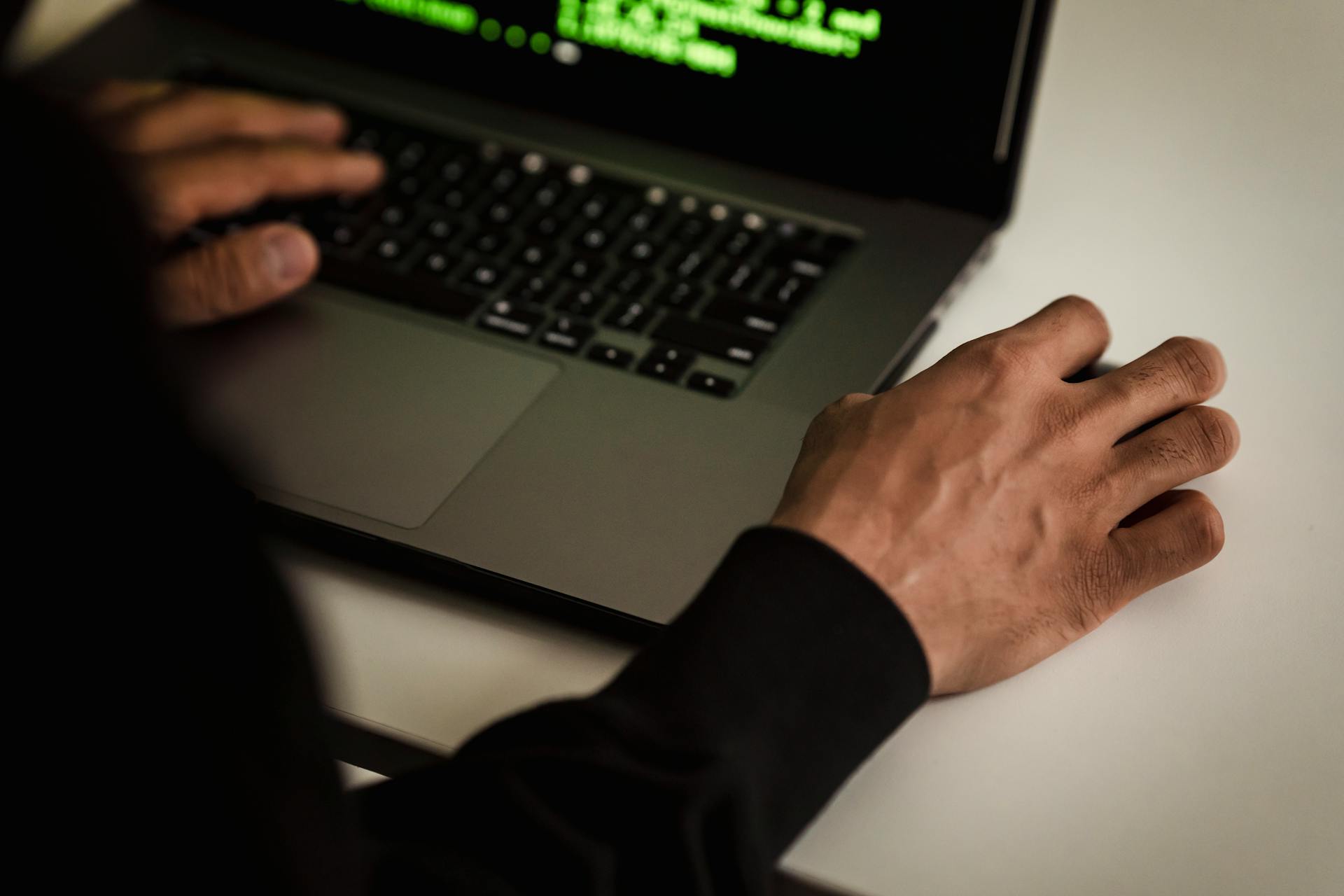
Configuring and optimizing Next.js routes is a crucial step in building a robust and scalable application. This guide will walk you through the process of setting up routes in Next.js using the `nextjs` library.
To create a new route, you can use the `getStaticProps` method in a page component, as shown in the example: `export async function getStaticProps() { return { props: { title: 'Home' } }; }`. This method allows you to pre-render pages at build time.
Route configuration in Next.js involves defining the routes for your application. You can do this by creating a new file in the `pages` directory, such as `pages/about.tsx`. This file will be automatically recognized as a route by Next.js.
The `nextjs` library provides several features for optimizing routes, including support for dynamic routing and internationalization. By leveraging these features, you can create a more flexible and user-friendly routing system.
