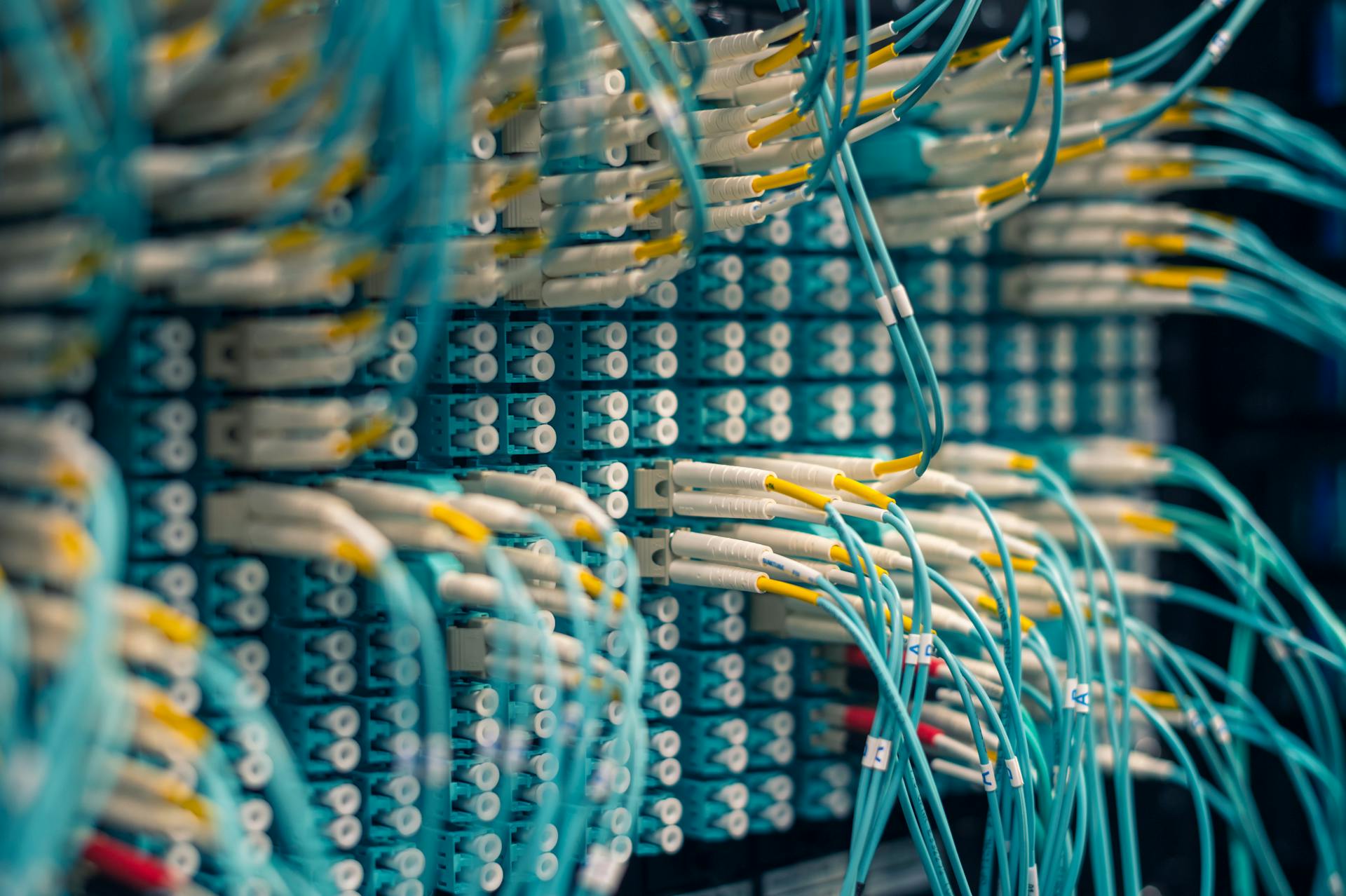
Setting up a Python website hosting environment requires a solid foundation in web development and server configuration. This guide will walk you through the process of setting up a Python website hosting environment using popular frameworks such as Flask and Django.
