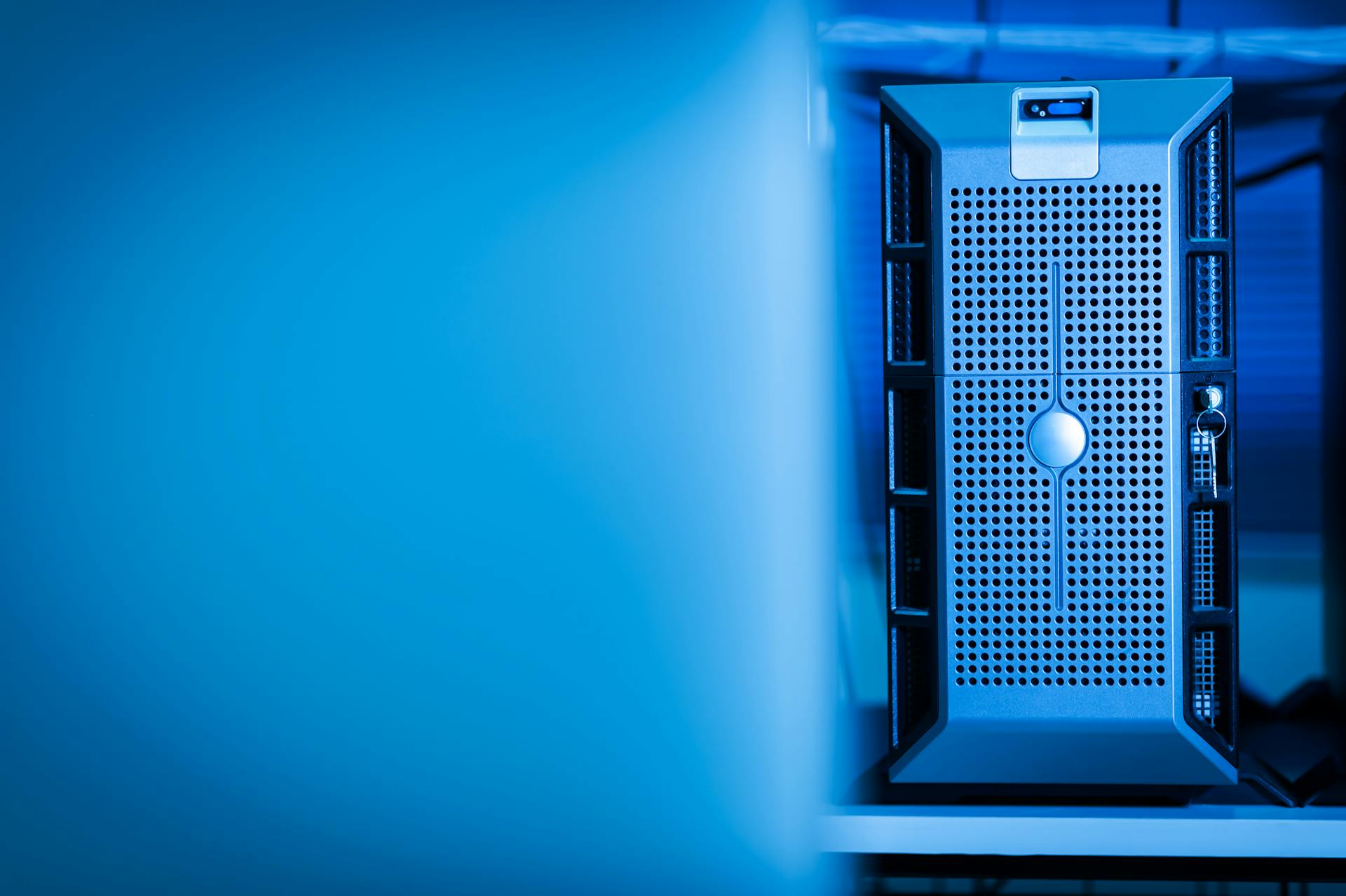
To start using Azure OpenTelemetry Python, you'll need to install the OpenTelemetry SDK and the Azure Monitor SDK.
You can do this by running pip install opentelemetry-sdk and pip install azure-monitor-sdk-python in your terminal.
First, import the necessary libraries in your Python code, including opentelemetry.sdk.trace and opentelemetry.sdk.metrics.
Make sure to initialize the OpenTelemetry SDK with a tracer provider, such as opentelemetry.sdk.trace.tracer_provider.get_tracer_provider().
Prerequisites
To get started with Azure OpenTelemetry Python, you'll need to meet some basic prerequisites.
