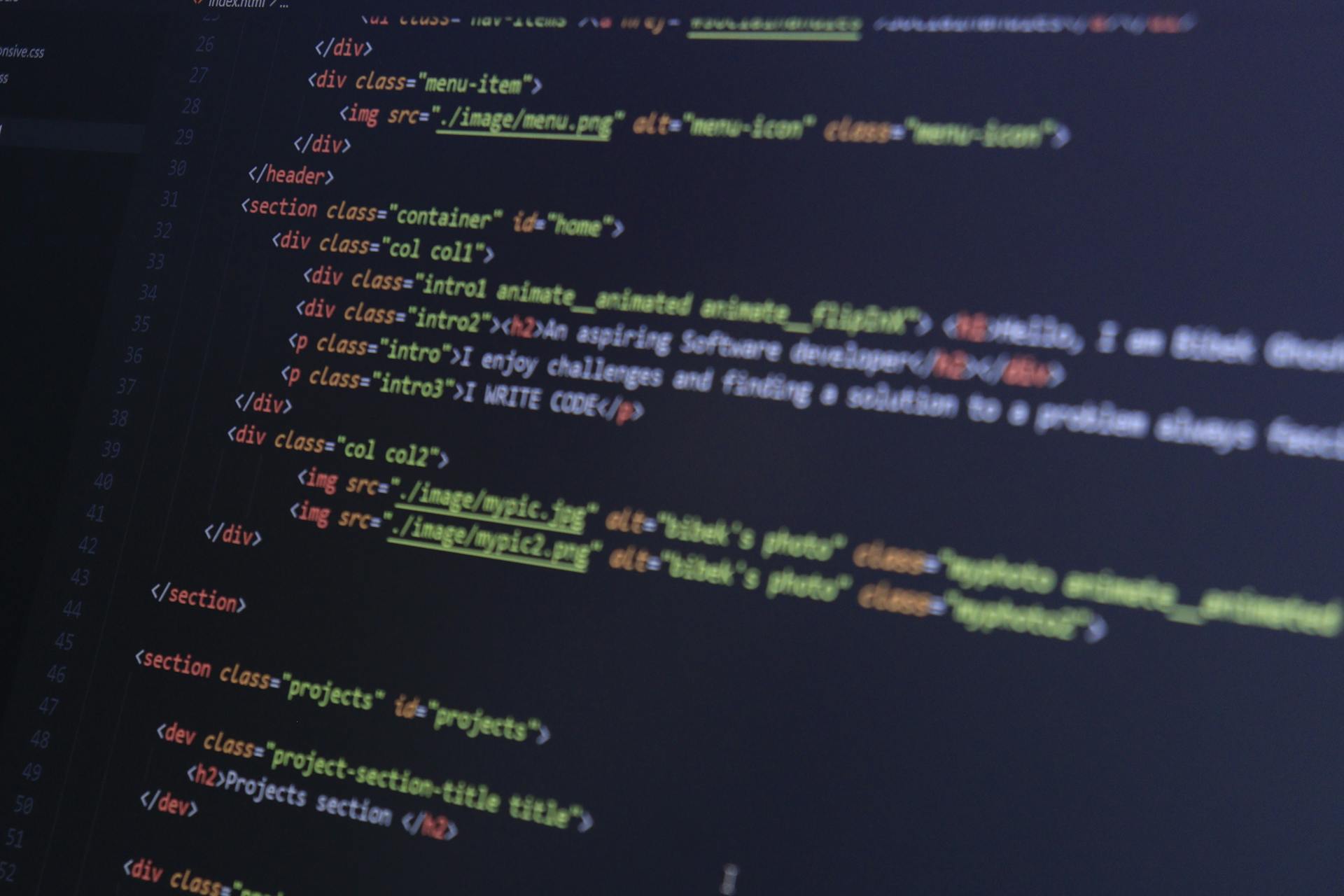
As a web developer, you know how crucial it is to be prepared for those tough interview questions. A good understanding of web programming concepts is essential to ace a web programming interview.
Interviewers often ask behavioral questions to assess your problem-solving skills and experience. Be prepared to answer questions like "Can you explain a time when you had to debug a complex issue?" or "Tell me about a project you worked on and your role in it."
