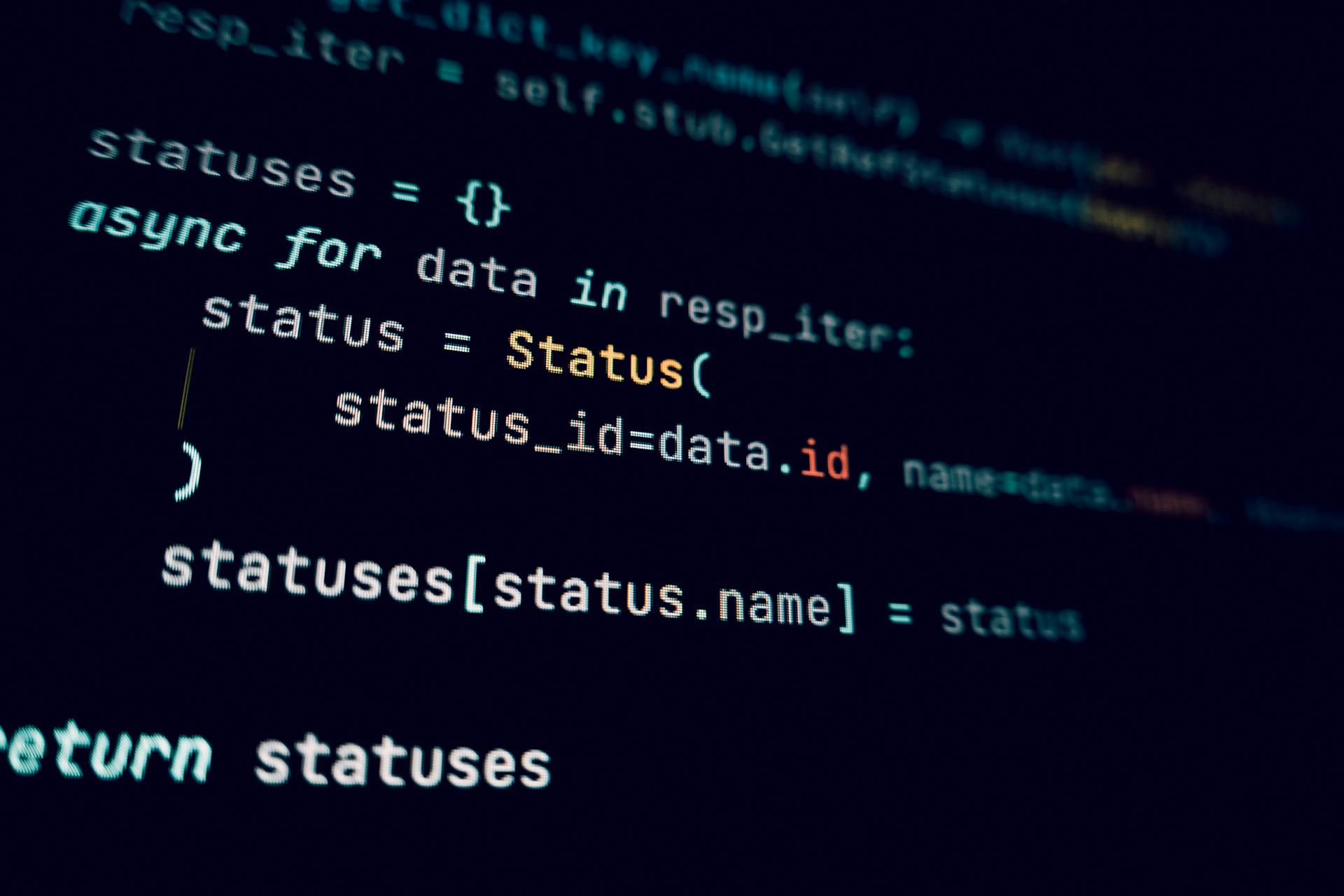
As a Python developer, you're likely no stranger to the Azure Identity Client Library, which provides a simple and efficient way to authenticate and authorize your applications with Azure Active Directory (AAD).
The Azure Identity Client Library for Python Developers is a must-have tool for any Python dev working with Azure services, allowing you to easily integrate authentication and authorization into your code.
With the library, you can use the `DefaultAzureCredential` class to automatically authenticate and authorize your applications, eliminating the need for manual configuration and reducing the risk of authentication errors.
This library is particularly useful for developers who want to build cloud-based applications that can securely access Azure resources without having to manage complex authentication flows.
Broaden your view: Azure Auth Json Website Azure Ad Authentication
Azure Client Library
The Azure Client Library is a crucial part of the Azure Identity library for Python, providing Microsoft Entra ID token authentication support across the Azure SDK.
