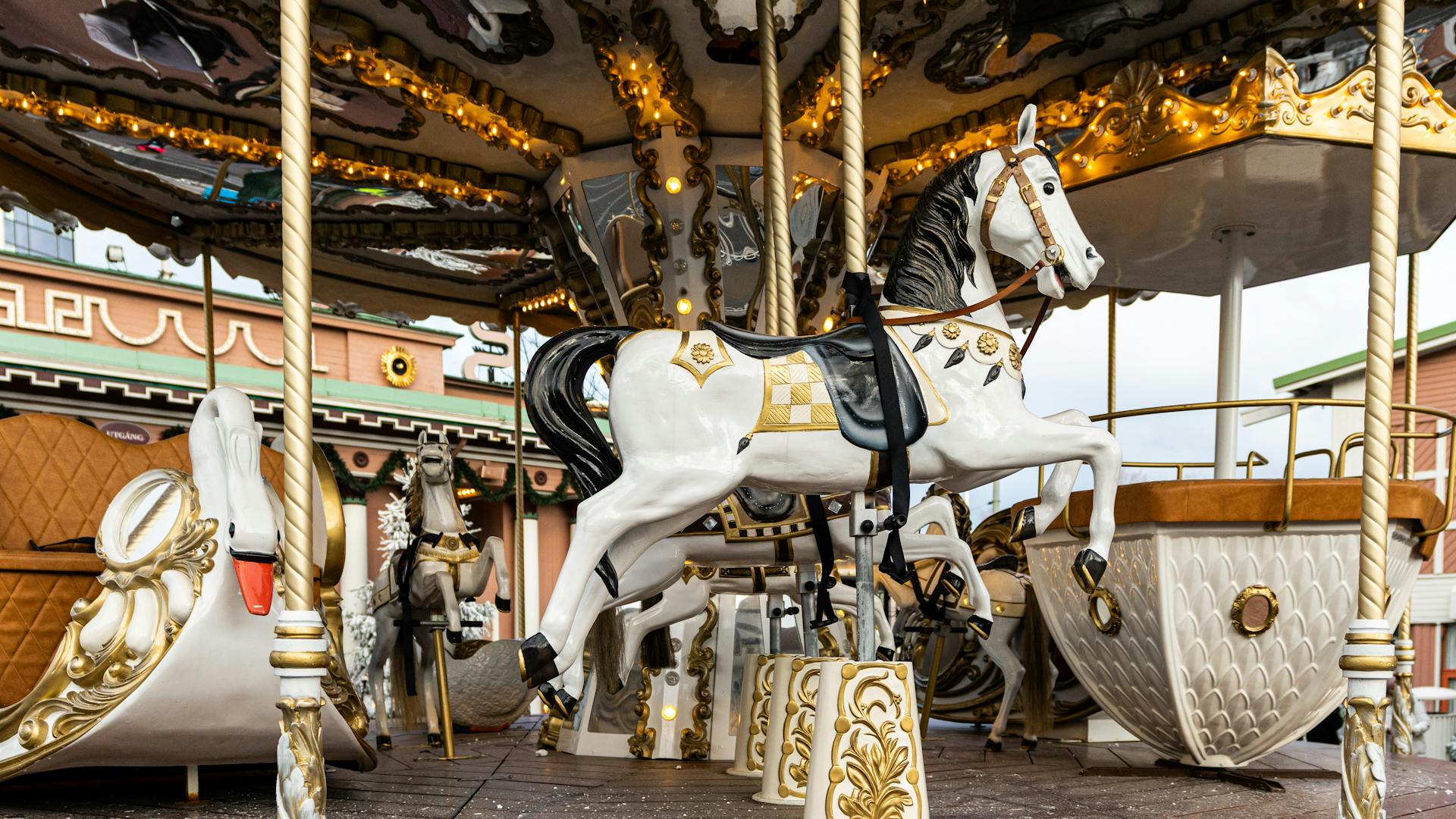
Creating a carousel with Tailwind CSS and React can be a bit tricky, but don't worry, we've got you covered.
To start, you'll need to install the necessary packages, including Tailwind CSS and the carousel component.
In the example, we used the `@tailwindcss/forms` plugin to enable Tailwind's form styling, which is required for the carousel component to work properly.
With the packages installed, you can now create your carousel component using the `Carousel` component from the `react-responsive-carousel` library.
Building the Carousel
The goal is to create a carousel using React class components. This involves defining the key components and their interactions.
To start, you'll need to create a fixed-width container that houses several child items, mainly images with overlays. The child items need to be arranged horizontally with an equal gap between them.
The carousel will hide any child items that overflow the container's bounds, yet make them scrollable to bring them into view along the horizontal axis.
Suggestion: Tailwind Css Social Media Components Free
Here's a simple example of what we're building: a carousel with images inside a clickable link, then a heading level 3 that will be displayed on hover.
The demo can be viewed here: https://codesandbox.io/s/react-multi-item-carousel-uvmchp.
The key point in the demo is the carousel.jsx component that we'll see in a minute.
Here are the main goals we want to achieve:
- Create a carousel using React class components.
- Style the carousel using Tailwind CSS.
- Implement the carousel auto-play functionality.
- Implement the swipe functionality in mobile devices.
Carousel Component
To create a carousel component, you'll first need to import the CarouselData component to access the images that will be displayed. This is done by importing the Swipe package to add swipe functionality on mobile devices.
The state is defined with an active state set to 0, which will be modified by a function to increase or decrease it accordingly. This determines which slide to display.