Next.js App Router
The Next.js App Router presents some unique challenges for using Redux properly. This includes per-request safe Redux store creation, where the Redux store should be created per request and not shared across requests.
The App Router architecture supports aggressive server caching, which means the ideal store architecture should be compatible with this caching. This is a key consideration for developers building Next.js applications with the App Router.
To handle these challenges, developers can use the next-redux-wrapper library, which integrates a Redux store with the App Router data fetching methods like getServerSideProps.
Next.js App Router Mistakes and Fixes
The App Router in Next.js can be a bit tricky to set up, especially for those new to the framework. One common mistake is not properly configuring the routes, leading to errors like "No matching route found".
Make sure to define your routes correctly, with a unique path for each page. This will prevent the App Router from getting confused and resulting in a 404 error.
Routing conflicts can arise if you have two or more pages with the same path. To avoid this, use a unique path for each page, like "/about" and "/about/team".
Don't forget to update your existing code to use the new App Router syntax. This includes replacing "getStaticProps" with "useAppParams" and "useRouter" with "useAppRouter".
If you're using a custom layout, ensure it's properly set up to work with the App Router. This may involve updating your layout component to use the new "useAppRouter" hook.
Remember, the App Router is designed to be flexible and scalable, so take advantage of its features to simplify your routing setup.
Introduction
Welcome to the world of Next.js App Router! This architecture presents some unique challenges for using Redux properly, including per-request safe Redux store creation.
Next.js servers can handle multiple requests simultaneously, so the Redux store should be created per request and not shared across requests.
A Next.js application is rendered twice: first on the server and again on the client. This means the Redux store will have to be initialized on the server and then re-initialized on the client with the same data to avoid hydration issues.
To achieve this, the store should be compatible with server caching, a feature supported by recent versions of Next.js, especially those using the App Router architecture.
Next.js applications support a hybrid model for client-side routing, where the first page load gets an SSR result from the server, and subsequent page navigation is handled by the client.
Optimizing Performance
Optimizing performance in Next.js route files is crucial for delivering fast and seamless user experiences. This can be achieved by minimizing unnecessary code and leveraging Next.js built-in optimization features.
One key optimization technique is to use the `getStaticProps` method to pre-render pages at build time, reducing the need for server-side rendering and subsequent page loads. This is particularly effective for pages with static content.
By implementing these optimization strategies, developers can significantly improve the performance of their Next.js applications, resulting in faster page loads and a better overall user experience.
Caching
Caching is a crucial aspect of optimizing performance in your application. The App Router has four separate caches, including fetch request and route caches.
The route cache is the most likely to cause issues, especially if your application accepts login and renders different data based on the user.
You'll need to disable the route cache by using the dynamic export from the route handler if you encounter problems. After a mutation, invalidate the cache by calling revalidatePath or revalidateTag as appropriate.
Ensuring Static Rendering
Statically prerendering all routes can significantly speed up page loads and save compute time on the server.
All our routes are statically prerendered, which means they can be cached on the server, speeding up our page loads and saving compute time on the server.
However, due to next-intl APIs loading translations, our routes implicitly opt-in to dynamic rendering for each request.
The next-intl team plans to address this in future updates, but they’ve provided a temporary workaround.
To implement this workaround, we need to address the [locale] dynamic route param.
Next.js doesn’t know how to fill values in that route segment during building unless we tell via the generateStaticParams function.
Let’s add this function to our layout.
We need to call unstable_setRequestLocale in the layout and all pages.
This will ensure that we're getting static rendering again when we run npm run build.
With this in place, running npm run build should reveal that we’re getting static rendering again.
Route Configuration
Route configuration is a crucial aspect of Next.js. It allows you to define how your application will be structured and how users will navigate through it.
You can configure routes in Next.js by creating a pages directory and adding pages to it. Each page in the pages directory will be rendered as a separate route.
For example, if you have a pages directory with a file called index.tsx, it will be rendered as the root route of your application. Similarly, if you have a pages directory with a file called about.tsx, it will be rendered as the /about route.
You can also use the getStaticProps method to pre-render pages at build time. This can improve performance and SEO by allowing users to view static versions of your pages.
For instance, if you have a page that doesn't change often, you can use getStaticProps to pre-render it at build time, making it available for users to view without having to wait for the page to be rendered dynamically.
Sources
- https://redux.js.org/usage/nextjs
- https://phrase.com/blog/posts/next-js-app-router-localization-next-intl/
- https://nextjs.org/docs/app/building-your-application/routing/parallel-routes
- https://vercel.com/blog/common-mistakes-with-the-next-js-app-router-and-how-to-fix-them
- https://www.propelauth.com/post/cookies-in-next-js
Featured Images: pexels.com