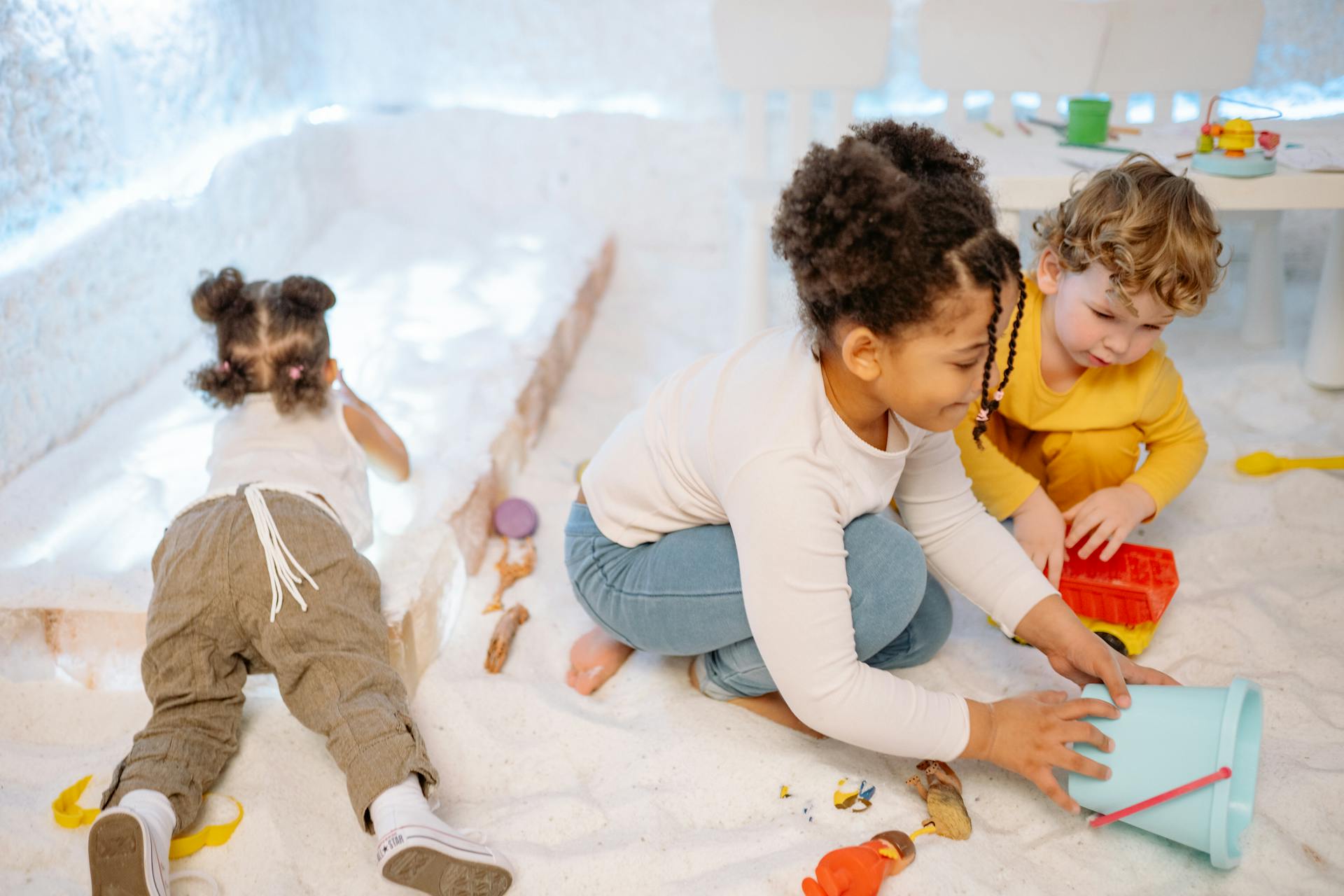
Creating an S3 bucket URL is a straightforward process, but it requires some understanding of the different components involved.
To access an S3 bucket, you need to use a URL that includes the bucket name and the object key.
The bucket name is the unique identifier for your S3 bucket, and it's used to create the URL.
You can create an S3 bucket URL by combining the bucket name with the object key in the following format: https://s3.amazonaws.com/bucket-name/object-key.
