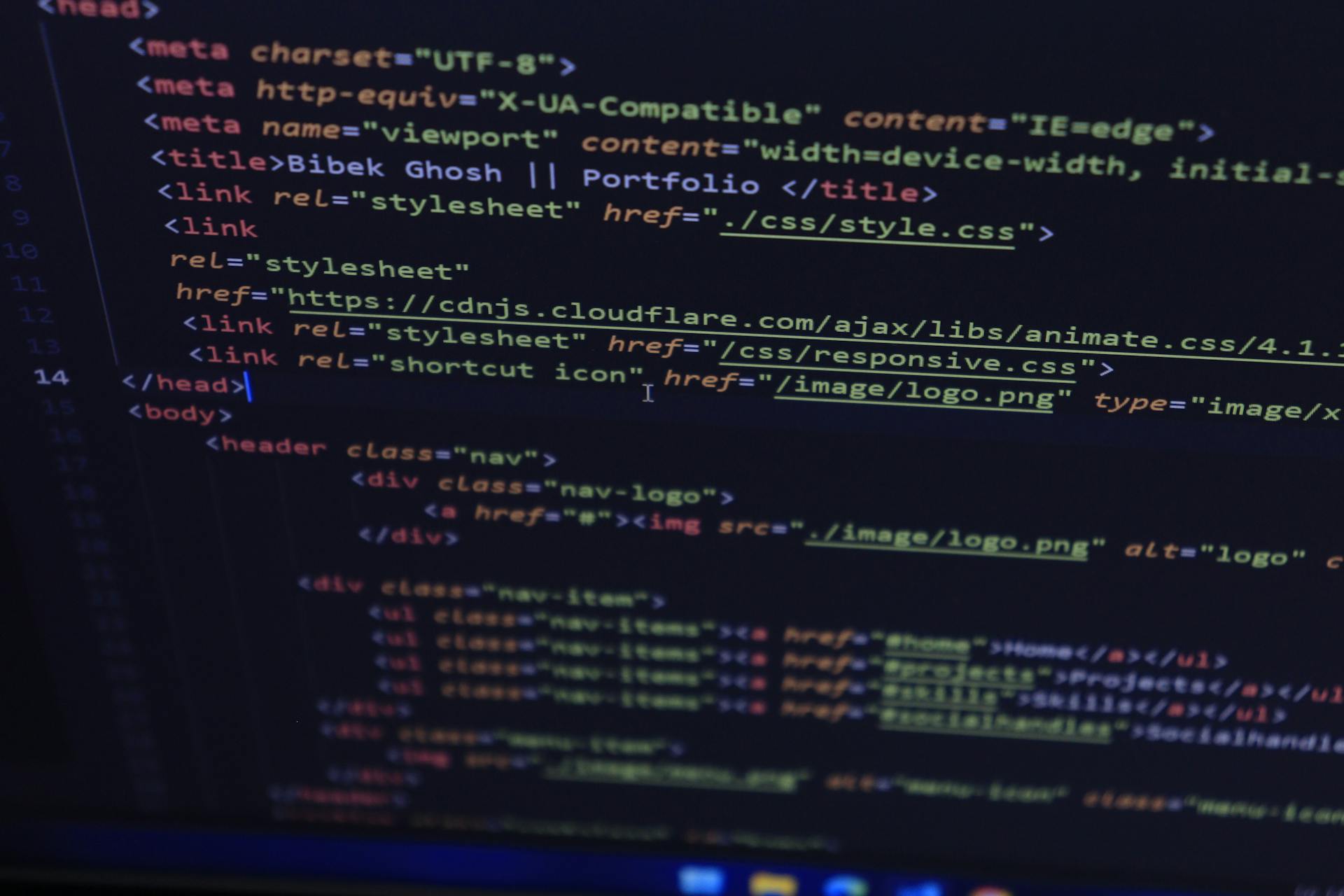
Query Selector CSS is a powerful tool for selecting elements on a webpage. It allows you to target elements based on their attributes, classes, and IDs.
With Query Selector CSS, you can select elements using various methods, including attribute selectors, class selectors, and ID selectors. These selectors are essential for creating dynamic and interactive web pages.
Attribute selectors, for example, enable you to target elements based on their attributes, such as the href attribute for links or the src attribute for images. This is particularly useful for selecting elements that have specific attributes or values.
Class selectors, on the other hand, allow you to target elements that have a particular class applied to them. This is useful for styling elements that share a common design or behavior.
Intriguing read: Tailwind Css Media Query
Basic
Query selector CSS is a powerful tool that allows you to select elements on a webpage using a simple syntax.
You can find all h2 elements using the basic selector, as shown in an example: it simply finds all h2 elements.
