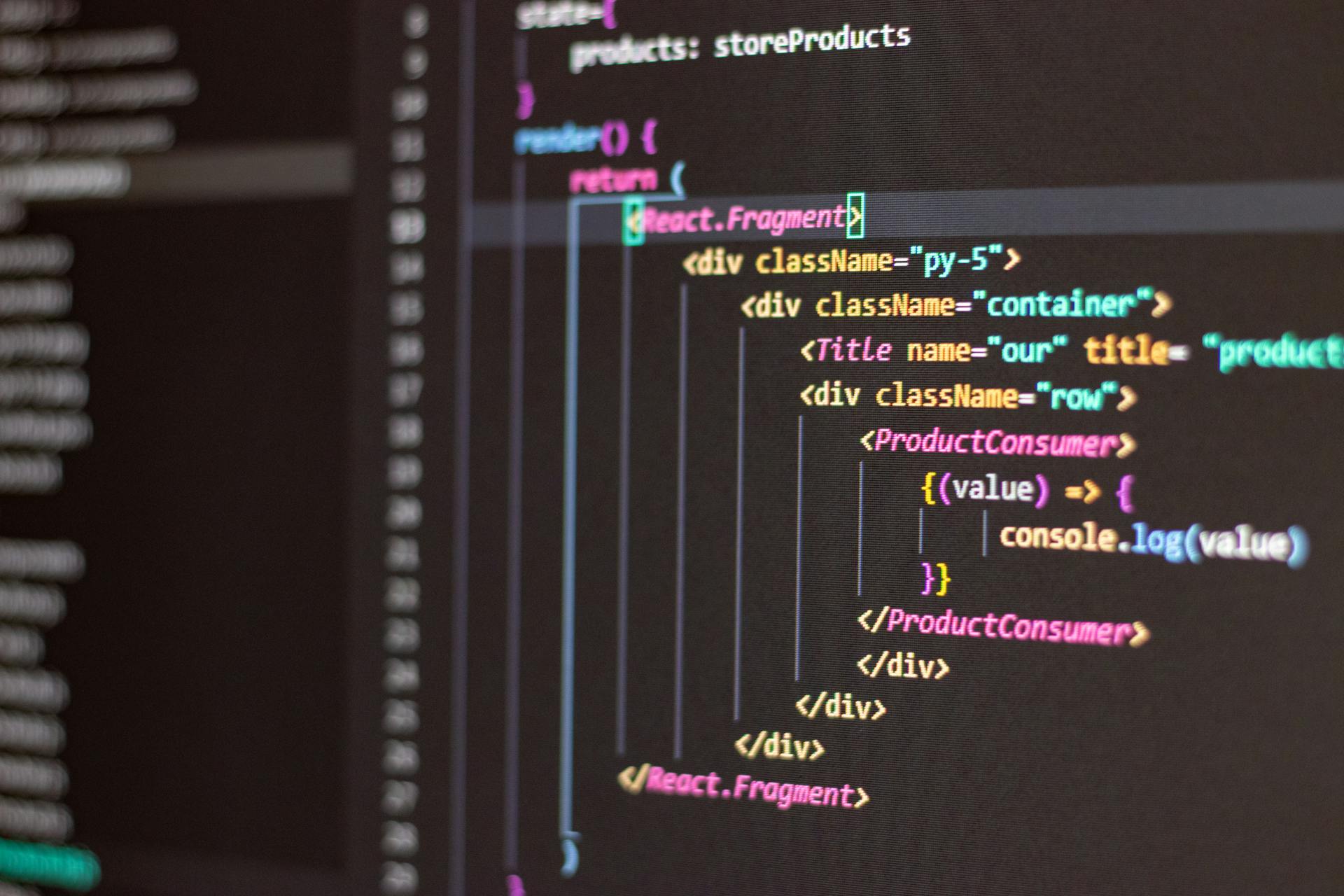
Cross-Origin Resource Sharing (CORS) is a fundamental concept in web development that allows web applications to make requests to a different origin than the one the application was loaded from. This is a crucial aspect of building modern web applications.
CORS is a security feature implemented in web browsers to prevent malicious scripts from making unauthorized requests on behalf of the user. By default, browsers only allow requests to be made to the same origin as the one the application was loaded from.
The CORS protocol relies on HTTP headers to determine whether a request should be allowed or blocked. The most common headers used in CORS are Access-Control-Allow-Origin, Access-Control-Allow-Methods, and Access-Control-Allow-Headers.
Why CORS is Needed
CORS is needed because it allows you to access endpoints from other origins without being blocked by cross-origin restrictions. This is especially useful when you have an application hosted on a different domain that needs to access your Next.js API routes.
If you try to access endpoints from another origin without CORS, your requests will be blocked by the browser. You need to enable CORS to allow cross-origin requests for specific origins.
Enabling CORS in your Next.js application is particularly important for modern web applications that rely on third-party services for functionalities like authentication, data storage, and more. This allows you to fetch data from external APIs and interact with resources on different domains seamlessly.
Readers also liked: Next Js Fetch Data save in Context and Next Route
Prerequisites and Setup
To get started with configuring CORS in Next.js, you'll need to meet some prerequisites. Node.js v18+ needs to be installed on your machine, and you'll also need a Next.js v13+ app.
You can use either the Pages Router or the App Router for your project, as the proposed solutions will work in both scenarios. To follow this tutorial, it's assumed that all API routes in your Next.js project are under the /api path.
Here are the specific prerequisites to keep in mind:
- Node.js v18+ installed on your machine
- A Next.js v13+ app
NextJS Environment Setup
To get started with NextJS, you'll need to run two services: NextJS as an API and a simple Client-Side application that makes HTTP requests to the API.