For example, if your bucket name is "my-bucket" and your object key is "image.jpg", the URL would be https://s3.amazonaws.com/my-bucket/image.jpg.
What is an S3 Bucket URL?
An S3 Bucket URL is essentially a link to a private object in your Amazon S3 bucket. This link is used to access the object, but it's not publicly accessible by default.
Pre-signed URLs are a type of S3 Bucket URL that provide short-term access to a private object. They work by appending an AWS Access Key, expiration time, and Sigv4 signature as query parameters to the S3 object.
There are two common use cases for S3 Bucket URLs: simple, occasional sharing of private files and frequent, programmatic access to view or upload a file in an application.
Here are the two main use cases for S3 Bucket URLs:
- Simple, occasional sharing of private files.
- Frequent, programmatic access to view or upload a file in an application.
Finding an S3 Bucket URL
To find an S3 bucket URL, you can click on the name of the bucket from the bucket list and choose the Overview tab for a list of the files in the bucket.
If you're looking for the URL of a specific object, you can use the search bar to locate the file, then click to the right of the file name to find the file's endpoint using the Object URL field.
The endpoint URL is also sometimes called the S3 URL.
You can find the endpoint URL by clicking on the Static Website Hosting card, where the first bit of information on the card is the endpoint address.
Some applications might ask for your bucket's endpoint, so it's good to know how to find it.
Generating a URL
Generating a URL is a straightforward process. You can find the S3 bucket URL by clicking on the bucket name, then choosing the Overview tab for a list of files in the bucket.
If you need to share a specific object, you can locate it using the search bar and click to the right of the file name, which will reveal the Object URL field in a slide-out panel.
To generate a pre-signed S3 URL, you can use the AWS Tools for Powershell, the AWS CLI, or MSP360 Explorer. The AWS Tools for Powershell allow you to use the Get-S3PreSignedURLcmdlet to generate a pre-signed URL in your Powershell.
The AWS CLI provides a simple command, aws s3 presign, to generate a pre-signed URL. On a Windows system, the command is aws s3 presign s3://bucketname/objectname.
MSP360 Explorer is another option, allowing you to generate a one-off URL by choosing the object, selecting the "Web URL" button, and configuring the expiration date.
Temporary Access and Security
Temporary access to an S3 bucket can be granted to users through pre-signed URLs, which allow for temporary, automated access in your application code.
You can generate a pre-signed URL that allows users to view an S3 object in their browser by using the GetObject API call. This URL is validated by AWS at request time, so if the server is not authorized to perform actions on the given bucket, the user will see a permission error.
The server never talks directly to S3 to generate a pre-signed URL, it uses its own set of AWS credentials, such as an IAM instance profile, to do so. Everyone with valid AWS credentials can generate a pre-signed URL using the AWS Signature v4 protocol.
Temporary Automated Access in Application Code
You can use S3 URLs for temporary, automated access in your application code. This is useful for generating short-term access to an S3 bucket, such as when users need to download or upload files.
You can perform both read and write operations with the AWS SDKs for any language. For example, you can use Boto 3, the AWS SDK for Python, to generate pre-signed URLs in your application code.
To provide temporary read access to an S3 object, you can use the GetObject API call. This allows users to view files in their browser with limited permissions.
You can generate a pre-signed URL that can be used for POST requests, allowing clients to upload large files directly from the browser. This is useful for applications that need to handle large file uploads.
For instance, you can use the generate_presigned_post method to construct an Amazon S3 URL and return it to the client. This method also allows you to add conditions, such as ensuring the file size is no larger than 1 MB.
The response will include a URL property, as well as a fields property with a set of key-value pairs. These key-value pairs must be sent with the file as part of a multipart/form-data request.
You can use the Boto 3 library to interact with S3 and generate pre-signed URLs in your application code. This makes it easy to provide temporary access to S3 objects and buckets.
Security Considerations
The server never talks directly to S3, it can generate pre-signed URLs autonomously using its own set of AWS credentials.
This is because anyone with valid AWS credentials can generate a pre-signed URL using the AWS Signature v4 protocol.
The URL is validated by AWS at request time, when a user makes an HTTP call with that URL, and AWS considers the request as if it was performed by the entity that generated the pre-signed URL.
