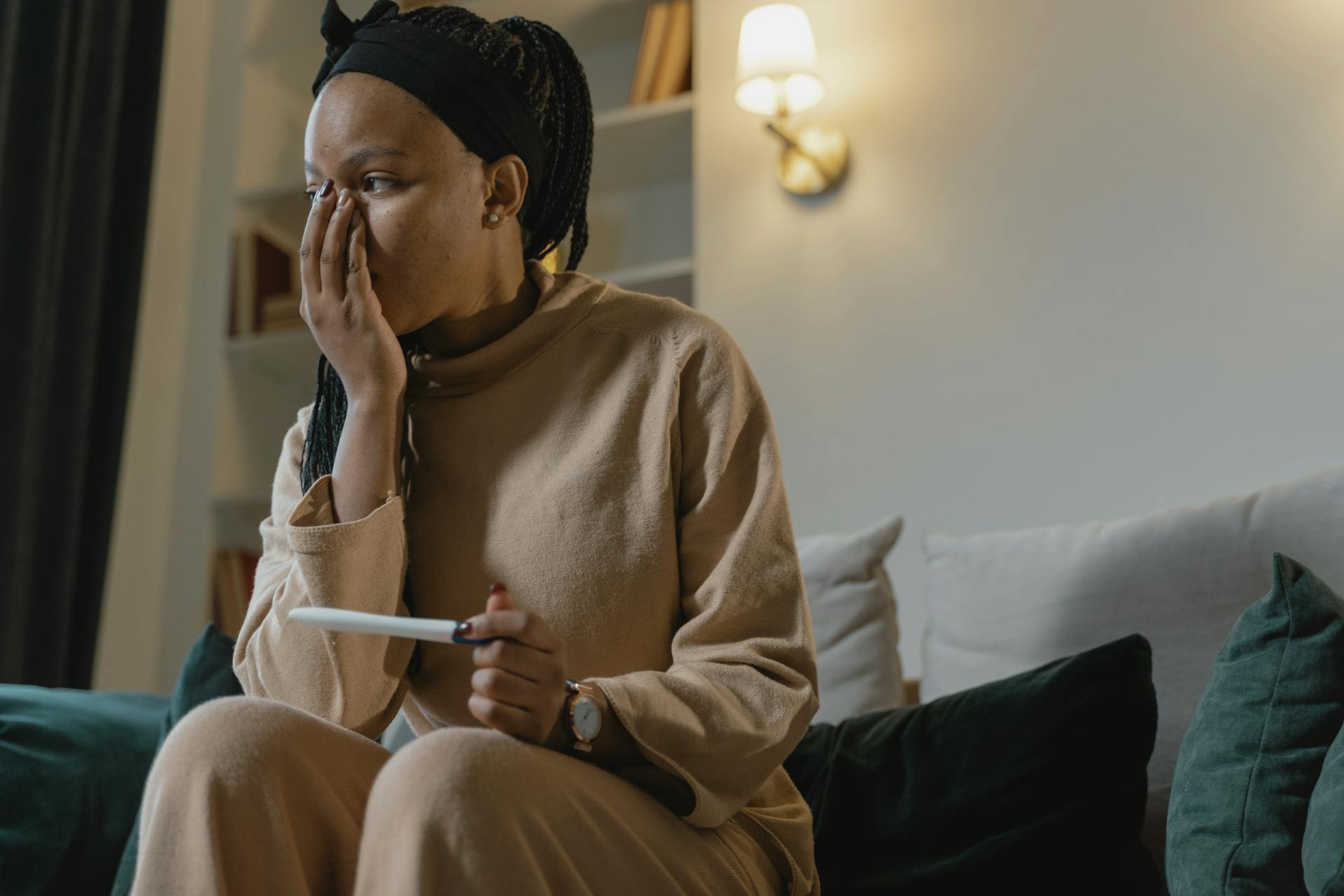
React Convert Text to HTML for Dynamic Content is a powerful feature that allows you to render text as HTML, making it perfect for dynamic content.