First and foremost, you'll need an Azure subscription, which is free to create. You can sign up for one in just a few minutes.
You'll also need to have Azure Monitor set up, specifically application insights, which will help you understand how your application is performing.
To collect and export telemetry data, you'll need to install the OpenTelemetry SDK for Python.
Finally, you'll need to have Python 3.8 or later installed on your machine.
Configuration
Configuration is a crucial aspect of Azure OpenTelemetry Python. You can access OpenTelemetry configurations through environment variables while using the Azure Monitor OpenTelemetry Distros.
The APPLICATIONINSIGHTS_CONNECTION_STRING environment variable sets the connection string for your Application Insights resource. This is the same connection string used to connect to Application Insights without OpenTelemetry enabled.
The APPLICATIONINSIGHTS_STATSBEAT_DISABLED environment variable can be set to true to opt out of internal metrics collection.
You can also pass key-value pairs as resource attributes using the OTEL_RESOURCE_ATTRIBUTES environment variable. Resource attributes are used to provide additional context to your telemetry data.
For Spring Boot native applications, the OpenTelemetry Java SDK configurations are available. You can find more information about OpenTelemetry SDK configuration in the OpenTelemetry documentation.
Here are some configurable options for the OpenTelemetry Python exporter:
You can also configure application settings in your function app based on the OpenTelemetry output destination. This includes settings for Application Insights and OTLP Exporter.
For example, you can set the APPLICATIONINSIGHTS_CONNECTION_STRING environment variable to the connection string for an Application Insights workspace. You can also set the OTEL_EXPORTER_OTLP_ENDPOINT environment variable to an OTLP exporter endpoint URL.
Remember to remove the APPLICATIONINSIGHTS_CONNECTION_STRING setting unless you also want OpenTelemetry output from the host sent to Application Insights.
Instrumentation
Instrumentation is a key feature of OpenTelemetry, allowing you to automatically collect requests sent from underlying instrumented libraries.
To instrument your Python application with OpenTelemetry, you can use the requests instrumentation package, which can be installed using pip install opentelemetry-instrumentation-requests.
OpenTelemetry also comes with several officially supported instrumentations, including ones for Azure Core Tracing, Django, FastAPI, Flask, and more. These instrumentations are enabled by default in the Azure Monitor distro.
Here's a list of some of the officially supported OpenTelemetry instrumentations:
Instrumentation with Requests
You can instrument with the requests library using OpenTelemetry.
The requests instrumentation package can be installed using pip with the command `pip install opentelemetry-instrumentation-requests`.
To use the requests instrumentation, you'll need to install the OpenTelemetry instrumentation package specifically designed for requests.
This instrumentation will automatically collect requests sent from the underlying requests library.
Telemetry Processors
In OpenCensus Python, telemetry processors allow users to modify their telemetry before it's sent to the exporter. This is a powerful mechanism that enables customization of telemetry data.
There's no direct equivalent in OpenTelemetry, but you can replicate the same behavior using APIs and classes.
Usage
You can use the `configure_azure_monitor` function to set up instrumentation for your app to Azure Monitor. This function supports several optional arguments that you can pass in to customize the configuration.
The `connection_string` parameter is required to set up instrumentation for your app to Azure Monitor. If you don't explicitly pass in a connection string, it will be automatically populated from the `APPLICATIONINSIGHTS_CONNECTION_STRING` environment variable.
You can also enable or disable specific instrumentations using the `instrumentation_options` parameter. This parameter is a nested dictionary that determines which instrumentations to enable or disable. For example, you can disable Azure Core Tracing and the Flask instrumentation but leave Django and the other default instrumentations enabled.
Here's a table summarizing the optional arguments supported by `configure_azure_monitor`:
You can also configure further with OpenTelemetry environment variables. These environment variables allow you to customize the behavior of the SDK and control what data is collected and exported.
Export and Storage
Azure Monitor OpenTelemetry-based offerings write to offline/local storage by default when an application loses its connection with Application Insights.
The allowable time for sending telemetry is up to 48 hours, after which it's occasionally dropped.
To override the default directory for offline storage, you should set AzureMonitorOptions.StorageDirectory or AzureMonitorExporterOptions.StorageDirectory.
The AzureMonitorExporter uses one of the following locations for offline storage, listed in order of precedence: the directory specified by AzureMonitorOptions.StorageDirectory, the directory specified by AzureMonitorExporterOptions.StorageDirectory, or the default directory.
To disable offline storage, you should set AzureMonitorOptions.DisableOfflineStorage or AzureMonitorExporterOptions.DisableOfflineStorage to true.
