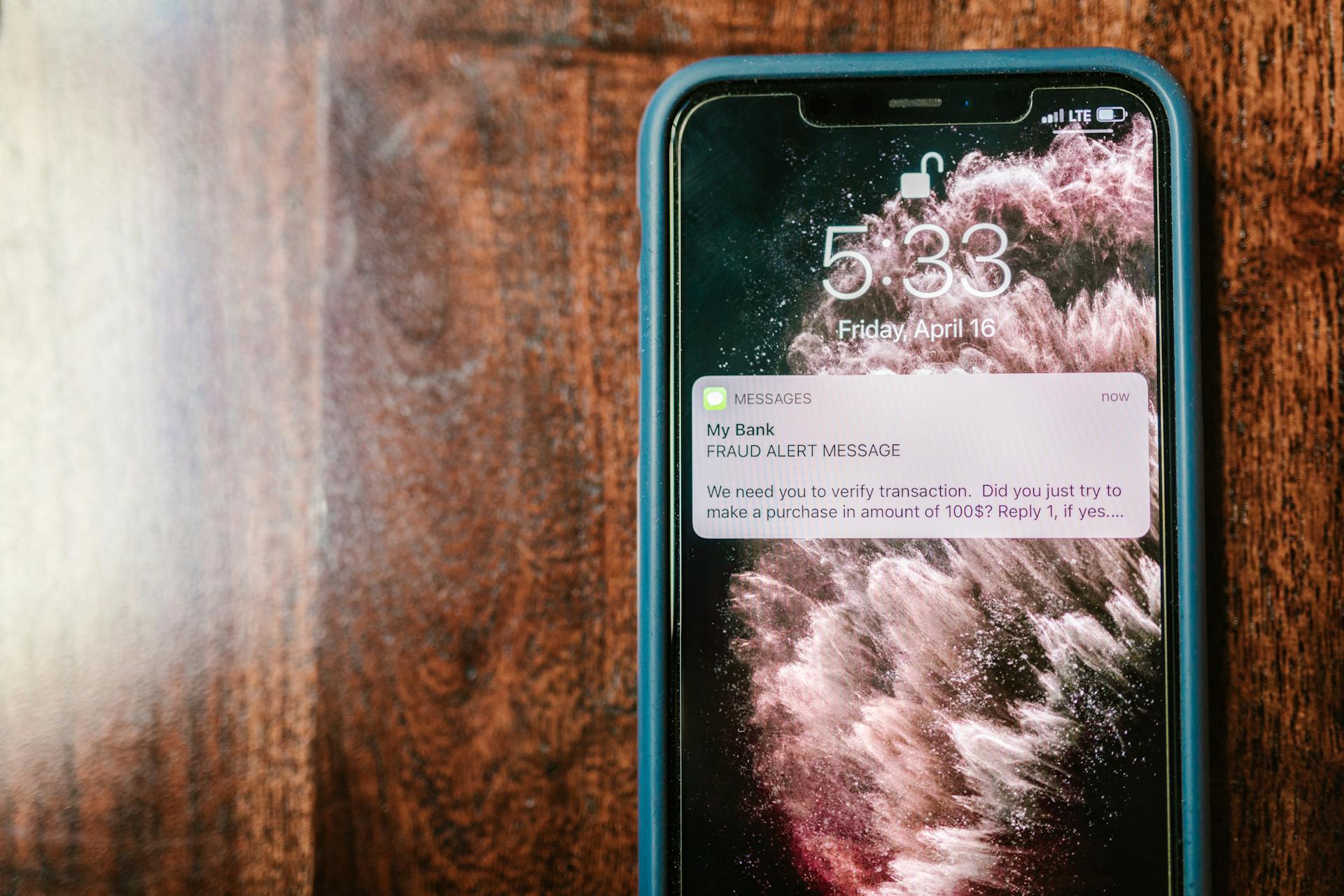
The isOpen Boolean is a crucial aspect of dialog behavior. It returns a Boolean value indicating whether a dialog is open or not.