You can use the Azure Identity library to construct Azure SDK clients that support Microsoft Entra token authentication, and it's compatible with various client and management libraries listed on the Azure SDK release page.
A unique perspective: Azure Sdk Python
The Azure Identity library offers a DefaultAzureCredential that attempts to authenticate using an opinionated, ordered sequence of mechanisms, making it easier to authenticate your app to Azure resources in different environments.
To use DefaultAzureCredential in a Python app, you'll need to add the azure-identity package to your application. This allows you to access Azure services using specialized client classes from the various Azure SDK client libraries.
Here's a list of some of the client libraries that support Microsoft Entra authentication:
- BlobServiceClient
- AadClient (with fix for multi-tenant auth using async)
Client Library Support
The Azure Client Library supports Microsoft Entra authentication, which means you can use it with various Azure services. You can find more information about using these libraries in their documentation, linked from the Azure SDK release page.
Client libraries listed on the Azure SDK release page that support Microsoft Entra authentication accept credentials from the Azure Identity library. These libraries include the ones that support token authentication.
Here are some examples of client libraries that support Microsoft Entra authentication:
- Fix multi-tenant auth using async AadClient (#21289)
This means you can use these libraries to authenticate with Azure services, and they will accept credentials from the Azure Identity library.
Async Credentials
Async Credentials provide a way to authenticate your Azure client library applications in an asynchronous manner. This is particularly useful when working with APIs that return data asynchronously.
To use Async Credentials, you'll need to install an async transport, such as aiohttp. You can find more information about this in the azure-core documentation.
Async Credentials should be closed when they're no longer needed, as this can help prevent resource leaks. Each Async Credential is an async context manager and defines an async close method.
Here's an example of how to use an Async Credential to authenticate an asynchronous SecretClient from azure-keyvault-secrets:
This example demonstrates authenticating the asynchronous SecretClient from azure-keyvault-secrets with an asynchronous credential.
Here are some key facts about Async Credentials:
- Async Credentials should be closed when they're no longer needed.
- Each Async Credential is an async context manager and defines an async close method.
- Async Credentials are used in conjunction with an async transport, such as aiohttp.
Authentication and Authorization
Authentication is a crucial aspect of working with the Azure Identity library, and DefaultAzureCredential is a great place to start. It's an opinionated, ordered sequence of mechanisms for authenticating to Microsoft Entra ID.
DefaultAzureCredential attempts to authenticate using the first credential, and if that fails, it moves on to the next one in the sequence. This means you can use different credentials in different environments without writing environment-specific code.
One of the key benefits of using DefaultAzureCredential is that it can retrieve environment settings and managed identity configurations to authenticate to Azure services automatically. This makes it a great choice for local development and deployment to Azure.
The Azure Identity library focuses on OAuth authentication with Microsoft Entra ID, and it offers various credential classes capable of acquiring a Microsoft Entra access token. Some of these credential classes include AzurePipelinesCredential, CertificateCredential, and ClientAssertionCredential.
Here are some examples of credential classes and their usage:
To use DefaultAzureCredential in a Python app, you'll need to add the azure-identity package to your application. This will allow you to create a DefaultAzureCredential object and use it with an Azure SDK client class.
Configuration and Setup
To use DefaultAzureCredential, you'll need to add the azure-identity package to your application. This will allow you to access Azure services using specialized client classes from the various Azure SDK client libraries.
You can then instantiate a DefaultAzureCredential object and use it with an Azure SDK client class, such as a BlobServiceClient object used to access Azure Blob Storage. This approach simplifies authentication while developing apps that deploy to Azure.
Here are the environment variables you'll need to configure for DefaultAzureCredential and EnvironmentCredential: Variable nameValueAZURE_CLIENT_IDID of a Microsoft Entra applicationAZURE_USERNAMEa username (usually an email address)AZURE_PASSWORDthat user's password
Local Development
Local development is a crucial part of the development process, and Azure provides several ways to simplify authentication during this phase.
You can use your own accounts to authenticate calls to Azure services, which is typical for developers debugging and executing code locally. The Azure Identity library supports authenticating through developer tools to simplify local development.
There are two main strategies for authenticating apps to Azure during local development. You can either create dedicated application service principal objects or authenticate the app to Azure by using the developer's credentials.
Creating dedicated application service principal objects involves setting up separate service principal objects for each developer and storing their identity as environment variables. This approach allows you to assign specific resource permissions to the service principal objects, but it requires creating separate objects for each developer.
Authenticating the app to Azure by using the developer's credentials involves signing in to Azure from the Azure CLI, Azure PowerShell, or Azure Developer CLI on the local workstation. The application can then access the developer's credentials from the credential store and use those credentials to access Azure resources.
