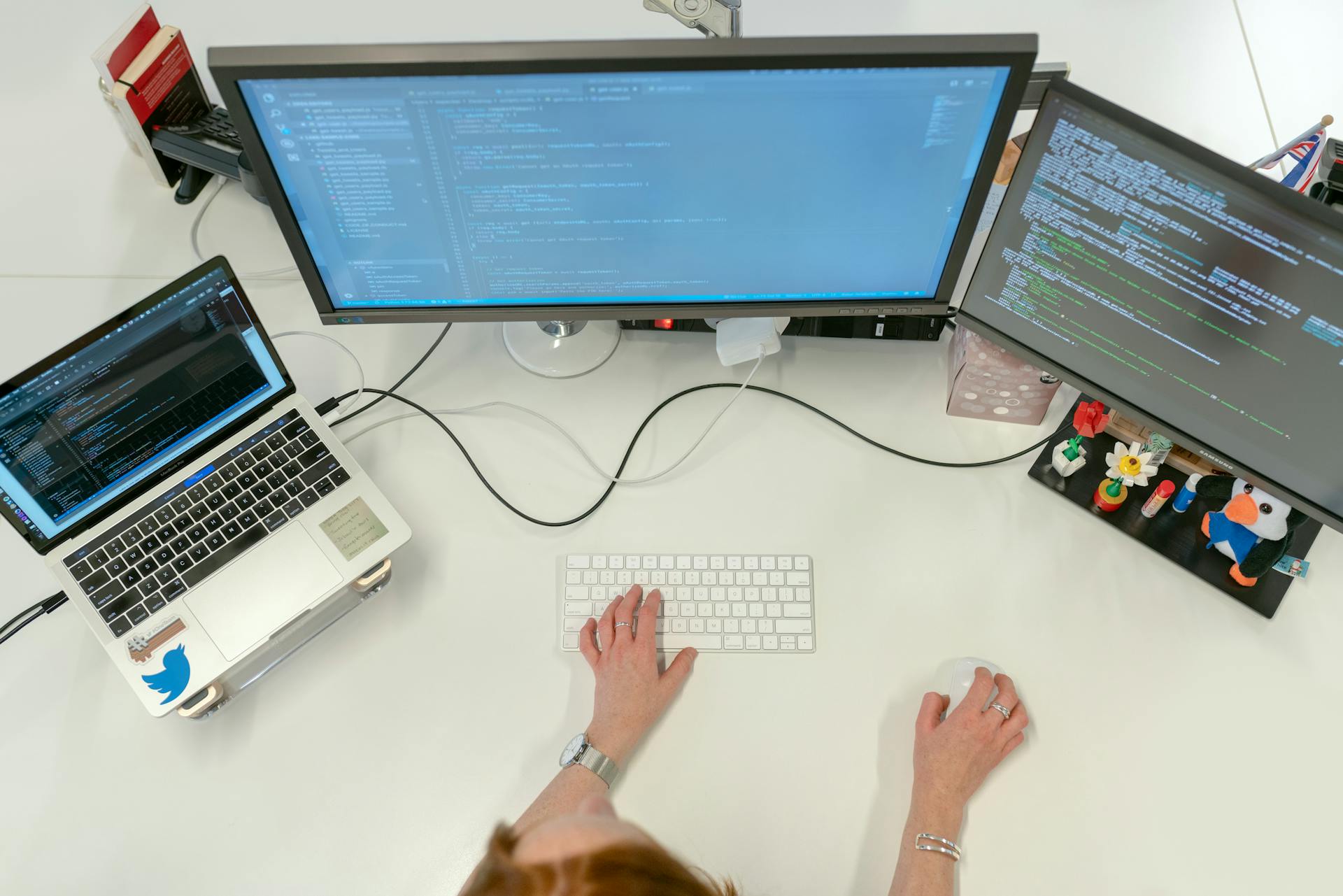
To get started with the Redux DevTools Extension, you'll first need to install it as an extension in your web browser. This can be done through the Chrome Web Store or Mozilla Add-ons.
The Redux DevTools Extension is compatible with most modern browsers, including Chrome, Firefox, and Edge.
For Chrome users, navigate to the Chrome Web Store, search for "Redux DevTools", and click the "Add to Chrome" button. For Firefox users, head to Mozilla Add-ons, search for "Redux DevTools", and click the "Add to Firefox" button.
Check this out: Responsive Web Design Chrome Extension
What is Redux DevTools Extension
The Redux DevTools Extension is a browser extension that helps you debug and understand the state of your Redux application. It provides a graphical representation of the state tree, making it easier to identify and fix issues.
It's available as a separate download from the official Redux website.
On a similar theme: Redux Nextjs
Installation and Setup
To get started with the Redux DevTools Extension, you'll need to install the redux-devtools-extension package from npm.
This package makes things easier by providing a convenient way to install the devToolsEnhancer. Simply run the command in your terminal to install it.
