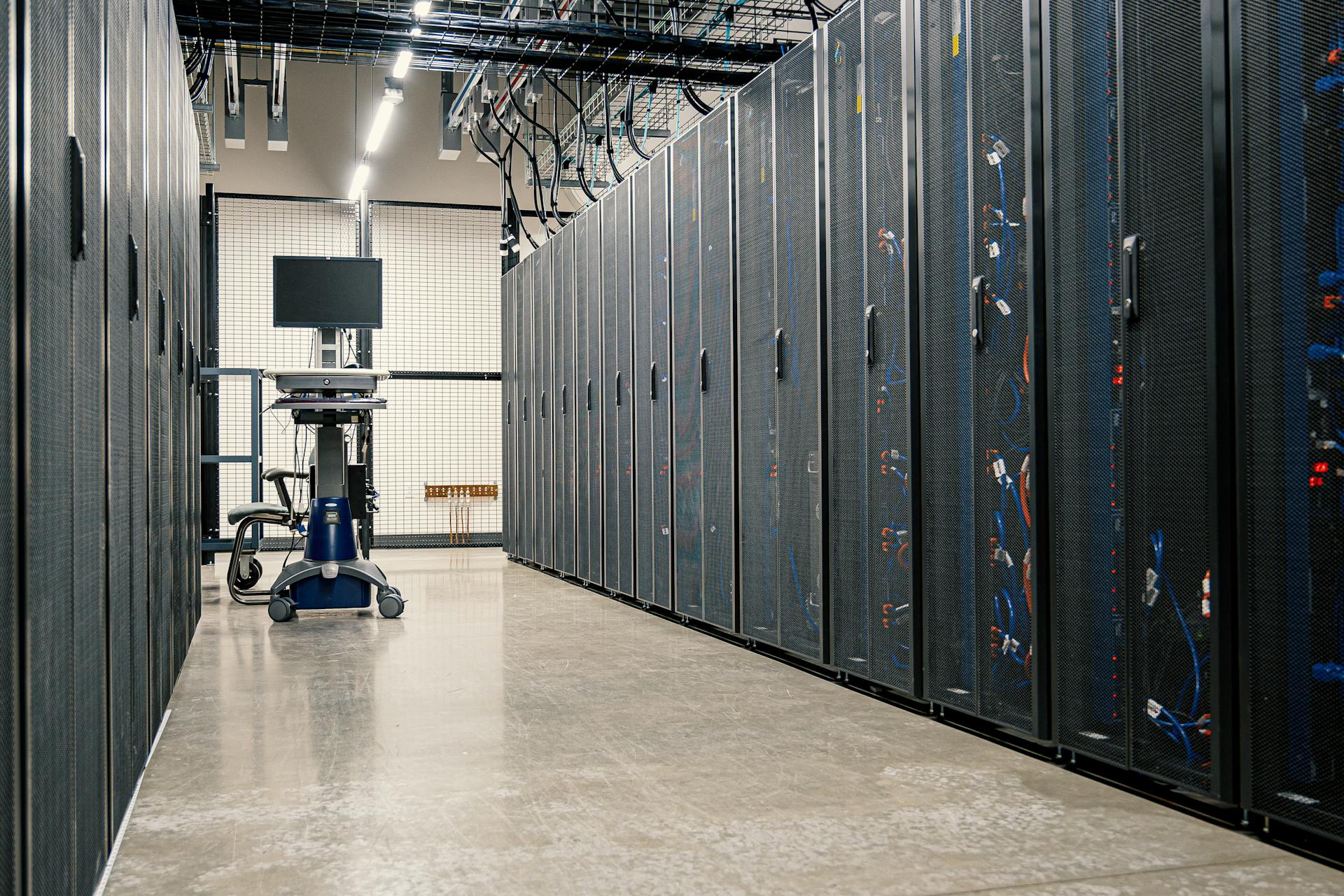
If you're looking to build a scalable and efficient serverless architecture, Azure Function Queue Trigger is a great place to start.
You can use Azure Function Queue Trigger to process messages from Azure Storage Queues, Service Bus queues, and Event Grid topics.
To get started, you'll need to create an Azure Storage Queue or Service Bus queue to store your messages.
This will serve as the trigger for your Azure Function, which will be executed whenever a new message is added to the queue.
In this tutorial, we'll walk you through the process of setting up an Azure Function Queue Trigger and provide best practices for implementing it in your applications.
