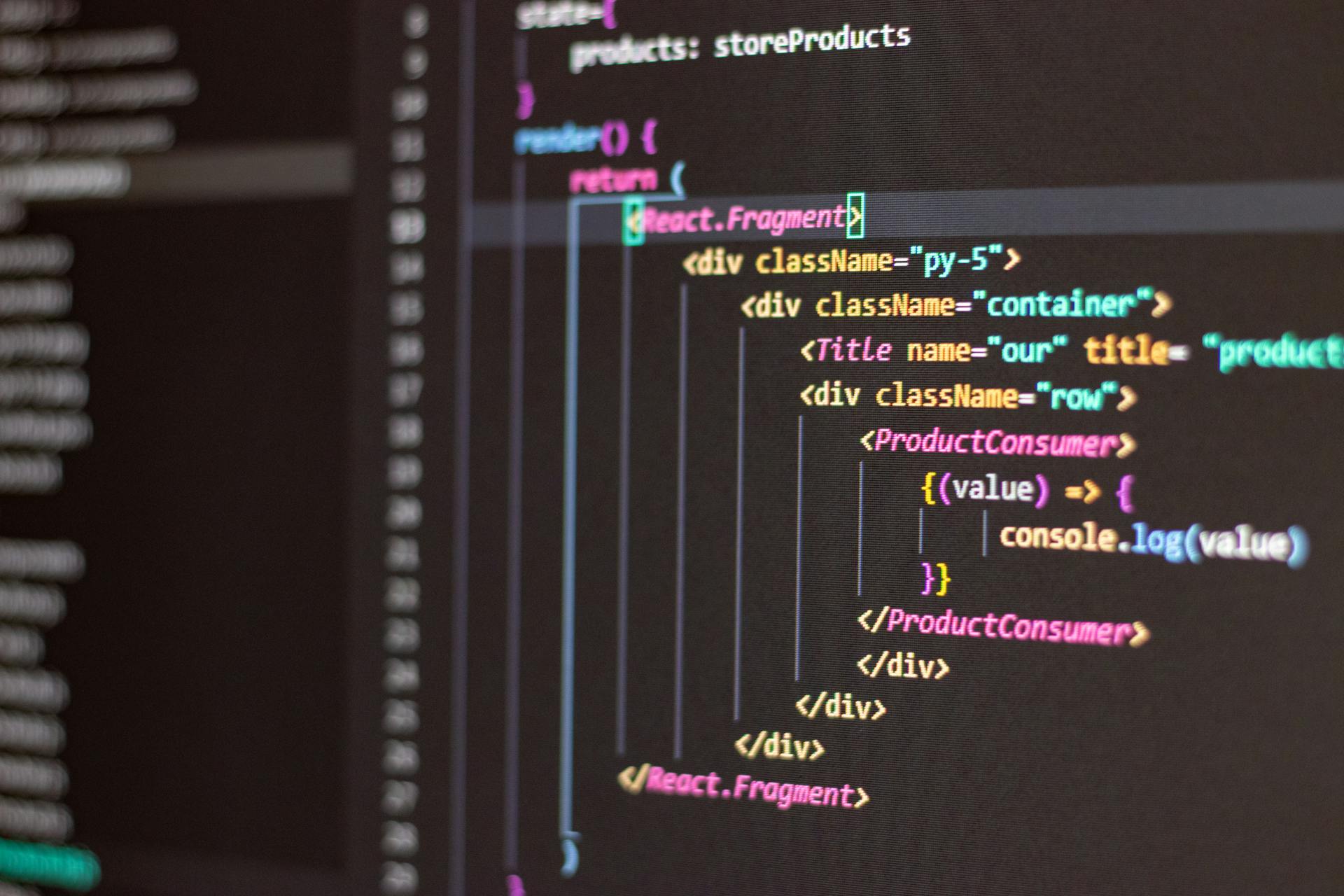
Next.js provides a built-in support for Service Workers, which enables offline support and performance enhancement for web applications.
Service Workers can cache resources, such as images and scripts, to improve page load times and provide a seamless user experience even when the user is offline.
By using a Service Worker with Next.js, you can also enable push notifications and background synchronization.
A Service Worker can cache resources and provide a fallback when the network is unavailable, which is especially useful for web applications that rely on APIs or third-party services.
Project Setup
To set up a Next.js project, run the command `npm create next-app my-app` and then open the project in your preferred code editor.
Once installed, you'll need to run `npm run dev` in the terminal window to see your service workers in action. This is where you'll notice the process of service workers being installed, activated, and used.
The demo website for your project is a great place to check the "Offline" option and see that the page still loads fine.