You can invoke the isOpen method by using the following syntax: $( ".selector" ).dialog( "isOpen" ). This will return a Boolean value, which you can store in a variable if needed.
Here's a simple example: var isOpen = $( ".selector" ).dialog( "isOpen" ); This will check if the dialog is open and store the result in the isOpen variable.
The isOpen method is a quick way to determine the state of a dialog. You can use this information to trigger other actions or update the UI accordingly.
In summary, the isOpen Boolean is a useful tool for dialog behavior.
A different take: How to Open Html Editor Ona Website
Remove "stop
Removing "stop" can be as simple as binding an event listener to the dialogdragstop event. This can be done using the jQuery method .on().
You can bind the event listener to the dialogdragstop event like this: $( ".selector" ).on( "dialogdragstop", function( event, ui ) {} );. This will allow you to execute code when the drag is stopped.
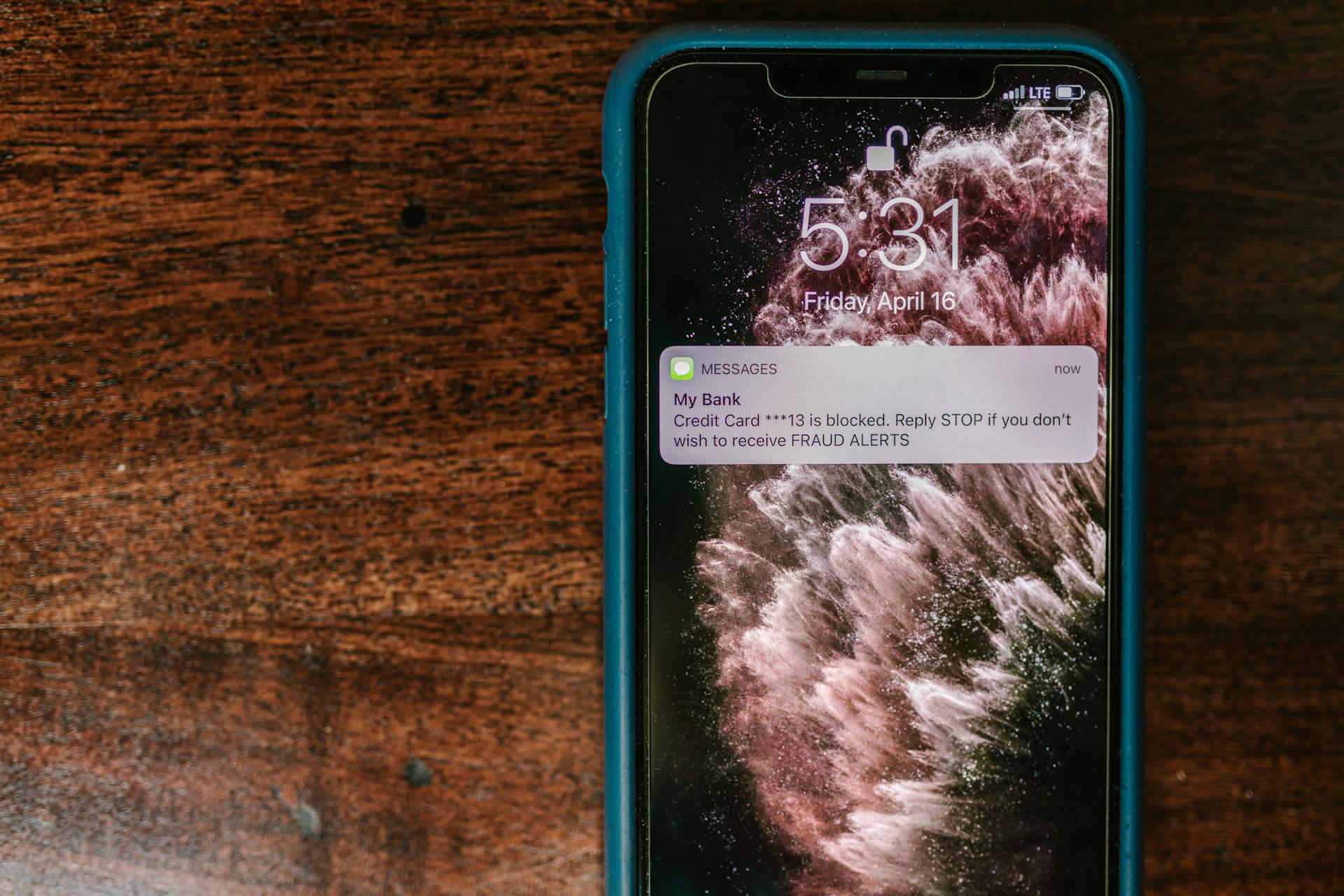
To remove the "stop" part, you can simply initialize the dialog without specifying the dragStop callback. This will prevent the drag from stopping.