The basic selector is straightforward and easy to use, making it a great starting point for beginners.
For instance, finding all h2 elements is as simple as typing "h2" in your query selector.
Expand your knowledge: Gsheets Query
Selectors
Selectors are a crucial part of CSS, allowing you to target specific elements on a web page. CSS selectors pierce open shadow DOM, giving you more flexibility in your queries.
You can use a comma-separated list of CSS selectors to match all elements that can be selected by one of the selectors in that list. This is done using the :is() pseudo-class, which is an experimental CSS pseudo-class.
Descendant selectors are also available, selecting all elements that are descendants of a given ancestor. This is done using the "ancestor descendant" syntax.
Here are some examples of attribute selectors:
- Attribute Contains Word Selector [name~="value"]
- Attribute Starts With Selector [name^="value"]
- Attribute Ends With Selector [name$="value"]
- Attribute Equals Selector [name="value"]
- Attribute Contains Prefix Selector [name|="value"]
- Attribute Contains Suffix Selector [name*="value"]
Note that attribute selectors can also be used to match property values in React locators, which are experimental and prefixed with _.
Universal
The universal selector is a powerful tool in the world of CSS selectors. It's denoted by an asterisk (*) and matches all elements of any type.
This single character can be used to select all elements in a document, making it a great choice for tasks that require global changes. The universal selector is denoted by * that matches all elements of any type.
See what others are reading: Css Selector First of Type
Class
Class selectors are a powerful tool in CSS, allowing you to target elements based on the classes they have.
To find the element with a given CSS class, you use the class selector syntax. This syntax is written with a dot (.) followed by the class name.
The class selector can be used to find a single element, like the first element with the menu-item class.
You can also use the class selector to find all elements with a given class, such as all elements with the menu class.
For another approach, see: How to Find Css Selector of an Element
Data-Test-Id
Data-Test-Id is a powerful way to locate elements on a page. It's recommended over other methods because it's more specific and can help avoid issues with duplicate selectors.
You can use data-test-id with the querySelectorAll() method, but only if you're targeting a unique element, since it's not relevant for non-unique elements.
Playwright supports shorthand for selecting elements using data-test-id. You can use the following attributes to select elements: id, data-testid, data-test-id, and data-test.
Here's a list of supported attributes:
- id
- data-testid
- data-test-id
- data-test
For example, css=[data-test="login"]:enabled is equivalent to a proper CSS selector, but it's not supported in attribute selectors.
General Sibling Combinator
The General Sibling Combinator is a powerful tool in the world of selectors. It's used to select siblings that share the same parent.
The ~ combinator is the key to unlocking this selector's potential. It selects siblings that follow the specified element, immediately or not.
For example, if you have a paragraph of text and you want to select all the links that follow a specific heading, you can use the ~ combinator to achieve this.
This selector is particularly useful when you need to target elements that are nested deeply within a document. By using the ~ combinator, you can avoid having to write complex selectors that target specific elements by their position.
As you can see, the General Sibling Combinator is a simple yet effective way to select elements that share the same parent.
Explore further: Css Selector Select Child of Parent
Locator
Locators are a powerful tool in selecting elements on a page. They allow you to pinpoint specific elements with precision.
If this caught your attention, see: Css Selector the Last 2 Child Elements
Playwright can locate an element by CSS selector, which pierces open shadow DOM. This means you can access elements that are nested within a shadow DOM.
Playwright also adds custom pseudo-classes like :visible, :has-text(), :has(), :is(), :nth-match() and more. These pseudo-classes give you even more flexibility in selecting elements.
You can narrow down a query to the n-th match using the nth= locator, passing a zero-based index.
React and Vue locators are experimental and prefixed with _. They allow finding elements by their component name and property values, similar to CSS attribute selectors.
In React locator, component names are transcribed with CamelCase, while in Vue locator, they are transcribed with kebab-case.
Legacy text locator matches elements that contain passed text, with a few variations. You can use text=, text=", or a JavaScript-like regex wrapped in / symbols to match text.
Here are some examples of legacy text locators:
- text=Log in - default matching is case-insensitive, trims whitespace and searches for a substring.
- text="Log in" - text body can be escaped with single or double quotes to search for a text node with exact content after trimming whitespace.
- /Log\s*in/i - body can be a JavaScript-like regex wrapped in / symbols.
String selectors starting and ending with a quote (either " or ') are assumed to be a legacy text locators.
Matching Visible Elements
You can use the :visible pseudo class in CSS selectors to match only visible elements. This is especially useful when working with similar elements that differ in visibility.
For example, if you have a page with two buttons, one invisible and one visible, you can use `css=button:visible` to find only the visible button.
Using `css=button` would throw a strictness violation error, as it would try to find both buttons. To avoid this, use the `:visible` pseudo class to specify that you only want to find visible elements.
