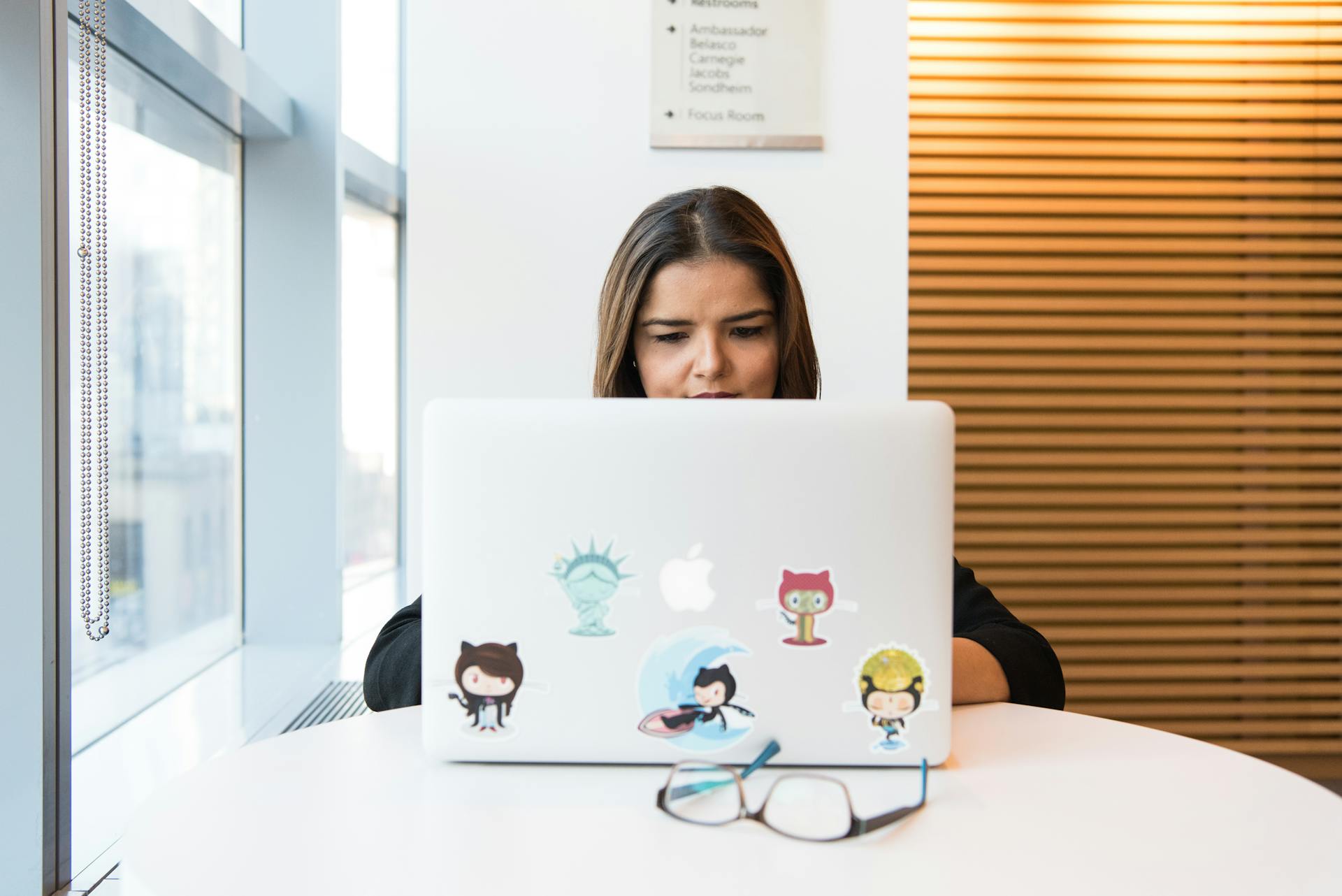
Using environment variables in Next.js is a great way to keep your application secure and scalable. You can store sensitive information like API keys and database credentials in environment variables, which are then injected into your application at runtime.
This approach helps to prevent sensitive information from being hardcoded into your application's code. For example, if you're using a third-party API, you can store your API key in an environment variable and then use it in your code.
Environment variables can also be used to configure your application for different environments, such as development, staging, and production. This makes it easier to manage different settings for each environment and ensures that your application behaves consistently across all environments.
Next.js provides a built-in way to handle environment variables through its `next.config.js` file.
