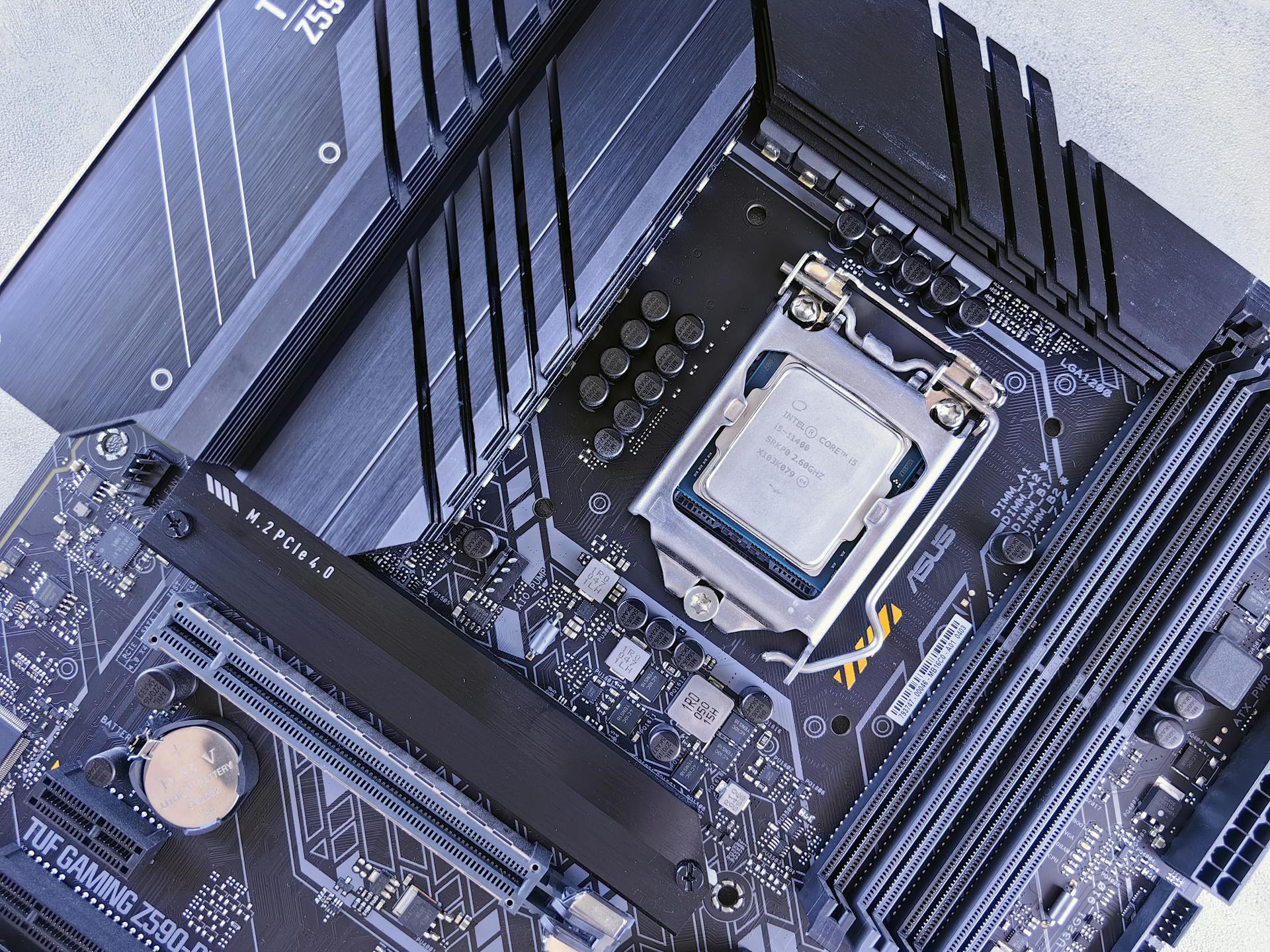
In programming, a stack is a fundamental data structure that helps us manage and organize data in a specific order. It's like a vertical pile of plates where you add and remove plates from the top.
Think of it like a call stack, where functions are added and removed in a Last-In-First-Out (LIFO) order. This is because the most recently added function is the first one to be executed when the stack is popped.
A stack is a linear data structure, meaning it only has one way to access its elements, from the top. This makes it efficient for tasks like parsing expressions and evaluating postfix notation.
You might like: Azure Data Class a Means
What is Stack
A stack is a fundamental data structure that follows the Last In, First Out (LIFO) principle, meaning the last item added to the stack is the first one to be removed.
It's a linear data structure, meaning items are stored in a linear sequence, and it's often used in programming to implement recursive algorithms.
A stack can be thought of as a vertical pile of plates, where the top plate is the most accessible and the first to be removed.