You can use the `dangerouslySetInnerHTML` attribute to render text as HTML, but be cautious of security risks.
This attribute allows you to set the `innerHTML` property of an element, but it can be vulnerable to cross-site scripting (XSS) attacks if not used properly.
To safely use `dangerouslySetInnerHTML`, you can use a library like DOMPurify to sanitize the input text and prevent XSS attacks.
By using `dangerouslySetInnerHTML` or a library like DOMPurify, you can create dynamic content that is both secure and visually appealing.
For another approach, see: Text Content Does Not Match Server-rendered Html
String Manipulation
String Manipulation is a crucial aspect of working with React, allowing us to update HTML content based on user input or dynamic data sources.
React's state system makes it easy to update HTML content, as seen in the example where a button updates the state, which in turn updates the HTML content being rendered.
You can use JavaScript to manipulate HTML strings, making it possible to dynamically update the HTML content being rendered by React.
This can be achieved by updating the state in response to user input or other dynamic data sources, as demonstrated in the example code.
Broaden your view: Content Type Text Html
Manipulating Strings with JavaScript
React's state system allows us to update the HTML content based on user input or other dynamic data sources.
Updating state in React is a powerful way to make your application interactive and dynamic. This can be achieved by using a button to update the state, which in turn updates the HTML content being rendered.
In React, you can use dangerouslySetInnerHTML to insert HTML directly, but be aware that this can open up risks of XSS.
To safely render HTML strings in React, consider sanitizing the HTML using a library like DOMPurify. This will help prevent malicious code from being executed.