In a web programming interview, you'll likely be asked technical questions that test your knowledge of languages, frameworks, and technologies. Make sure you're familiar with the basics of HTML, CSS, and JavaScript, as well as popular frameworks like React and Angular.
To give you an edge, we've compiled a comprehensive guide to web programming interview questions, covering topics from data structures to front-end development.
On a similar theme: Web Programming Frameworks
Web Development Fundamentals
Web development is all about building websites and applications that users can interact with. It involves a combination of programming languages, frameworks, and tools to create a seamless online experience.
The client-side of web development is primarily handled by JavaScript, which is executed by the user's web browser. The server-side, on the other hand, is typically managed by languages like Python and Ruby.
Understanding the basics of HTML, CSS, and JavaScript is essential for any web developer, as they form the foundation of web development.
Worth a look: Best Programming Language for Web Development
What Is SOAP and REST?
SOAP and REST are two fundamental concepts in web development, and understanding their differences is crucial for building robust and efficient web applications.
SOAP is a web development protocol that works with XML, whereas REST is an architectural platform that can work with XML, HTML, and plain text.
One key difference between SOAP and REST is that SOAP is a protocol, whereas REST is a platform. This distinction is important to grasp when designing web services.
SOAP cannot use REST, whereas REST can make use of SOAP. This means that if you're building a web service that uses SOAP, you can't also use REST, but if you're building a web service that uses REST, you can use SOAP as well.
Here's a quick summary of the differences between SOAP and REST:
By understanding these fundamental differences, you'll be better equipped to design and build web applications that meet the needs of your users.
What Is an Application?
So, what is an application? A web application, to be specific, is a software that runs on the web and provides a service or solution to users. It's like a website, but with more interactive features.
A web application framework, such as a WAF, is a pre-built set of libraries that help developers create the website. It has common codes for different websites, like authentication, which the developer can use and focus on the unique part of the website.
An application usually has a front-end, back-end, and database. The front-end is what users interact with, the back-end handles the logic and data, and the database stores the data.
If this caught your attention, see: Azure Data Engineering Interview Questions
What Are For?
WebSockets are used for real-time, event-driven communication between a client and server, allowing bi-directional communication where both can send data to each other at any period.
Bi-directional communication is a key feature of WebSockets, enabling a seamless exchange of information between the client and server.
If this caught your attention, see: C Programming Web Server
Advantages of Using a CDN in jQuery
Using a Content Delivery Network (CDN) in jQuery can be a game-changer for web developers. CDNs are widely used in jQuery as they offer an ample number of advantages for users.
CDNs cause a significant reduction in the load for the server. This means that your website's server doesn't have to work as hard to deliver content, which can lead to faster page loads and improved user experience.
They provide large amounts of savings in the bandwidth. This is especially important for websites with high traffic, as it can help reduce the strain on your server and save you money on bandwidth costs.
jQuery frameworks load faster due to optimizations. This is because CDNs can optimize the way your jQuery framework is delivered, resulting in faster load times and improved performance.
CDNs have a caching ability that adds to quicker load times. This means that your website's content is stored in a cache, which can be quickly retrieved by users, reducing the time it takes for your website to load.
Here are some key benefits of using a CDN in jQuery:
- Reduced server load
- Large savings in bandwidth
- Faster jQuery framework load times
- Improved load times through caching
Significance of Git
Git is a crucial tool in web development, allowing multiple developers to work together without overwriting each other's changes.
Git tracks all changes made to code, giving you a clear history of your project's development.
This feature enables distributed teams to develop software efficiently by versioning source code and facilitating parallel workstreams.
By managing code history and merging updated code from different contributors, Git coordinates collaboration and prevents conflicts.
This makes it easier for developers to work together on large projects, knowing that their changes won't be lost or overwritten.
Take a look at this: Web Development Software
Web Performance Optimization
Web performance optimization is crucial for a smooth user experience. It involves making changes to your website to improve its loading speed.
Reducing page loading time is a key aspect of web performance optimization. One way to do this is by reducing image size. This can be achieved by compressing images without compromising their quality.
Removal of unnecessary widgets is another method to reduce page loading time. This includes removing any unused code or plugins that are not essential to the website's functionality.
