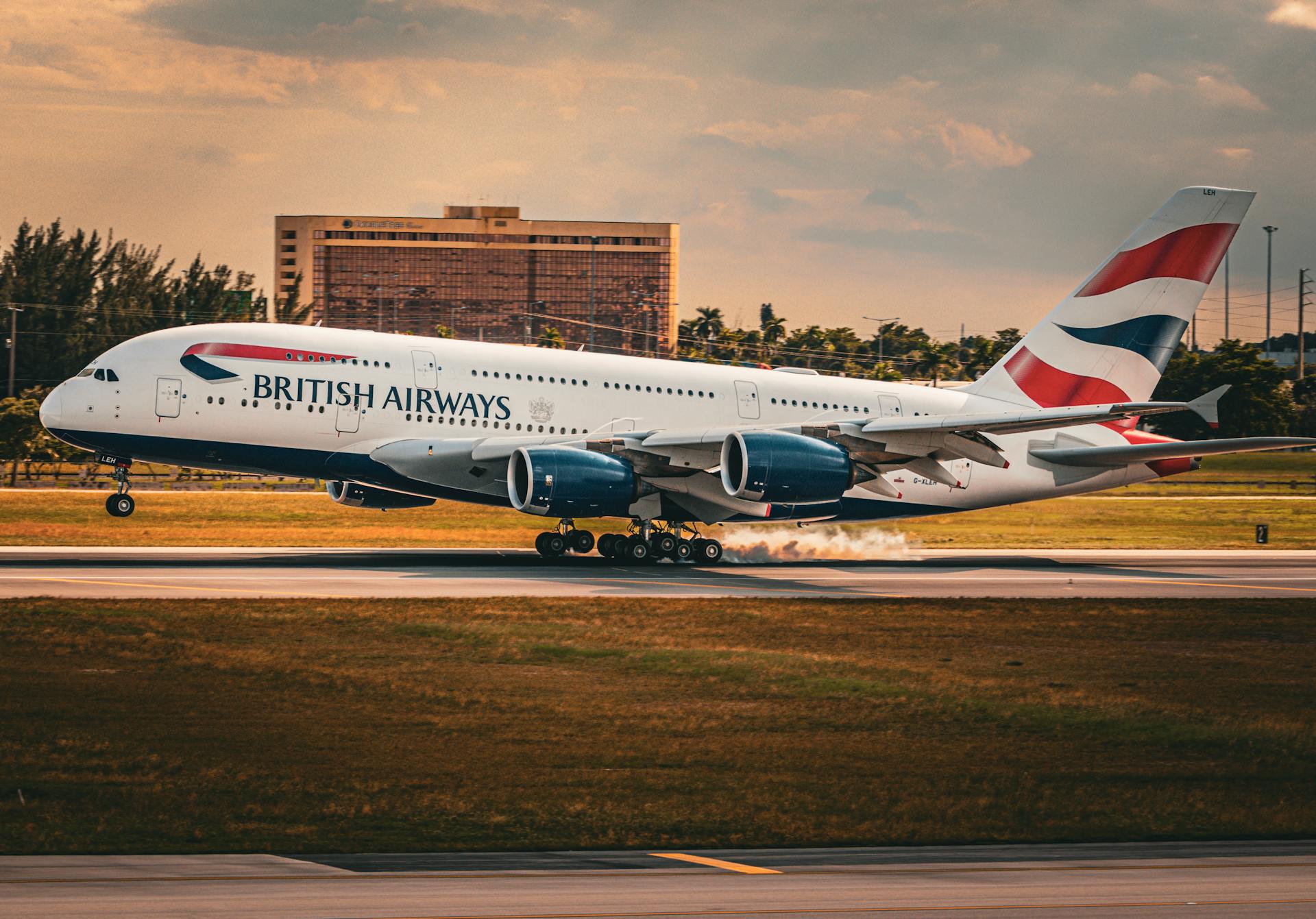
You can create a smooth and seamless user experience by mastering Tailwind CSS transitions. A transition is a way to change the appearance of an element over a specified duration.