To get started, you'll need to choose a web hosting service that supports Python. Some popular options include AWS Elastic Beanstalk, Heroku, and DigitalOcean. These services provide pre-configured environments for Python development, making it easier to deploy and manage your website.
When selecting a web hosting service, consider factors such as scalability, security, and cost. For example, AWS Elastic Beanstalk offers a free tier for small projects, while Heroku provides a generous free plan for hobby projects. DigitalOcean, on the other hand, offers a range of pricing plans to suit different project needs.
Project Setup
To set up your Python web application, you'll need to create a project folder and give it a descriptive name, such as hello-app. Inside this folder, you'll need three files: main.py, requirements.txt, and app.yaml.
You'll start with main.py, which contains your Python code wrapped in a minimal implementation of the Flask web framework, using fewer than ten lines of code.
Here are the three files you'll need for your project:
- main.py: Your Python code wrapped in a minimal implementation of the Flask web framework.
- requirements.txt: Lists all the dependencies your code needs to work properly.
- app.yaml: Helps Google App Engine decide which settings to use on its server.
You can download the complete source code for your project by clicking the link provided, and then test your code locally before deploying it to Google App Engine.
Deploying Your Application
Deploying your application is a crucial step in making it accessible to a broad range of users. You'll need to refactor your code into a web application and deploy it to the Internet.
To make your application accessible, you'll need to run it on a back-end server, where the program processes incoming requests and responds through a shared protocol that's understood by all browsers. This is where Python web frameworks like Flask come in, automatically taking care of the details.
You'll need to set up a deployment process, both in the cloud and locally, to bring your app online. This involves completing the necessary setup in the cloud, selecting a region to host your application, and confirming the deployment.
Here are the required applications for installing additional packages/libraries:
- You will need Secure Shell (SSH) access to the server to install custom Python modules.
- You can install Python applications on your cPanel when you use the Apache web server via the “Setup Python App” function.
- You can also get PIP, a Python package manager that comes standard with Python versions 3.4 and higher.
To deploy your application, you'll need to use a command-line interface (CLI) like gcloud to fetch your authentication credentials and project ID information. You'll then need to select a region to host your application and confirm the deployment.
Once your deployment is complete, you'll be able to access your live web app by navigating to the mentioned URL in your browser or using the suggested command gcloud app browse.
Security and Performance
The http.server module is not recommended for production due to its severe security and performance limitations.
The official documentation warns that it only implements basic security checks, making it vulnerable to information leakage and other unexpected side effects.
On a Unix-like operating system, you can simulate this issue by creating a directory structure with symbolic links to sensitive files.
Creating a symbolic link to a secret file with a password and another to the entire private folder can demonstrate this vulnerability.
To start an HTTP server in the public folder, use the -d parameter to sandbox the files.
Because the public folder contains symbolic links, the server will follow them, allowing you to view linked secret files.
The http.server module is limited by its poor speed and scalability, making it too slow for large-scale or high-traffic websites.
It lacks advanced features like SSL support and user session management, which are expected from a full-fledged web framework.
Hosting and Infrastructure
To host a Python website, you'll need to ensure your hosting provider meets certain basic requirements. These include supporting the Python version your application was created with, offering a web server like Apache or Nginx, and allowing you to install necessary Python packages and libraries.
A good hosting service should also provide support for databases like MySQL, MongoDB, and PostgreSQL, as well as give you access to the file system to upload and manage your Python code and other files.
Here are the key requirements to consider when choosing a hosting provider:
- Supported Python version
- Web server (e.g. Apache, Nginx)
- Ability to install Python packages and libraries
- Database support (e.g. MySQL, MongoDB, PostgreSQL)
- File system access
Kamatera, for example, meets these requirements with its cutting-edge hardware, global network reach, and elastic infrastructure that scales to accommodate your needs.
Data Centers Globally
Having a global presence is crucial for hosting, and Kamatera has 21 data centers across four continents.
