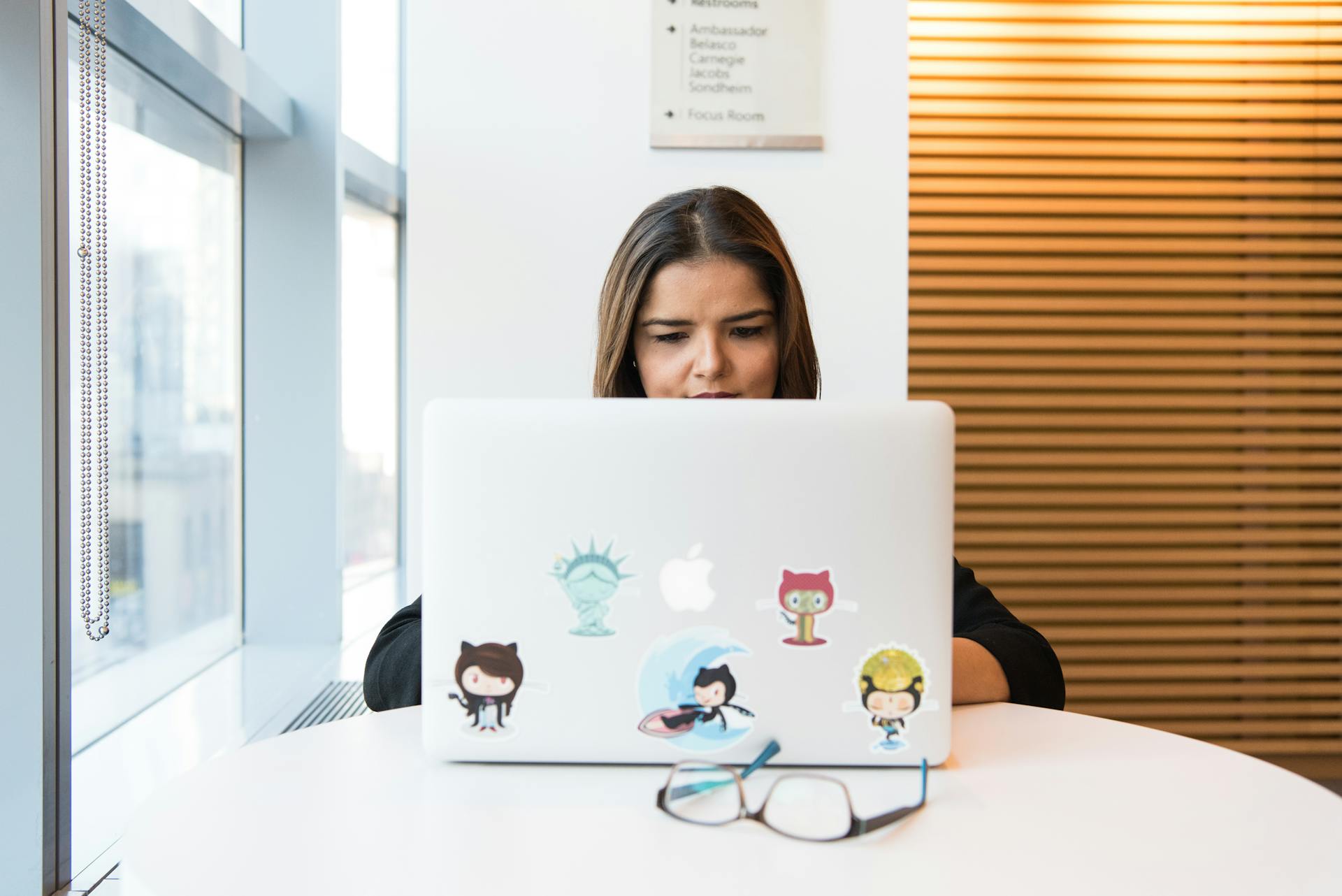
To get the most out of Next JS Module Federation, it's essential to understand the best practices and guidelines. This powerful feature allows you to break down large applications into smaller, independent modules, making it easier to manage and scale your codebase.