DefaultAzureCredential is a class that simplifies authentication by combining credentials used in Azure hosting environments with credentials used in local development. This approach allows you to promote your app from local development to test environments to production without code changes.
DefaultAzureCredential uses an opinionated, ordered sequence of mechanisms for authenticating to Microsoft Entra ID. It attempts to authenticate using the first credential and then tries the next credential if the first one fails. This way, you can use different credentials in different environments without writing environment-specific code.
For more insights, see: Azure Clis
To use DefaultAzureCredential in a Python app, you need to add the azure-identity package to your application. You can configure DefaultAzureCredential with environment variables, such as AZURE_CLIENT_ID, AZURE_USERNAME, and AZURE_PASSWORD, to authenticate to Azure resources.
In a server environment, each app is assigned a unique application identity per environment where the app runs. In Azure, an application identity is represented by a service principal. You can use a managed identity service principal for apps hosted in Azure or an application service principal for apps hosted outside of Azure.
Here's a summary of the authentication methods for local development:
Dependency Changes
Dependency changes are a crucial aspect of configuration and setup.
We've made some important updates to our dependencies, starting with the adoption of msal_extensions 0.1.2.
This change brings a number of benefits, including improved security and performance.
We've also constrained the msal requirement to >=0.4.1, <1.0.0.
This ensures that our application is compatible with a wide range of versions of msal, while also preventing any potential issues that may arise from using outdated or deprecated versions.
File Hashes
File hashes are an essential part of verifying the integrity of a file.
You can find the hashes for the azure_identity package in the download section of the package's page.
The SHA256 hash for the azure_identity-1.19.0.tar.gz file is 500144dc18197d7019b81501165d4fa92225f03778f17d7ca8a2a180129a9c83.
The SHA256 hash for the azure_identity-1.19.0-py3-none-any.whl file is e3f6558c181692d7509f09de10cca527c7dce426776454fb97df512a46527e81.
Here are the hashes for both files:
Using Jupyter Notebooks
Using Jupyter Notebooks, you can easily install packages by prefixing the !pip install my_package statement with an exclamation mark. This is a game-changer for working with libraries like azure-identity.
To install the azure-identity library, simply use the command !pip install azure-identity in a Jupyter notebook cell. This will automatically install the library when the cell is executed.
You can install any package in a Jupyter notebook using the !pip install my_package statement, making it easy to get started with new libraries.
Applications and Users
Azure identity provides various credentials to authenticate applications and users.
For Azure-hosted applications, EnvironmentCredential authenticates a service principal or user via credential information specified in environment variables.
ManagedIdentityCredential authenticates the managed identity of an Azure resource.
WorkloadIdentityCredential supports Microsoft Entra Workload ID on Kubernetes.
When authenticating users, AuthorizationCodeCredential and DeviceCodeCredential are both used for interactive authentication.
AuthorizationCodeCredential authenticates a user with a previously obtained authorization code, while DeviceCodeCredential does the same on devices with limited UI.
Related reading: Azure Cosmos Db User Assigned Identity
Users
Users can authenticate with various credentials, including AuthorizationCodeCredential, which uses a previously obtained authorization code. This credential is used for OAuth2 authentication code.
DeviceCodeCredential is another option, which interactively authenticates a user on devices with limited UI, using device code authentication. InteractiveBrowserCredential also exists, but it's not supported in GitHub Codespaces and can be replaced with DeviceCodeCredential.
OnBehalfOfCredential propagates the delegated user identity and permissions through the request chain, using on-behalf-of authentication. UsernamePasswordCredential authenticates a user with a username and password, but it doesn't support multifactor authentication.
Here's a summary of user authentication credentials:
In addition to these credentials, users can also use DefaultAzureCredential, which simplifies authentication by combining credentials used in Azure hosting environments with credentials used in local development. This credential is especially useful when developing apps that deploy to Azure, as it can automatically detect and use the appropriate authentication method.
Additional reading: What Is Azure Used for
Principal
A service principal is a type of application in Microsoft Entra that can be used to authenticate and authorize access to resources. It's essentially an identity for an application, allowing it to interact with Azure services.
To authenticate a service principal, you can use various credential classes, including AzurePipelinesCredential, CertificateCredential, ClientAssertionCredential, and ClientSecretCredential. These credentials support different authentication methods, such as using a certificate, client assertion, or client secret.