If the server is not authorized to perform actions on a given bucket, the user will see a permission error when trying to use the generated URL.
Revoking
Revoking pre-signed URLs can be a challenge. There's no practical way to revoke individual pre-signed URLs once they're generated and shared.
You might think to invalidate credentials every time you want to invalidate a given URL, but it's highly impractical because it will invalidate all pre-signed URLs generated with the same credentials.
Short expiration times are probably the best approach, but you need to evaluate what that means in the context of your application.
File Operations
File operations with S3 bucket URLs can be done in various ways, including generating pre-signed URLs for uploading and downloading files.
You can use the AWS Tools for PowerShell, the AWS CLI, or CloudBerry Explorer to generate these URLs in an ad-hoc fashion, as mentioned in Example 1.
To generate a pre-signed URL for uploading a file, you can use the AWS Tools for PowerShell, the AWS CLI, or CloudBerry Explorer. This can be done by following the steps outlined in Example 2, which includes using the server's credentials to generate the URL.
Here are the steps to generate a pre-signed URL for uploading a file:
- Instantiate a new S3 Client
- Define a PutObject command specifying the name of the bucket and the key for which we want to authorize the upload
- Use the client and the command to get a signed URL. Optionally you can specify an expiry in seconds.
A typical PUT request using a pre-signed URL would look like this, as shown in Example 5. This includes sending a PUT request to the S3 server using the pre-signed URL and passing the Content-Length header.
Use Cases
File operations can be a complex task, but understanding the use cases can help simplify the process.
Mobile applications often require users to upload photos for their avatars, which means implementing an endpoint to allow the mobile app to upload the picture.
In cloud-based document management platforms, users need to be able to upload and download their files, while also managing access control to ensure they can only access their own files.
A marketing campaign's landing page may require users to fill out a subscription form or newsletter to receive a confirmation email with a link to download a whitepaper.
Inter-system communication workflows often involve exchanging large files, but instead of putting the payload in the message, a URL to an attachment is provided for the receiving system to download.
Here are some common use cases for file operations:
- Mobile app photo uploads
- Cloud-based document management
- Marketing campaign whitepaper downloads
- Inter-system file exchange
Upload Example
To upload a file to an S3 bucket, you can use pre-signed URLs. A pre-signed URL is a temporary URL that allows a user to upload a file directly to an S3 bucket without needing to authenticate with AWS credentials.
Here's a step-by-step process to generate a pre-signed URL for uploading a file:
1. A user communicates to the server that they intend to upload a file.
2. The server recognizes the user and verifies their authorization to perform the upload.
3. The server generates an S3 pre-signed URL for uploading an object with a predetermined name into a given S3 bucket.
4. The user receives the pre-signed URL from the server.
The pre-signed URL can be used to upload files using either a PUT or POST request. The main difference between the two is that a POST request uses the "multipart/form-data" encoding, which is a common protocol used to serialize the content of web forms with file attachments.
Here are the key differences between PUT and POST requests:
The policy mechanism in POST requests allows the signer to define rules that control what can be uploaded, such as file size or content type. This makes it a more flexible option for uploading files.
In the example of using the AWS SDK for JavaScript (v3) to perform a pre-signed POST request to S3, the `createPresignedPost` function returns a URL and a set of fields that can be used to generate a web form. This web form can be used to allow a user to upload a file to an S3 bucket using nothing else than a web browser.
Frequently Asked Questions
What is the format of the S3 URL?
The format of an S3 URL is s3://
What is the S3 endpoint URL?
The S3 endpoint URL is in the format https://s3.
What is S3 signed URL?
A pre-signed S3 URL is a temporary link to a private object in your S3 bucket, granting access to others without sharing your AWS Access Key. This secure link is ideal for occasional sharing of private files.
Sources
- https://darkroomphotos.com/how-allow-public-access-amazon-bucket/
- https://stackoverflow.com/questions/26604977/url-for-public-amazon-s3-bucket
- https://serverfault.com/questions/397290/how-to-get-a-custom-amazon-s3-url
- https://www.msp360.com/resources/blog/s3-pre-signed-url-guide/
- https://fourtheorem.com/the-illustrated-guide-to-s3-pre-signed-urls/
Featured Images: pexels.com