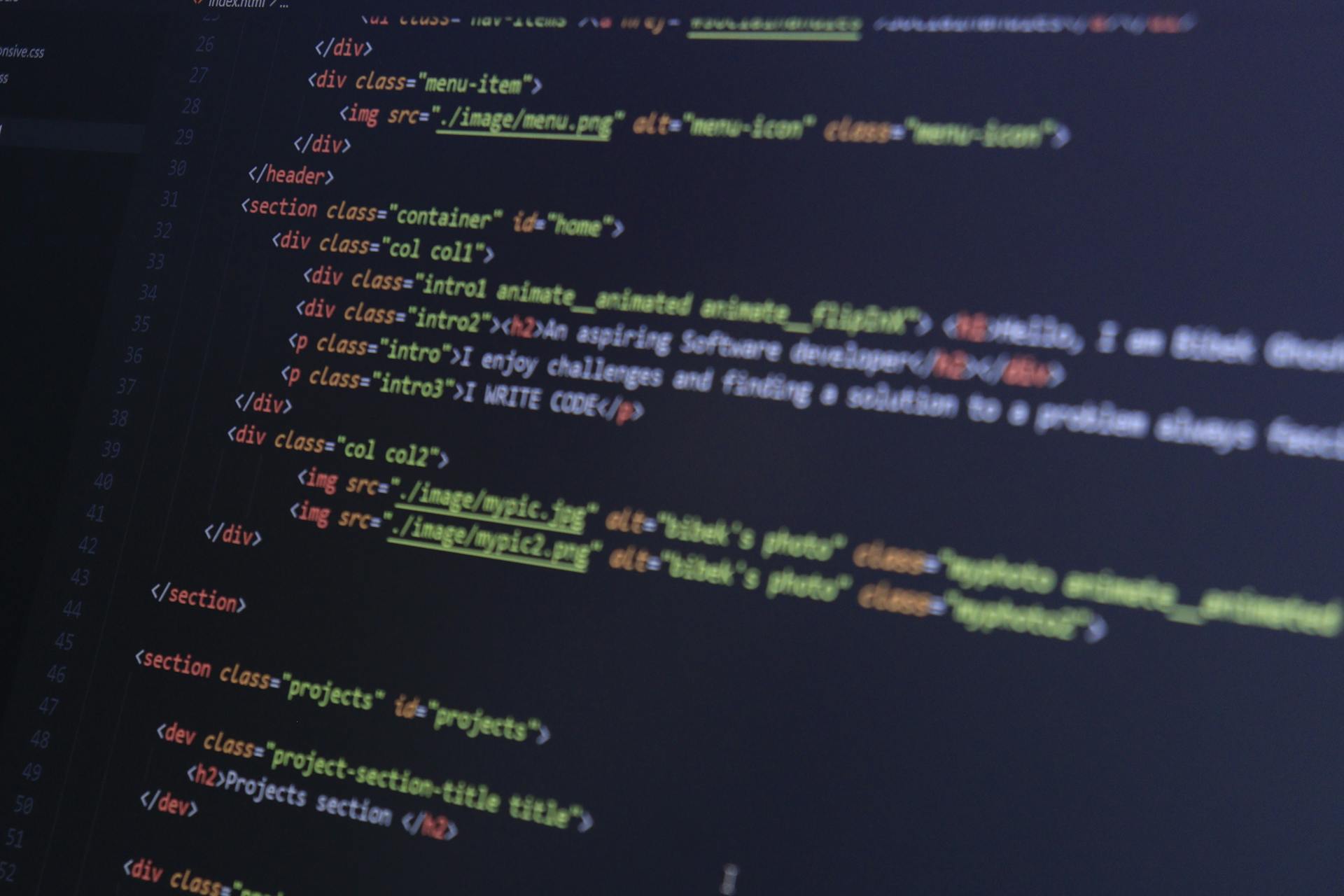
If you're a Next.js developer, you're likely no stranger to the frustration of dealing with no-CORS issues. In this article, we'll walk through a step-by-step guide to help you overcome these problems.