See what others are reading: Free Blog Website Templates Html
HTTP compression is also an effective way to speed up page loading. It reduces the size of web pages and images by compressing them, making them load faster.
Minimal redirection and caching can also help in reducing page loading time. This involves minimizing the number of redirects a user has to go through to access a page and caching frequently accessed pages.
Here are some methods that can be implemented to reduce page loading time:
- Reduction in image size
- Removal of unnecessary widgets
- HTTP compression
- Reduction in lookups
- Minimal redirection and caching
CSS Advanced Topics
Child selectors in CSS are powerful tools for targeting specific elements that are direct children of another element, denoted by the “greater than” symbol (>). They allow developers to apply styles or perform actions exclusively on immediate child elements.
Using child selectors, developers can create refined and granular styles, controlling the appearance of specific child elements within a parent container, and achieving more precise CSS targeting.
CSS preprocessors like SASS and LESS extend regular CSS with extra features, adding variables for reusable values, nesting to organize code, and functions for complex operations. This makes writing CSS more efficient, reusable, and maintainable.
Explore further: Css Selector Precenednce Cheat Sheet
Pseudo-Classes in CSS
Pseudo-classes in CSS are a powerful technique to change the style of an element when it changes its state.
Pseudo-classes are used to style an element based on its state, such as when the mouse is over it or when it's out of focus.
You can use pseudo-classes for out-of-focus animations, which can be particularly useful for creating a visually appealing effect.
For example, you can use pseudo-classes to provide styles for external links, making them stand out from internal links.
Pseudo-classes are also useful for creating hover effects, where the style of an element changes when the mouse moves over it.
Here are some examples of when you might use pseudo-classes:
- For the style change when the mouse moves over the element
- For out-of-focus animations
- For providing styles for external links
By using pseudo-classes, you can add a new level of interactivity to your website, making it more engaging and user-friendly.
What Are Media Queries in CSS3?
Media queries in CSS3 are a powerful tool for creating responsive designs. They allow you to define styles that adapt to different screen sizes and orientations.
Media queries are used to adjust the height and width of your website, making sure it looks great on any device. You can also use them to adjust the viewport, which is the visible area of your website on a user's screen.
The entities that media queries can adjust include height, width, viewport, resolution, and orientation. This means you can tailor your website's layout to suit different devices and screen sizes.
For example, you might use media queries to change the layout of your website when it's viewed on a mobile device versus a desktop computer. This can be especially useful for websites that need to display complex information or have a lot of interactive elements.
Here are some of the entities that media queries can adjust:
- Height
- Width
- Viewport
- Resolution
- Orientation
Changing Styles or Classes in Elements
Changing Styles or Classes in Elements can be a bit tricky, but with the right tools, it's a breeze. CSS selectors are used to find and select HTML elements based on factors like name, ID, or attribute, making it easy to style them.
To change styles or classes in elements, JavaScript comes to the rescue. You can use the following syntax:
Pseudo-classes are also useful when changing styles, as they can be used to change the style of an element when it changes its state, such as when the mouse moves over it. With pseudo-classes, you can create animations and styles for external links, out-of-focus elements, and more.
CSS Best Practices
When working with CSS, it's essential to follow best practices to ensure your code is maintainable, efficient, and scalable. This includes using a consistent naming convention, such as BEM or OOCSS, to organize your classes and avoid CSS specificity issues.
Use the box model correctly to avoid layout problems. This means using the margin, border, and padding properties together to control the size and position of elements.
Avoid using inline styles and instead opt for CSS classes or IDs to separate presentation from structure. This will make it easier to update your styles without modifying your HTML.
Benefits of Bem Methodology in CSS Coding
The BEM methodology is a game-changer for CSS coding. It helps organize code by naming classes after the HTML components they style, reflecting the relationship between structure and appearance.
BEM improves CSS readability by making class names more meaningful. This is a huge plus for developers who work on complex projects.
BEM's structured approach also makes maintenance and scalability easier. By organizing code in a logical way, developers can quickly identify and fix issues.
With BEM, class names become a clear representation of the HTML components they style. This clarity is especially helpful when working on large projects with multiple developers.
BEM's benefits are numerous, but its improved maintenance and scalability are arguably the most significant advantages. By making code more organized and logical, BEM helps developers work more efficiently.
