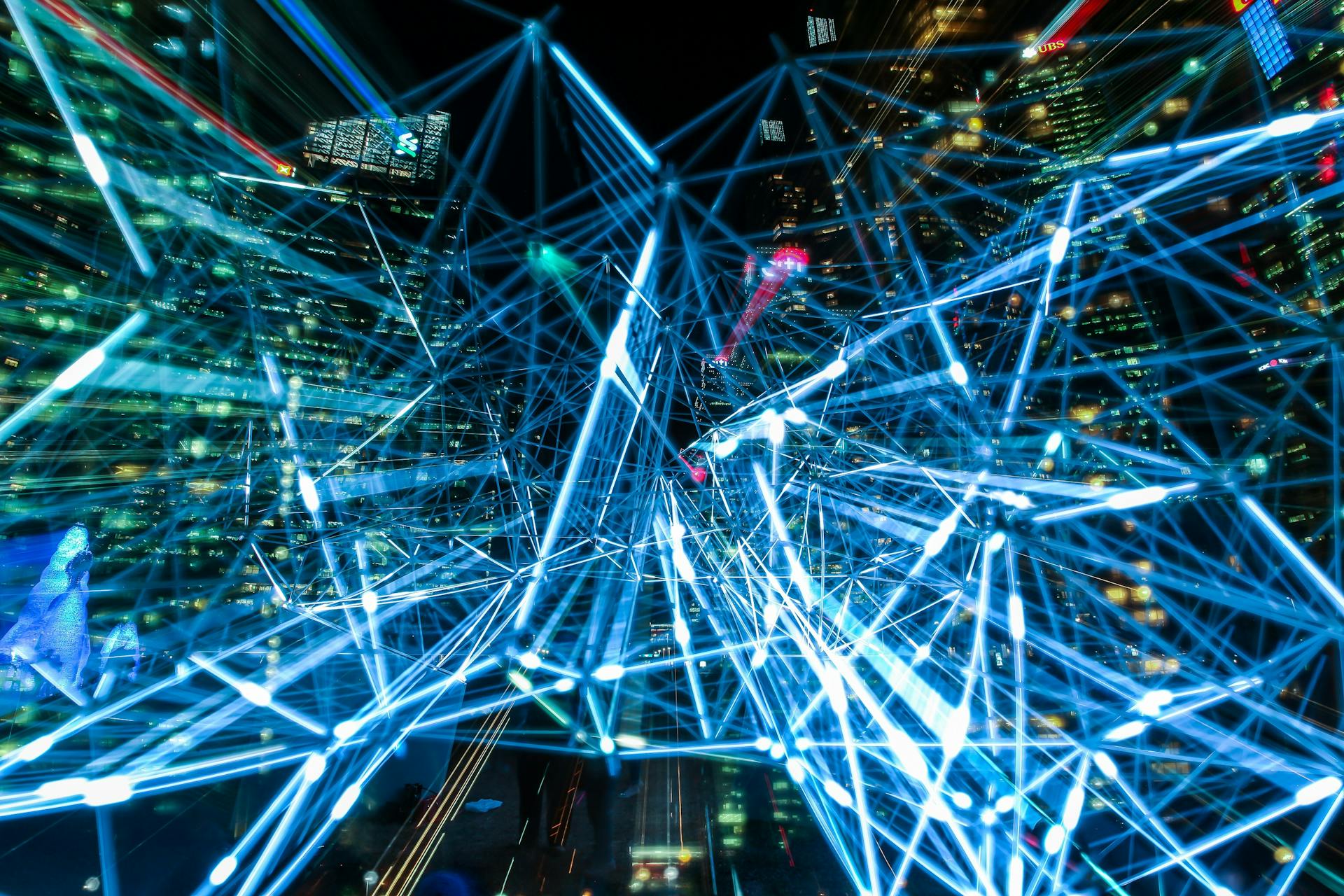
NetworkX PageRank is a powerful algorithm that helps us understand the importance of each node in a network. It's based on the original PageRank algorithm developed by Google founders Larry Page and Sergey Brin.
