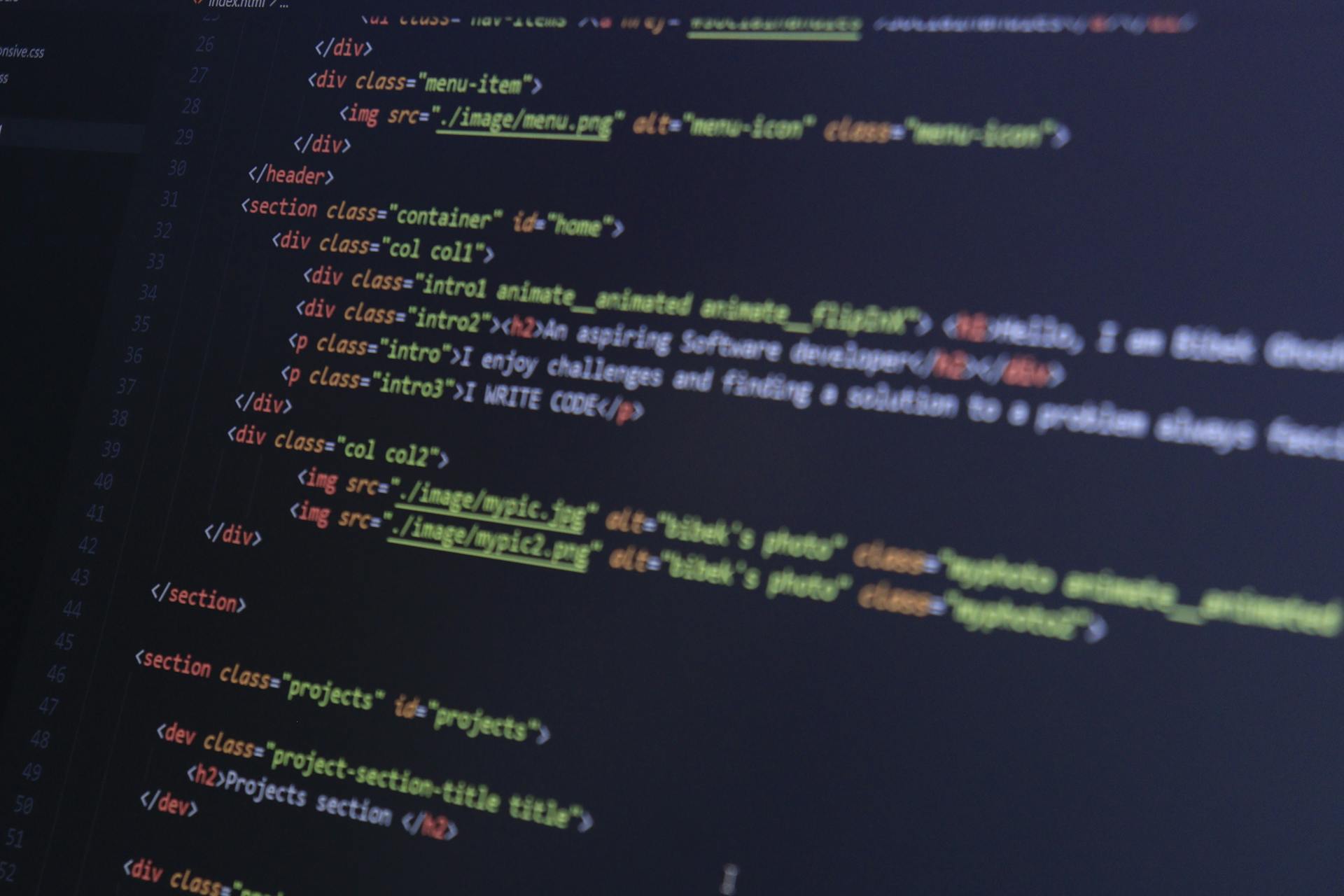
To set up Tailwind CSS with React, you'll need to install the necessary packages. This involves running the command `npm install tailwindcss postcss autoprefixer` in your terminal.
Tailwind CSS is a utility-first CSS framework that allows you to write more concise and maintainable CSS code. Its configuration file, `tailwind.config.js`, is where you'll customize the framework's settings.
In the `tailwind.config.js` file, you can configure the default font family, colors, and spacing. For example, you can set the default font family to `font-family: 'Open Sans', sans-serif;`.
To integrate Tailwind CSS with your React app, you'll need to create a PostCSS configuration file, `postcss.config.js`. This file will help you compile and process your CSS code.
A unique perspective: Tailwind Css Examples Code
Project Setup
To set up a React project, you can create a new React application using Create React App. Make sure you have a React project set up before integrating Tailwind CSS.
Once your React project is ready, you can start integrating Tailwind CSS. You can do this by installing Tailwind and its dependencies using npm, running the command npm install tailwindcss postcss autoprefixer. This will install the necessary packages for Tailwind CSS to work.
Before using Tailwind CSS, you need to configure PostCSS, which is a tool for transforming styles with JS plugins. You can create a postcss.config.js file in your project's root directory with the following content to ensure that PostCSS processes your CSS files correctly.
Readers also liked: Tailwind Css Vite React
Configure Template Paths
To configure template paths in Tailwind CSS, you'll need to add them to the tailwind.config.js file. This is where you tell Tailwind which files to check for CSS classes.
