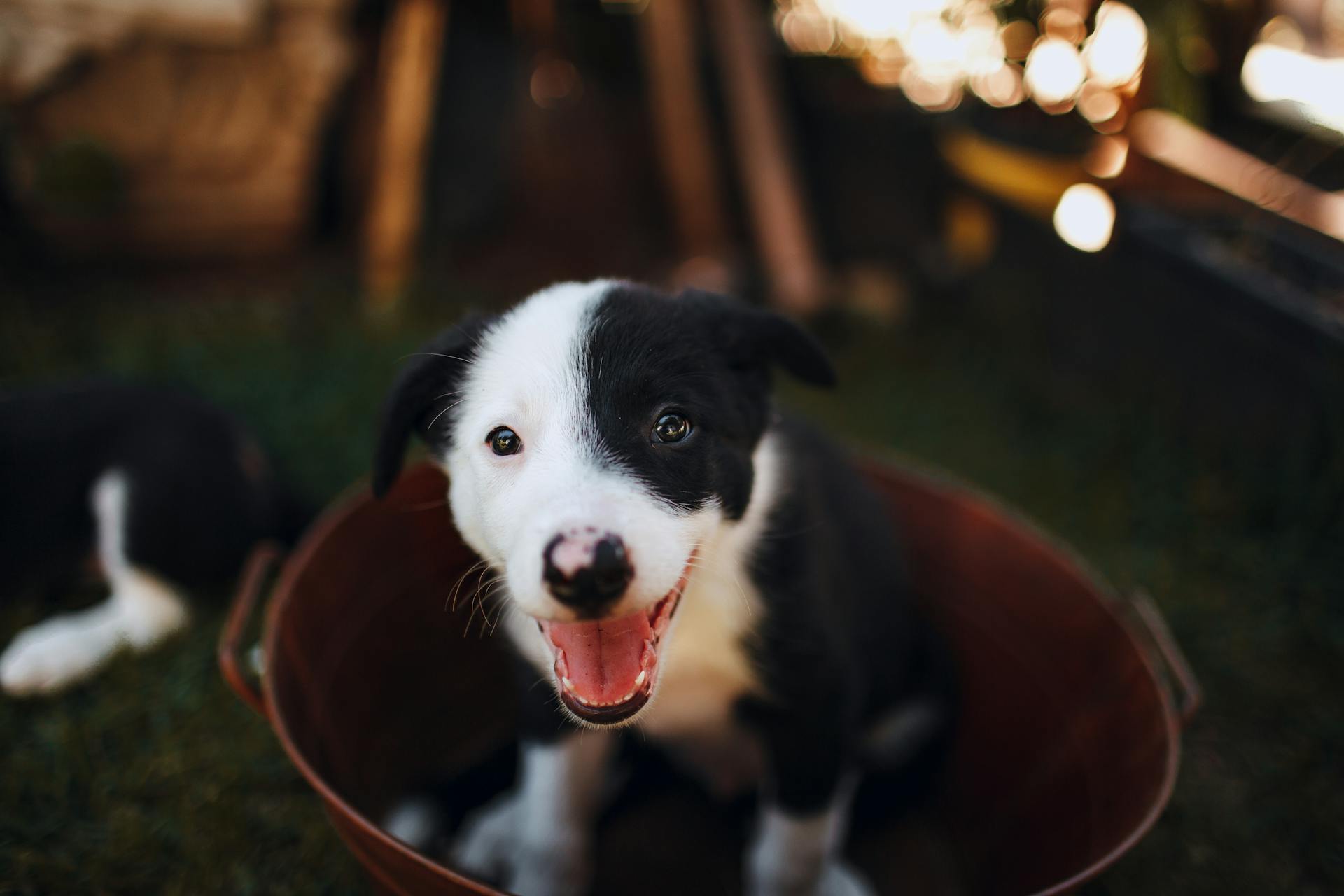
Boto is a Python library that makes it easy to interact with AWS services, including S3.
S3 stands for Simple Storage Service, which is a cloud-based object storage service that can store any type of data.