PageRank assigns a score to each node, which represents its relative importance in the network. This score is calculated by analyzing the number of incoming links to each node.
The PageRank score is a number between 0 and 1, with higher scores indicating more importance. For example, a node with a score of 0.8 is more important than a node with a score of 0.2.
NetworkX provides a simple way to calculate PageRank scores using the `pagerank` function. This function takes a network as input and returns a dictionary with the PageRank scores for each node.
If this caught your attention, see: Pagerank Algorithm
PageRank in NetworkX
PageRank in NetworkX is a powerful algorithm that helps us understand the importance of nodes in a graph. It's based on the idea that a link from page A to page B is like a vote from page A for page B.
Discover more: Pagerank Paper
The algorithm is robust against spam because it's hard for a web page owner to add links from important pages to their own page. This is because page importance is calculated as the sum of the votes from its incoming links.
NetworkX makes it easy to run PageRank on a specific group of nodes, which is useful when dealing with large graphs. You can save a sub-graph in a variable and then provide it as an argument to the algorithm using the project() function.
For another approach, see: High Pagerank Links
How It Works
PageRank is a system that interprets links from one page to another as votes, with more important pages casting more valuable votes. It's based on the idea that a link from page A to page B is a vote from page A to page B.
The algorithm takes into account the importance of the page giving out the vote, with more important pages casting more valuable votes. This means that if page A is more important, its links are worth more and will help rank up the pages it links to.
PageRank is mathematically defined as PR(A) = (1 - d) + d i=1nPR(Ti)C(Ti), where d is a damping factor set between 0 and 1, usually set to 0.85. This formula shows how the algorithm calculates the importance of each page.
The algorithm is robust against spam since it's not easy for a web page owner to add in links to their page from other important pages. However, it favors older pages because new pages won't have many links going towards them.
The PageRank algorithm has some default arguments, including max_iterations, damping_factor, and stop_epsilon, which are the same as in the NetworkX PageRank implementation. These arguments control the number of iterations, the damping factor, and the stopping criteria for the algorithm.
Here are the default arguments for the PageRank algorithm:
Running on a Subgraph
You can run PageRank only on a specific group of nodes with the project() function. This is useful if you only need to analyze a subset of your data.
To do this, save the sub-graph in a variable, then provide it as the first argument of the algorithm. This is a great way to reduce computation time and focus on the most important parts of your network.
As with all other algorithms in the MAGE open-source library, you can use the project() function to run PageRank on a subgraph. This is a powerful tool for analyzing complex networks.
If your application is highly time-sensitive, consider using the Dynamic PageRank feature. This allows the preservation of the previously processed state, so you can quickly update your analysis as new nodes and relationships arrive.
Method Output
The output of the PageRank method in NetworkX is a dictionary. This dictionary has nodes as keys and PageRank values as the corresponding values.
The dictionary structure is straightforward, making it easy to access and work with the PageRank values.
Personalized
Personalized PageRank is a type of PageRank that's super useful in recommendation systems. It allows you to restrain the random walk by starting from a specific set of nodes and jumping only to a given set.
This brings out central nodes from the perspective of that set of specific nodes. For example, Twitter uses Personalized PageRank to recommend who to follow online.
A sequel of a well-liked movie will automatically be more popular than just a random new title because it already has an established fan base. In graph terms, the biggest node pointing to an adjacent node makes it more important.
PageRank can be used as a measure of influence for a variety of applications, not just for website pages and movie rankings.
Custom Query Module
Memgraph is integrated with NetworkX, allowing it to transform NetworkX graphs into Memgraph graphs, along with the set of NetworkX algorithms.
A custom query module can be developed in the Query Modules section by creating a new module, which can be used to run NetworkX algorithms on NetworkX DiGraph objects.
NetworkX algorithms inside Memgraph are optimized for the best performance and run on Memgraph DiGraph objects.