You can do this by adding the template paths under the content key in tailwind.config.js. This allows Tailwind to identify and remove unused classes, which reduces the size of the generated CSS.
For example, after running the command npx tailwindcss init -p, you can add the template paths to the tailwind.config.js file.
For another approach, see: Bootstrap and Node Js
Initialize
To initialize your project, you'll want to create a Tailwind CSS configuration file by running `npx tailwindcss init -p`. This command generates a `tailwind.config.js` file in your project's root directory.
Next, you can run the `tailwind init` command to generate Tailwind CSS default configuration files. This creates `tailwind.config.js` in the root folder, which stores all of Tailwind's configuration files.
Make sure to install Tailwind CSS and its dependencies using npm by running `npm install tailwindcss postcss autoprefixer`. This will get you set up with the necessary tools to start customizing Tailwind.
Creating a `tailwind.css` file is also a good idea, as well as an `index.css` file, which you can use to import Tailwind CSS at the beginning of the file.
Related reading: Tailwindcss Install Css
Integrating with Tailwind CSS
To integrate Tailwind CSS into your React project, follow these steps. First, install Tailwind and its dependencies. Create React App does not support PostCSS 8 yet, so you'll install the version of PostCSS 7 that is compatible with Tailwind CSS v2.
You'll get a file that matches the default configuration file Tailwind uses internally. Inside that folder, create a tailwind.css and an index.css file. The index.css file will store the styles generated by Tailwind CSS.
With Tailwind CSS, you don't need to write the CSS rules for each class. Instead, you use utility classes. These are classes scoped to a single CSS property. For instance, if you want to create a button with a black background and white text color, you need to use the bg-black and text-white utility classes.
Here's a quick rundown of the main Tailwind CSS directives:
- @tailwind base injects Tailwind's base styles.
- @tailwind components injects any component classes registered by plugins defined in your tailwind config file.
- @tailwind utilities injects all of Tailwind's utility classes.
These directives are used to inject the default base styles, components, utilities, and custom configurations into your CSS file.
Navbar Component
To build a navbar with React and Tailwind CSS, you'll first need to create a Navbar component. This can be done by creating a new React component in a file like Navbar.js.
In this component, you can use Tailwind CSS classes to style the navbar and make it responsive, meaning it will be hidden on small screens.
Navbar Component
To create a Navbar Component, you can place it in a file like Navbar.js. This component is a crucial part of any web application, providing a navigation menu for users to access different sections of the site.
In the component, you use Tailwind CSS classes to style the navbar, make it responsive, and apply hover effects to the links. Tailwind CSS classes are a game-changer for styling React components, allowing you to write less code that's easy to understand.
You can start by creating a new React component for the navbar, which will serve as the foundation for your navigation menu. This component will be used throughout your application to provide a consistent navigation experience for users.
Recommended read: Tailwind Css Class
Import Navbar Component
Importing the Navbar Component is a crucial step in using it in your application. You can import the Navbar component in your main layout component or where you want to display the navbar.
To import the Navbar component, you'll need to use the import statement in your code. This is done by typing 'import Navbar from './Navbar';' in your code file, as seen in example code.
You can place the imported Navbar component in your main layout component, giving your application a consistent look and feel. This is often where you'll want to display the navbar.
The Navbar component can be imported in any file where you want to use it, making it a flexible tool for building your application.
Discover more: Tailwindcss Cards
Styling and Customization
Tailwind CSS provides extensive customization options through the tailwind.config.js file, allowing you to modify colors, fonts, spacing, and more to match your project's design requirements.
You can change the colors, font family, and more in tailwind.config.js, giving you full control over the theme.
On a similar theme: Npm Next Js
To change the colors, you can define your own colors or modify the default colors. For example, you can add a new color like turquoise by adding it to the theme.extend.colors object in tailwind.config.js.
You can also specify the value for different shades of a color using numeric scales or keys like lightest, light, DEFAULT, dark, darkest.
Tailwind CSS also allows you to customize the font family and more, all from the same tailwind.config.js file.
Here are some ways to customize the theme's colors:
Advanced Configuration
To get the most out of Tailwind CSS, you need to configure it properly. This involves creating a Tailwind CSS configuration file by running `npx tailwindcss init -p`, which generates a `tailwind.config.js` file in your project's root directory.
You also need to configure PostCSS, which involves creating a `postcss.config.js` file with specific content. This ensures that PostCSS processes your CSS files correctly.
To optimize your project's CSS, you should configure template paths in Tailwind CSS. This involves adding the template paths under the `content` key in your `tailwind.config.js` file.
Frequently Asked Questions
Is Tailwind better than bootstrap for React?
For React projects, Tailwind CSS is a better choice if you want to customize your design and eliminate unused styles, while Bootstrap is a good option if you need a more straightforward and widely-supported framework.
Can we use CSS in Reactjs?
Yes, we can use CSS in Reactjs, and there are several ways to do it, including CSS-in-JS libraries like styled-components and Emotion.
Sources
- https://www.yourteaminindia.com/tech-insights/guide-to-using-tailwind-css-with-react
- https://www.makeuseof.com/tailwind-css-in-react/
- https://www.mikefrancis.dev/blog/create-react-app-tailwind-css
- https://www.sitepoint.com/react-tailwind-css-build-site/
- https://blog.logrocket.com/tailwind-css-configure-create-react-app/
Featured Images: pexels.com