Next JS Module Federation is built on top of the Module Federation plugin for Webpack, which enables seamless sharing of code between modules. This is particularly useful for applications that require a high degree of customization and flexibility.
By using Next JS Module Federation, you can create a more modular and maintainable codebase, reducing the complexity and overhead associated with large-scale applications. This approach also allows for easier collaboration and reuse of code across different projects.
To get started with Next JS Module Federation, you'll need to set up a federated application, which involves creating a shared manifest file that outlines the modules and their dependencies.
Worth a look: Next Js Css Modules
Getting Started
Before you start using Module Federation with Next.js, make sure you have the necessary foundation in place. You can refer to the checklist provided to get started.
To begin with, ensure you have a solid understanding of Next.js. Next.js is a popular React-based framework for building server-side rendered (SSR) and statically generated websites.
Having a grasp of Next.js fundamentals will make it easier to implement Module Federation. This is because Module Federation builds upon the existing Next.js architecture.
Next, familiarize yourself with the concept of Module Federation. It's a feature that allows you to split your application into smaller, independent modules that can be reused across multiple projects.
With a solid foundation in Next.js and Module Federation, you'll be well on your way to implementing it in your project.
Next.js Concepts
Server-side rendering, static site generation, and API routes are all fundamental concepts you should be familiar with when working with Next.js.
If you're not already familiar with these concepts, take some time to learn about them, as they form the building blocks of a Next.js application.
Module Federation is another key concept to grasp, particularly its benefits in enabling micro-frontends architecture. This approach allows for independent development and testing of remote applications, which can be consumed by a host application.
On a similar theme: Can Nextjs Be Used on Traditional Web Application
With Module Federation, you can import components from remote applications, making it easier to manage dependencies and coordinate development efforts.
Here are some benefits of importing a single module per page:
- Simplified Integration: Importing a single module per page simplifies the integration process, as there are fewer dependencies to manage and coordinate.
- Clear Responsibility: Each remote application is responsible for a specific page or functionality, resulting in a clear separation of concerns and making it easier to understand and maintain the overall application.
- Faster Load Times: Loading a single module per page can potentially reduce the amount of data fetched and processed by the browser, leading to improved performance and faster load times.
- Enhanced Autonomy: Development teams can work independently on their respective pages or micro frontends without affecting other parts of the application, fostering better collaboration and efficient development processes.
- Easier Updates: With a single module per page, updates or changes can be made in isolation without impacting other pages, streamlining the deployment process.
To avoid hydration errors, use React.lazy instead of next/dynamic for lazy loading federated components.
Setup and Configuration
To set up and configure Next.js Module Federation, you'll need to create a new Next.js application for the remote module and update the next.config.js file to expose the desired modules.
First, create a new Next.js application by running the command `npx create-next-app remote`. This will act as the remote module that will be consumed by the Host application.
Next, install the `@module-federation/nextjs-mf` package using npm or yarn by running `npm install @module-federation/nextjs-mf` or `yarn add @module-federation/nextjs-mf`.
To configure the Host application, update the next.config.js file to import the NextFederationPlugin and configure it with the required options. Add the following code to your next.config.js file to import the NextFederationPlugin:
See what others are reading: Next Js Installation
```
const { NextFederationPlugin } = require('@module-federation/nextjs-mf');
```
Configure the NextFederationPlugin with the required options using the following example:
```
const nextConfig = {
reactStrictMode: true,
webpack(config, { isServer }) {
config.plugins.push(
new NextFederationPlugin({
name: 'host',
filename: 'static/chunks/remoteEntry.js',
remotes: remotes(isServer),
exposes: {
// Host app also can expose modules
}
})
);
return config;
},
};
```
Here's a breakdown of the configuration options:
By configuring the NextFederationPlugin in your next.config.js file, you've enabled Module Federation in your Host application.
Consuming Remote Modules
Consuming Remote Modules is a crucial step in Next.js Module Federation. You need to configure the Host application to consume the remote modules you've set up.
To do this, you'll need to expose the desired modules in the remote module. This is done by following a series of steps, with the fourth step being to consume the remote modules.
Now that we have set up the remote module and exposed the desired modules, we can configure the Host application to consume these remote modules. This is done by creating a new file, such as pages/index.js, and adding the necessary code.