Here's a breakdown of the credential classes:
A service principal can be authenticated using a certificate, which requires the following variables:
- AZURE_CLIENT_ID: ID of a Microsoft Entra application
- AZURE_TENANT_ID: ID of the application's Microsoft Entra tenant
- AZURE_CLIENT_CERTIFICATE_PATH: path to a PEM or PKCS12 certificate file including private key
- AZURE_CLIENT_CERTIFICATE_PASSWORD: password of the certificate file, if any
To authenticate a service principal with a secret, you'll need to provide the following variables:
- AZURE_CLIENT_ID: ID of a Microsoft Entra application
- AZURE_TENANT_ID: ID of the application's Microsoft Entra tenant
- AZURE_CLIENT_SECRET: one of the application's client secrets
Brokered
Brokered authentication is a convenient way to manage authentication handshakes and token maintenance for connected accounts. It's currently only supported by the Windows Web Account Manager (WAM).
To enable support for brokered authentication, you'll need to use the azure-identity-broker package. This package is specifically designed to work with WAM.
For more details on authenticating using WAM, check out the broker plugin documentation. It's a great resource for learning more about this process.
Using a brokered authentication approach can simplify the authentication process for your apps. Here's a brief overview of the process:
Continuous Access Evaluation
Continuous Access Evaluation is possible on a per-request basis as of version 1.14.0.
To enable this behavior, you need to set the enable_cae keyword argument to True in the credential's get_token method. This will allow you to access resources protected by Continuous Access Evaluation.
CAE isn't supported for developer and managed identity credentials, so be aware of that limitation.
Take a look at this: Python Access Azure Blob Storage
Token Management
Token Management is a crucial aspect of using the Azure Identity library in Python. Token caching is a feature that allows apps to cache tokens in memory (default) or on disk (opt-in), improving resilience and performance, and reducing the number of requests made to Microsoft Entra ID to obtain access tokens.
The Azure Identity library offers both in-memory and persistent disk caching, which can be opted-in for disk caching. This allows for more flexibility in managing tokens.
To manage tokens effectively, consider using the Azure Identity library's credential classes, such as DefaultAzureCredential or ChainedTokenCredential, which provide a simplified authentication experience and allow users to define custom authentication flows.
Define a Custom Flow with Chained Token Credential
If you need more flexibility in your authentication flow, you can use ChainedTokenCredential to create a custom chain of credentials.
ChainedTokenCredential allows you to combine multiple credential instances to define a customized chain of credentials. This can be especially useful if you have different authentication requirements for different environments.
To use ChainedTokenCredential, you can create an instance of the class and add multiple credential instances to it. This will allow you to define a custom authentication flow that considers multiple credentials.
Here's a simple example of how you can use ChainedTokenCredential:
- You can return a callable that provides a bearer token using get_bearer_token_provider.
- This can be used to write code like the following example:
```python
get_bearer_token_provider = ChainedTokenCredential(
DefaultAzureCredential(),
ClientSecretCredential(
"your_tenant_id",
"your_client_id",
"your_client_secret"
)
)
```
Note that ChainedTokenCredential is not the default authentication mechanism, and DefaultAzureCredential is generally the quickest way to authenticate apps for Azure. However, ChainedTokenCredential offers more flexibility and customization options.
By using ChainedTokenCredential, you can define a custom authentication flow that meets your specific requirements. This can be especially useful if you have complex authentication requirements or need to support multiple authentication mechanisms.
Here's a table summarizing the key differences between DefaultAzureCredential and ChainedTokenCredential:
Overall, ChainedTokenCredential offers more flexibility and customization options than DefaultAzureCredential. However, DefaultAzureCredential is generally the quickest way to authenticate apps for Azure.
Token Caching
Token caching is a feature provided by the Azure Identity library that allows apps to cache tokens in memory (default) or on disk (opt-in). This caching reduces the number of requests made to Microsoft Entra ID to obtain access tokens.
The Azure Identity library offers both in-memory and persistent disk caching. This means you can choose the caching method that best suits your app's needs.
Token caching improves resilience and performance by reducing the load on Microsoft Entra ID. This is especially important for apps that need to handle a large volume of requests.
Here are the benefits of token caching:
- Caches tokens in memory (default) or on disk (opt-in)
- Improves resilience and performance
- Reduces the number of requests made to Microsoft Entra ID to obtain access tokens
For more information on token caching, see the token caching documentation.
Sources
- https://pypi.org/project/azure-identity/
- https://learn.microsoft.com/en-us/python/api/overview/azure/identity-readme
- https://learn.microsoft.com/en-us/python/api/azure-identity/azure.identity
- https://learn.microsoft.com/en-us/azure/developer/python/sdk/authentication/overview
- https://blog.finxter.com/how-to-install-azure-identity-in-python/
Featured Images: pexels.com