You might like: Azure Logic App Blob Storage Trigger
Configuration and Setup
To set up an Azure Function Queue Trigger, you'll need to configure the options object passed to the app.storageQueue() method. This involves setting the queueName property to the name of the queue to poll and the connection property to the name of an app setting or setting collection that specifies how to connect to Azure Queues.
Expand your knowledge: Azure Linux Function App
You'll also need to configure the binding in the function.json file. This involves setting the type property to queueTrigger, the direction property to in, the name property to the name of the variable that contains the queue item payload in the function code, and the queueName property to the name of the queue to poll.
Here are the properties you can set in the function.json file:
Configuration
The configuration for Azure Queue Storage is quite straightforward. You can set properties on the options object passed to the app.storageQueue() method, such as queueName and connection.
The queueName property specifies the name of the queue to poll, while the connection property is a reference to environment configuration that specifies how the app should connect to Azure Queues.
To obtain a connection string, you can follow the steps shown at Manage storage account access keys.
You can also set binding configuration properties in the function.json file, such as type, direction, name, queueName, and connection.
You might like: Azure App Insights vs Azure Monitor
Here are the valid values for the type property:
- queueTrigger
- queue
The direction property must be set to in for queueTrigger and out for queue.
The name property specifies the name of the variable that contains the queue item payload in the function code or represents the queue in function code.
The queueName property specifies the name of the queue to poll or the name of the queue.
The connection property is a reference to environment configuration that specifies how the app should connect to Azure Queues.
Attributes
Attributes play a crucial role in configuring your Azure Functions. You can use the QueueOutputAttribute to specify the name of the queue when running in an isolated worker process.
The QueueOutputAttribute applies to an out parameter or the return value of the function, and its constructor takes the name of the queue as an argument.
There are three properties you can use with the QueueOutputAttribute: arg_name, queue_name, and connection. The arg_name property is the name of the variable that represents the queue in function code, while the queue_name property is the name of the queue itself.
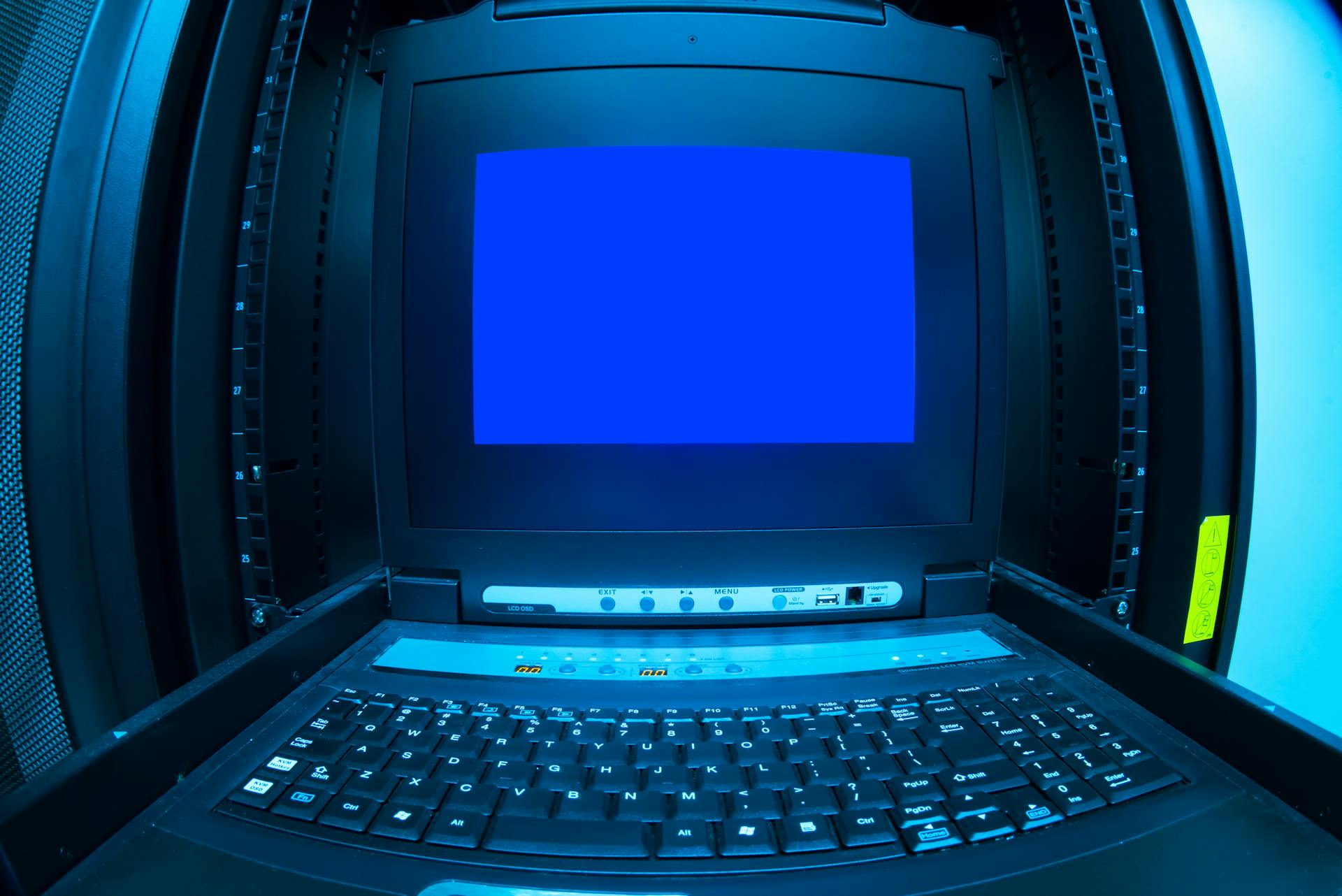
The connection property specifies how to connect to Azure Queues, and it can be an app setting or setting collection.
Here's a summary of the QueueOutputAttribute properties:
Triggering and Function
Triggering a Function App from Azure Queue Storage is a straightforward process. You can trigger a Function App from a queue in Azure by selecting the Azure Queue Storage trigger as the template when creating a new function.
To create the queue triggered function, you need to provide the name of the new trigger function, the queue name, and the storage account connection. You can either use the storage account used by the Function App or create a new one.