Related reading: What Does First Copy Mean?
Types of Stacks
Stacks can be implemented in programming languages, and some languages make stack operations like push and pop available on their standard list/array types.
Perl, LISP, JavaScript, and Python are examples of languages that provide stack operations on their standard list/array types.
Some languages, such as those in the Forth family, are designed around language-defined stacks that are directly visible and manipulable by the programmer.
The C++ Standard Library container types have push_back and pop_back operations with LIFO (Last In, First Out) semantics, and the stack template class adapts existing containers to provide a restricted API with only push/pop operations.
Java's library contains a Stack class that is a specialization of Vector, and PHP has an SplStack class.
Discover more: Web Dev Stacks
MEAN vs MERN vs MEVN
Let's break down the differences between MEAN, MERN, and MEVN stacks. These three tech concepts are used to build web applications, and they all share the same back-end development tools: MongoDB, Express.js, and Node.js.
Related reading: Next Js Stack
The primary difference between them lies in the front-end development options. MERN replaces AngularJS with ReactJS, while MEVN replaces it with Vue.js.
Developer familiarity is a key factor in choosing which framework to work with. Many development teams will choose a stack based on team member experience and comfort levels.
The MEAN stack is often chosen for complex, feature-rich single page applications (SPAs) because AngularJS is well-suited for complicated web applications.
ReactJS, part of the MERN stack, is a JavaScript library that's especially useful in building fast, interactive user interfaces. It offers high performance and scalability, making it a good choice for large-scale applications.
Vue.js, part of the MEVN stack, is a progressive JavaScript framework that's simple to use and easy to integrate. It's a good choice for developers who want simple, rapid development with small- to mid-sized applications.
Here's a quick summary of the differences:
Ultimately, the choice between MEAN, MERN, and MEVN stacks comes down to the specific needs of your project and the preferences of your development team.
Other Programming Stacks
Some programming languages, like Perl, LISP, JavaScript, and Python, offer standard list/array types with push and pop operations.
Perl, LISP, JavaScript, and Python make stack operations easily accessible.
In the Forth family, including PostScript, language-defined stacks are directly visible and manipulable by programmers.
The C++ Standard Library's container types have push_back and pop_back operations with LIFO (Last In, First Out) semantics.
C++'s stack template class adapts existing containers to provide a restricted API with only push/pop operations.
PHP has an SplStack class for working with stacks.
Java's library contains a Stack class that's a specialization of Vector.
Array and Linked List Stacks
An array can be used to implement a stack by treating the first element as the bottom and the last element as the top. This approach requires keeping track of the size of the stack using a variable top that records the number of items pushed so far.
The push operation in an array-based stack adds an element and increments the top index, after checking for overflow. This is done by adding an element to the array and then incrementing the top index.
Arrays can be dynamic, allowing the stack to grow or shrink as needed. This implementation is very efficient, requiring amortized O(1) time to add or remove items from the end of the array.
A linked list can also be used to implement a stack, with a pointer to the "head" of the list keeping track of the stack's contents. A counter can be used to keep track of the size of the list.
Pushing and popping items in a linked list-based stack happens at the head of the list, making overflow impossible unless memory is exhausted. This is because the linked list can grow or shrink as needed, allowing the stack to adapt to changing requirements.
Advantages and Applications
The MEAN stack offers numerous advantages, particularly when it comes to real-time applications. It's ideal for cloud-based and single-page web applications.
MEAN applications can be used in various ways, including workflow management tools, news aggregation sites, to-do and calendar applications, and interactive forums. These use cases showcase the versatility of the MEAN stack.
The integration between the components of the stack is intuitive and straightforward, thanks to the fact that all components are based on JavaScript and JSON. Here are some examples of MEAN stack applications:
- Workflow management tools.
- News aggregation sites.
- To-do and calendar applications.
- Interactive forums.
All components of the MEAN stack are open source, allowing developers to use them freely without incurring any costs.
Advantages
The MEAN stack offers a range of advantages that make it an attractive choice for developers. Its cross-platform, write-once approach allows for flexibility and ease of use.
One of the key benefits of MEAN is its suitability for real-time applications, particularly those running natively in the cloud and single-page web applications built in Angular.js. This makes it an ideal choice for high-throughput APIs.
The integration between the components of the MEAN stack is intuitive and straightforward, thanks to the fact that all components are based on JavaScript and JSON. This simplifies the development process and reduces the risk of errors.