A fresh viewpoint: Html Meta Http-equiv Content-type Content Text Html Charset Utf-8
Converting to Static
Converting React components into static HTML is useful for server-side rendering or generating HTML files for static sites. This can be a game-changer for websites that don't require a lot of dynamic content.
There are scenarios where you might want to convert your react components into static HTML. This can be useful for server-side rendering or generating HTML files for static sites.
For more insights, see: Image and Text Side by Side Html Css
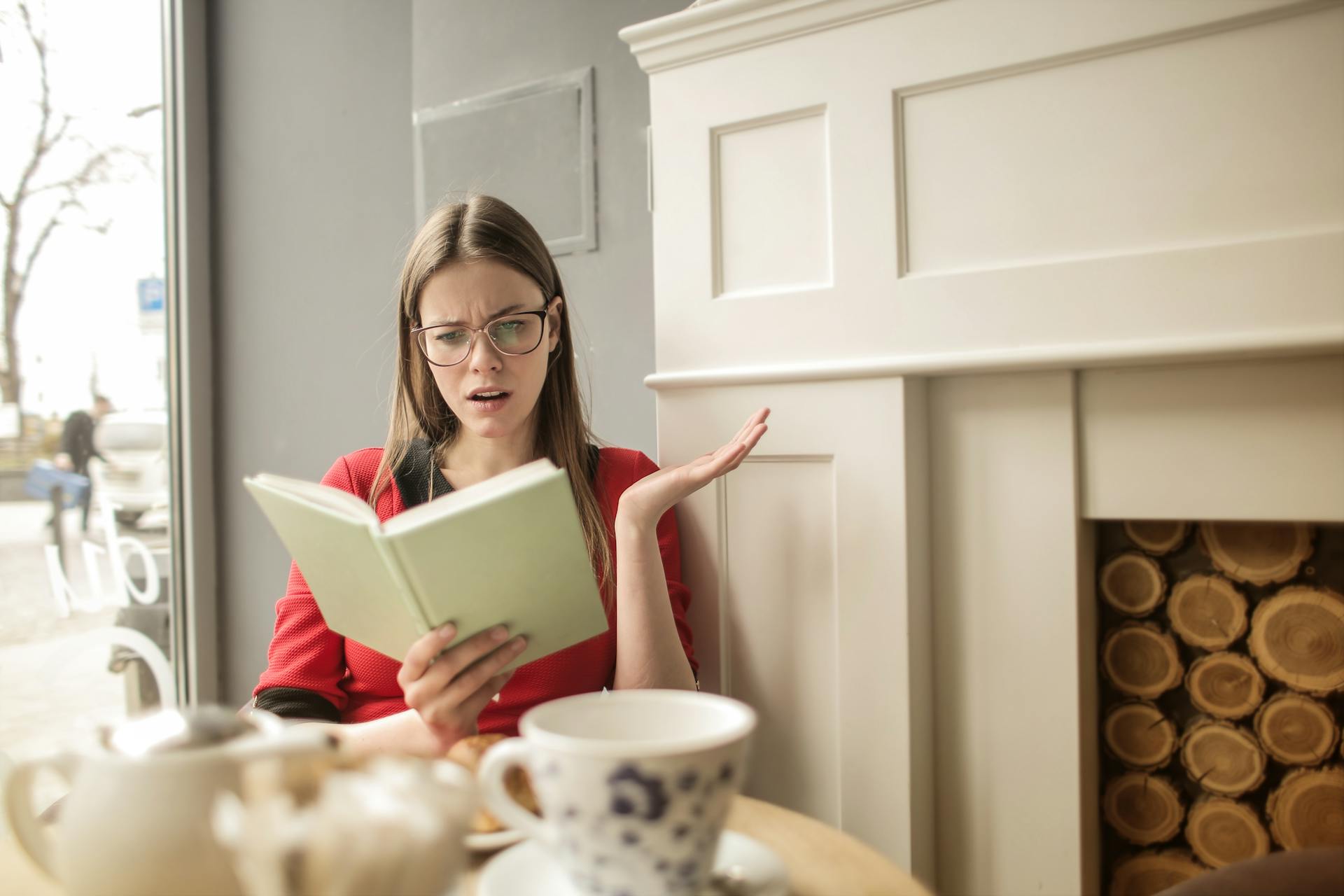
Server-side rendering can improve the performance of your website by reducing the amount of data that needs to be loaded on the client-side. This can be especially beneficial for websites with complex layouts or large datasets.
Generating HTML files for static sites can also be done using React components. This can be useful for creating websites that don't require a lot of dynamic content, such as blogs or portfolios.
Component Integration
Component integration is key to converting text to HTML in React. You can inject HTML into React components securely using the dangerouslySetInnerHTML property.
To do this, create a function that returns an object with a property __html containing the HTML string. This allows you to render the HTML content.
For more complex scenarios, you might need to use advanced techniques like JSX code, template literals, or JavaScript to manipulate HTML strings. This can be a bit more involved, but it's a powerful tool in your React toolkit.
On a similar theme: React Js Html Editor
Component Fundamentals
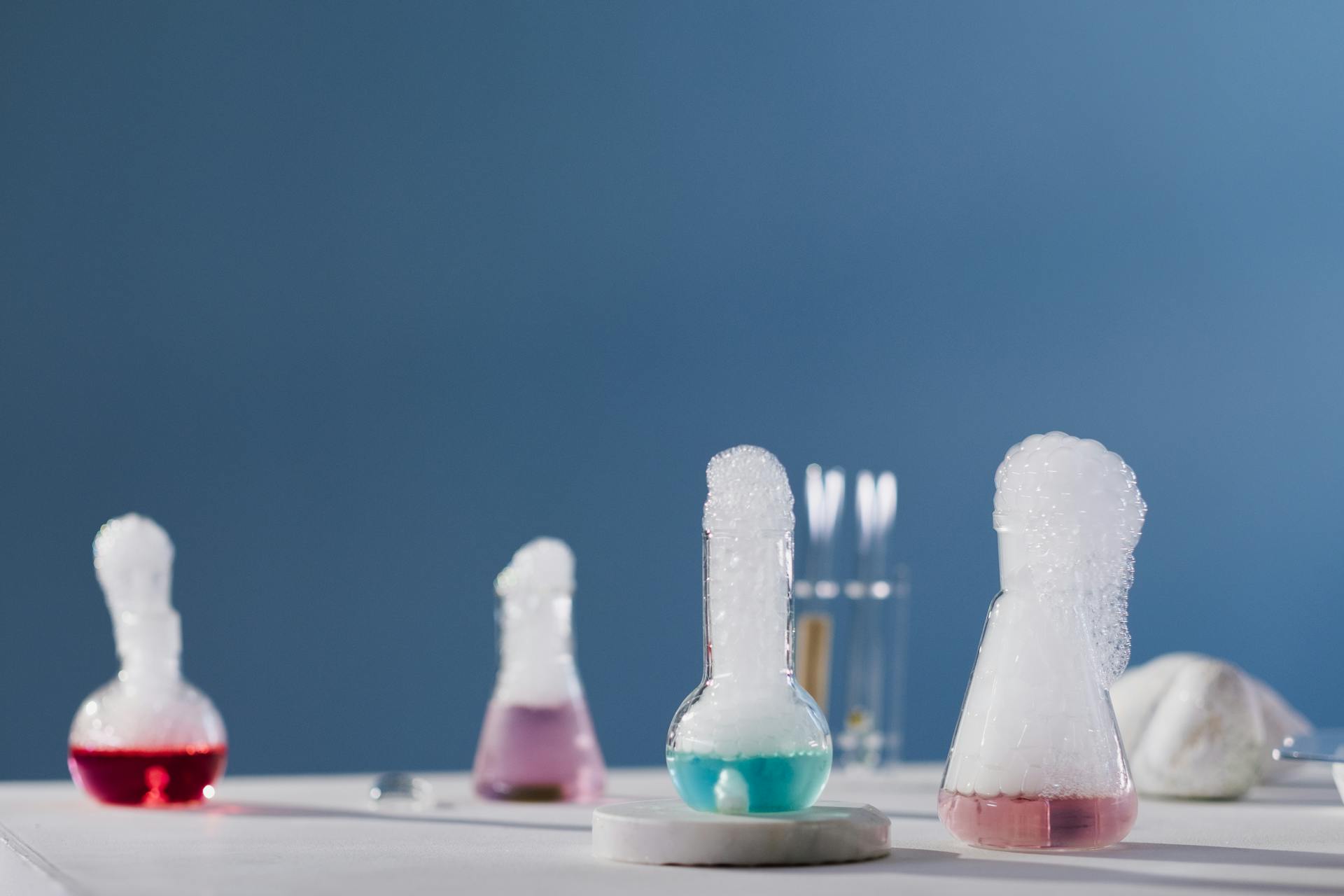
React components can render dynamic HTML content that comes from an external source.
To do this, you can use the dangerouslySetInnerHTML property, which sets raw HTML directly from a string. This property is a necessary evil in React development, but it must be used securely to prevent cross-site scripting attacks.