You can use S3 to store and serve web content, such as images and videos, as well as to store and retrieve large files.
To get started with S3 Boto Bucket Tutorial for Python Developers, you'll need to install the Boto library using pip.
Prerequisites
To start automating Amazon S3 operations, you'll need to configure your Python environment. You'll need to have Python 3 installed.
Python 3 is a must-have for working with Amazon S3. Make sure you have it installed on your machine.
You'll also need to install Boto3, which is a client that allows you to access the low-level API data. This includes accessing API response data in JSON format.
Boto3 is a powerful tool that will make your life easier when working with Amazon S3. You can access API response data in JSON format using Boto3.
Additionally, you'll need to install AWS CLI tools. These tools will allow you to interact with Amazon S3 and other AWS services.
AWS CLI tools are a must-have for any AWS developer. They'll make it easy to interact with Amazon S3 and other AWS services.
Here's a summary of the prerequisites you'll need to get started:
- Python 3
- Boto3
- AWS CLI tools
Having these prerequisites installed will ensure that you can successfully automate Amazon S3 operations and make API calls to the Amazon S3 service.
Getting Started
To get started with S3 boto bucket, you'll need to connect to S3 using Boto3.
You can instantiate the Boto3 client to start working with Amazon S3 APIs by using the boto3.resource method.
To start managing the Amazon S3 service, you can instantiate the Boto3 S3 client or resource in your code.
This will give you access to the S3 APIs, allowing you to start working with your bucket right away.
As soon as you've connected to S3, you can start managing your bucket and its contents.
You can use the Boto3 client to upload, download, and delete files from your S3 bucket.
Creating and Managing Resources
You can list existing S3 Buckets using the S3 resource with Boto3. This will show you all the buckets currently available in your account.
To create and delete an S3 Bucket, you'll need to create a "config.properties" file with your AWS User credentials and region. Then, you can create a bucket with a unique name using the "create-s3-bucket.py" script, and delete it with the "delete-s3-bucket.py" script.
Every Amazon S3 Bucket must have a unique name, which must be unique across all AWS accounts and customers.
Creating Resource
Creating a resource is a crucial step in managing your Amazon S3 bucket. To avoid various exceptions, you should define a specific AWS Region from its default region for both the client and the S3 Bucket Configuration.
You can use the Boto3 client or resource to create an Amazon S3 bucket. The Boto3 client requires defining a specific AWS Region, while the Boto3 resource uses the list_buckets() method of the client resource or the all() method of the S3 buckets resource.
When creating a bucket, you need to specify a unique name that is unique across all AWS accounts and customers. This is a requirement for every Amazon S3 Bucket.
You can create a bucket using the Boto3 client with the create_bucket() method, or using the Boto3 resource with the create_bucket() method. The create_bucket() method allows you to specify the bucket name and other configuration options.
Here are the methods you can use to create a bucket:
- create_bucket() method of the Boto3 client
- create_bucket() method of the Boto3 resource
Remember to define a specific AWS Region for both the client and the S3 Bucket Configuration to avoid exceptions.
Creating Client
To create a client for managing AWS resources, you'll need to define a specific AWS Region for both the client and the resource configuration. This is crucial to avoid various exceptions while working with the Amazon S3 service.
Defining a specific AWS Region is essential for both the client and the S3 Bucket Configuration. This helps prevent exceptions and ensures smooth interaction with the AWS service.
