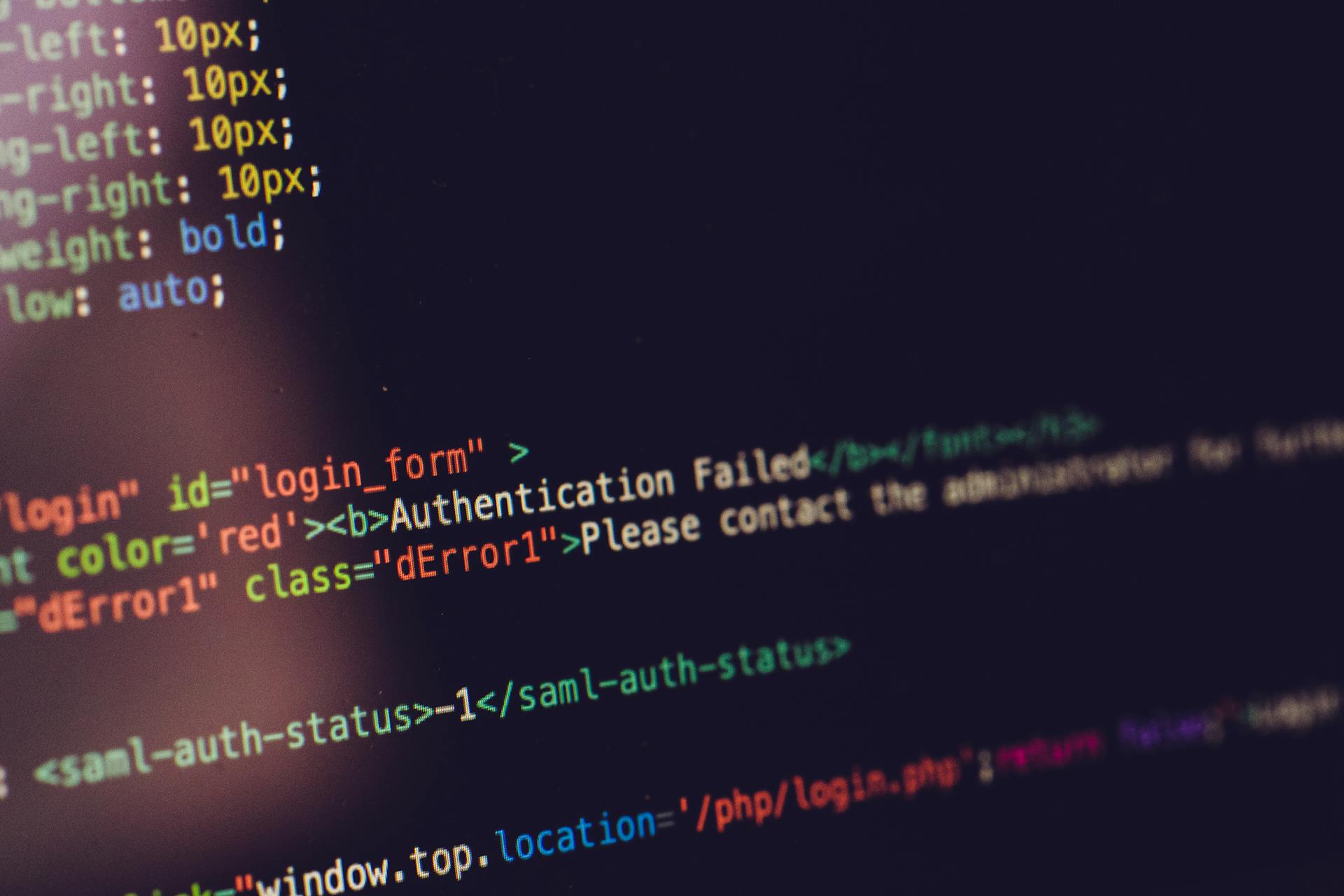
Nextjs Image Style is a powerful tool for optimizing images on the web. It allows you to use a single image and have it adapt to different screen sizes and devices.
By using the `image` component, you can easily add responsive images to your Nextjs project. This component takes care of resizing and compressing images for you, making it a huge time-saver.
The `layout` prop is particularly useful for controlling the layout of your images. For example, you can use it to create a grid of images that adapts to the screen size.
On a similar theme: Why Use Next Js
What Is Next.js Image Style?