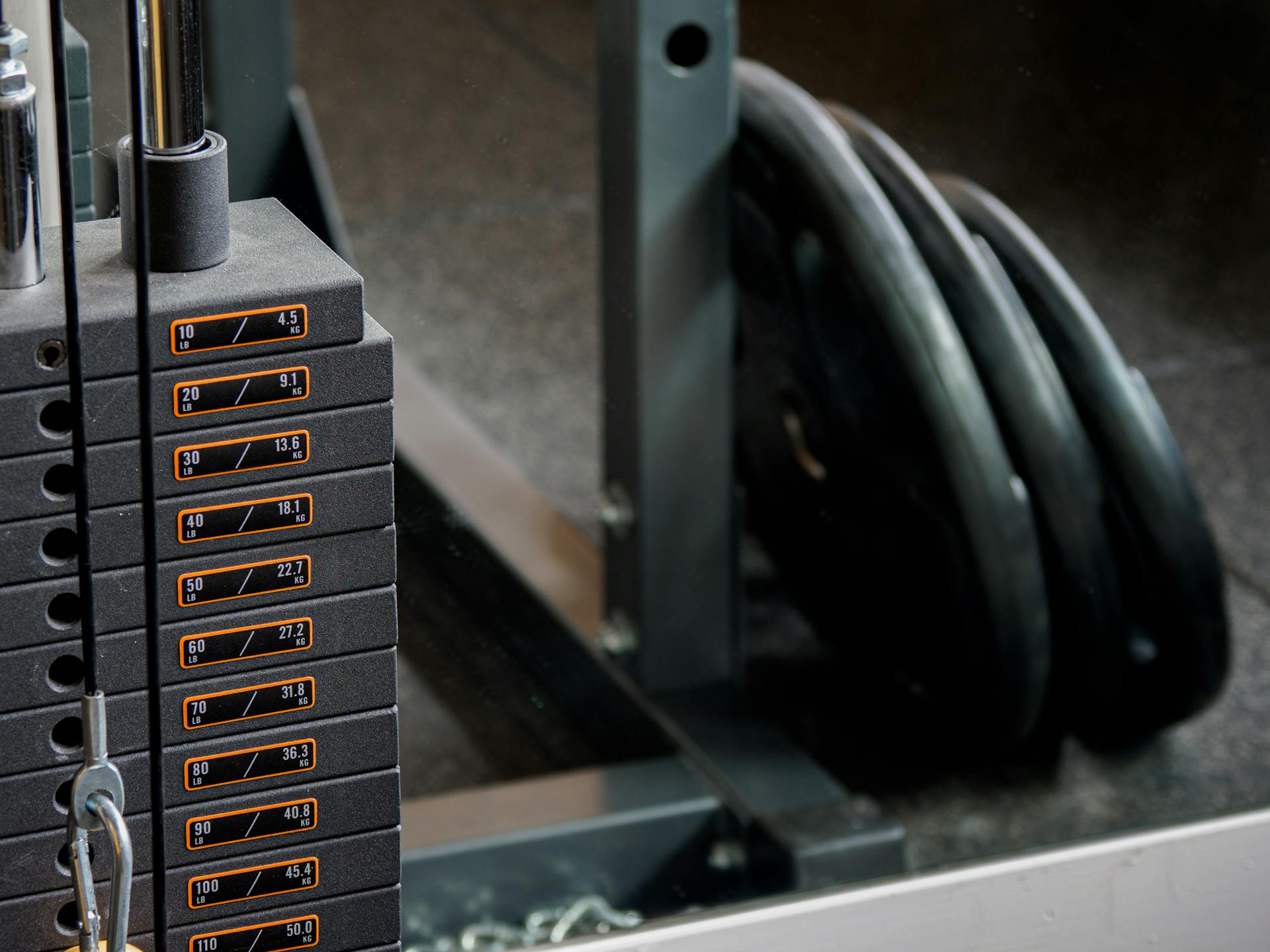
Developers can use the MEAN stack for a variety of applications, including workflow management tools, news aggregation sites, to-do and calendar applications, and interactive forums. Here are some specific examples:
- Workflow management tools.
- News aggregation sites.
- To-do and calendar applications.
- Interactive forums.
The E and A of MEAN (Express and Angular) are two of the most popular and well-supported JavaScript frameworks for back-end and front-end development, respectively. This means that developers have access to extensive documentation and community support.
Applications of Stacks
Stacks are a fundamental data structure with numerous practical applications. They're used to implement recursive algorithms in a non-recursive way, which is particularly useful for handling problems with a large number of recursive calls.
One of the most common applications of stacks is parsing expressions in a programming language. This involves evaluating the order of operations and resolving any syntax errors.
In the context of web development, stacks are used to manage the call stack, which is essential for handling user input, processing requests, and generating responses.
The stack data structure is also used in algorithmic problems involving function calls, such as evaluating the validity of parentheses in an expression.
In addition to these examples, stacks are used in evaluating postfix expressions, which is a type of notation where operators follow their operands.
Featured Images: pexels.com