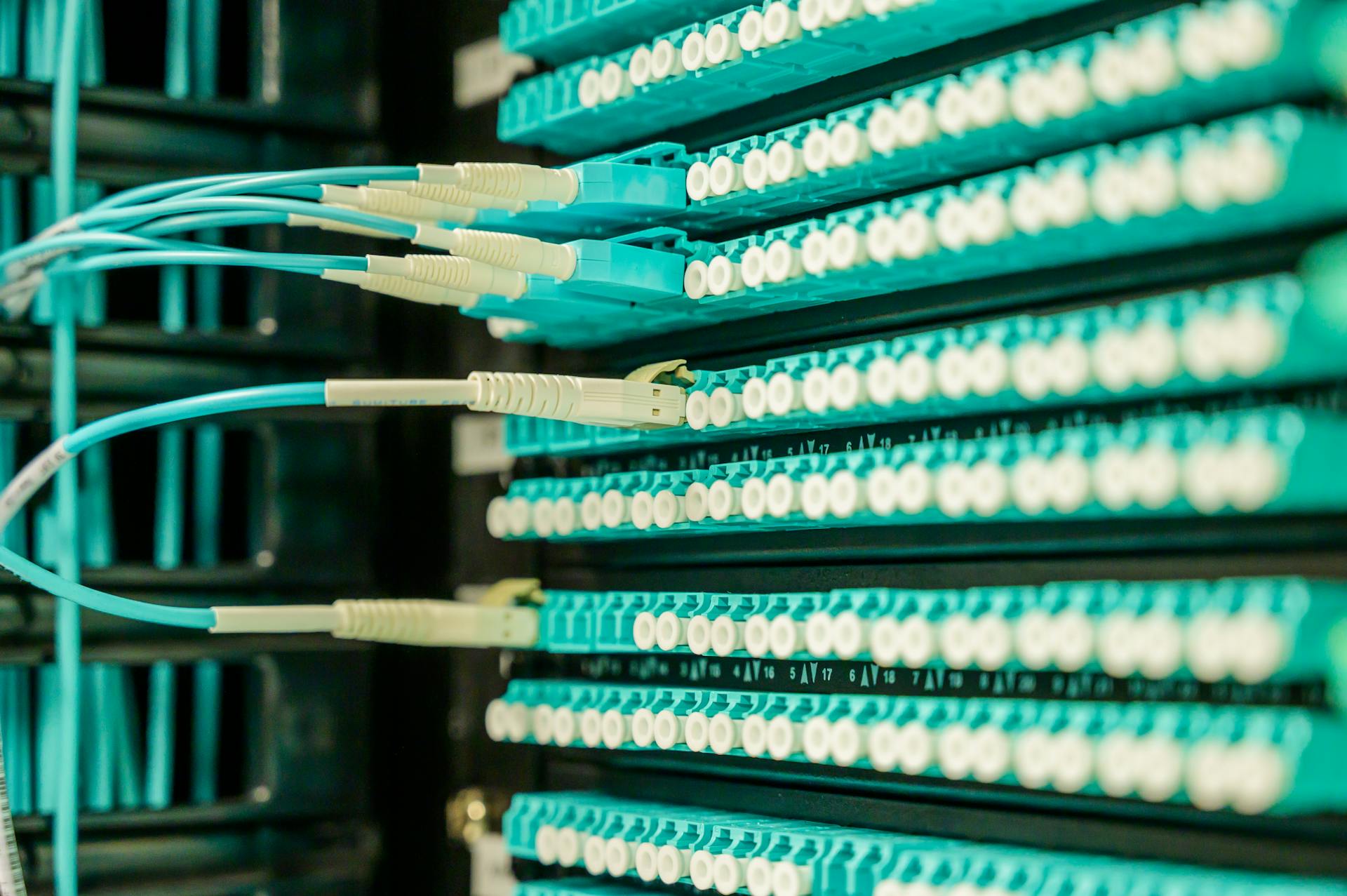
To create a Boto3 client, you can use the `boto3.session()` method. This method creates a Boto3 session that can be used to connect to AWS services.
A Boto3 client is a low-level AWS service class that provides methods to connect and access AWS services similar to the API service. You can create a Boto3 client using the `boto3.client('s3')` method.
Here are the steps to create a Boto3 client:
- Create a Boto3 session using the `boto3.session()` method
- Create the Boto3 s3 client using the `boto3.client('s3')` method
By following these steps, you'll be able to create a Boto3 client that can be used to manage AWS resources.
Copying Objects Within a
Copying objects within a single S3 bucket or between buckets is a straightforward process using Boto3.
You can use the copy_s3_object method to copy an S3 object within the same S3 bucket or between S3 buckets.
This method is a versatile tool that can be used for various tasks, including renaming S3 objects, as you'll see in the next section.