Next.js Image Style is a powerful tool for displaying images on your website. It allows you to display a single image or responsive images with different variations optimized for different screen sizes.
The Next.js Image Component can be used to display a single image with no variants. This is useful when you only need to display a single image on your website.
Responsive images with different variations can be achieved by using the Next.js Image Component, which optimizes images for different screen sizes. This is especially useful for websites that need to cater to a wide range of devices and screen sizes.
See what others are reading: Css Image Responsive
Benefits of Optimization
Optimizing your images is a crucial step in improving the performance of your Next.js app. The Next.js Image component automatically optimizes images on-demand, serving them in the most efficient format based on the user's device and browser capabilities.
Using the Next.js Image component can significantly improve the loading times of your app, thanks to lazy loading, which loads images only when they enter the viewport. This can make a huge difference in user experience.
To minimize the performance impact of background images, consider optimizing their file size using tools like ImageOptim or TinyPNG. Compressing images without losing quality can make a big difference.
Choosing the right image format is also essential. WebP formats offer superior compression than conventional formats like PNG and JPG, but be sure to include fallbacks for browsers that don't support them.
Here are the key benefits of using the Next.js Image component for optimization:
- Automatic Image Optimization: Images are optimized on-demand based on user device and browser capabilities.
- Lazy Loading: Images are loaded only when they enter the viewport.
- Responsive Images: Images adapt to different screen sizes, providing an optimal experience across devices.
- Placeholder Support: A placeholder is displayed until the image is fully loaded.
Responsive Images
Responsive images are a crucial aspect of creating a seamless user experience in Next.js. You can use the built-in next/image component to implement responsive images, which automatically optimizes images for different screen sizes and resolutions.
The next/image component offers several benefits for image optimization, including automatic image optimization, lazy loading, responsive images, and placeholder support.
To make your image fully responsive, you need to set position: relative on its parent element, as well as either a width and height or an aspectRatio. This ensures that the aspect ratio of the image is preserved.
You can use the fill property to ensure your Next.js Image Component fills the parent container, but using fill alone will cause your image to stretch, and does not preserve the original aspect ratio. To make your image fully responsive, you need to set position: relative on its parent element, as well as either a width and height or an aspectRatio.
Here are some key properties to customize your images on the Next.js Image Component:
- src: Use the relative path of your image in the public directory or the full path to the image if it's stored remotely.
- alt: Add an alt attribute to provide a text description of the image.
- layout: Set the layout attribute to responsive to ensure your images adapt to different screen sizes.
- sizes: Use the sizes property to tell the browser what percentage of the viewport's width the image should fit, especially useful when the image's display size changes at different breakpoints.
Here's an example of how to use the sizes attribute directly on the Image component:
sizes="(max-width: 768px) 100vw, (max-width: 1200px) 50vw, 33vw"
Advanced Techniques
Next.js offers advanced techniques for enhancing images' responsiveness and visual appeal. These techniques include using placeholders to improve perceived performance.
Using placeholders allows you to serve a low-quality image initially, which can be replaced with the full-quality image once it's loaded, improving the user experience.
Next.js also supports implementing art direction to serve different images based on the device or context. This means you can serve a different image based on the screen size, device type, or even the user's location.
By implementing these advanced techniques, you can take your image style to the next level and provide a better experience for your users.
For more insights, see: Css Image Styling
Implementing Art Direction with Multiple Sources
Implementing Art Direction with Multiple Sources is a powerful technique that allows you to choose different images based on the user's screen size or other factors.
With Next.js, you can manually define srcSet to include different images for different viewport sizes. This is done by providing a list of sources and their intended viewport conditions.
The picture element is a key part of implementing art direction, as it wraps different source elements, each with a media attribute defining the condition under which that source should be used.
You can specify multiple image sources using the srcSet attribute, which is automatically handled by Next.js for different resolutions. However, you can also manually define srcSet to include different images for different viewport sizes.
The Image component is a fallback for environments without supporting the picture element, providing a reliable way to display images even when art direction isn't possible.
Blurring Techniques
Blurring Techniques can be a game-changer for image responsiveness. Next.js offers a powerful way to maintain visual stability as images load by using placeholders and blurring effects.
A blurred version of the image can be displayed while the full image is loading, creating a smooth visual transition. To achieve this, you must provide a blurDataURL, a small image or a base64-encoded string representing the larger image.