The root cause of no-CORS issues in Next.js lies in the way the framework handles requests to external APIs. Specifically, it's related to the Content Security Policy (CSP) and the way Next.js sets the Access-Control-Allow-Origin header.
To start solving no-CORS problems, you need to understand the different types of requests Next.js makes to external APIs. These include GET, POST, PUT, and DELETE requests, each with its own set of challenges and workarounds.
The solution to no-CORS issues often involves modifying the Next.js configuration files, specifically the next.config.js file. This file contains settings that control how Next.js behaves, including its handling of requests to external APIs.
For your interest: Nextjs Server Actions File Upload
Configuring CORS
Configuring CORS is a crucial step in resolving nextjs no-cors issues.
Cross-Origin Resource Sharing (CORS) is an HTTP-header based mechanism that allows a server to indicate any origins other than its own from which a browser should permit loading resources.
If you're working with NextJS 13, you'll notice that there are changes that make it harder to get up and running with existing libraries, such as nextjs-cors.
You can configure CORS in three ways: using Next Config, Route Handler, or Middleware.
One way to get things fixed quickly is to apply a blanket statement over all endpoints through the NextJS config file.
However, this method has a downfall: you can't dynamically change values if you want to allow more than one allowed origin.
Another option is to create CORS configurations directly in the route itself, which allows for more specific configurations but is siloed and doesn't provide a wider configuration to apply to many endpoints.
The recommended method is to use Middleware, which is the best of both worlds: it applies to multiple endpoints and allows for further customization through environment variables.
You might enjoy: Apply Css Nextjs
CORS Fixes
Cross-Origin Resource Sharing (CORS) issues can be frustrating to deal with.
If you're experiencing CORS issues with your NextJS 13 app router, there are three ways to configure CORS to allow resource sharing between your backend API and frontend.
One of the quickest ways to fix CORS issues is to apply a blanket statement over all endpoints through the NextJS config file.
However, this option has a downfall: you can't dynamically change values if you want to allow more than one allowed origin.
Creating CORS configurations directly in the route itself is another option.
This method allows you to be specific about which endpoint gets specific CORS configurations and leverage environment variables to switch out values without deploying hard-coded values.
However, this option is siloed and doesn't provide a wider configuration to apply to many endpoints.
The recommended method is to use middleware, which is the best of both worlds: applying to multiple endpoints and allowing for further configuration through environment variables.
To use middleware, you'll need to create a new file called middleware.ts and add CORS configurations to your .env file.
You might like: Nextjs Middleware Firebase Auth
Handling Requests
In a Next.js application, handling requests is crucial when dealing with no-CORS policies. The browser will block requests to external APIs due to security restrictions.
To bypass this restriction, you can use the `fetch` API with the `mode` option set to `cors`. This allows the browser to make requests to external APIs.
However, this approach has its limitations. The `cors` mode only works if the external API allows CORS requests.
In some cases, you may need to make requests to external APIs without using the `fetch` API. One way to achieve this is by using the `XMLHttpRequest` object.
The `XMLHttpRequest` object provides a way to make requests to external APIs without relying on the `fetch` API. However, it's not as modern or efficient as the `fetch` API.
If you're using a library like Axios, you can configure it to make requests to external APIs without using the `fetch` API. This can be useful if you need to make requests to multiple external APIs.
See what others are reading: Using State in Next Js
Sources
- https://nextjs.org/docs/pages/api-reference/next-config-js/headers
- https://codingwithmanny.medium.com/3-ways-to-configure-cors-for-nextjs-13-app-router-api-route-handlers-427e10929818
- https://github.com/vercel/next.js/discussions/47933
- https://stackoverflow.com/questions/65058598/nextjs-cors-issue
- https://www.dhiwise.com/post/nextjs-cors-implementing-cross-origin-resource-sharing
Featured Images: pexels.com