Kamatera's global network reach allows for low-latency access to your server, regardless of your users' geographical locations.
This means you can expect consistent performance for geographically distributed teams.
Kamatera's infrastructure is strategically located to minimize lag and ensure seamless communication with your users worldwide.
With data centers in so many locations, Kamatera is well-equipped to handle the needs of users from all over the globe.
Hosting Service Requirements
To host your Python application, you'll need to consider several key requirements.
First and foremost, your hosting provider should support the Python version your application was created with. This is crucial, as some hosting services only support specific versions of Python.
To ensure your Python application runs smoothly, you'll also need a web server that works with Python programs. The most popular web servers for Python applications are Apache and Nginx.
You should also be able to install any necessary Python packages and libraries through your hosting service. Some providers may have restrictions on the types of packages that can be installed, so it's essential to verify their support.
In addition to these requirements, your hosting provider should offer support for databases, such as MySQL, MongoDB, and PostgreSQL.
Your hosting provider should also allow you to alter the server settings to suit your application's requirements. This includes installing necessary dependencies, adjusting server settings, and setting up environment variables.
To summarize, here are the key hosting service requirements for your Python application:
- Supported Python version
- Web server (e.g. Apache, Nginx)
- Ability to install Python packages and libraries
- Support for databases
- Server configuration flexibility
- File access
Linux Hosting Compatible Applications
Python is a flexible language that can be used for various purposes and applications, including web development, data processing, machine learning, and more.
Some popular Python applications that can run on Linux Shared Hosting and Linux VPS Hosting include Django, Flask, Pyramid, Bottle, Pandas, NumPy, SciPy, Matplotlib, Seaborn, Scikit-learn, Keras, TensorFlow, Pygame, PyOpenGL, PyQt, and wxPython.
To run Python applications on Linux Shared Hosting, you'll need to determine whether Python is pre-installed on the server. If it's not, you can install it using the terminal access provided by the hosting provider.
Secure Shell (SSH) access is required to install custom Python modules on the server. This allows you to securely connect to your server and perform tasks using the Linux command line interface.
You can also install Python applications on your cPanel using the "Setup Python App" function when you use the Apache web server. This function allows you to test the functionality of the application.
Here are some of the supported applications with Python:
- Web development: Django, Flask, Pyramid, Bottle
- Data processing and visualization: Pandas, NumPy, SciPy, Matplotlib, Seaborn
- Machine learning and artificial intelligence: Scikit-learn, Keras, TensorFlow
- Automation and scripting: web scraping, system administration
- Scientific computing: numerical simulations, modeling
- Game development: Pygame, PyOpenGL
- Desktop applications: PyQt, wxPython
Scripting and Execution
Python website hosting allows you to deploy your Python code online, making it accessible to users through a web browser.
You can convert a script into a web application by saving it as a Python script, testing it, and then integrating it into your Flask app. To do this, you need to consider two main points: execution and user input.
Execution is handled by adding the code to a function and assigning a route to it using the @app.route decorator. However, you'll encounter a problem when running the code in your development server.
To collect user input, you'll need to learn how to escape it, which is not necessary when using templates in larger web applications.
You can share your temperature conversion web app with others and allow them to convert Celsius temperatures to Fahrenheit temperatures.
Here are some supported applications with Python:
- Web development using frameworks like Flask, Django, and Pyramid
- Data processing and visualization using libraries like Pandas, NumPy, and Matplotlib
- Machine learning and artificial intelligence using libraries like Scikit-learn, Keras, and TensorFlow
- Automation and scripting using libraries like Pygame and PyOpenGL
- Scientific computing using libraries like SciPy and SymPy
- Desktop applications using frameworks like PyQt and wxPython
To install custom Python modules, you'll need Secure Shell (SSH) access to the server, which allows you to securely connect to your server and perform tasks using the Linux command line interface.
You can also install Python applications on your cPanel using the "Setup Python App" function, or use pip, the Python package manager, to install any required Python packages or libraries for your application.