Next.js will enlarge and blur this small image to serve as the placeholder. This technique is particularly useful for improving perceived performance and visual appeal.
By using a placeholder prop set to 'blur' in the next/image component, you can implement a blur placeholder in your Next.js project. The blurDataURL prop holds a base64-encoded string of a small image, which is used as the placeholder until the full image is loaded.
Advanced Responsiveness Techniques
To take your Next.js app to the next level, you need to think beyond the basics of image responsiveness. This means using placeholders and blurring effects to improve perceived performance. You can also implement art direction to serve different images based on the device or context.
Implementing art direction is a great way to have more creative control over how images are presented. With Next.js, you can use the srcSet attribute to specify multiple image sources. This allows you to manually define srcSet to include different images for different viewport sizes.
For example, you can use the picture element to wrap different source elements, each with a media attribute defining the condition under which that source should be used. The Image component is a fallback for environments without supporting the picture element.
To make sure your background images scale and reposition correctly across different screen sizes, you can use several strategies. One approach is to set position: relative on the parent element, as well as either a width and height or an aspectRatio. This will preserve the original aspect ratio of the image.
If you want to ensure your images are fully responsive, you need to set the aspectRatio property of the Next.js Image Component. This will prevent your images from stretching and preserve their original proportions.
Performance Considerations
Implementing responsive images in a Next.js application is a great way to improve performance. Efficient image loading can significantly improve the speed and responsiveness of your web pages, contributing to a better user experience and potentially higher SEO rankings.
Serving images in modern formats like WebP or AVIF can offer better compression and quality than traditional formats. Next.js can automatically serve images in these formats using the next/image component.
Optimizing image sizes is crucial, as it only loads the largest necessary image for the user's device. Use the sizes attribute to inform the browser of the expected image size relative to the viewport.
Lazy loading is enabled by default in Next.js, which means images are only loaded as they approach the viewport. This reduces initial page load time and saves bandwidth.
You can also use placeholders, such as a blur-up technique, to maintain layout stability and provide a better user experience as images load. This can be especially useful for above-the-fold images.
Here are some key performance considerations to keep in mind:
- Serve images in modern formats like WebP or AVIF.
- Optimize image sizes using the sizes attribute.
- Implement lazy loading.
- Use placeholders to maintain layout stability.
- Cache images to serve them quickly to repeat visitors.
You can also prioritize critical images by using the priority attribute to ensure they are preloaded. This can improve the Largest Contentful Paint (LCP) metric, which is essential for performance.
Removing and Converting Images
Removing and Converting Images is a crucial step in Next.js Image Style.
You can use Image component to remove and convert images, which automatically optimizes images for web use.
The Image component can also handle image formats like JPEG, PNG, and WebP.
To convert an image, you can use the `blurDataURL` property, which automatically converts the image to a blurred version.
For example, you can use `blurDataURL` to convert an image to a blurred version, like this: `blurDataURL="data:image/png;base64,iVBORw0KGg..."`.
Remove
Removing images can be a bit of a challenge, but it's a crucial step in the process of converting them.
You can remove images from a document by deleting the image object, which can be done by selecting the image and pressing the delete key.
Removing images can also be done by using the "Remove" feature in image editing software, such as Adobe Photoshop.
The "Remove" feature in image editing software can be used to remove unwanted objects or blemishes from an image.