A unique perspective: Next Js Npm Install
Caching Assets
Caching assets is a game-changer for Next.js service workers, allowing you to provide an offline-first experience for your users. This can be achieved by using the Cache API to store assets in the browser cache, also known as precaching.
Caching all assets of the current page at once is a more straightforward approach, especially for Next.js projects. This can be done by using the clone() method of the Response object in the fetch event handler of the service worker.
You can specify scopes for a service worker with the scope property while registering the service worker, limiting the scope to a specific page or section of your application. This can be beneficial for large-scale applications with lots of assets.
Caching application assets on the user's device can improve performance by allowing for quicker access, especially in situations with unreliable internet connections. This can be done by including specific code in the service-worker.js file.
Consider reading: Next Js Build Collecting Page Data
To view the cached assets, open the Application tab in the developer tools and check the Cache Storage section. You can also simulate an offline experience by checking the Offline option under the Service Worker section and reloading the page.
Workbox is a popular tool for caching and serving files with service workers. It provides a routing and strategies module that can be used together to cache and serve files, making it a great option for large-scale applications.
Implementing
Implementing service workers in Next.js requires some basic setup. You should be familiar with using Next.js for development to follow along with this tutorial.
To build a basic Next.js application, you'll need to make it available offline using service workers. This involves registering a service worker in the global scope environment.
First, you need to check if the browser supports service workers. Fortunately, all modern web browsers do support service workers.
To register a service worker, you'll need to specify the file path where the service worker is located. This can be done in the activation phase.
Here are the steps to register a service worker:
- Check if the browser supports service workers.
- Specify the file path where the service worker is located.
You can include this code right after the registration process to install and activate the service worker. This involves listening to the install and activate events using JavaScript event listeners.
Check this out: Install Next Js
Enhancing Performance
Service workers can cache resources, which can improve the user experience by reducing the time it takes to load pages.
Using service workers, you can intercept requests and handle them on the client-side, reducing the load on your server and improving performance.
Service workers can provide offline support, allowing users to access your app even when they don't have an internet connection.
Implementing service workers can be complex and may require careful consideration of their benefits and drawbacks.
By implementing service workers, you can improve the performance of your Next.js app, but it's essential to weigh the potential benefits against the complexities involved.
Broaden your view: Next Js App Router Tailwind Spinner Loading Page
Offline Fallback
Offline fallback is a crucial feature for Next.js service workers, ensuring a smooth user experience even when the requested route isn't available.
The workbox-routing module has a setCatchHandler() function that allows you to handle route errors and set up an offline fallback. This is particularly useful for notifying users that a requested route isn't currently available.
The combination of Workbox and the Cache Storage API provides an offline fallback using a cache-only strategy.
During the service worker's install lifecycle, the offline-fallbacks cache is opened and the array of offline fallbacks is added to the cache.
The destination of the request that threw an error is determined, and the offline-fallbacks cache is opened.
If the destination is a document, the content of the offline fallback is returned to the user.
If that doesn't exist, or the destination isn't a document, an error response is returned.
You can extend this pattern not just for documents but for images, videos, fonts, really anything that you'd want to provide as an offline fallback.
Worth a look: Caching in Next Js
Use Cases and Drawbacks
Before diving into the details of using service workers in Next.js, let's take a look at some of the features it can be used for. Service workers in Next.js can be used for various purposes.
One of the key features is handling offline support, which is crucial for applications that need to work even when the internet connection is down. This is particularly useful for applications that require users to access information even when they're not connected to the internet.
Another feature is caching, which can improve the performance of your Next.js application. Service workers can cache frequently accessed resources, reducing the number of requests made to the server and improving the overall user experience.
However, there are some common concerns you should be aware of before diving into using service workers in your Next.js application.
Some of the drawbacks include the added complexity of implementing and maintaining service workers, which can be a challenge for developers. This is because service workers require a different approach to development and testing, which can be time-consuming and resource-intensive.
Use Cases
Service workers in Next.js can be used to enhance the user experience, particularly in areas with poor internet connectivity. This is because they can cache resources locally, reducing the need for repeated requests to the server.