The devToolsEnhancer is a key part of setting up the Redux DevTools Extension, and it's what allows you to use the extension's features.
Install Npm Package
To install the redux-devtools-extension package, simply run the command "npm install redux-devtools-extension" in your terminal or command prompt. This package makes it easier to get started with Redux.
The package can be used by importing it into your project and using it like so: devToolsEnhancer.
For Electron
For Electron, you'll want to make sure you have the necessary tools installed. Node.js is a must-have, as it's the runtime environment for Electron.
Electron uses Chromium as its rendering engine, which means you can use all the great features of Chrome in your app, including its rendering engine and APIs. The Electron framework is built on top of Node.js, making it easy to create cross-platform desktop apps.
You can use the Electron CLI to create a new project, which will give you a basic directory structure and a few example files to get you started. This will save you time and effort in setting up your project.
Integrating Redux with Angular
To integrate Redux with Angular, you'll need to install the @ngrx/store package. This package is the core of the Redux library for Angular and provides a simple way to manage global state.
The @ngrx/store package is a dependency of the @ngrx/effects and @ngrx/entity packages, so you'll need to install it first. You can install it using npm or yarn by running the command npm install @ngrx/store or yarn add @ngrx/store.
Once installed, you can import the StoreModule in your app module to enable the Redux store. This will allow you to manage global state across your application.
For example, you can import the StoreModule in your app module like this: import { StoreModule } from '@ngrx/store'; @NgModule({ imports: [ StoreModule.forRoot(reducers)], ... }) export class AppModule { }
Using Redux DevTools Extension
Redux DevTools Extension is a powerful tool that helps you debug and optimize your Redux application. It's automatically set up by Redux Toolkit's configureStore, which calls createStore with the Redux DevTools Extension connection.
In development, Redux DevTools Extension is automatically added to your Redux store by configureStore. This means you don't have to worry about setting it up yourself.
Here's a brief overview of what Redux DevTools Extension can do:
- Inspect the state of your Redux store
- Time travel through your app's history
- Identify and fix common mistakes like state mutations
By using Redux DevTools Extension, you'll be able to catch and fix issues in your application more efficiently, making your development process smoother and more productive.
Dev Tools
The Redux DevTools Extension is a powerful tool for monitoring and debugging your Redux application. It allows you to commit the current set of actions, so you can monitor state across page transitions.
You can commit actions from the Inspector mode, and once committed, you can revert back to this state anytime. This feature is especially useful for large-scale applications with hundreds of actions.
To enable support for the Redux DevTools browser extension, you can pass a boolean value to configureStore. If you pass an object, the DevTools Extension will be enabled, and the options object will be passed to composeWithDevtools().
Here's a quick rundown of the options you can pass to composeWithDevtools():
The DevTools Extension can be used in various ways, including monitoring state, committing actions, and reverting back to previous states. It's a valuable tool for any Redux developer.
Using in Production
You can restrict Redux DevTools to only work in production by using the logOnlyInProduction feature, which allows you to log only in production.
To use it in production, you'll need to add 'process.env.NODE_ENV': JSON.stringify('production') to your Webpack config for the production bundle.
If you're already checking process.env.NODE_ENV when creating the store, include the logOnly option for production environment.
It's also possible to use the developmentOnly feature to completely disable the extension in production.
Using Redux DevTools in production can actually be helpful for debugging issues that arise after deployment.
Multi-Platform Apps
If you're building a multi-platform app, you've got some options for using Redux DevTools Extension. For React Native, you can use react-native-debugger, which already includes the same API as Redux DevTools Extension.
To set up remote monitoring for most platforms, include Remote Redux DevTools's store enhancer. From the extension's context menu, choose 'Open Remote DevTools' for remote monitoring.
Intriguing read: React Query Devtools
Tracing Editor Actions
The Redux DevTools Extension allows you to see the call stack that triggered an action, making it easier to debug complex issues.
This feature is especially useful in large-scale applications where the same actions are triggered simultaneously from different parts of the app. By selecting any action from the history, you can see the cause of the action dispatch.
The trace feature in Redux DevTools can be configured to capture the call stack for specific actions only, which can help keep the development experience smooth and performant.
You can also define a custom implementation for the trace feature, which can be helpful for action dispatched via side effect libraries like redux-saga or other event listeners.
By using the trace feature, you can navigate to the exact point of the codebase where the action was dispatched, saving you time and effort in debugging.
In addition, the Redux DevTools Extension allows you to disable or remove an action from the timeline, which is useful when analyzing application behavior.
Frequently Asked Questions
How to install Redux devtools extension in VS Code?
To install Redux Devtools in VS Code, open the Command Palette with CMD + Shift + P and select "Open Devtool to the Side". This will allow you to configure the extension and connect to your Remotedev server.
Sources
Featured Images: pexels.com