By following these steps, you've successfully consumed the remote module in your Host application. In the next step, we'll discuss managing shared dependencies between the Host and Remote applications.
Related reading: Can Vercel Host Nextjs Servers
Managing Dependencies
Managing dependencies is a crucial step in building a micro-frontend architecture using Next.js and Module Federation. To avoid code duplication and optimize performance, you need to manage shared dependencies correctly.
In a micro-frontend architecture, multiple applications may share common dependencies, such as libraries or utilities. Without proper management, these shared dependencies can lead to code duplication, increased bundle sizes, and slower load times.
You can declare shared dependencies in the NextFederationPlugin configuration using the shared option. This option allows you to specify a list of modules that should be shared across different applications.
Here are some benefits of configuring shared dependencies:
To configure shared dependencies, you need to specify the shared option in the NextFederationPlugin configuration. This option allows you to declare a list of modules that should be shared across different applications. For example, you can configure the shared option to include the @emotion/, @chakra-ui/, and ./utils modules.
By following these best practices for managing shared dependencies, you can ensure a more efficient and scalable micro-frontend architecture using Next.js and Module Federation.
Testing and Troubleshooting
Testing your Module Federation setup locally is a crucial step before deploying it to production. In fact, you can test it locally by starting both applications, either by running npm start or yarn start in both the Host and Remote applications.
To verify remote module loading, open the Host application in your browser and check if the remote module is loaded correctly. You can use the browser's developer tools to inspect the network requests and ensure the remote module is being fetched from the correct URL.
Testing the functionality of the remote module is also essential. This includes verifying that the module is rendering correctly, handling events correctly, and interacting with other parts of the application as expected.
During local testing, you may encounter some common issues. To troubleshoot these issues, you can refer to the following solutions:
Specifically, if you encounter a "Module not found" issue, make sure to verify that the module is correctly exposed in the Remote application's next.config.js file. Similarly, if you encounter a "Module loading errors" issue, check the browser's console for error messages.
When testing your setup, you can use specific URLs to verify the rendering of components. For example, you can test the rendering of the Mario component by visiting localhost:3000/mario, and the Luigi component by visiting localhost:3000/luigi.
Here's an interesting read: Nextjs Pathname Server Component
Deploy
Deploying your Next.js Module Federation setup is a crucial step in making your application live and accessible to users. You've got to test your setup thoroughly before deploying.
To prepare for deployment, you need to test your setup, optimize your code, and configure your environment variables. Testing your setup ensures that everything is working as expected, while optimizing your code reduces file sizes and improves page load times. Configuring environment variables is also essential for a smooth deployment.
You have two primary options for deploying your Module Federation setup: server-side rendering (SSR) and client-side rendering (CSR). SSR involves deploying your Host and Remote applications on a server, allowing for server-side rendering of remote modules. CSR, on the other hand, involves deploying your Host and Remote applications on a client-side platform, such as a CDN or a cloud provider.
Before deploying, make sure you've completed the following steps:
- Tested your setup
- Optimized your code
- Configured your environment variables
To deploy your federated modules, follow these steps:
- Build your applications using npm run build or yarn build
- Upload your built applications to a CDN or cloud provider
- Configure routing for your Host and Remote applications
- Monitor and debug your deployment
By following these guidelines, you can successfully deploy your Module Federation setup and provide a seamless experience for your users.
Frequently Asked Questions
Do I need module federation?
Module federation is useful when you need to share third-party packages across multiple projects, reducing unnecessary downloads and improving performance
What is the difference between module federation and Monorepo?
Module Federation shares code between applications at runtime, while a Monorepo shares code at build time, making them distinct approaches to code reuse
Sources
- https://nextjsstarter.com/blog/nextjs-module-federation-quickstart-guide/
- https://alibek.dev/micro-frontends-with-nextjs-and-module-federation
- https://www.npmjs.com/package/@module-federation/nextjs-mf/v/8.1.10
- https://redhabayuanggara.hashnode.dev/module-federation-in-nextjs-microfrontend
- https://boro.im/blog/module-federation
Featured Images: pexels.com