The function will be triggered by the queue as soon as a message is posted to it. You can access the message data by using a method parameter such as a string paramName, which is the value specified in the QueueTriggerAttribute.
Here are the types that the queue trigger can bind to:
Triggering the Function
To trigger a Function App from Azure Queue Storage, you need to configure the queue trigger in the Function App.
You can test the queue trigger by posting a message to the queue. Open the queue that has been created and click on Add message.
The function has been triggered by the queue, and as soon as the message was posted, the function has been executed and the results are printed on the console.
The queue trigger can bind to the following types: string, byte[], JSON serializable types, QueueMessage, and BinaryData.
To use these types, you need to reference Microsoft.Azure.Functions.Worker.Extensions.Storage.Queues 5.2.0 or later and the common dependencies for SDK type bindings.
The QueueTrigger annotation gives you access to the queue message that triggered the function, and you can access it by using a method parameter such as string paramName.
Here are the types that can be bound to the queue trigger:
The queue trigger implements a random exponential back-off algorithm to reduce the effect of idle-queue polling on storage transaction costs.
Concurrency
Concurrency is a powerful feature that allows multiple queue messages to be processed simultaneously. By default, the batch size is 16, and the runtime gets another batch when the number being processed gets down to 8.
This means that the maximum number of concurrent messages being processed per function on one virtual machine (VM) is 24. The batch size and threshold for getting a new batch are configurable in the host.json file.
If you want to minimize parallel execution for queue-triggered functions, you can set the batch size to 1. This setting eliminates concurrency, but only if your function app runs on a single virtual machine (VM).
The queue trigger automatically prevents a function from processing a queue message multiple times simultaneously, which is a great feature to prevent duplicate processing.
See what others are reading: Azure Devops Trigger Batch
Queue Trigger Details
A function has a single trigger and one or more bindings, with the type of binding being either input or output.
Queue Storage in Azure allows users to store multiple messages in it, with a queue able to store data up to 64 KB in size.
To trigger a function from Queue Storage, you need to post a message to the queue, which can be done by clicking on Add message in the queue.
The function has been triggered by the queue as soon as the message was posted, and the results are printed on the console.
Triggers and bindings are defined differently depending on the development language, with C# class library functions configured by decorating methods and parameters with C# attributes.
For C# class library functions, the HTTP trigger (HttpTrigger) is defined on the Run method for a function named HttpExample that returns a MultiResponse object.