The container div is given a class of mt-8, which adds a margin of 2rem to move the carousel away from the top margin.
A different take: Tailwind Css Margin
The Multi-Item Component
The Multi-Item Component is where things get really interesting. It's built using the carousel.jsx component, which imports various hooks from React, including useState, useRef, and useEffect.
The component uses data from a JSON file, which can be replaced with data from your own JSON data, API, or database. This data is crucial for making the carousel work.
Each button in the carousel has a disabled property calculated using the isDisabled() function, which we'll cover later in the article. This function is essential for ensuring the carousel behaves as expected.
The buttons also have a lot of Tailwind classes applied to them, which control their appearance and behavior. These classes are used to style the buttons and make them interactive.
To make the carousel accessible to screen readers, a simple span element with the sr-only class is included. This element provides a text description of the button, making it easier for visually impaired users to understand.
The carousel component uses a classic React pattern of looping through an array with the .map() function, which generates repeated JSX for each iteration. This pattern is useful for creating dynamic content that changes based on the data.
For each resource item, the carousel produces specific JSX, including an image, a title, and a description.
For more insights, see: Tailwindcss Buttons
The JSX
JSX is a syntax extension for JavaScript that allows you to write HTML-like code in your JavaScript files.
JSX is not compiled to JavaScript, but rather to React Elements, which are then rendered to the DOM. This makes it easier to write reusable UI components.
You can use JSX to create a Carousel component by defining a class that extends the React.Component class.
The Carousel component can have a render method that returns a div element containing a ul element, which in turn contains li elements for each slide.
Carousel Features
One of the key features of the Tailwind CSS carousel in React is the ability to add indicators to make navigation easier.
Using the showIndicators property allows you to add these indicators to the carousel, which can be especially helpful when there are many images to navigate through.
Adding indicators alongside the controls can make it easier to switch between available images.
You might like: How to Add Custom Css in Tailwind
Crossfade
Crossfade is a sleek and sophisticated transition effect that can elevate your presentations to the next level. You can easily add a fade transition to your slides by adding the crossfade property to the TECarousel.
Using crossfade transitions can make your content feel more polished and refined. It's a great way to add some visual interest to your slides without overwhelming your audience.
To implement crossfade, simply add the crossfade property to the TECarousel. This is a straightforward process that requires minimal effort.
You might like: Tailwindcss Transition
Default Slider
To create a default slider, you can use the data-carousel attribute and apply a unique id to the parent element. This attribute can be set to "static" or "slide" to control the carousel's behavior.
You can add as many carousel items as you want, but each item must have the data-carousel-item attribute.
Here are the possible values for the data-carousel attribute:
- data-carousel="static" to prevent the carousel sliding by default
- data-carousel="slide" to infinitely cycle through the items
To add custom effects, use the duration-* and animation classes from Tailwind CSS. Don't forget to set the hidden class by default to prevent flickering.
Indicators
Adding indicators to your carousel can greatly enhance the user experience. This can be done by using the showIndicators property.
Using indicators makes it easier to navigate between the available images, especially when you have a large number of slides.
To show the carousel indicators, you need to add the data-carousel-slide-to attribute to any number or style of indicator elements. The value of this attribute should equal the position of the slider element.
Customization
Customization is key to making your carousel truly unique. You can pass custom classes via the theme prop to achieve this.
To change the styles of the carousel elements, create an object with the classes you want to modify. For example, you can change the styles of the active indicator element by adding the `activeIndicator` class.
The `indicatorsWrapper` class allows you to set styles to the indicators wrapper element, including its position, size, and layout. You can also use the `indicator` class to set styles to the individual indicator elements, such as their size, color, and hover effects.
Here's a breakdown of some of the classes you can use for customization:
By using these classes, you can create a custom carousel that fits your design needs.
Basic Styles
Basic styles are crucial for a clean and polished look.
The main App component, app.jsx, has a simple starting style that pulls in the carousel component and wraps it in a div with Tailwind classes to fix the width on large screens.
Basic Tailwind classes are used to add nice padding around the carousel for better display purposes.
In the styles.css file, Tailwind imports are necessary for the styles to work.
The styles.css file strips off the padding and margin from the body and HTML, and sets all box-sizing to border-box.
Additional reading: Tailwind Css Classes
Classes
Customization is all about tailoring your carousel to fit your unique needs. You can do this by passing custom classes via the theme prop.
To create a custom class, simply create an object with the desired classes and pass it to the prop. For example, you can create a class called `activeIndicator` that sets styles to the active indicator element.
Some classes you can use include `activeIndicator`, `block`, `carouselWrapper`, and `indicatorsWrapper`. These classes set styles to various elements within the carousel, such as the active indicator, display, wrapper, and indicators wrapper.
Here are some specific classes you can use:
You can also use classes like `nextBtn` and `prevBtn` to set styles to the next and previous button elements. These classes include styles for the button's appearance, behavior, and interaction.
For example, the `nextBtn` class includes styles for the button's position, size, and text color. It also includes styles for the button's hover and focus states.
Sources
- https://robkendal.co.uk/blog/how-to-build-a-multi-image-carousel-in-react-and-tailwind
- https://tw-elements.com/docs/react/components/carousel/
- https://www.cloudzilla.ai/dev-education/how-to-create-a-carousel-using-react-class-components-and-tailwind-css/
- https://www.penguinui.com/components/carousel
- https://flowbite.com/docs/components/carousel/
Featured Images: pexels.com