A custom query module can be used to run the NetworkX PageRank algorithm on the NetworkX DiGraph object for a fairer comparison.
The code for the custom query module is used for the comparison, where a procedure pagerank extracts a graph from the context and creates an instance of NetworkX DiGraph.
The NetworkX PageRank algorithm is then run on that DiGraph.
Procedures from custom query modules are run from the Query Execution section.
The pagerank() procedure from the measure query module is called with the following Cypher query: CALL measure.pagerank() YIELD node, rank.
PageRank Applications
PageRank can help identify nodes likely to fail and if they would cascade to other nodes in the network.
Critical infrastructures, such as energy infrastructure, can be represented as a network of highly interdependent nodes and relationships, where failure in one node may result in a cascade of failures in other nodes.
Using PageRank algorithm outputs to identify vulnerabilities in the topology is invaluable and can save time, money, and frustration for both companies and users.
Fraud Detection
Fraud detection is a crucial application of PageRank.
PageRank can be used as an additional feature to a machine learning algorithm to improve classification and reduce false positives.
Users who are involved in fraudulent transactions with shared cards are more likely to be fraudsters.
Nodes can be ranked based on how much money flows through each one to flag transactions that move much more money than what's average for a specific user.
In fact, nodes involved in transactions with known fraudsters can be a valuable piece of information for machine learning models to predict and detect fraud.
Network Optimization
Network optimization is a powerful application of PageRank. Critical infrastructures are systems that can be represented as a network of highly interdependent nodes and relationships.
The failure of one node in a network can result in a cascade of failures in other nodes. This makes it crucial to identify potential vulnerabilities in the topology.
PageRank can help identify nodes likely to fail and if they would cascade to other nodes in the network. This information can be invaluable for companies and users alike.
Using PageRank algorithm outputs to identify vulnerabilities in the energy infrastructure topology can save time, money, and frustration.
Example
Let's take a look at how to use the PageRank algorithm in Python.
Python's NetworkX library implements the PageRank algorithm, which is a key component of its Link Analysis algorithms.
You can use the PageRank algorithm to analyze the importance of nodes in a network, but how does it actually work?
The PageRank algorithm works by assigning a score to each node based on the number and quality of its links.
Here's a breakdown of the different components of the PageRank algorithm:
- Python code: You can use the PageRank algorithm in Python by importing the NetworkX library and creating a graph object.
- Output: The output of the PageRank algorithm is a dictionary where the keys are the node IDs and the values are the corresponding PageRank scores.
- Visualization: You can visualize the PageRank scores using a variety of tools, such as NetworkX's built-in visualization functions or third-party libraries like Matplotlib.
To get started with the PageRank algorithm, you'll need to have Python and the NetworkX library installed on your machine.
The Comparison
Memgraph's get() procedure is called from the pagerank module, and the custom procedure pagerank() from the measure module, to get a fair comparison.
The results show that Memgraph is more than 5 times faster than NetworkX in performing the PageRank algorithm on a graph of Wikipedia articles dataset scale.
This dataset has 78,181 nodes and 310,227 relationships.
Memgraph's C++ implementation and highly optimized storage memory usage are key factors contributing to its superior performance.
PageRank is just one example of a graph algorithm that Memgraph offers out of the box.
For real-time use cases, such as credit card fraud detection, Memgraph shines due to its dynamic graph algorithms and ability to give newly updated results as soon as the graph object is consumed.
Sources
- https://memgraph.com/blog/pagerank-algorithm-for-graph-databases
- https://memgraph.github.io/networkx-guide/algorithms/centrality-algorithms/pagerank/
- https://stackoverflow.com/questions/43196867/using-pythons-networkx-to-compute-personalized-page-rank
- https://medium.com/web-mining-is688-spring-2021/graph-analysis-using-pagerank-and-networkx-for-twitter-account-beb7e239a71f
- https://memgraph.com/blog/who-ranks-better-memgraph-vs-networkx-pagerank
Featured Images: pexels.com