By using this property, you can render HTML content that's been fetched from an external source, like a database or an API.
However, using contentEditable in React apps can be tricky, especially when you need to capture input before the blur event. This can cause cursor jumping and other undesirable behaviors.
The react-contenteditable package solves most of these common issues, making it a great addition to your React app.
Additional reading: Open Source Html Text Editor
Injecting into Components
You can inject HTML into React components using JSX, which resembles HTML tags within JavaScript. This JSX code is then transformed into HTML elements that are rendered on the web page.
To inject HTML safely, use the dangerouslySetInnerHTML property, which sets raw HTML directly from a string. This property must be used with caution to prevent cross-site scripting attacks.
You can create a function that returns an object with a property __html that contains the HTML string. The dangerouslySetInnerHTML attribute then renders this HTML content.
React components can also be exported as static HTML using server-side rendering techniques or tools like ReactDOMServer. This is achieved by using ReactDOMServer.renderToString to convert the react component into a string of HTML.
For more complex scenarios, you might need to use advanced techniques to integrate HTML and React. This could involve using JSX code, template literals, or JavaScript to manipulate HTML strings.
To render HTML content using JSX, developers define a simple React component that returns an HTML element with some text. This JSX code is then transformed into HTML elements that are rendered on the web page.
The dangerouslySetInnerHTML property can be used to inject HTML into React components, but it should be used with caution to prevent cross-site scripting attacks. This property sets raw HTML directly from a string.