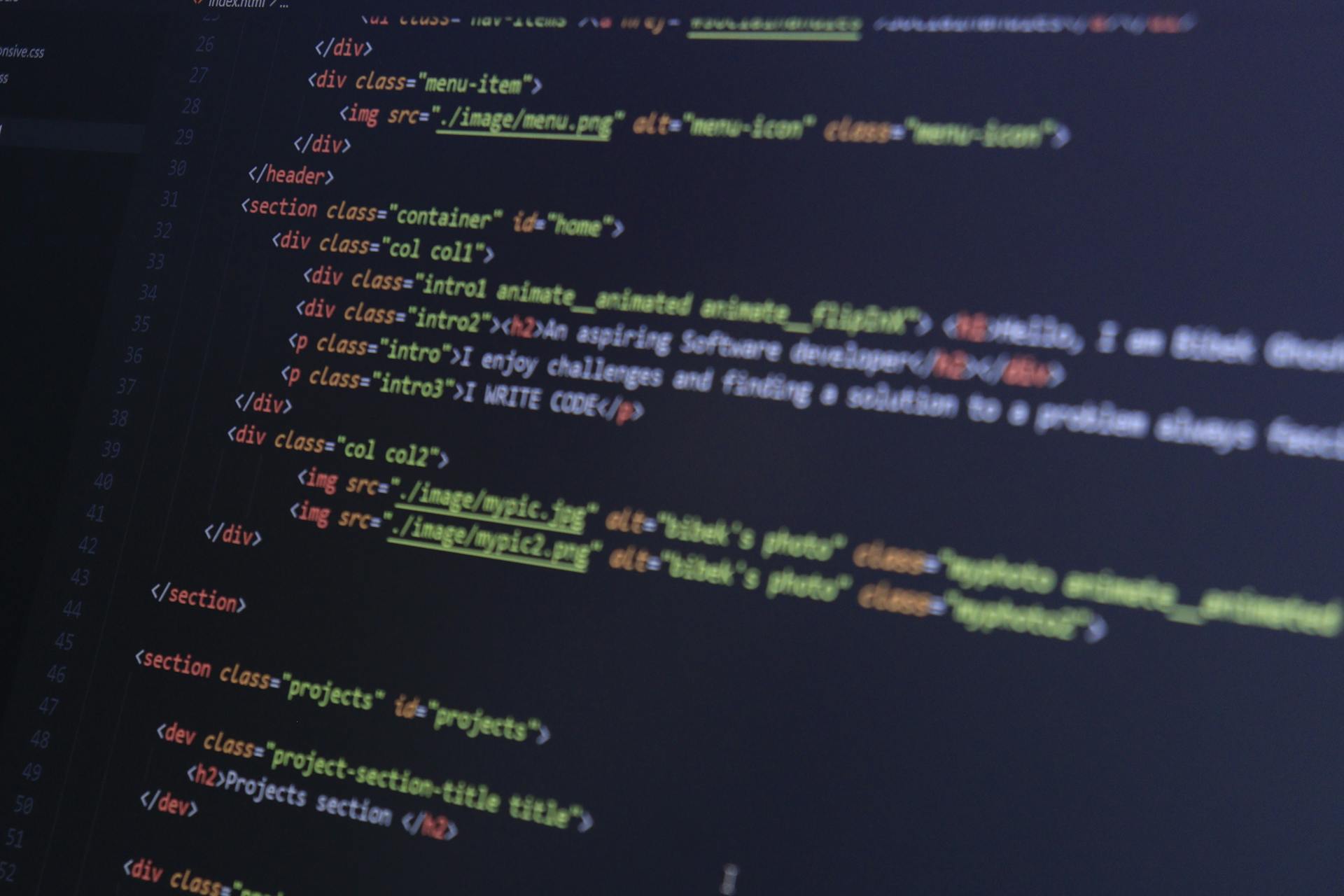
Before implementing service workers, it's essential to consider the features they can offer. Service workers can be used for caching, push notifications, and offline support.
One of the primary benefits of service workers is their ability to cache resources locally. This means that even when the user is offline, they can still access cached resources, such as static files and API responses.
Drawbacks to Using
Using service workers in Next.js can be a bit tricky, and there are some drawbacks to consider. There are some common concerns you should be aware of before diving in.
One of the main concerns is the potential for service workers to slow down your application. This is because service workers can intercept and cache requests, which can sometimes lead to delays.
Another concern is the complexity of implementing service workers. It can be a bit overwhelming, especially for developers who are new to the concept.