Here's a table to illustrate the difference:
By not specifying the dragStop callback, you can effectively remove the "stop" part and allow the drag to continue uninterrupted.
Dialog Appearance
The appearance of a jQuery UI dialog is customizable to suit your needs. You can use the hide option to hide the dialog widget, which is useful when you want to temporarily remove it from the page.
To show the dialog again, you can use the show option. This is particularly useful if you need to display the dialog after it's been hidden.
The height option allows you to specify the height of the dialog, giving you more control over its appearance.
Modal
To make your dialog truly modal, you'll want to specify the modal option when initializing it. This can be done by adding the modal: true option to the dialog function, like this: $( ".selector" ).dialog({modal: true}).
The dialog will handle collisions so that as much of the dialog is visible as possible, making it a great option for situations where you need to ensure the user's attention is focused on the dialog.
You can get or set the modal option after initialization using the dialog option method. For example, to get the current modal setting, you can use the following code: var modal = $( ".selector" ).dialog( "option", "modal" ).
To set the modal option to true, you can use the following code: $( ".selector" ).dialog( "option", "modal", true ).
A fresh viewpoint: No Code Html Editor
Resizable
To get or set the resizable option, you can use the "option" method. For example, to get the resizable option, you can use $( ".selector" ).dialog( "option", "resizable" );. To set it, you can use $( ".selector" ).dialog( "option", "resizable", false );.
The "option" method is versatile and can be used to get or set various options, not just the resizable option. The optionName parameter specifies the name of the option to get or set. In the case of the resizable option, you would use "resizable" as the optionName.
If this caught your attention, see: Jquery Set Img Src
Max Height
Max Height is a crucial option when it comes to customizing the appearance of a dialog. You can get or set the maxHeight option after initialization.
To get the current maxHeight value, you can use the following code: var maxHeight = $( ".selector" ).dialog( "option", "maxHeight" );. This will return the current maximum height of the dialog.
You can also set a new maxHeight value using the following code: $( ".selector" ).dialog( "option", "maxHeight", 600 );. This will set the maximum height of the dialog to 600 pixels.
In some cases, you may need to adjust the maxHeight value based on the content of the dialog. For example, if the dialog contains a lot of text, you may want to increase the maxHeight value to accommodate the extra content.
Text
The closeText option is used to get or set the text that appears when a dialog is closed. This can be set to a custom string, such as "hide".
You can use the dialog's option method to get or set the closeText option. For example, you can use `$( ".selector" ).dialog( "option", "closeText" );` to get the current closeText option.
Title
The title of a dialog is a crucial aspect of its appearance. You can get or set the title option after initialization using the "option" method.
To get the current title, you can use the following code: `var title = $( ".selector" ).dialog( "option", "title" );`. This will return the current title of the dialog.
You can also set a new title by using the same "option" method with the title as the second argument: `$( ".selector" ).dialog( "option", "title", "Dialog Title" );`. This will update the title of the dialog.