To copy an S3 object within the same S3 bucket, you can use the copy_from method, which is a part of the copy_s3_object method.
There's no need to worry about the complexity of S3 object management, as Boto3 takes care of the details for you.
The copy_s3_object method will handle the copying process, giving you more time to focus on other tasks.
You can also use the copy_from method to copy an S3 object between S3 buckets, making it a powerful tool for managing your resources.
There's no single API call to rename an S3 object, so you'll need to copy it to a new object with a new name and then delete the old object.
Enabling Versioning
Enabling Versioning is a crucial step in managing your S3 Bucket resources. To do this, you need to use the enable_version() method.
S3 Bucket versioning allows you to keep track of the S3 Bucket object's versions and its latest version over time. It safeguards against accidental object deletion of a currently existing object.
Enabling versioning for an S3 Bucket takes up storage space, with each new version of an object added to the storage size of the original file. A 2MB file with 5 versions, for example, will take up 10MB of space in the storage class.
Boto3 will retrieve the most recent version of a versioned object on request.
Attach Tags
You can attach tags to your S3 bucket to make it easier to manage and organize.
To create a bucket, you'll need to provide a unique name that follows the naming rules. This can be done using the `create_bucket` method, which takes parameters like `ACL` and `Bucket`.
If you want to add tags to your bucket, you'll need to confirm with the user first. This can be done using the `input()` function to ask the user if they want to proceed.
You can then use the `put_bucket_tagging` method to add tags to the bucket. This method takes parameters like `Bucket`, `Tagging`, `tag_key`, and `tag_value`.
Here's a step-by-step process to attach tags to your S3 bucket:
- Confirm with the user if they want to proceed with tagging the bucket.
- Ask the user to input the key and value for the tag.
- Use the `put_bucket_tagging` method to add the tag to the bucket.
Note: Make sure to check the official documentation for the `put_bucket_tagging` method to see what parameters are required.
All
Creating and managing resources with Boto3 is a breeze. You can use the Boto3 resource to create an Amazon S3 bucket using the list_buckets() method of the client resource or the all() method of the S3 buckets resource.