You'll need Node.js v18+ installed on your machine to set up NextJS. This is a must-have for any NextJS project.
NextJS v13+ is required for this setup. Make sure your project meets this prerequisite before proceeding.
You can use either the Pages Router or the App Router for your Next.js project, and the proposed solutions will work in both scenarios.
All API routes in your Next.js project should be under the /api path. This is the assumption we'll be working with from now on.
Here's an interesting read: Next Js Userouter
Prerequisites for Configuration
To get started with configuring CORS in your Next.js application, you'll need to meet some prerequisites. Node.js v18+ installed on your machine is a must, so make sure you have that covered.
A Next.js v13+ app is also required. Don't worry if your project is based on the Pages Router or the App Router, the solutions will work in both scenarios.
You'll also want to assume that all API routes in your Next.js project are under the /api path.
Here are the specific requirements you need to meet:
- Node.js v18+
- Next.js v13+
By meeting these prerequisites, you'll be well on your way to configuring CORS in your Next.js application.
Configuring CORS
Configuring CORS is a crucial step in enabling cross-origin requests in your Next.js application. To start, you'll need to meet the prerequisites, which include having Node.js v18+ installed on your machine and a Next.js v13+ app.
You can manually set the CORS headers in Next.js by using the headers key in next.config.js. This allows you to specify custom HTTP headers for incoming requests. The source must follow a specific syntax, and if an incorrect path regex is used, Next.js will throw an error.
The most common approach to CORS is to set it up globally on all API endpoints. There are three methods for using CORS in Next.js, which include using the headers config, a Vercel configuration file, and the middleware solution.
To handle more complex CORS setups, you can use asynchronous functions to dynamically determine CORS settings based on the request. You can also set up conditional logic within your middleware or directly within your route handlers.
Take a look at this: Auth Middleware Nextjs
The Access-Control-Allow-Origin header specifies the origin that is allowed to access the resource. You can set it to a specific origin or use * to allow all origins. The access-control-allow-methods header specifies which HTTP methods are permitted when accessing the resource.
Here's a summary of the CORS headers:
To handle different domains and routes, you can set up conditional logic within your middleware or directly within your route handlers. You can also use the crossOrigin configuration option to specify how the resource should be fetched with a CORS request.
Headers and Policies
The headers config in Next.js is an async function that must return an array of objects. This function is used to set the CORS headers manually in the next.config.js file.
You can set the CORS headers to a specific origin or use * to allow all origins. The access-control-allow-methods header specifies which HTTP methods are permitted when accessing the resource, ensuring that only certain types of requests are allowed.
Here's an interesting read: Next Config Js
The access-control-allow-headers header specifies the headers that can be used when making the actual request. The access-control-expose-headers header lets you whitelist headers that clients can access.
The access-control-max-age header specifies how long the results of a preflight request can be cached. By setting up these headers, you can ensure that your Next.js application can handle cross-origin requests securely and efficiently.
The Strict-Origin-When-Cross-Origin policy provides more privacy and security by sending the full URL only when the origin of the request matches the origin of the resource. This policy can be set in the headers section of your Next.js application.
To set the Access-Control-Allow-Origin header, you can specify the allowed origin in the headers config. This is especially useful when dealing with multiple domains and external APIs.
The CORS headers can be configured in a Next.js app deployed on Vercel by initializing a vercel.json file in the root folder of your project. The headers key has a similar syntax to the equivalent one in next.config.js, but with a different format for the source field.
To enable CORS in actual GET and POST requests, ensure that you set the appropriate CORS headers in your response. When fetching data from your Next.js API routes that have CORS enabled, ensure your client-side requests include the necessary headers.
Additional reading: Next Js Header
Middleware and Implementation
To implement CORS in Next.js, you can use middleware. This is the recommended approach according to Example 8: CORS Fix Option 3— Middleware (Recommended). By using middleware, you can configure CORS settings globally for all API endpoints.
You can create a new file in the root of your project called middleware.ts. This is where you'll define your CORS middleware. For example, you can add the following code to your middleware.ts file:
```typescript
import { NextApiRequest, NextApiResponse } from 'next';
const corsMiddleware = async (req: NextApiRequest, res: NextApiResponse) => {
res.setHeader('Access-Control-Allow-Origin', '*');
res.setHeader('Access-Control-Allow-Methods', 'GET, POST, PUT, DELETE');
res.setHeader('Access-Control-Allow-Headers', 'Content-Type, Accept');
return next();
};
export default corsMiddleware;
```
This middleware sets the necessary CORS headers for all API endpoints. You can customize the headers to fit your specific needs.
Here are the available CORS headers that you can set:
- Access-Control-Allow-Origin: specifies the origin that is allowed to access the resource
- Access-Control-Allow-Methods: specifies which HTTP methods are permitted when accessing the resource
- Access-Control-Allow-Headers: specifies the headers that can be used when making the actual request
- Access-Control-Allow-Credentials: indicates whether the request's response can be exposed
- Access-Control-Expose-Headers: lets you whitelist headers that clients can access
- Access-Control-Max-Age: specifies how long the results of a preflight request can be cached
By using middleware, you can easily enable CORS for all API routes in your Next.js application.
Sources
- https://codingwithmanny.medium.com/3-ways-to-configure-cors-for-nextjs-13-app-router-api-route-handlers-427e10929818
- https://blog.logrocket.com/using-cors-next-js-handle-cross-origin-requests/
- https://www.dhiwise.com/post/nextjs-cors-implementing-cross-origin-resource-sharing
- https://www.sanity.io/docs/connect-your-content-to-next-js
- https://www.getfishtank.com/insights/how-to-resolve-no-access-control-allow-origin-error-for-custom-fonts-in-cms-when-using-nextjs
Featured Images: pexels.com