If you want to specify the title when initializing the dialog, you can do so by adding the `title` option to the dialog initialization object: `$( ".selector" ).dialog({title: "Dialog Title"});`. This will set the title of the dialog to the specified value.
The title can be changed at any time using the "option" method.
Button, Title, and Position
The button, title, and position options are key to customizing the appearance of your dialog. You can use these options to add a title to your dialog, specify the position of the dialog on the screen, and even add custom buttons.
The title option can be set using the "option" method, like this: $( ".selector" ).dialog( "option", "title", "Dialog Title" );. This will change the title of the dialog to "Dialog Title".
You can also use the position option to specify where the dialog should appear on the screen. This option can be set to an object, string, or array. If you want to position the dialog relative to another element, you can use the "of" option.
Here are the possible values for the position option:
- Object: Identifies the position of the dialog when opened. The of option defaults to the window, but you can specify another element to position against.
- String: A string representing the position within the viewport. Possible values: "center", "left", "right", "top", "bottom".
- Array: An array containing an x, y coordinate pair in pixel offset from the top left corner of the viewport or the name of a possible string value.
Note that the string and array forms of the position option are deprecated, so it's best to use the object form for maximum flexibility.
To get or set the position option, you can use the "option" method, like this: $( ".selector" ).dialog( "option", "position", { my: "left top", at: "left bottom", of: button } ).
Dialog Interaction
Dialog Interaction is a crucial aspect of jQuery UI Dialog. It allows users to interact with the dialog box in various ways.
You can open a dialog box programmatically by calling the dialog method on a jQuery object. For example, $('#dialog').dialog('open'); opens the dialog box with the id 'dialog'.
The modal property determines whether the dialog box is modal or not. A modal dialog box prevents users from interacting with the rest of the page until they close it.
Quick Examples
Dialogs can be moved, resized, and closed with the 'x' icon by default.
A dialog window is a floating window that contains a title bar and a content area. This layout is a standard feature of dialog boxes.
If the content length exceeds the maximum height, a scrollbar will automatically appear. This is a convenient feature that helps users navigate through long dialog content.
Here are some key features of a dialog window:
Buttons
Buttons are a crucial part of dialog interaction, allowing users to take specific actions. They can be customized with various attributes, properties, and event handlers.
Each button is defined by an object with keys for label and value, which is an array of objects. Each element in the array must be an object with its own attributes, properties, and event handlers.
A button's icon can be controlled using the key "icon", and its text option can be controlled using the key "showText". If "showText" is set to false, the label will be used as a tooltip.
Here are the basic properties of a button object:
- text: the label of the button
- icon: the icon of the button
- click: the callback function when the button is clicked
- showText: whether to show the button's text or not
To initialize a dialog with buttons, you can use the "buttons" option, like this: $( ".selector" ).dialog({buttons: [ {text: "Ok",icon: "ui-icon-heart",click: function() { $( this ).dialog( "close" ); }} ]});
You can also get or set the buttons option after initialization using the "option" method: $( ".selector" ).dialog( "option", "buttons", [ {text: "Ok",icon: "ui-icon-heart",click: function() { $( this ).dialog( "close" ); }} ]);
Suggestion: Data Text Html Base64
able
To get or set the draggable option, you can use the "option" method, like this: var draggable = $( ".selector" ).dialog( "option", "draggable" ); or $( ".selector" ).dialog( "option", "draggable", false );
The draggable option can be either true or false, and it determines whether the dialog can be dragged around the screen.
A unique perspective: Jquery Ui Draggable
Hide, Show, and Height
The dialog widget in JqueryUI offers a range of options to customize its appearance and behavior. One of these options is the ability to control the visibility of the dialog using the hide and show methods.
To hide a dialog, simply call the hide method on the dialog object. This will instantly hide the dialog from view.
You can also use the show method to display the dialog again. This is useful when you want to toggle the visibility of the dialog based on user input or other conditions.
The height option can be used to set the height of the dialog. This can be particularly useful when you want to create a dialog that is taller than the default height.