Here are some examples:
- `css=button` will find both buttons and throw a strictness violation error.
- `css=button:visible` will only find the visible button.
If you're working with multiple elements that have varying visibility, using the `:visible` pseudo class can help you avoid errors and ensure that your code only targets the elements you intend to.
Contains Word [Name="CSS"]
Contains Word [Name="CSS"] is a powerful selector that allows you to target elements based on the presence of a specific word in their attribute values.
According to the article, the Attribute Contains Word Selector [name~="value"] selects elements that have the specified attribute with a value containing a given word, delimited by spaces. For example, if you have an element with the attribute name="hello world" and you want to target it using Contains Word [Name="CSS"], it will match because "world" is a word contained in the attribute value.
Consider reading: Css Name Selector
This selector is useful when you need to target elements that have a specific word in their attribute values, but you don't know the exact attribute name or value. For instance, if you have multiple elements with different attribute names and values, but they all contain the word "css", you can use Contains Word [Name="CSS"] to target all of them.
In the article, it's mentioned that the Attribute Contains Word Selector [name~="value"] is similar to the Attribute Contains Prefix Selector [name|="value"], but the main difference is that it looks for words instead of prefixes.
Broaden your view: Css Attribute Selector
Header
Headers in CSS selectors are a breeze to work with. You can select all elements that are headers, like h1, h2, h3 and so on, using the :header Selector.
The :header Selector is a special type of selector that matches all header elements, including h1, h2, h3, and more.
You can also select all elements with the given tag name, such as h1, using the Element Selector (“element”) method. This is similar to the Type selector, which also selects elements by node name.
To select the first h1 element in the document, you would use the Element Selector (“element”) method, as shown in the example.
Suggestion: Css Type Selector
Empty
The empty selector is a useful tool for selecting elements that have no children, including text nodes.
This means that if an element has no child elements, no text content, and no other attributes, the empty selector will select it.
For example, if you have an element with no children, the empty selector will select it, as seen in the example: ":empty Selector / Select all elements that have no children (including text nodes)".
For your interest: Css No Class
Eq()
The ":eq() Selector" is a powerful tool for selecting specific elements within a matched set. It allows you to target the element at a specific index.
You can use the ":eq()" selector to select the first element in a matched set with ":eq(0)".
This selector is useful for targeting specific elements within a list or table, where the order of the elements matters.
Worth a look: Css Selector That Targets a Specific Style Declaration
File
Let's talk about selecting specific types of elements on a webpage using CSS selectors.
The :file selector is a powerful tool that selects all elements of type file.
This selector can be super helpful when you need to style or target file input fields on a webpage.
For example, you can use the :file selector to change the background color or border of file input fields to make them stand out.
The :file selector is a simple yet effective way to target file input fields and apply custom styles to them.
You might enjoy: Css in Html File
Image
The "image" selector is a powerful tool. It selects all elements of type image.
If you're working on a project with a lot of images, this selector can be a lifesaver.
Intriguing read: Css Image in Text
Multiple
Using multiple selectors is a powerful way to refine your search. You can specify multiple selectors separated by commas, like this: "selector1, selector2, selectorN". This combination of selectors selects the combined results of all the specified selectors.
For example, if you're looking for a product with a specific color and size, you can use multiple selectors to find the exact match. Just type in the different criteria, separated by commas.
The more specific you are with your selectors, the more accurate the results will be.
If this caught your attention, see: Css Selector Multiple Attributes
Nth-Last-Of-Type()
The :nth-last-of-type() selector is a powerful tool for selecting elements based on their position among siblings with the same name.
It counts from the last element to the first, making it a bit more complex than the :last-of-type selector.
To use :nth-last-of-type(), you need to specify the position of the element you want to select, for example, :nth-last-of-type(3) would select the third element from the last among its siblings with the same name.
This selector is useful when you need to target a specific element in a list or a grid, and you know its position from the end.
Additional reading: Css Last of Class
Password
The password selector is a specific type of selector that's used to target elements of type password. This selector is useful for accessing and styling password input fields.
You can use the ":password" selector to select all elements of type password, which is exactly what it does.
In CSS, selectors like ":password" help developers target specific elements and apply styles or behaviors to them.
Frequently Asked Questions
What is query selector in CSS?
Query selector is a JavaScript method that selects the first element matching a CSS selector, returning the element if found or null otherwise
What is querySelectorAll()?
querySelectorAll() is a JavaScript method that selects multiple elements in a webpage based on a CSS selector. It returns a list of matching elements, making it a powerful tool for web development.
When to use querySelector?
Use querySelector when you need to select a single specific element from the DOM tree, such as retrieving a unique form field or button
Featured Images: pexels.com