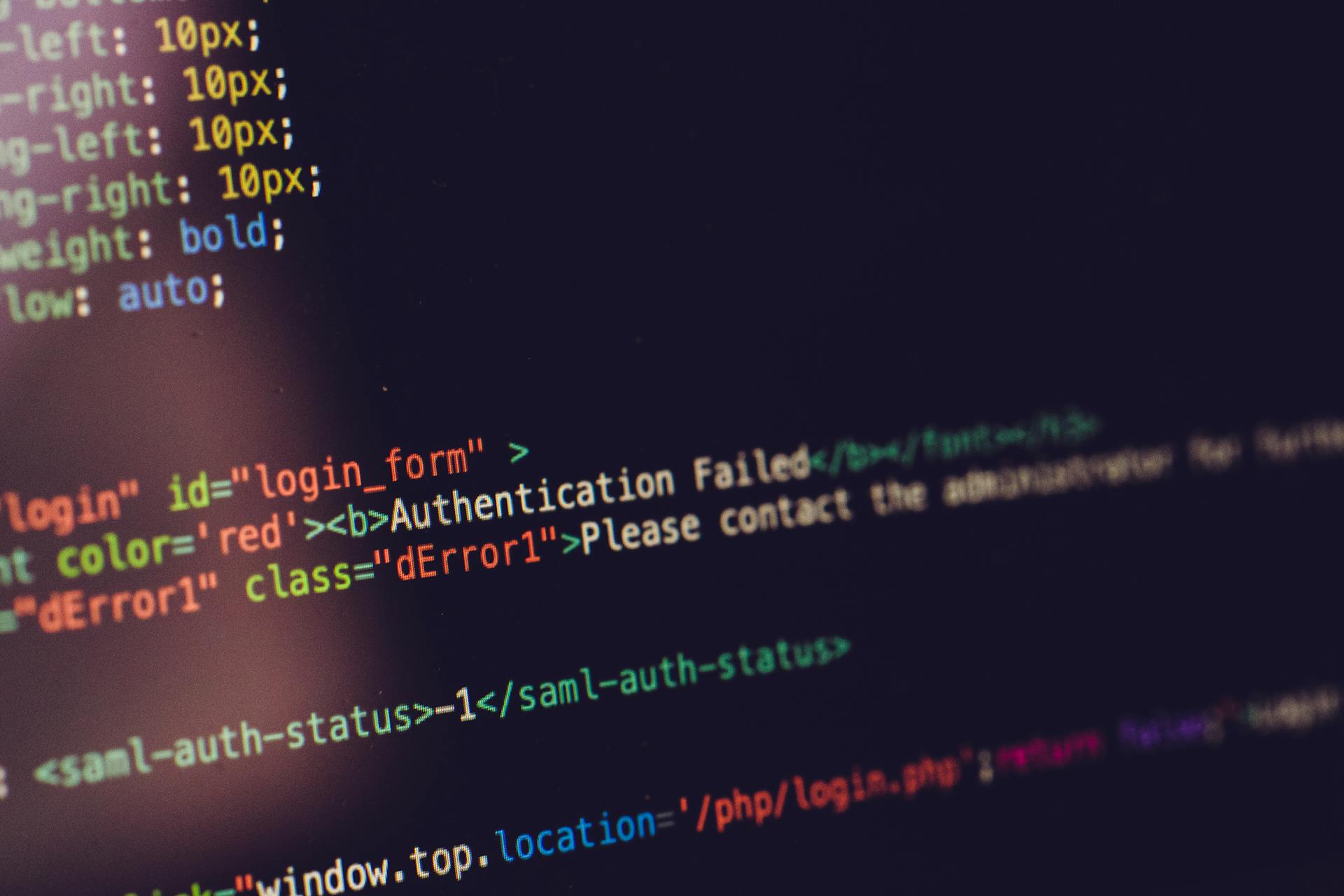
Service workers can also cause issues with caching, which can lead to stale data being displayed to users. This can be frustrating for users and damage your application's reputation.
In some cases, service workers can be difficult to debug, making it hard to identify and fix issues. This can be a major headache for developers.
Overall, while service workers can be a powerful tool, it's essential to carefully weigh the pros and cons before deciding whether to use them in your Next.js application.
Frequently Asked Questions
What does service worker js do?
Service workers enable offline access and boost page performance by acting as a proxy between browsers and servers. They improve reliability and speed up web page loading.
What is the difference between js Web Worker and service worker?
Web Workers offload CPU-intensive tasks, while Service Workers handle network requests and provide offline support. Essentially, they serve different purposes in optimizing web performance and user experience
Are service workers worth it?
Yes, service workers are worth it, as they enable offline functionality and improve the user experience on slow or unreliable networks. By caching assets, service workers provide a seamless experience even when users are disconnected from the internet.
Sources
- https://blog.logrocket.com/implementing-service-workers-next-js/
- https://developer.mozilla.org/en-US/docs/Web/Progressive_web_apps/Tutorials/js13kGames/Offline_Service_workers
- https://www.makeuseof.com/service-workers-nextjs-applications-how-integrate/
- https://web.dev/learn/pwa/workbox
- https://nextjs.org/docs/pages/building-your-application/optimizing/scripts
Featured Images: pexels.com