You can also disable offline storage by setting disableOfflineStorage to true or disable_offline_storage to True.
The Azure Monitor OpenTelemetry Distro comes with some popular OpenTelemetry Python instrumentations out of the box, so no extra code is necessary.
The distro includes the AzureMonitorExporter, which you can use with other exporters like this example.
Troubleshooting
When working with Azure OpenTelemetry Python, it's essential to know how to troubleshoot issues that arise.
The exporter raises exceptions defined in Azure Core. This means you'll need to be aware of these exceptions and how to handle them.
To resolve issues efficiently, familiarize yourself with the exceptions and error messages. This will enable you to quickly identify and address problems.
Troubleshooting
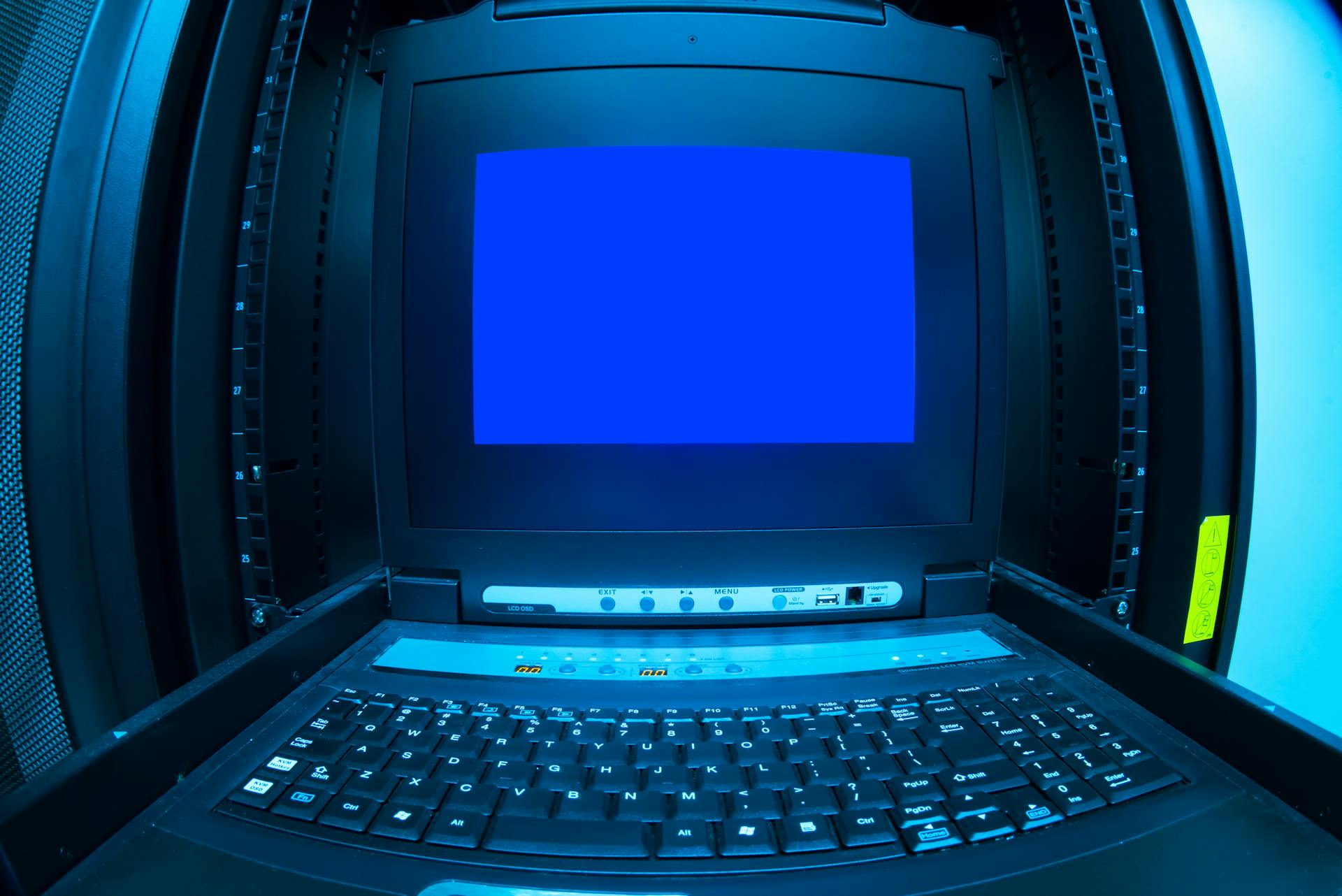
Troubleshooting can be a challenge, but understanding the basics can make all the difference. The exporter raises exceptions defined in Azure Core.