Additional reading: Api Directory Nextjs
How to Use Environment Variables
Environment variables are a crucial part of Next.js development, and understanding how to use them is essential for a smooth and secure experience.
To add environment variables to a Next.js app, you'll need to create a file called `env.local` in your project's root directory. This file will load all the variables into the Node.js process.
Next.js automatically loads environment variables from the `env.local` file, making them available for use in the Node.js process.
You can reference other variables in the same `env.local` file using a dollar sign ($). For example, if you have a variable called `PROTECTED_URL` that contains a URL with query parameters, you can reference it like this: `process.env.PROTECTED_URL`.
To use environment variables in your Next.js app, you'll need to access them using `process.env.VariableName`. For example, if you have a variable called `API_URL`, you can access it like this: `process.env.API_URL`.
You can also use environment variables in your `getServerSideProps` function, but you'll need to use `global.process.env` instead of just `process.env`.
Next.js automatically loads environment variables based on the `NODE_ENV` setting, which can be set to `development`, `production`, or `test`. Make sure to correctly set the `NODE_ENV` in your environment to match the intended `.env` files.
Here's a quick rundown of the different environment files you can use in Next.js:
By following these best practices for managing environment variables, you can ensure that your Next.js app is secure, reliable, and easy to maintain.
Types of Environment Variables
Environment variables in Next.js can be broadly categorized into two types: runtime environment variables and build-time environment variables.
Runtime environment variables are set during the application's runtime, allowing for dynamic configuration and flexibility.
Build-time environment variables, on the other hand, are set during the build process, enabling static site generation and improved performance.
In Next.js, you can use the `process.env` object to access both types of environment variables, making it easy to manage and utilize them in your application.
Intriguing read: Nextjs Build Fails Environment Variables
Types of
In Next.js, you can have multiple environment variables to manage different configurations for your application. This is especially useful when you need to specify different settings for various environments.
You can think of environment variables like a set of instructions that tell your application how to behave in different situations. For example, you might want your application to behave differently in development mode compared to production mode.
Next.js allows you to manage three main application environments: development, production, and test. Each environment has its own set of environment variables.
Here are the three main application environments in Next.js:
Having multiple environment variables can help you manage your application's settings more efficiently. For example, you might want to use a different database connection string in development mode compared to production mode.
Naming Best Practices
Naming your environment variables effectively is crucial for maintaining security and functionality in your Next.js project.
To start with, any variable that needs to be accessed from the client-side code must be prefixed with NEXT_PUBLIC_. This is a non-negotiable rule that ensures only explicitly marked variables are exposed to the browser.
Using UPPER_CASE and underscores is the recommended naming convention for environment variables. This makes them easily identifiable in your code and separates them from regular variables.
Descriptive names are also essential for environment variables. This means ensuring that the names clearly describe their purpose, increasing code readability and maintainability.
Here's a quick recap of the naming best practices:
Default and Production Settings
When working with Next.js environment variables, it's essential to understand how to set up default and production settings. You can use a .env file to specify a default configuration for all three application environments.
This default configuration can be overridden by a .env.local file, which should be added to .gitignore to keep sensitive application data secure.
For production environment variables, use a .env.production file, which should also be kept in your project directory.
Curious to learn more? Check out: Why Use Next Js
Default
In a Next.js project, you can specify default environment variables using a .env file. This file serves as a configuration template for all three application environments.
The .env file should be added to .gitignore because it stores sensitive application data. This ensures that sensitive information is not accidentally committed to your repository.
You can override default variables in a .env.local file. This file has priority over the .env file, so if a variable is declared in both files, the .env.local value will be used.
Here's a quick rundown of the default environment variables and how they can be overridden:
By separating default and environment-specific variables, you can maintain clarity and prevent sensitive information from leaking between environments.
Production
In a production environment, you'll want to keep environment variables specific to production in a separate file.
Use a .env.production file for this purpose, and keep it in your project directory.
For live deployments, you can add environment variables through your dashboard, specifically in the Settings section where you can click on Environment Variables.
The UI for this should look similar to the one shown in the example, making it easy to manage your production environment variables.
Variable Configuration
Variable Configuration is a crucial aspect of managing environment variables in Next.js. You can configure environment variables in the Next.js Supabase Starter Kit by defining them in the .env file in the root of the apps/web package.
You'll find that most environment variables are defined in the apps/web/.env file, which is shared between environments. This is where you'll store configuration, paths, feature flags, and other non-sensitive information.