Template literals and JSX can be combined to create complex HTML structures within React components. This is achieved by using a template literal to inject a variable into an HTML string, which is then rendered using dangerouslySetInnerHTML.
To safely render, create, and manipulate HTML within React components, developers can use the dangerouslySetInnerHTML property and template literals with JSX. This will enhance the user experience while maintaining the integrity of their applications.
Related reading: Code to Justify Text in Html
Implementing DOMPurify for Secure Rendering
DOMPurify is a widely-used library that helps to sanitize and secure HTML content in web applications.
It can be easily installed and integrated into a React project.
To use DOMPurify, you'll need to import the library and use its sanitize method to clean a string of HTML content.
This ensures the HTML is safe to render in your React application.
DOMPurify can be used to prevent XSS attacks by sanitizing any HTML strings that are inserted into your application.
It's a robust solution for complex applications that require secure rendering.
By using DOMPurify, you can rest assured that your React application is secure and performant.
Dynamic Content
Dynamic content is a common requirement in modern web applications. Handling dynamic HTML content can be a challenge, but React's state management can be used to update the HTML content displayed to the user.
React's state management system allows for seamless updates to dynamic content. This means you can easily change the HTML content displayed to the user without having to reload the page.
In React, dynamic HTML content is often used to display user-generated content, such as comments or posts. This type of content requires frequent updates, making React's state management system a valuable tool.
React's ability to update dynamic content in real-time makes it a popular choice for web applications that require fast and efficient updates.
Integration Techniques
You can integrate external HTML files into your React application by importing them directly, making it easy to reuse templates or snippets from external sources.
For more complex scenarios, you might need to use advanced techniques like JSX code, template literals, or JavaScript to manipulate HTML strings, allowing you to customize and transform the HTML as needed.
Sometimes, you might want to convert text to HTML within your React application, which can be achieved by using JavaScript to manipulate HTML strings or by using JSX code to create dynamic HTML elements.
Advanced techniques for HTML and React integration can also involve using template literals, which allow you to embed expressions inside string literals, making it easier to create dynamic HTML content.
In React, you can use JSX code to create dynamic HTML elements, which can be a powerful tool for converting text to HTML within your application.
Explore further: Editor Html Javascript
Conclusion: Mastering Injection
Mastering the art of HTML injection in React is crucial for building dynamic and secure web applications. This is because it allows developers to enhance the user experience while maintaining the integrity of their applications.
By understanding how to safely render, create, and manipulate HTML within React components, developers can achieve a dynamic and engaging user experience.
To build dynamic web applications, developers must learn how to master HTML injection in React. This involves understanding the best practices for rendering, creating, and manipulating HTML within React components.
Understanding how to safely render HTML within React components is essential for building secure web applications. This is because it helps prevent common web vulnerabilities, such as cross-site scripting (XSS) attacks.
Mastering HTML injection in React requires a combination of knowledge and practice. Developers must be able to safely render, create, and manipulate HTML within React components to achieve a dynamic and engaging user experience.
Frequently Asked Questions
How to convert string to HTML code in React?
To convert a string to HTML code in React, use the "dangerouslySetInnerHTML" attribute or consider using a library for safer alternatives.
How do I convert a text file to HTML?
To convert a text file to HTML, simply drag and drop your TXT file onto the page and select HTML as the target format. Your HTML file will be ready for download once MConverter finishes processing it.
Can I convert React to HTML?
Yes, React apps can be converted to static HTML, CSS, and JS, making it possible to deploy them without a server. This process is included in the default setup using Create React App.
Sources
- https://reactnative.dev/docs/text
- https://blixtdev.com/how-to-use-contenteditable-with-react/
- https://www.smashingmagazine.com/2024/04/converting-text-encoded-html-vanilla-javascript/
- https://www.dhiwise.com/post/react-inject-html-a-comprehensive-guide
- https://stackoverflow.com/questions/19266197/reactjs-convert-html-string-to-jsx
Featured Images: pexels.com