Transitions are defined using the `transition` property, which takes a duration value and can be combined with other properties like `ease-in-out` and `duration-300`. For example, `transition: duration-300 ease-in-out` creates a transition that lasts for 300 milliseconds and follows an ease-in-out curve.
Tailwind CSS provides a range of pre-defined transition classes that can be used to simplify the process. These classes include `transition`, `duration-300`, `ease-in-out`, and many more.
You might enjoy: Tailwind Css Class
Transition Basics
To transition a conditionally rendered element, you simply wrap it in the Transition component and use the show prop to indicate whether it's open or closed. This is the basic example of how to get started with transitions in Tailwind CSS.
You can use native CSS transition styles to apply an animation by specifying the element's closed styles by targeting the data-closed attribute that the Transition component exposes. This attribute is used as the starting point when transitioning in as well as the ending point when transitioning out.
For a smoother animation, you can use Tailwind's transition utilities, which provide a set of utility classes to handle transitions in your UI elements.
Discover more: Why Use Tailwind Css
With Ease
You can specify the timing function of a transition with the ease-{type} class, where {type} can be ease-linear, ease-in, ease-out, or ease-in-out. This will give a fluid visual effect.
The ease-in-out timing function combines the ease-in and ease-out functions, making it a great choice for smooth transitions.
Ease functions can be used to create a variety of effects, from slow and gradual to fast and sudden.
Here are some common ease functions you can use in your transitions:
- ease-linear
- ease-in
- ease-out
- ease-in-out
Remember to use these ease functions in conjunction with the transition utility classes to create smooth and engaging transitions in your UI.
Understanding JIT Engine
Tailwind's JIT engine is a game-changer for developers. It loads only the CSS that's needed in the app, eliminating the need to load unnecessary styles.
Browsers start to behave funny when a website's CSS becomes too large, around 10 MB in size. This can cause performance drawbacks, especially when using devtools in development.
Tailwind's JIT engine helps to mitigate this issue by only loading the necessary CSS. With a file size of up to 3 MB after installation, it's a more efficient option than the standard Tailwind configuration.
Suggestion: Tailwind Css Font Size
Built-in Animations
Tailwind CSS comes with some amazing built-in animations that can be used to add a touch of magic to your UI elements.
These animations are perfect for loading indicators, such as the ones on a button in forms, and can be added with utility classes prefixed by the animate keyword.
The animate-spin utility class, for example, adds a linear and infinite spin animation to your HTML elements, making it ideal for creating loading indicators.
Using animation in such cases helps the user understand that their action has been acknowledged and the appropriate response is being triggered.
Animations can also be used just for aesthetics, making your UI more visually appealing and engaging.
Tailwind CSS provides four basic animations: spin, pulse, ping, and bounce, which can be used to create a variety of effects.
The spin animation is especially useful for creating loading indicators, and can be added to any HTML element using the animate-spin utility class.
Intriguing read: Css Text Animation Left to Right
By using built-in animations, you can create a more engaging and interactive user experience without having to write a single line of custom code.
The official Tailwind CSS documentation is a great resource to check out the other built-in animations in action and learn more about how to use them effectively.
Custom Animations
Creating custom animations in Tailwind CSS is a breeze. You can define keyframes of your animation and extend the theme configuration to create a new animation.
Tailwind CSS allows you to use arbitrary values for one-off custom animations, which is perfect for scenarios where you only need an animation once in your entire app. To use arbitrary values, wrap the animation properties, separated by underscores, inside a pair of square brackets after the animate keyword in the markup.
You can also add @keyframes to a Tailwind CSS config file by defining a keyframes rule for the animation, which can have multiple different styles at different stages of the animation. This is done by creating an empty keyframes object inside theme.extend in the tailwind.config.js file and adding your new animation and its behavior.