For local development, you can store secret keys and sensitive information in the .env.local file, which is not committed to Git. This keeps your sensitive info safe.
To manage different environments, you'll want to use .env.local for local development overrides, .env.development for development settings, .env.test for test configurations, and .env.production for production settings.
Here's a breakdown of the different .env files you'll use:
Conditional loading is also important, as Next.js automatically loads environment variables based on the NODE_ENV setting. Make sure to correctly set the NODE_ENV in your environment to match the intended .env files.
Storing Sensitive Information
Storing sensitive information is a crucial aspect of Next.js environment variables. You should never commit .env files to your version control system, as this can expose sensitive data publicly in repositories.
To avoid this, add your .env files to your .gitignore file. This will prevent sensitive information from being exposed.
You can use environment-specific files for different environments, such as local, development, and production. This allows you to tailor the visibility and accessibility of sensitive information as needed.
Server-side secrets are also a best practice, keeping sensitive data like database passwords or private API keys out of client-side code. This is especially true for Next.js, where it's best to handle secrets server-side whenever possible.
To securely store secrets for local development, use the env.local file. This file should be added to your .gitignore file to avoid checking it into a version control system like Git.
Here are some examples of sensitive information that should be stored securely:
Remember to generate a strong password for the SUPABASE_DB_WEBHOOK_SECRET variable. This should be a random string, such as a strong password generated using a password manager.
Accessing Environment Variables
You can access environment variables in your Next.js application through process.env on the server-side. This unrestricted access allows you to use both public and private variables for operations like fetching data or connecting to databases.
Only variables prefixed with NEXT_PUBLIC_ are exposed to the browser on the client-side, ensuring sensitive information remains secure. These variables are embedded during the build time and can be accessed anywhere in your client-side code.
To ensure consistency in your environment variables across all environments, use default values as a fallback in your code to handle missing or undefined environment variables gracefully.
Here's a quick rundown of how environment variables behave in different scenarios:
Environment Variable Files
In Next.js, environment variable files are a game-changer for managing settings across different environments.
These files are used for local development settings that shouldn't be shared publicly, and are loaded for all environments except when running Next.js in production mode. You can create a .env.local file for this purpose.
You can also create .env.development and .env.production files for development and production environment settings, respectively. These files get loaded when you run next dev and next build or next start, respectively.
The order of environment variable lookup in Next.js is as follows:
- process.env
- env.${NODE_ENV}.local
- env.local (env.local is not checked when NODE_ENV is test)
- env.${NODE_ENV}
- .env
This means that if you declare the same environment variable in multiple files, the environment variable declared in the lowest file in the above list wins.
Supabase and Feature Flags
To use Supabase, you'll need to add some environment variables. This will give your application the necessary settings to connect with Supabase's database.
The environment variables for Supabase are added separately from the feature flags. To enable or disable certain application features, you can toggle the values of the following feature flags:
Supabase
To set up Supabase, you'll need to add three environment variables. The first one is NEXT_PUBLIC_SUPABASE_URL, which is the URL of your Supabase project.
You can find this URL in your Supabase project settings. The second variable is NEXT_PUBLIC_SUPABASE_ANON_KEY, which is the anonymous key of your Supabase project.
This key is also found in your Supabase project settings. To complete the setup, you'll need to generate a secret key for SUPABASE_DB_WEBHOOK_SECRET.
This secret key should be a strong password, ideally generated using a password manager.
See what others are reading: Nextjs Url Parameter Is Not Allowed
Feature Flags
Feature Flags are a powerful tool in Supabase, allowing you to toggle certain application features on or off with ease.
To enable or disable features, you can simply toggle the values of the following feature flags:
- NEXT_PUBLIC_ENABLE_THEME_TOGGLE - hides the theme toggle, forcing a single theme
- NEXT_PUBLIC_ENABLE_PERSONAL_ACCOUNT_DELETION - prevents users from self-deleting their personal account
- NEXT_PUBLIC_ENABLE_PERSONAL_ACCOUNT_BILLING - enables or disables billing for personal accounts
- NEXT_PUBLIC_ENABLE_TEAM_ACCOUNTS_DELETION - prevents team accounts from self-deleting the account
- NEXT_PUBLIC_ENABLE_TEAM_ACCOUNTS_BILLING - enables or disables billing for team accounts
- NEXT_PUBLIC_ENABLE_TEAM_ACCOUNTS - disables team accounts
- NEXT_PUBLIC_ENABLE_TEAM_ACCOUNTS_CREATION - enables or disables the ability to create a team account
The starter kit supports two types of accounts, and to leave them both enabled, you can simply leave them as they are.