To list existing S3 Buckets, you can use the S3 resource or the S3 client. The S3 resource has an all() method that returns a list of all S3 buckets, while the S3 client has a list_buckets() method that does the same thing.
You can create and delete an S3 Bucket using the Boto3 resource. To do this, you'll need to create a file called "config.properties" that contains your AWS User credentials and region. Then, you can create a file called "create-s3-bucket.py" that contains the code to create a bucket, and a file called "delete-s3-bucket.py" that contains the code to delete a bucket.
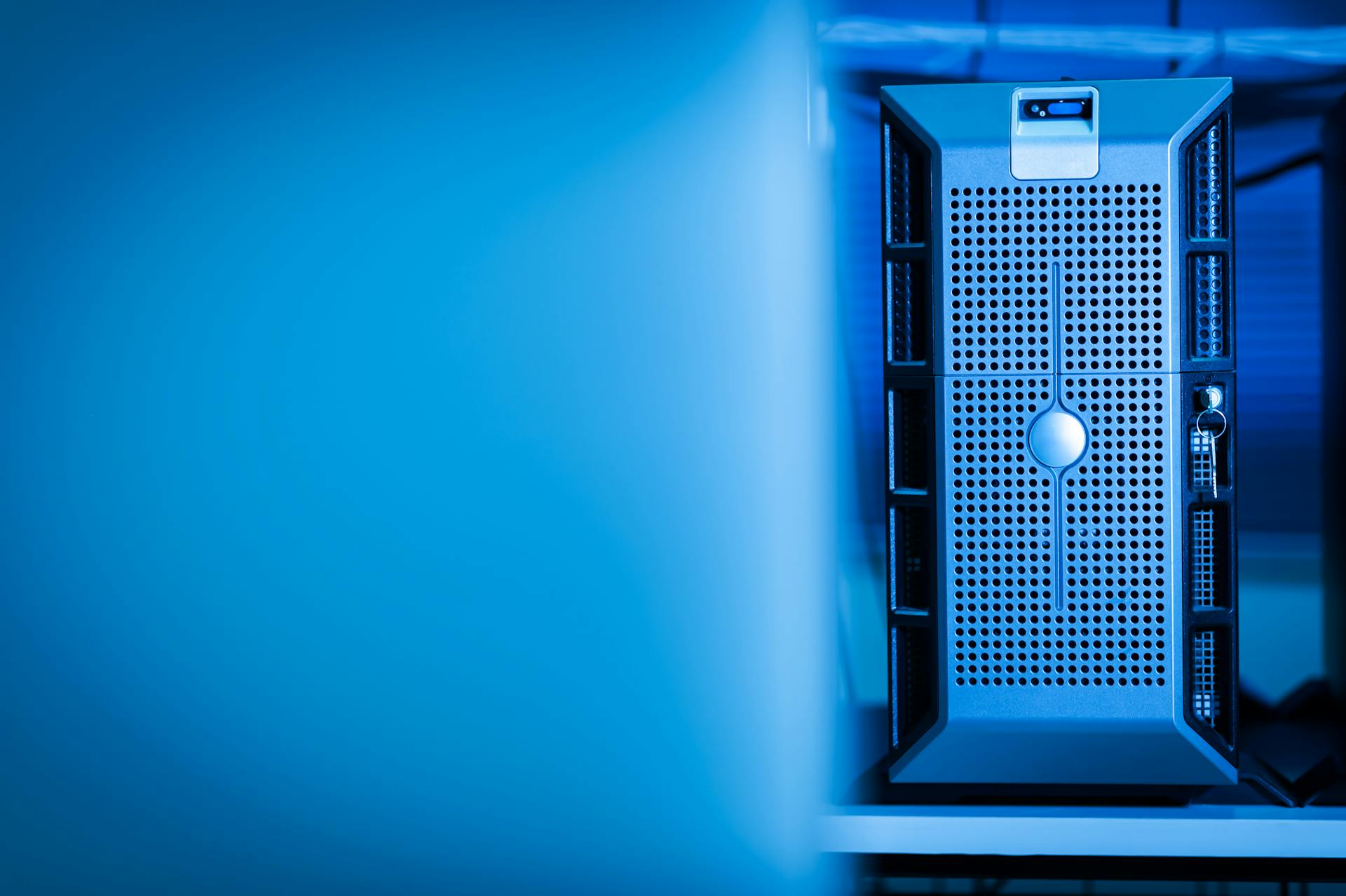
Here are the steps to create and delete a bucket:
- Create a bucket by executing the file "create-s3-bucket.py" using the command "python create-s3-bucket.py"
- Delete a bucket by executing the file "delete-s3-bucket.py" using the command "python delete-s3-bucket.py"
If you need to copy file objects within an S3 bucket, you can use the copy_s3_object() method of the Boto3 client. This method can copy objects within the same S3 bucket or between S3 buckets.
Here are some examples of how to use the copy_s3_object() method:
- Copy an object within the same S3 bucket
- Copy an object from one S3 bucket to another
Note that the copy_s3_object() method requires you to specify the source and destination buckets, as well as the object to be copied.
Frequently Asked Questions
What is a bucket in boto3?
In boto3, a bucket is a resource representing an Amazon Simple Storage Service (S3) bucket, used for storing and retrieving objects. It serves as the foundation for interacting with S3 resources in your code.
What is the use of boto3?
Boto3 is the official AWS SDK for Python, used to create, configure, and manage AWS services such as S3. It simplifies interactions with AWS resources, making it easier to build and manage cloud-based applications.
Sources
- https://hands-on.cloud/boto3/s3/
- https://www.geeksforgeeks.org/how-to-list-s3-buckets-with-boto3/
- https://www.howtoforge.com/how-to-create-an-s3-bucket-using-python-boto3-on-aws/
- https://www.dheeraj3choudhary.com/listcreate-and-delete-s3-buckets-using-python-boto3-script/
- https://dev.to/aws-builders/how-to-list-contents-of-s3-bucket-using-boto3-python-47mm
Featured Images: pexels.com