In many cases, the root of the issue lies in how we handle exceptions. The exporter raises exceptions defined in Azure Core.
To effectively troubleshoot, it's essential to identify the specific exception being raised. Azure Core provides a robust framework for handling exceptions.
When you're dealing with complex systems, it's easy to get lost in the details. The exporter raises exceptions defined in Azure Core.
Taking a step back and examining the bigger picture can help you pinpoint the problem more quickly. The exporter raises exceptions defined in Azure Core.
By following these principles, you can develop a systematic approach to troubleshooting that saves time and reduces frustration.
Changes and Limitations
As you start troubleshooting with OpenTelemetry, it's essential to be aware of the changes and limitations that might affect your experience.
Migrating from OpenCensus to OpenTelemetry can be a bit tricky, and you might encounter some changes along the way.

Only logs and traces are exported when the host is configured to use OpenTelemetry, so you won't see host metrics in your data.
You can't run your app project locally using Core Tools when OpenTelemetry is enabled in the host, so be prepared to deploy your code to Azure for testing.
Currently, only HTTP trigger and Azure SDK-based triggers are supported with OpenTelemetry outputs, so make sure to check your trigger types before proceeding.
Key Concepts
OpenTelemetry is a set of libraries used to collect and export telemetry data (metrics, logs, and traces) for analysis. This data helps you understand your software's performance and behavior.
Instrumentation is the ability to call the OpenTelemetry API directly by any application, and a library that enables OpenTelemetry observability for another library is called an instrumentation library.
Here's a brief overview of the key concepts in OpenTelemetry:
Sampling is a mechanism to control the noise and overhead introduced by OpenTelemetry by reducing the number of samples of traces collected and sent to the backend.
Key Concepts
OpenTelemetry is a set of libraries used to collect and export telemetry data for analysis. This data includes metrics, logs, and traces, which help understand your software's performance and behavior.
OpenTelemetry uses instrumentation to facilitate direct access to its API. Instrumentation libraries enable OpenTelemetry observability for other libraries.
A log refers to capturing logging, exception, and events. Log records represent a log record emitted from a supported logging library.
Logger providers offer a logger for a given instrumentation library. Log record processors hook the log record emitting action.
Logging handlers write logging records in OpenTelemetry format from the standard Python logging library. AzureMonitorLogExporter sends logging related telemetry to Azure Monitor.
Metrics record raw measurements with predefined aggregation and sets of attributes for a period in time. Measurements represent a data point recorded at a point in time.
Instruments report measurements. Meters create instruments. Meter providers offer a meter for a given instrumentation library.
AzureMonitorMetricExporter sends metric related telemetry to Azure Monitor. Metric readers provide common configurable aspects of the OpenTelemetry Metrics SDK.
Traces represent distributed tracing, a set of events consolidated across various components of an application. A trace can be thought of as a directed acyclic graph (DAG) of spans.
Spans represent a single operation within a trace. Tracers create spans. Tracer providers offer a tracer for use by a given instrumentation library.
Span processors hook SDK span start and end method invocations. AzureMonitorTraceExporter sends tracing related telemetry to Azure Monitor.
Sampling controls noise and overhead by reducing the number of trace samples collected and sent to the backend. ApplicationInsightsSampler is used for consistent sampling across Application Insights SDKs and OpenTelemetry-based SDKs.
Cohesion
Cohesion is crucial in distributed tracing, allowing you to connect the dots between different parts of your system.
In Azure Functions, you can use the package opencensus-extension-azure-functions to create a connected distributed graph.
This package was provided to address a specific use case where Python applications call other Python applications within an Azure function.
Currently, OpenTelemetry solutions for Azure Monitor don't support this scenario, so you might need to rely on workarounds.
Manually propagating the trace context in your Azure functions application is one such workaround, as shown in an example.
Sources
- https://azure.github.io/azure-sdk/releases/latest/python.html
- https://learn.microsoft.com/en-us/azure/azure-monitor/app/opentelemetry-configuration
- https://learn.microsoft.com/en-us/python/api/overview/azure/monitor-opentelemetry-exporter-readme
- https://learn.microsoft.com/en-us/azure/azure-functions/opentelemetry-howto
- https://learn.microsoft.com/en-us/python/api/overview/azure/monitor-opentelemetry-readme
- https://learn.microsoft.com/en-us/azure/azure-monitor/app/opentelemetry-python-opencensus-migrate
Featured Images: pexels.com