Dialog Management
Dialog Management is a crucial aspect of working with jQuery UI Dialog. You can destroy a dialog instance using the destroy method.
This method is only available in the plugin version of jQuery UI Dialog. To invoke it, you simply call the destroy method on the dialog instance.
For example, if you have a dialog instance with the class selector, you can destroy it like this: `$( ".selector" ).dialog( "destroy" );`.
This is all there is to it. The destroy method is a straightforward way to remove a dialog instance from the DOM.
Dialog Positioning
Dialog Positioning is a crucial aspect of creating engaging and user-friendly experiences with jQuery UI Dialog. The position option can be specified when initializing the dialog, allowing you to control where the dialog appears on the screen.
You can use the position option to identify the position of the dialog when opened, and it defaults to the window, but you can specify another element to position against. This can be useful when you want the dialog to appear relative to a specific button or element on the page.
The position option can be specified in three ways: as an object, a string, or an array. The object form is recommended, as it provides the most flexibility and control.
Here are the possible values for the string form of the position option:
- center
- left
- right
- top
- bottom
Note that the string and array forms are deprecated, so it's best to use the object form for new code.
Dialog Events
Dialog events are a crucial part of creating interactive and dynamic user experiences with the jQuery UI dialog. You can bind event listeners to various events, such as dialogcreate, dialogdragstart, and dialogresize, to execute custom code when these events occur.
To initialize a dialog with a callback, you can use the dialog method and specify the callback function. For example, to initialize a dialog with the create callback specified, you can use the code: $( ".selector" ).dialog({create: function( event, ui ) {}});
Here is a list of some common dialog events and how to bind event listeners to them:
By binding event listeners to these events, you can create a more engaging and interactive user experience with the jQuery UI dialog.
Before Event
Before an event occurs, it's essential to understand the different types of events that can be triggered in a dialog. The dialogbeforeclose event is triggered when a dialog attempts to close, and if the beforeClose event handler returns false, the close will be prevented.