Here are the possible C# runtime models for configuring triggers and bindings:
- Isolated worker model
- In-process model
In Java functions, triggers and bindings are configured by annotating specific methods and parameters, with the HTTP trigger (@HttpTrigger) defined on the run method for a function named HttpTriggerQueueOutput.
In Node.js for Functions version 4, you configure triggers and bindings using objects exported from the @azure/functions module, with the http method on the exported app object defining an HTTP trigger.
In Node.js for Functions version 3, you configure triggers and bindings in a function-specific function.json file in the same folder as your code, with the function.json file defining the HTTP trigger function that returns an HTTP response and writes to a storage queue.
Recommended read: Azure Function Change Runtime Version
Bindings and Code
For Azure Function Queue Trigger, triggers and bindings are defined differently depending on the development language. Make sure to select your language at the top of the article.
In C# class library functions, triggers and bindings are configured by decorating methods and parameters with C# attributes, where the specific attribute applied might depend on the C# runtime model: isolated worker model or in-process model.
You can find more examples of specific binding types that show you how to work with bindings in your functions in the following table:
In Python for Functions version 1, bindings code for Python depends on the Python model version, and you can find more examples in the article.
For another approach, see: Azure Azure-common Python Module
Bindings
Bindings are a crucial part of Azure Functions, allowing you to connect your code to various services and triggers.
In Azure Functions, triggers and bindings are defined differently depending on the development language. For example, in C# class library functions, triggers and bindings are configured by decorating methods and parameters with C# attributes.
A fresh viewpoint: Azure Function Trigger
You can configure triggers and bindings in Azure Functions using various methods, including annotating methods and parameters in Java functions or using objects exported from the @azure/functions module in Node.js.
Bindings can be used to connect your Azure Functions to various services, such as Azure Storage queues. For example, you can use the QueueOutput annotation to write a message as the output of a function.
The type of binding is either input or output, and not all services support both input and output bindings. For instance, the Azure Storage queue binding is an output binding that allows you to write messages to a queue.
Here are some common types of bindings used in Azure Functions:
- QueueOutput: used to write messages to a queue
- QueueTrigger: used to trigger a function when a message is received in a queue
- HttpTrigger: used to trigger a function when an HTTP request is received
These bindings can be configured using various properties, such as the queue name, connection string, and parameter name. For example, the QueueTrigger annotation gives you access to the queue that triggers the function, and the QueueOutput annotation allows you to write a message as the output of a function.
By using bindings, you can connect your Azure Functions to various services and triggers, making it easier to build and deploy scalable and efficient applications.
Next Steps
Now that you've set up your Azure Function Queue Trigger, it's time to think about what happens next.
One key step is to run a function as queue storage data changes. This is a trigger that will fire whenever there's new data in your queue.
To take your Azure Function Queue Trigger to the next level, consider the following steps:
- Run a function as queue storage data changes (Trigger)
By following these steps, you'll be well on your way to creating a robust and efficient Azure Function Queue Trigger system.
Frequently Asked Questions
What is queue trigger in Azure Function?
The queue trigger in Azure Function runs a function as messages are added to Azure Queue storage, allowing for automatic execution of tasks. This is achieved through target-based scaling, which optimizes resource utilization.
What is the timeout for Azure Function queue trigger?
Azure Function queue triggers have a timeout of 5-10 minutes, similar to other consumption model functions. However, the HTTP trigger has a specific timeout of 230 seconds, which cannot be changed.
What is the trigger for message queue?
A trigger for message queue is a mechanism that forwards messages from the queue to a Cloud Functions function for processing, reducing the load on the queue. It acts as a messenger, picking up messages and sending them for further handling.
What is the batch size of Azure Function storage queue trigger?
The batch size for Azure Function storage queue triggers is 32, allowing for parallel processing of up to 32 messages at a time. This setting determines the maximum number of messages retrieved and processed simultaneously.
Sources
- https://learn.microsoft.com/en-us/azure/azure-functions/functions-bindings-storage-queue-trigger
- https://keda.sh/docs/2.13/scalers/azure-storage-queue/
- https://www.sqlshack.com/triggering-function-apps-from-azure-queue-storage/
- https://learn.microsoft.com/en-us/azure/azure-functions/functions-bindings-storage-queue-output
- https://learn.microsoft.com/en-us/azure/azure-functions/functions-triggers-bindings
Featured Images: pexels.com