By extending the theme in Tailwind CSS, you can customize the animation duration, delay, iterations, timing function, and more. This is achieved by adding the keyframes rule to the theme object and then adding the animation to theme.extend.animation.
Related reading: Css Animations for Text
Creating Custom Animations
Creating custom animations in Tailwind CSS is a breeze. You don't need to create a new stylesheet or link it to your markup just to add a new animation to your app. Instead, define the keyframes of your animation and extend the theme configuration to create a new animation.
To add custom animations, you'll need to define a keyframes rule for the animation, which is done using the @keyframes CSS at-rule. This rule allows you to define the value of CSS properties of an element during the initial, intermediate, and final waypoints of the animation.
You can extend the theme configuration in your tailwind.config.js file by creating an empty keyframes object inside theme.extend. Inside this keyframes object, you can add your new animation and define its behavior.
For example, to create a custom waving hand animation, you can add the following code to your tailwind.config.js file: `theme.extend.keyframes.wave = { /* animation code here */ };`. This will define the keyframes for your animation.
Check this out: Tailwind Css Examples Code
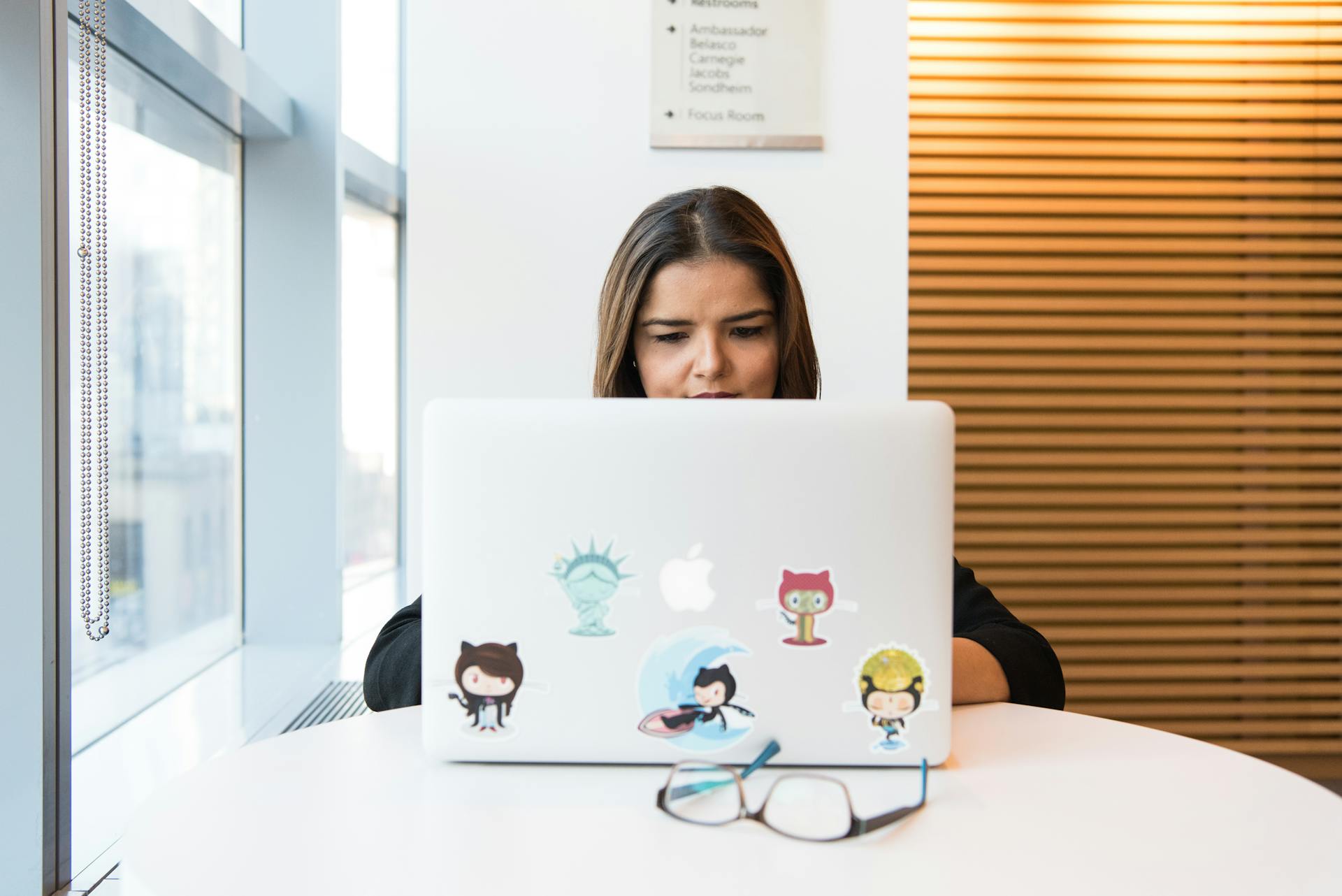
Once you've defined the keyframes, you can create a new animation by adding the animation to theme.extend.animation. For example, to create an animation that transitions for two seconds linearly in each cycle and keeps animating infinitely, you can use the following code: `theme.extend.animation.wave = { animation: 'wave 2s linear infinite' };`.
With these steps, you can create custom animations in Tailwind CSS and use them in your app.
Curious to learn more? Check out: Loading Animation Tailwind Css
Gradient Effect
Gradient effects can be a game-changer for website visuals.
To create a gradient hover effect, you can use a simple trick: increase the size of the background image and then change its position. This is like zooming in on an image and focusing on different aspects.
We can animate the background-position property to achieve this effect, which is currently not possible with the CSS transition property.
Adding a size-200 utility will make the gradient cover 200% of the div's width and height.
A different take: Instagram Linear Gradient Tailwind Css