Hosting and Configuration
To host a Python website, you need to ensure that your hosting service meets the basic requirements, which include a supported Python version, a compatible web server, and access to Python packages and libraries.
The most popular web servers for Python applications are Apache and Nginx, and you should verify that your hosting provider supports your preferred web server. Additionally, you should be able to install any necessary Python packages and libraries through your hosting service.
Here are the basic requirements for hosting a Python website:
- Supported Python version
- Compatible web server (e.g. Apache, Nginx)
- Access to Python packages and libraries
- Support for databases (e.g. MySQL, MongoDB, PostgreSQL)
- Server configuration options
- File access to upload and manage Python code and files
By meeting these conditions, you can ensure that your Python website will function properly on the hosting service.
Google App Engine Setup
To set up a project on Google App Engine, start by creating a project folder and naming it something descriptive, like "hello-app". This folder will contain three essential files: main.py, requirements.txt, and app.yaml.
These files are the bare minimum required for Google App Engine to deploy your project. main.py contains your Python code wrapped in a minimal Flask web framework, while requirements.txt lists the dependencies your code needs to work properly.
For this tutorial, the main.py file contains just nine lines of code, making it a great example of minimal setup. You can download the complete source code and follow along.
Requirements.txt is where you specify the dependencies your project needs. Since Flask is the only dependency for this project, that's all you need to specify in this file.
app.yaml helps Google App Engine set up the right server environment for your code. This file requires only one line, which defines the Python runtime as Python 3.8.
To deploy your project, you'll need to sign in to the Google Cloud Platform and navigate to the dashboard view. From there, select the downward-facing arrow button to view a list of your projects.
If you haven't created any projects yet, the list will be empty. Click the NEW PROJECT button to create a new project and give it a name, like "hello-app". The project ID will be displayed below the project name input field.
You can see your project ID, which consists of the name you entered and a number that Google App Engine adds. Make sure to copy your project ID, as you'll need it later for deploying.
Here's a quick rundown of the steps to create a new project on Google App Engine:
- Sign in to the Google Cloud Platform and navigate to the dashboard view.
- Select the downward-facing arrow button to view a list of your projects.
- Click the NEW PROJECT button to create a new project.
- Give your project a name and copy your project ID.
- Wait for the project to be set up on Google App Engine's side.
Once your project is set up, you'll be redirected to the Google App Engine dashboard view of your new project. From there, you can proceed with deploying your app to the Internet.
Create Self-Signed Certificate
To create a self-signed certificate, you'll need to use OpenSSL, a command-line tool for generating and managing certificates.
The OpenSSL command for generating a self-signed certificate is `openssl req -x509 -newkey rsa:2048 -nodes -keyout key.pem -out cert.pem -days 365`.
This command creates a private key and certificate in separate files, key.pem and cert.pem, respectively.
The -x509 option tells OpenSSL to generate a self-signed certificate, while -newkey rsa:2048 generates a new RSA private key with a key size of 2048 bits.
The -nodes option prevents OpenSSL from encrypting the private key with a pass phrase.
CloudLinux Configuration
CloudLinux provides an easy-to-use interface for managing the hosting environment and deploying Python applications when used with cPanel.
You can run Python applications well on CloudLinux, which is designed for web hosting companies.
For hosting Python apps, use CloudLinux and cPanel together to get the best results.
CloudLinux is a popular operating system designed specifically for web hosting companies.
Frequently Asked Questions
Which hosting is best for Python?
For Python web development, A2 Hosting is a top choice due to its fast dedicated servers that boost loading speed, making it ideal for high-performance applications. Its Python web hosting solution is specifically designed to optimize speed and efficiency.
Where can I host a Python website for free?
Host your Python website for free on Anvil, a platform that lets you build and deploy web apps without any costs. With Anvil, you can focus on coding, not infrastructure
Is Python good for making websites?
Yes, Python is a great choice for making dynamic websites, and you don't need to be a pro to get started. Learn how to use Python for web development with our step-by-step guide.
Featured Images: pexels.com