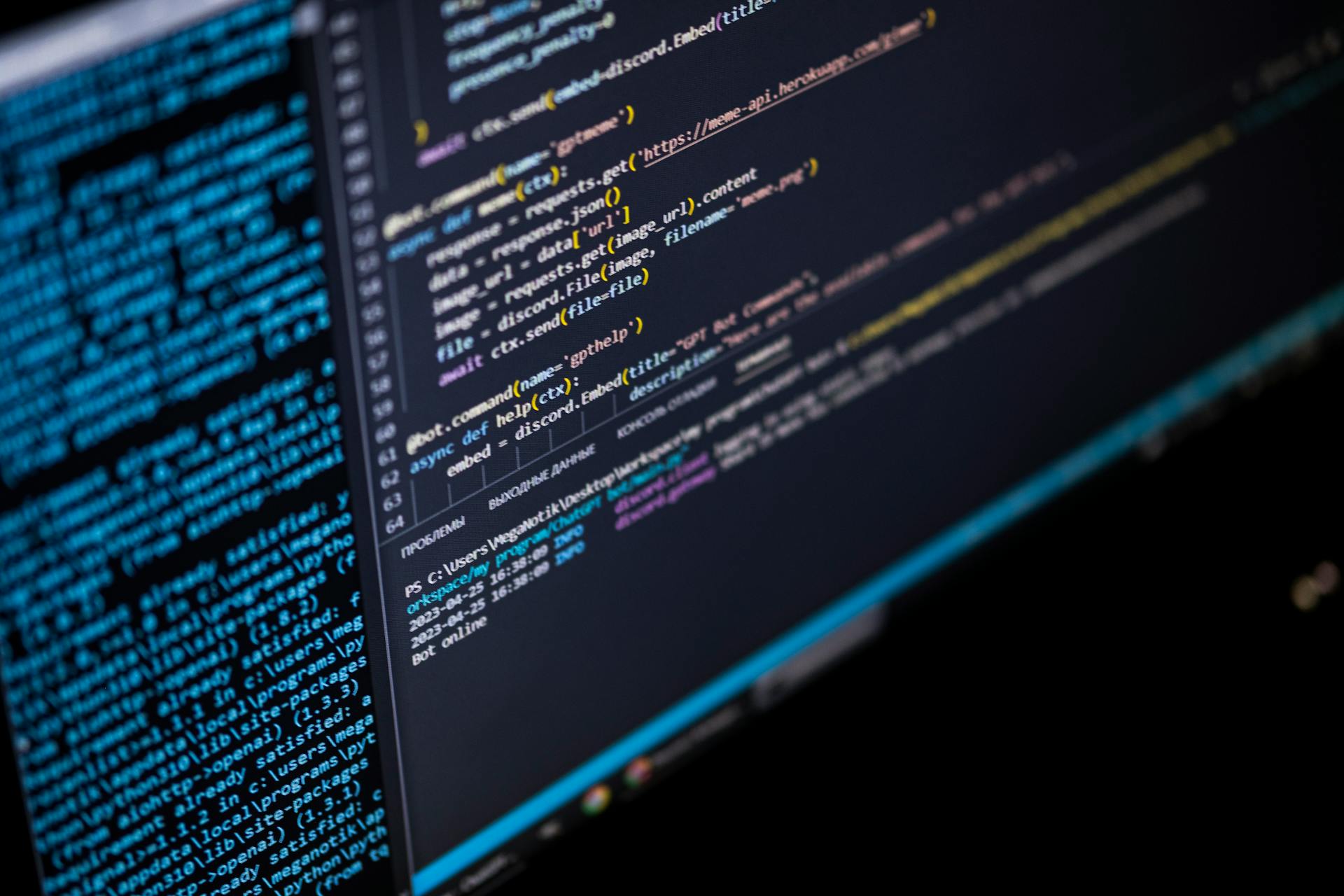
React Query DevTools is a game-changer for managing state in your React applications. It provides a visual representation of your data fetching and caching, making it easier to identify and debug issues.
With React Query DevTools, you can see the entire cache in one place, including the data, status, and error messages for each query. This makes it easy to identify and fix problems.
Using the DevTools, you can also see the query history, which shows you all the queries that have been executed, including the data and status for each one. This is especially useful for debugging issues.
By mastering React Query DevTools, you can improve the performance and reliability of your React applications.
For another approach, see: Data Lake Query
Getting Started
React Query Devtools can be installed using npm or yarn by running the command `npm install --save-dev @react-query/devtools` or `yarn add @react-query/devtools --dev`.
To use the Devtools, you need to wrap your app with the `QueryClientProvider` component, which will make the Devtools available in the React DevTools.
A unique perspective: React Web Dev
The Devtools can be opened in the React DevTools by clicking on the "React Query" tab and then clicking on the "Open Devtools" button.
React Query Devtools can be configured by passing options to the `useQueryClient` hook, such as `useQueryClient({ defaultOptions: { queries: { staleTime: 30000 } } })`.
To see the queries in the Devtools, you need to enable the "Show Queries" option in the React Devtools settings.