JavaScript Fundamentals
JavaScript supports the following data types: Boolean, Number, Object, Undefined, Null, String, and Function.
In JavaScript, a Boolean data type can only have one of two values: true or false. This is useful for making decisions in your code.
Here's a list of the basic data types in JavaScript, which is helpful to keep handy when coding:
- Boolean
- Number
- Object
- Undefined
- Null
- String
- Function
localStorage vs sessionStorage
Local storage and session storage are two essential concepts in JavaScript that help you manage data on the client-side.
localStorage is a type of storage that doesn't have an expiry date for the stored data, which means it remains even after the user closes the window.
One of the main differences between localStorage and sessionStorage is how long the data is stored. Here's a quick comparison:
The choice between localStorage and sessionStorage depends on your specific use case. If you need data to persist even after the user closes the window, localStorage might be the better choice.
Type Conversion Handling
JavaScript is a weakly typed language, which means it automatically converts data types as needed. This is often referred to as "duck typing" because if it walks like a duck and talks like a duck, it's a duck.
JavaScript supports automatic type conversion, which eliminates errors and warnings associated with data types. This makes it easy to pass functions as arguments into other functions.
You can easily pass a function as an argument into another function in JavaScript because of its weak typing. This is a powerful feature that simplifies coding.
Automatic type conversion ensures that values are converted to the required data type, making programming easier and more efficient.
Worth a look: Azure Data Factory Interview Questions
What is Variable Scope?
Variable scope in JavaScript is the accessibility of functions and underlying variables in the running environment.
There are two scopes supported in JavaScript: local scope and global scope. Local scope refers to values and functions declared inside the same function, which can only be accessed within that function and not outside it.
In local scope, variables and functions are contained within a specific function, much like how a private room is only accessible from within that room.
The following is an example of local scope in action: a variable declared inside a function is only accessible within that function.
Here's a quick rundown of the two scopes:
Global scope, on the other hand, allows variables to be accessed from anywhere in the application.
Your Experience
JavaScript is a versatile language that can be used for both front-end and back-end development.
You can start building dynamic web pages with JavaScript from scratch, using basic syntax like variables, data types, and operators.
A unique perspective: Web Dev Javascript
One of the most significant benefits of JavaScript is its ability to handle events and create interactive user interfaces.
This is achieved through the use of event listeners and callback functions, which we covered in the "Events and Callbacks" section.
With JavaScript, you can also create functions that can be reused throughout your code, making it more efficient and organized.
This is a fundamental concept in JavaScript, as we discussed in the "Functions" section.
In addition, JavaScript allows you to work with arrays and objects, which are essential data structures in programming.
We saw how to use arrays and objects in the "Arrays and Objects" section.
As you gain more experience with JavaScript, you'll appreciate its ability to handle asynchronous programming.
This is crucial for handling multiple tasks simultaneously, which we explored in the "Asynchronous Programming" section.
Here's an interesting read: Azure Functions Interview Questions
Technical Skills
JavaScript is a versatile language that's widely used in web development. It's used for both front-end and back-end development, making it a valuable skill to have.
You can use JavaScript to create interactive web pages, web applications, and mobile applications. It's also used for server-side programming with technologies like Node.js.
To become proficient in JavaScript, you need to have a good understanding of its syntax and semantics. This includes variables, data types, operators, control structures, functions, and object-oriented programming concepts.
Here are some key JavaScript concepts to focus on:
- Variables: JavaScript has several types of variables, including var, let, and const.
- Data types: JavaScript has several built-in data types, including number, string, boolean, array, and object.
- Operators: JavaScript has several types of operators, including arithmetic, comparison, logical, assignment, and bitwise operators.
- Control structures: JavaScript has several types of control structures, including if/else statements, switch statements, for loops, and while loops.
- Functions: JavaScript functions are reusable blocks of code that can be called multiple times.
- Object-oriented programming: JavaScript supports object-oriented programming concepts like encapsulation, inheritance, and polymorphism.
To improve your JavaScript skills, practice coding exercises, and work on real-world projects. You can also use online resources like CodePen, JSFiddle, and CodeSandbox to experiment with JavaScript code.
In a technical interview, you may be asked questions like "What are the advantages of HTTP 2.0 over HTTP 1.1?" or "As a Web Developer, what steps do you take to optimize your site's loading time?" Be prepared to answer these questions and provide specific examples from your experience.