To create a gradient transition from red to white, set the start of the gradient to red with from-red-500, the middle to black with via-black, and the end to white with to-white.
On hover, change the background-position to the bottom right corner using the bg-right-bottom class.
The direction of the gradient can be adjusted by setting the start position to the bottom right edge using the bg-gradient-to-br class.
Take a look at this: Gradient Border Tailwind Css
Different Enter/Leave
Different Enter/Leave transitions can be achieved using the data-enter and data-leave attributes.
These attributes allow you to specify different transition styles for entering and leaving, giving you more control over the animation.
You can combine the data-enter and data-closed attributes to specify the starting point of the enter transition.
The data-enter and data-leave attributes can also be used to specify different enter and leave durations.
This means you can create animations that are tailored to your specific needs and design goals.
Check this out: Gradient Text Css That Can Transition
Component API
Custom Animations often require a bit of setup to get right. This is where the Component API comes in – it's a powerful tool for creating custom animations.
Consider reading: How to Add Custom Css in Tailwind
The as prop determines what the transition should render as, and it defaults to Fragment. This means you can choose whether it's a string or a component.
You can control the visibility of the children with the show prop, which is a boolean value. If you set it to true, the children will be shown, and if you set it to false, they'll be hidden.
The appear prop is used to determine whether the transition should run on initial mount. By default, it's set to false, which means the transition won't run initially.
Here's a quick rundown of the props you can use with the Component API:
You can also use callbacks to customize the transition. For example, you can use the beforeEnter and afterEnter callbacks to add custom animations before and after the transition.
Sources
- https://tw-elements.com/docs/standard/content-styles/animations/
- https://blog.logrocket.com/creating-custom-animations-tailwind-css/
- https://medium.com/flat-pack-tech/css-transitions-with-tailwindcss-and-react-js-on-the-ikea-self-help-kiosks-12bd021a7287
- https://headlessui.com/react/transition
- https://birdeatsbug.com/blog/creating-hover-effects-with-tailwind-css
Featured Images: pexels.com