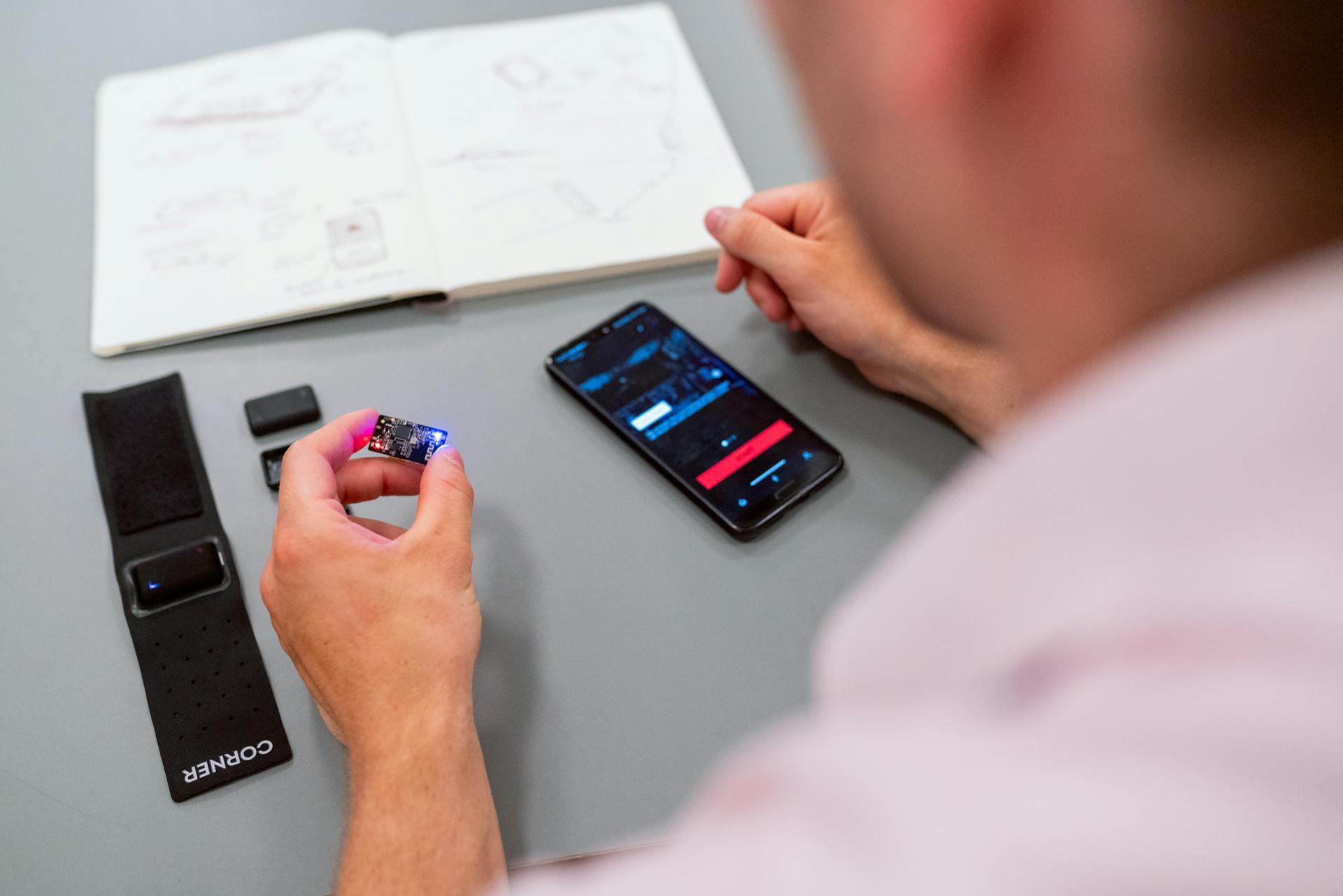
In some cases, removing images can also involve resizing or cropping the image to fit a specific size or shape.
Removing images can be a time-consuming process, especially if you're working with large or complex images.
However, with the right tools and techniques, you can remove images quickly and efficiently.
The goal of removing images is to create a clean and distraction-free image that is ready for conversion.
Convert to Modern Formats
Converting images to modern formats can significantly reduce file sizes without compromising image quality. This is especially useful for web development, where smaller file sizes can lead to faster page loads.
You can specify your preferred formats in your next.config.js file, and your images will be served in that format. For example, modern formats like WebP and AVIF are great options.
These formats are designed to provide high-quality images at smaller file sizes, making them ideal for web use.
Project Setup and Dependencies
To set up a Next.js project, you'll need to install Next.js and its dependencies. This can be done by creating a new Next.js application in your project directory with a simple command that scaffolds a new project with all the necessary files and configurations.
The installation command will take care of everything, so just sit back and wait for it to complete.
Once the installation is complete, navigate to your project directory to start working on your application.
Next.js comes with the Image Optimization API, so you can start serving optimized images immediately without additional packages.
See what others are reading: Speed up a Next Js Application Fetching Data
Sources
- https://www.dhiwise.com/post/how-to-add-a-background-image-in-nextjs-a-comprehensive-guide
- https://nextjs.org/docs/pages/api-reference/components/image
- https://www.dhiwise.com/post/how-to-implement-next.js-responsive-image-features
- https://nextjs.org/docs/pages/building-your-application/optimizing/images
- https://www.contentful.com/blog/nextjs-image-component/
Featured Images: pexels.com