You can supply a callback function to handle the beforeClose event as an init option, like this: $( ".selector" ).dialog({beforeClose: function(event, ui) { ... }}); or bind to the beforeClose event by type: dialogbeforeclose, like this: $( ".selector" ).bind( "dialogbeforeclose", function(event, ui) { ... });.
This event is crucial for preventing accidental closes or allowing the user to confirm whether they want to close the dialog.
Event Management
Event management is a crucial aspect of creating engaging and interactive dialog boxes. You can bind event listeners to various events that occur during the dialog's lifecycle, such as when it's created, opened, or closed.
To initialize a dialog with a callback function, you can specify it as an option when creating the dialog. For example, you can initialize a dialog with a create callback specified: $( ".selector" ).dialog({create: function( event, ui ) {}});
You can also bind an event listener to the dialogcreate event: $( ".selector" ).on( "dialogcreate", function( event, ui ) {} );
Readers also liked: Create Css Selector from Webpage
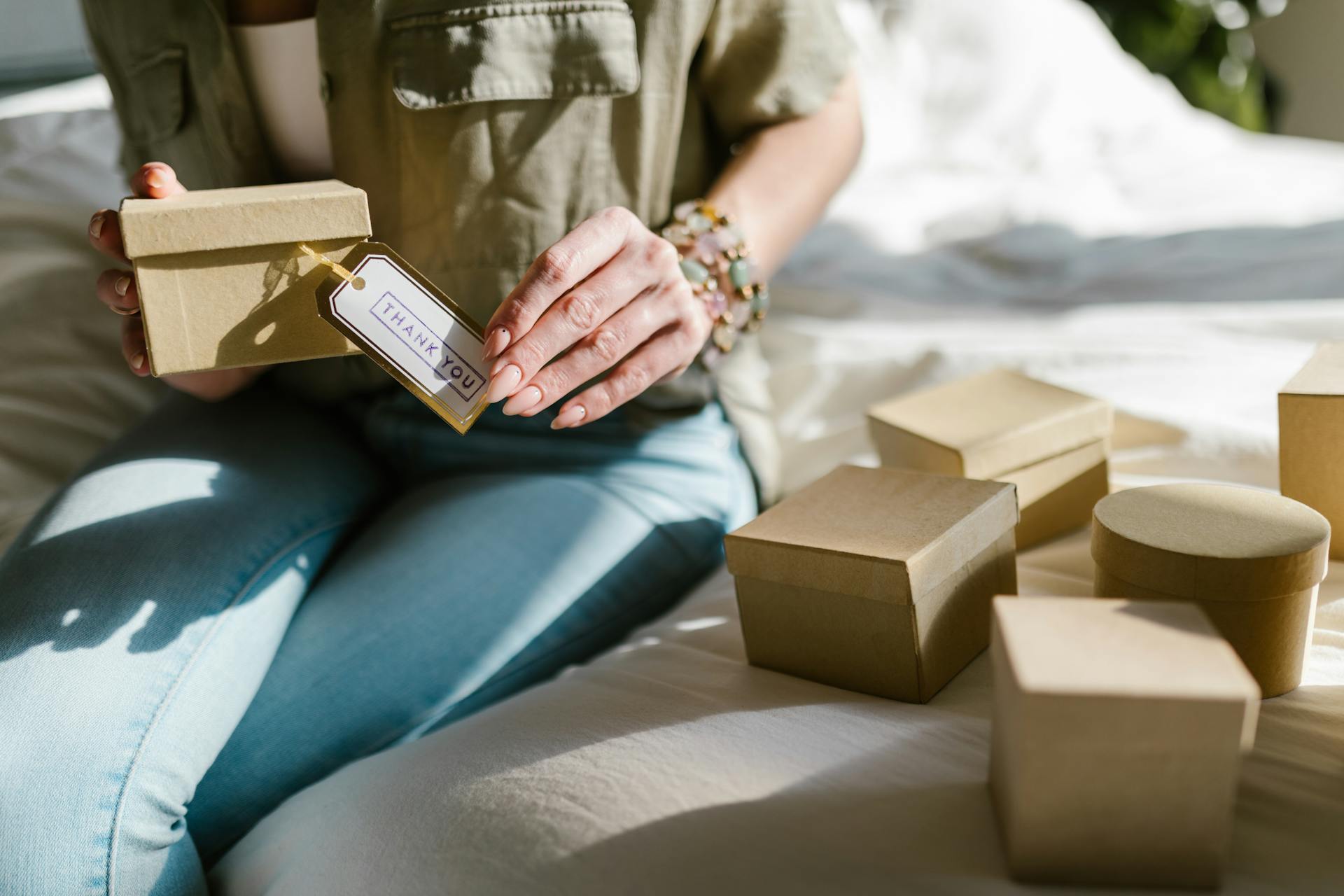
There are several events you can bind to, including dialogopen, dialogfocus, dialogdragstart, dialogdrag, dialogdragstop, dialogresizestart, dialogresize, and dialogresizestop.
Here's a list of some of the most common events:
- dialogcreate: triggered when the dialog is created
- dialogopen: triggered when the dialog is opened
- dialogfocus: triggered when the dialog gains focus
- dialogdragstart: triggered at the beginning of the dialog being dragged
- dialogdrag: triggered when the dialog is dragged
- dialogdragstop: triggered after the dialog has been dragged
- dialogresizestart: triggered at the beginning of the dialog being resized
- dialogresize: triggered when the dialog is resized
- dialogresizestop: triggered after the dialog has been resized
You can bind event listeners to these events using the on() method, like this: $( ".selector" ).on( "dialogopen", function( event, ui ) {} );
By binding event listeners to these events, you can create a more interactive and engaging dialog box that responds to user actions.
Dialog Methods
The dialog methods in jQuery UI are incredibly versatile and useful. You can close the dialog box with a single line of code: `$(".selector").dialog("close");`.