Payment and Billing Settings
You can control what users see when it comes to billing by setting NEXT_PUBLIC_ENABLE_PERSONAL_ACCOUNT_BILLING to true or false. This determines whether users can add their billing information for personal accounts.
If you want to allow team accounts to have billing, set NEXT_PUBLIC_ENABLE_TEAM_ACCOUNTS_BILLING to true. This will show the billing form to users.
To use Stripe as your billing provider, you need to set STRIPE_SECRET_KEY to your Stripe secret key. This is a required step if you're using Stripe.
A fresh viewpoint: Nextjs Set Hostname
Billing Provider
To set up your billing provider, you'll need to choose between Stripe and lemon-squeezy.
You can select the billing provider to use by setting the NEXT_PUBLIC_BILLING_PROVIDER variable to either stripe or lemon-squeezy.
If you decide to use Stripe, you'll need to set the STRIPE_SECRET_KEY variable to your Stripe secret key.
Stripe Publishable Key
To set up Stripe as your billing provider, you need to obtain a Stripe publishable key.
This key is a crucial piece of information that allows Stripe to process payments on your behalf. You can find your Stripe publishable key in the Stripe dashboard.
Once you have your Stripe publishable key, you need to set it as the value of the NEXT_PUBLIC_STRIPE_PUBLISHABLE_KEY variable. This ensures that Stripe can securely process payments for your application.
By following these steps, you can successfully integrate Stripe into your payment and billing settings.
Stripe Secret Key
To set up a Stripe secret key, you need to obtain your Stripe secret key from your Stripe account.
This key is required to securely connect your Stripe account to your application.
If you're using Stripe as your billing provider, you need to set this variable to your Stripe secret key.
You can find your Stripe secret key in your Stripe dashboard, under the "Developers" tab.
This key is essential for verifying and authenticating webhooks sent from Stripe to your application.
Make sure to keep your Stripe secret key secure and don't share it with anyone.
Email and Captcha Settings
Email and Captcha Settings are crucial for Next.js projects.
To use Nodemailer as your mailer provider, you need to set the EMAIL_HOST variable to your SMTP host.
If you want to protect your endpoints using Cloudflare's Captcha, you need to set both the NEXT_PUBLIC_CAPTCHA_SITE_KEY and CAPTCHA_SECRET_TOKEN variables.
Email Host
If you're using Nodemailer as your mailer provider, you need to set your EMAIL_HOST variable to your SMTP host.
You can find this information by checking your mailer provider's settings or documentation.
Your SMTP host is usually provided by your email service provider, such as Gmail or Outlook.
Make sure to update your EMAIL_HOST variable with the correct SMTP host to ensure your emails are sent successfully.
Captcha Site Key
To set up Cloudflare's Captcha, you'll need to obtain a Captcha site key and secret token. The Captcha site key is set using the NEXT_PUBLIC_CAPTCHA_SITE_KEY variable, while the secret token is set using the CAPTCHA_SECRET_TOKEN variable.
You'll need to replace these variables with your actual Captcha site key and secret token, which can be obtained from the Cloudflare dashboard. Make sure to keep these sensitive credentials secure.
If you're using Cloudflare's Captcha, it's essential to have both the site key and secret token set up correctly to ensure your endpoints are properly protected.
A unique perspective: Azure App Service Environment Variables Key Vault
Frequently Asked Questions
How to store environment variables in NextJS?
Store environment variables in NextJS using a .env file to load them, or prefix browser variables with NEXT_PUBLIC_ for bundling. This allows you to manage sensitive data securely and efficiently.
Are Next_Public environment variables safe?
Yes, Next_PUBLIC environment variables are safe as they are only exposed to the browser and embedded during build time, keeping sensitive information secure
Sources
- https://refine.dev/blog/next-js-environment-variables/
- https://www.dhiwise.com/post/how-to-manage-nextjs-environment-variables-for-better-security
- https://blog.logrocket.com/customizing-environment-variables-next-js-13/
- https://makerkit.dev/docs/next-supabase-turbo/environment-variables
- https://notes.dt.in.th/NextRuntimeEnv
Featured Images: pexels.com