Here are some common JavaScript interview questions to prepare for:
- What specific coding languages do you work with?
- What web development tools do you use?
- How do you organize your JavaScript code?
- How do you approach web accessibility and make sure your websites and applications are accessible to users?
Remember, practice is key to improving your JavaScript skills. Keep coding, and you'll become proficient in no time!
JavaScript Libraries
JavaScript Libraries are a crucial part of web development, and knowing which ones to use can make a big difference in your coding career.
Lodash is a popular JavaScript library that provides a wide range of utility functions for tasks such as data manipulation, array filtering, and object transformation. It's often used in conjunction with other libraries like React and Angular.
Some common use cases for Lodash include avoiding errors with its built-in functions like `_.isEmpty()` and `_.isNil()`, and simplifying code with its array methods like `_.map()` and `_.filter()`.
Namespace Definition
A namespace in JavaScript is a global object that holds methods, properties, and other objects, making it easier to use and reuse code.
This modularity provided by namespaces helps avoid naming conflicts, which can be a real pain to deal with, especially when working on large projects.
Namespaces are essentially a way to organize your code, keeping related functions and variables grouped together for better maintainability.
By using namespaces, you can create a simple yet effective way to structure your code, making it easier to read and understand for both you and your team.
What Is the Each() Function in jQuery?
The each() function in jQuery is a game-changer for simplifying the process of applying operations or modifications to multiple elements.
It's used to iterate over a collection of elements and perform a specified action for each element, making it a vital tool for web developers.
This function is incredibly efficient, allowing you to accomplish complex tasks in a concise manner.
By using each(), you can apply the same action to multiple elements, saving you time and effort in the long run.
Preventing Event Default Action with jQuery
If a jQuery event handler returns false, it prevents the default behavior of the event and stops the event from propagating further.
This can be used to cancel actions like form submission or link navigation when certain conditions are not met.
Returning false from a jQuery event handler is a powerful tool for controlling how your JavaScript code responds to user interactions.
It's a simple yet effective way to prevent default actions and keep your application running smoothly.
By using this technique, you can ensure that your code behaves exactly as intended, even in unexpected situations.
Broaden your view: Themeroller Jquery Ui
What Are CDN Types in jQuery?
When working with JavaScript libraries like jQuery, it's essential to understand the different types of CDNs (Content Delivery Networks) that are supported.
There are two main types of CDNs in jQuery: Microsoft and Google.
Microsoft's CDN is used to load jQuery from the jQuery AJAX CDN. This is a reliable option for loading jQuery, especially if you're already using Microsoft's services.
Google's CDN, on the other hand, is used to load jQuery from the Google Libraries API. This option is great if you want to take advantage of Google's caching and content delivery capabilities.
Here's a quick rundown of the supported CDNs in jQuery:
- Microsoft: Used to load from jQuery AJAX CDN
- Google: Used to load jQuery from the Google Libraries API
PHP
PHP is a versatile language that's perfect for web development. PHP is used in popular content management systems like WordPress, Joomla, and Drupal.
These platforms are built using PHP and are widely used for building websites and web applications. PHP's flexibility and ease of use make it a popular choice among developers.
Here are some popular PHP frameworks and libraries:
- CodeIgniter
- Phalcon
- Magento
These frameworks and libraries can help you build robust and scalable web applications using PHP. They provide pre-built functionality and tools to make development faster and more efficient.
Frequently Asked Questions
How to pass a web developer interview?
To pass a web developer interview, clearly articulate your problem-solving process and technical skills through concise explanations of how you'd tackle various web development challenges. Prepare to demonstrate your expertise in a logical and methodical way.
What are three types of web development?
There are three main types of web development roles: front-end, back-end, and full stack. Each role focuses on different aspects of building and maintaining a website.
Sources
- https://brainstation.io/career-guides/web-developer-interview-questions
- https://intellipaat.com/blog/interview-question/web-developer-interview-questions/
- https://www.javatpoint.com/web-developer-interview-questions
- https://arc.dev/talent-blog/web-developer-interview-questions/
- https://careers.webdew.com/blogs/web-development-interview-questions/
Featured Images: pexels.com