You can also check if the dialog box is open with the `isOpen()` method, like this: `$(".selector").dialog("isOpen");`. This returns a boolean value indicating whether the dialog is open or not.
Here are the different actions you can perform on a dialog box using the `.dialog()` method:
You can also use the `.dialog()` method to get or set options for the dialog, such as the show option, which can be used to specify how the dialog should be shown. For example: `$( ".selector" ).dialog({show: { effect: "blind", duration: 800 }});`.
$ selector method action params
The $ selector method action params is a crucial part of working with dialog boxes. You can perform various actions on a dialog box using this method.
To close a dialog box, you can use the action "close()" with no additional parameters. This method does not accept any arguments. For example, $(".selector").dialog("close");
There are several other actions you can perform on a dialog box, including destroying it, checking if it's open, moving it to the top, and opening it. Here's a list of the available actions:
You can also use the action "option()" to get or set options for the dialog box. This method can be used to get the value of a specific option, get an object containing all the current options, or set one or more options. For example, $(".selector").dialog("option", "disabled", true);
Hide
The "hide" method is a crucial part of dialog functionality, allowing you to control how your dialog is hidden from view.
You can specify the hide option when initializing the dialog, which can be done in several ways. For example, you can use a string value, such as "explode", to specify a jQuery UI effect. You can also use an object with properties like "effect", "duration", and "easing" to customize the hide animation.
Here are the possible values for the hide option:
To get or set the hide option after initialization, you can use the "option" method. For example, you can use the following code to get the hide option: `var hide = $( ".selector" ).dialog( "option", "hide" );`. To set the hide option, you can use the following code: `$( ".selector" ).dialog( "option", "hide", { effect: "explode", duration: 1000 } );`.
Show
The show option can be used to customize the appearance of a dialog. You can specify an effect, duration, and easing to create a smooth and visually appealing animation.
The show option can be used in several ways. It can be a boolean value, a number, a string, or an object. If it's a boolean value, setting it to false will show the dialog immediately without animation, while setting it to true will use the default animation.
Here are some examples of how to use the show option:
- To show the dialog with a specified effect, you can use a string value, such as "blind" or "fold".
- To show the dialog with a specified duration, you can use a number value, such as 800.
- To show the dialog with a specified easing, you can use an object value, such as { effect: "blind", duration: 800 }.
Here's a summary of the show option:
You can also get or set the show option after initialization using the "option" method. For example:
- To get the show option, you can use $( ".selector" ).dialog( "option", "show" );
- To set the show option, you can use $( ".selector" ).dialog( "option", "show", { effect: "blind", duration: 800 } );
By customizing the show option, you can create a more engaging and user-friendly dialog experience.
Methods
The dialog methods are a powerful tool for managing your dialog boxes. You can close the dialog box with a single method call, no matter what its current state.
To close the dialog box, use the close() method. This method does not accept any arguments and can be called on any dialog box.
You can also remove the dialog functionality completely with the destroy() method. This will return the element back to its pre-init state.
To check if the dialog box is open, use the isOpen() method. This method does not accept any arguments and returns true if the dialog is currently open.
If you want to position the dialog box to the foreground, use the moveToTop() method. This method does not accept any arguments and is useful when you have multiple dialog boxes open.
You can get or set any dialog option with the option() method. If no value is specified, the method will act as a getter.
Here are the dialog methods in a list format:
- .dialog("close") - Close the dialog box.
- .dialog("destroy") - Remove the dialog functionality completely.
- .dialog("isOpen") - Check if the dialog box is open.
- .dialog("moveToTop") - Position the dialog box to the foreground.
- .dialog("open") - Open the dialog box.
- .dialog("option", optionName) - Get or set any dialog option.
- .dialog("widget") - Returns the .ui-dialog element.
These dialog methods are a crucial part of any jQuery UI dialog implementation. By mastering these methods, you can take your dialog box management to the next level.
Featured Images: pexels.com