The Devtools also display the query status, which can be one of "loading", "success", or "error".
A fresh viewpoint: Azure Boards Queries
Core Concepts
React Query Devtools is a powerful tool that helps you understand and optimize your application's data fetching and caching behavior. It provides a visual representation of your data flow, making it easier to identify performance bottlenecks and debug issues.
The core concepts of React Query Devtools are built around the idea of queries, which are essentially requests for data from your application. These queries can be thought of as a pipeline of operations that fetch data from various sources, such as APIs, local storage, or even other components.
React Query Devtools allows you to visualize and manage these queries in real-time, giving you a clear understanding of what's happening behind the scenes. By doing so, you can optimize your queries to improve performance, reduce latency, and enhance the overall user experience.
Additional reading: Media Queries for Responsive Web Design
What Is
Let's break down the core concept of what it means to be a leader. A leader is someone who has the ability to inspire and motivate others to work towards a common goal. They are responsible for making tough decisions and taking calculated risks to achieve success.
Effective leaders are able to communicate their vision clearly and concisely, which is essential for building trust and credibility with their team. This is often achieved through active listening and open communication.
Strong leaders are adaptable and able to adjust their approach as needed to overcome obstacles and challenges. They are also resilient and able to bounce back from setbacks and failures.
How to Set Up
Setting up React Query DevTools is pretty easy. You can hook up a button or keyboard shortcut to toggle the visibility of the devtools.
It's a simple setup, as mentioned in the example. Integrating React Query DevTools is straightforward, and you can have it up and running in no time.
Cache Management
Cache Management is a powerful feature of React Query that allows you to inspect and manipulate the current cache. This can be super helpful for simulating and debugging various scenarios.
You can remove specific queries or clear the entire cache, which is especially useful when testing different scenarios. With this feature, you can see exactly how your app behaves under different conditions.
React Query DevTools even lets you inspect the current cache, which is a huge time-saver when debugging. This can help you identify issues and make changes to your code more efficiently.
Discover more: Next Js Cache
Managing Loading States
Managing Loading States is a crucial aspect of building a seamless user experience.
useQuery gives us more ways to handle loading, like: isIdle - It hasn't started fetching yetisLoading - It's fetchingisSuccess - It got the dataisError - Something went wrong
These states help us manage different stages of fetching data.
If there's an error, we can show it using the error property.
Create a UseSaveProject Custom Hook
To create a useSaveProject custom hook, you'll need to use React Query's useMutation. This hook allows you to handle complex data mutations in a predictable way.
The useSaveProject hook is created by importing the useQueryClient function and using it to create a mutation with the mutationFn property set to projectAPI.put(project). This function is called whenever the saveProject function is invoked.
Here's a basic example of how the useSaveProject hook is defined:
```html
export function useSaveProject() {
const queryClient = useQueryClient();
return useMutation({
mutationFn: (project) => projectAPI.put(project),
onSuccess: () => queryClient.invalidateQueries(['projects']),
});
}
```
The hook returns an object with two properties: mutate and isLoading. The mutate property is a function that can be used to save a project, and the isLoading property indicates whether the save operation is in progress.
To use the useSaveProject hook, you'll need to import it into the component where you want to save a project. In the example, the ProjectForm component imports the useSaveProject hook and uses it to save the project when the form is submitted.
```html
const { mutate: saveProject, isLoading } = useSaveProject();
```
The saveProject function is then called when the form is submitted, and the isLoading property is used to display a saving toast indicator while the save operation is in progress.
Explore further: Next Js vs Create React App
Debugging and Optimization
Debugging stale data can be a real pain, but DevTools can help. Use it to inspect the query's stale time and refetch intervals.
Monitoring the timeline is also crucial for optimizing performance. By spotting unnecessary refetches or overlapping queries, you can guide optimizations to reduce network requests and improve app responsiveness.
React Query Devtools has some amazing tools for debugging and fixing data fetching issues in real-time. These tools let you see what's happening with your data, making it easier to identify and fix problems.
Explorer
The Explorer feature is a game-changer for debugging and optimization. It offers a list of all the queries in use, allowing you to view their status, data, and other relevant details.
You can click on a query to reveal further insights into its cache lifecycle and refetch interval. This level of detail is invaluable for identifying and fixing issues.
The Explorer feature is part of a broader effort to build the next generation of data visualization.
Debugging
Debugging is a crucial part of the development process. Use DevTools to inspect the query's stale time and refetch intervals if you suspect data is becoming stale unexpectedly.
You can spot unnecessary refetches or overlapping queries by monitoring the timeline. This can guide optimizations to reduce network requests and improve app responsiveness.
React Query Devtools offer a range of helpful tools for checking and fixing your data fetching. These tools let you see what's happening with your data in real time.
The query explorer is a valuable feature that provides a list of all the queries in use. You can view their status, data, and other relevant details, and clicking on a query reveals further insights into its cache lifecycle and refetch interval.
If you suspect an issue with a specific query or want to manually trigger it, you can force a refetch using the 'refetch' button.
Take a look at this: Can I Use React Query with Nextjs
Featured Images: pexels.com